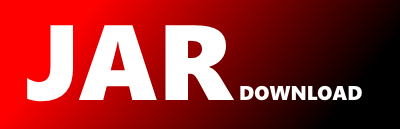
org.fuchss.objectcasket.tablemodule.impl.TransactionImpl Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of object-casket Show documentation
Show all versions of object-casket Show documentation
Object Casket is a simple O/R mapper that can be used together with the Java Persistence API (JPA). The aim is to provide a simple solution for small projects to store multi-related
entities in a simple manner.
package org.fuchss.objectcasket.tablemodule.impl;
import java.util.HashMap;
import java.util.HashSet;
import java.util.Map;
import java.util.Set;
import org.fuchss.objectcasket.common.CasketError.CE4;
import org.fuchss.objectcasket.common.CasketException;
class TransactionImpl {
protected Map> created = new HashMap<>();
protected Map> deleted = new HashMap<>();
protected Map> changed = new HashMap<>();
protected Object voucher;
public TransactionImpl(Object obj) {
this.voucher = obj;
}
protected void add2created(TableImpl tab, RowImpl row) {
Set createdRows = this.created.computeIfAbsent(tab, k -> new HashSet<>());
createdRows.add(row);
}
protected void add2deleted(TableImpl tab, RowImpl row) {
Set createdRows = this.created.get(tab);
Set changedRows = this.changed.get(tab);
Set deletedRows = this.deleted.computeIfAbsent(tab, k -> new HashSet<>());
if (createdRows != null)
createdRows.remove(row);
if (changedRows != null)
changedRows.remove(row);
deletedRows.add(row);
}
protected void add2changed(TableImpl tab, RowImpl row) {
Set createdRows = this.created.get(tab);
if ((createdRows != null) && createdRows.contains(row))
return;
Set changedRows = this.changed.computeIfAbsent(tab, k -> new HashSet<>());
changedRows.add(row);
}
protected void rollbackCreated(Object voucher) throws CasketException {
if (this.voucher != voucher)
throw CE4.UNKNOWN_MANAGED_OBJECT.defaultBuild("Voucher", voucher, this.getClass(), this);
this.created.forEach((tab, rows) -> tab.rollback(rows));
this.voucher = null;
}
protected void done() {
this.done(this.created);
this.done(this.changed);
this.done(this.deleted);
}
private void done(Map> map) {
for (Set rows : map.values()) {
if (rows == null)
continue;
rows.forEach(RowImpl::done);
}
this.voucher = null;
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy