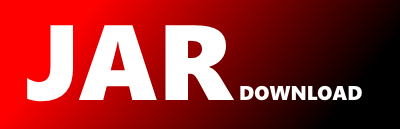
org.fudaa.dodico.rubar.io.RubarStReader Maven / Gradle / Ivy
/*
* @creation 4 d?c. 06
* @license GNU General Public License 2
* @copyright (c)1998-2001 CETMEF 2 bd Gambetta F-60231 Compiegne
* @mail [email protected]
*/
package org.fudaa.dodico.rubar.io;
import java.io.EOFException;
import java.io.File;
import java.io.IOException;
import java.util.ArrayList;
import java.util.List;
import org.locationtech.jts.geom.Coordinate;
import org.locationtech.jts.geom.LineString;
import org.fudaa.ctulu.CtuluLib;
import org.fudaa.ctulu.CtuluLibString;
import org.fudaa.ctulu.gis.GISAttributeConstants;
import org.fudaa.ctulu.gis.GISAttributeInterface;
import org.fudaa.ctulu.gis.GISAttributeModel;
import org.fudaa.ctulu.gis.GISLib;
import org.fudaa.ctulu.gis.GISZoneCollectionLigneBrisee;
import org.fudaa.dodico.fortran.FileOpReadCharSimpleAbstract;
/**
* Un reader pour un fichier Rubar (.st qui contient des profils, ou .cn qui contient des courbes de niveau). Un fichier .m
* est identique en lecture ? un fichier .st.
*
* @author fred deniger, Bertrand Marchand
* @version $Id$
*/
public class RubarStReader extends FileOpReadCharSimpleAbstract {
/** Le fichier est de type .st. */
boolean isSt_;
public RubarStReader() {
super();
}
@Override
public void setFile(final File _f) {
isSt_ = !_f.getName().toLowerCase().endsWith(".cn");
super.setFile(_f);
}
final String sep_ = "999.9990";
final String sep1_ = "999.999";
public boolean isSep(final String _s) {
return _s.equals(sep_) || _s.equals(sep1_);
}
/**
* Lit les profils et les lignes directrices.
* @return Un tableau GISZoneCollectionLigneBrisee[2]. [0] : la collection des profils (ou null si aucun profil),
* [1] : la collection de lignes directrices (ou null si aucune ligne directrice).
*/
@Override
protected GISZoneCollectionLigneBrisee[] internalRead() {
// Remarque : Cette impl?mentation empeche des noms semblables (les noms sont stock?s comme cl? de Map) sur les lignes
// directrices. Ce qui peut ?tre normal puisque c'est le m?me nom en un point de chaque profil qui permet de constituer
// la ligne.
if (in_ == null) {
return null;
}
// Vrai si les points dupliqu? doivent le rester.
boolean keepDuplicatePoints=true;
in_.setJumpBlankLine(true);
in_.setBlankZero(true);
in_.setCommentInOneField("#");
in_.setJumpCommentLine(true);
final List lignes = new ArrayList(200);
final List names=new ArrayList(200);
final List pks=new ArrayList(200);
final List coordinatesEnCours = new ArrayList();
// Listes des coordonn?es pour les lignes directrices
final List> lcoordldir=new ArrayList>(20);
// Liste des noms des lignes directrices
final List lnameldirs=new ArrayList(20);
final int[] fmt = new int[] { 13, 13, 13, 1, 3 };
final int[] fmt1Line=new int[]{6,6,6,6,13,1,42};
Coordinate last = null;
String name="";
String pk="";
try {
while (true) {
if (isSt_) {
in_.readFields(fmt1Line);
name=in_.stringField(6).trim();
pk=GISAttributeConstants.ATT_COMM_HYDRO_PK+"="+in_.stringField(4).trim();
}
String first = CtuluLibString.EMPTY_STRING;
String sec = CtuluLibString.EMPTY_STRING;
in_.readFields(fmt);
while (!isSep(first) && !isSep(sec)) {
Coordinate coordinate = new Coordinate(in_.doubleField(0), in_.doubleField(1), in_.doubleField(2));
// dans les fichier st, il se peut qu'il y ait des points en double, donc on fait ce test:
if (last == null || keepDuplicatePoints || !last.equals2D(coordinate)) {
coordinatesEnCours.add(coordinate);
last = coordinate;
}
// Ligne directrice eventuelle. Pas de controle que la ligne directrice est bien sur tous les profils.
String namedir=in_.stringField(4).trim();
if (!"".equals(namedir)) {
List coordldir;
int ind=lnameldirs.indexOf(namedir);
if (ind==-1) {
coordldir=new ArrayList(200);
lnameldirs.add(namedir);
lcoordldir.add(coordldir);
}
else {
coordldir=lcoordldir.get(ind);
}
coordldir.add((Coordinate) last.clone());
}
in_.readFields(fmt);
first = in_.stringField(0);
sec = in_.stringField(1);
}
final LineString str = GISLib.createLineOrLinearFromList(coordinatesEnCours);
if (str != null) {
lignes.add(str);
pks.add(pk);
// Le nom par d?faut correspond ? la position de la g?om?trie dans le fichier.
if ("".equals(name)) {
names.add(CtuluLib.getS(isSt_?"P":"N")+(names.size()+1));
}
else {
names.add(name);
}
}
coordinatesEnCours.clear();
}
}
catch (final EOFException _io) {}
catch (final IOException _io) {
analyze_.manageException(_io);
}
catch (final NumberFormatException _if) {
analyze_.manageException(_if, in_.getLineNumber());
}
final LineString str = GISLib.createLineOrLinearFromList(coordinatesEnCours);
if (str != null) {
lignes.add(str);
if ("".equals(name)) {
names.add(CtuluLib.getS(isSt_?"P":"N")+(names.size()+1));
}
else {
names.add(name);
}
}
GISZoneCollectionLigneBrisee[] ret=new GISZoneCollectionLigneBrisee[2];
// Profils / Lignes de niveaux
if (lignes.size() > 0) {
final GISZoneCollectionLigneBrisee zligs = new GISZoneCollectionLigneBrisee(null);
zligs.addAllLineStringClosedOrNode((LineString[]) lignes.toArray(new LineString[lignes.size()]), null);
GISAttributeInterface[] attrs;
if (isSt_)
attrs=new GISAttributeInterface[] {
GISAttributeConstants.BATHY, GISAttributeConstants.TITRE,
GISAttributeConstants.NATURE, GISAttributeConstants.COMMENTAIRE_HYDRO};
else
attrs=new GISAttributeInterface[] {
GISAttributeConstants.BATHY, GISAttributeConstants.TITRE,
GISAttributeConstants.NATURE};
zligs.setAttributes(attrs, null);
if(zligs.getAttributeIsZ()==null)
zligs.setAttributeIsZ(GISAttributeConstants.BATHY);
zligs.postImport(0);
// Affectation de l'attribut titre.
GISAttributeModel attmod=zligs.getModel(GISAttributeConstants.TITRE);
for (int i=0; i0) {
final GISZoneCollectionLigneBrisee zdirs = new GISZoneCollectionLigneBrisee(null);
// Pour ?tre sur de la correspondance nom/coordonn?es
String[] namedirs=lnameldirs.toArray(new String[0]);
LineString[] ldirs=new LineString[namedirs.length];
for (int i=0; i
© 2015 - 2025 Weber Informatics LLC | Privacy Policy