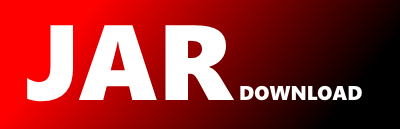
org.fudaa.ebli.graphe.Axe Maven / Gradle / Ivy
The newest version!
/**
* @creation 1999-07-29
* @modification $Date: 2007-06-05 08:58:38 $
* @license GNU General Public License 2
* @copyright (c)1998-2001 CETMEF 2 bd Gambetta F-60231 Compiegne
*/
package org.fudaa.ebli.graphe;
import java.awt.Color;
import java.awt.Font;
import java.awt.FontMetrics;
import java.awt.Graphics2D;
import java.text.DecimalFormat;
import java.util.ArrayList;
import java.util.List;
import com.memoire.fu.FuLog;
import org.fudaa.ctulu.CtuluLibString;
/**
* @version $Id: Axe.java,v 1.18 2007-06-05 08:58:38 deniger Exp $
* @author Guillaume Desnoix
*/
public class Axe {
public final static boolean DEBUG = BGraphe.DEBUG;
public String titre_;
public boolean visible_;
public String unite_;
public boolean vertical_;
public boolean graduations_;
/* ajout de benmaneu pour convertir un axe en heure minute */
public boolean conversionHM_;
/*---fin ajout benmaneu--*/
public boolean grille_;
public Font font_;
/**
* Ecart entre les axes verticaux.
*/
public int ecart_;
public double minimum_;
public double maximum_;
/**
* Le pas entre les marques.
*/
public double pas_;
public List etiquettes_;
public Aspect aspect_;
public Axe() {
titre_ = "";
visible_ = true;
unite_ = "";
vertical_ = false;
graduations_ = false;
/* ajout de benmaneu pour convertir un axe en heure minute */
conversionHM_ = false;
/*---fin ajout benmaneu--*/
grille_ = false;
ecart_ = 50;
minimum_ = 0.;
maximum_ = 10.;
pas_ = 0.;
etiquettes_ = new ArrayList(1);
aspect_ = new Aspect();
aspect_.contour_ = Color.black;
}
public static Axe parse(final Lecteur _lin) {
final Axe r = new Axe();
String t = _lin.get();
if (t.equals("axe")) {
t = _lin.get();
}
if (!t.equals("{")) {
FuLog.error("Erreur de syntaxe: " + t);
}
t = _lin.get();
while (!t.equals("") && !t.equals("}")) {
final String oui = "oui";
if (t.equals("titre")) {
r.titre_ = _lin.get();
} else if (t.equals("unite")) {
r.unite_ = _lin.get();
} else if (t.equals("orientation")) {
r.vertical_ = _lin.get().equals("vertical");
} else if (t.equals("graduations")) {
r.graduations_ = _lin.get().equals(oui);
} else if (t.equals("conversionHM")) {
r.conversionHM_ = true;
} else if (t.equals("grille")) {
r.grille_ = _lin.get().equals(oui);
} else if (t.equals("ecart")) {
try {
r.ecart_ = Integer.parseInt(_lin.get());
} catch (final NumberFormatException e) {}
} else if (t.equals("minimum")) {
r.minimum_ = Double.valueOf(_lin.get()).doubleValue();
} else if (t.equals("maximum")) {
r.maximum_ = Double.valueOf(_lin.get()).doubleValue();
} else if (t.equals("pas")) {
r.pas_ = Double.valueOf(_lin.get()).doubleValue();
} else if (t.equals("etiquettes")) {
r.etiquettes_ = Etiquette.parse(_lin);
} else if (t.equals("visible")) {
r.visible_ = _lin.get().equals(oui);
} else if (t.equals("aspect")) {
r.aspect_ = Aspect.parse(_lin);
} else {
System.err.println("Erreur de syntaxe: " + t);
}
t = _lin.get();
}
return r;
}
public boolean containsPoint(final double _x) {
return (_x <= maximum_) && (_x >= minimum_);
}
public void dessine(final Graphics2D _g, final int _x, final int _y, final int _w, final int _h) {
if (!visible_) {
return;
}
final Font old = _g.getFont();
if (font_ != null) {
_g.setFont(font_);
}
final FontMetrics fm = _g.getFontMetrics();
String t;
if (pas_ == 0.) {
pas_ = Math.ceil(Math.log(maximum_ - minimum_) / Math.log(10.) - 1.35); // 1.35 pour avoir 22 pas au maximum (lisibilit?)
pas_ = Math.pow(10., pas_);
if ((maximum_ - minimum_) / pas_ < 8.) pas_ = pas_ / 2.; // on double le nombre de pas s'il y en a trop peu
}
// BUG: il arrive que pas ressorte avec la valeur 0, d'ou boucle infinie
// dans le for qui suit!!
// -> hack temporaire
if (pas_ == 0.) {
pas_ = 1.;
}
maximum_ = Math.ceil(maximum_/pas_)*pas_;
minimum_ = Math.floor(minimum_/pas_)*pas_;
_g.setColor(aspect_.contour_);
final int rc = aspect_.contour_.getRed();
final int gc = aspect_.contour_.getGreen();
final int bc = aspect_.contour_.getBlue();
final int incr = Math.max(Math.max((200 - rc), (200 - gc)), (200 - bc));
final Color lightfg = new Color(Math.min(255, rc + incr / 5), Math.min(255, gc + incr / 5), Math.min(255, bc + incr / 5));
final Color superlightfg = new Color(Math.min(255, rc + incr), Math.min(255, gc + incr), Math.min(255, bc + incr));
dessineGraduations(_g, _x, _y, _w, _h, fm, lightfg, superlightfg);
t = titre_;
if (!unite_.equals("")) {
t += " [" + unite_ + "]";
}
_g.setColor(aspect_.contour_);
if (vertical_) {
_g.drawLine(_x - 1, _y, _x - 1, _y + _h - 1);
_g.drawLine(_x - 1, _y, _x - 3, _y + 2);
_g.drawLine(_x - 1, _y, _x + 1, _y + 2);
_g.drawString(t, _x - fm.stringWidth(t), _y - 6);
} else {
_g.drawLine(_x, _y, _x + _w - 1, _y);
_g.drawLine(_x + _w - 1, _y, _x + _w - 3, _y - 2);
_g.drawLine(_x + _w - 1, _y, _x + _w - 3, _y + 2);
_g.drawString(t, _x + _w + 3, _y + 2);
}
for (int i = 0; i < etiquettes_.size(); i++) {
final Etiquette o = (Etiquette) etiquettes_.get(i);
if (vertical_) {
final int ye = _y + _h - (int) (_h * ((o.s_ - minimum_) / (maximum_ - minimum_)));
_g.drawLine(_x - 2, ye, _x, ye);
_g.drawString(o.titre_, _x + 2, ye + 4);
} else {
final int xe = _x + (int) (_w * ((o.s_ - minimum_) / (maximum_ - minimum_)));
_g.drawLine(xe, _y - 1, xe, _y + 1);
_g.drawString(o.titre_, xe - fm.stringWidth(o.titre_) / 2, _y - 5);
}
}
_g.setFont(old);
}
private void dessineGraduations(final Graphics2D _g, final int _x, final int _y, final int _w, final int _h, final FontMetrics _fm, final Color _lightfg,
final Color _superlightfg) {
String t;
if (graduations_ || grille_) {
final java.text.NumberFormat nf = new DecimalFormat("0.##");
nf.setGroupingUsed(false);
if (vertical_) {
final double asc = _fm.getAscent();
final double fontHeight = _fm.getDescent() + asc;
final double xLeft = _x - 2;
final double yBas = _y + _h;
final double yCoef = -(double) _h / (maximum_ - minimum_);
boolean first = true;
// stocke le max du dernier champ ecrit. Evite aux graduations de se chevaucher
double maxLastIteration = Double.MAX_VALUE;
for (double s = minimum_; s <= maximum_; s += pas_) {
_g.setColor(_lightfg);
final int ye = (int) (yBas + (s - minimum_) * yCoef);
if (graduations_) {
_g.drawLine((int) xLeft, ye, _x, ye);
t = nf.format(s);
if (first) {
// le premier est dessine en haut pour eviter de chevaucher avec l'axe horizontal
_g.drawString(t, (int) (xLeft - _fm.stringWidth(t)), ye);
maxLastIteration = ye - fontHeight;
first = false;
} else if (maxLastIteration >= ye) {
if (conversionHM_) {
double t2 = Double.parseDouble(t.replace(',', '.'));
t2 = t2 * 3600;
final int val = (int) t2;
_g.drawString(determineHeureString(val), (int) (xLeft - _fm.stringWidth(t)), (int) (ye + asc / 2));
maxLastIteration = ye - fontHeight;
}
/** fin modif benmaneu * */
else {
_g.drawString(t, (int) (xLeft - _fm.stringWidth(t)), (int) (ye + asc / 2));
maxLastIteration = ye - fontHeight;
}
}
}
if ((s > minimum_) && grille_) {
_g.setColor(_superlightfg);
_g.drawLine(_x, ye, _x + _w, ye);
}
}
} else {
final double xCoef = _w / (maximum_ - minimum_);
final double yBas = _y + 2d;
double lastMaxX = -10;
final double ftHaut = _fm.getMaxAscent() + 5;
for (double s = minimum_; s <= maximum_; s += pas_) {
_g.setColor(_lightfg);
final int xe = (int) (_x + xCoef * (s - minimum_));
if (graduations_) {
_g.drawLine(xe, _y, xe, (int) yBas);
t = nf.format(s);
final double wordWidth = _fm.stringWidth(t);
final double xLeft = xe - wordWidth / 2;
// pour eviter que les graduations se chevauchent
//if (xLeft > (lastMaxX + 2)) {
/** modif benmaneu * */
if (conversionHM_) {
double t2 = Double.parseDouble(t.replace(',', '.'));
t2 = t2 * 3600;
final int val = (int) t2;
_g.drawString(determineHeureString(val), (int) xLeft, (int) (_y + ftHaut));
lastMaxX = xLeft + wordWidth;
} else {
_g.drawString(t, (int) xLeft, (int) (_y + ftHaut));
lastMaxX = xLeft + wordWidth;
}
if (xLeft > (lastMaxX + 2)) {
_g.drawString(t, (int) xLeft, (int) (_y + ftHaut));
lastMaxX = xLeft + wordWidth;
/** fin modif benmaneu * */
}
}
if ((s > minimum_) && grille_) {
_g.setColor(_superlightfg);
_g.drawLine(xe, _y, xe, _y - _h);
}
}
}
}
}
/**
*
*/
public String getTitre() {
return titre_;
}
/**
*
*/
public boolean isVisible() {
return visible_;
}
public static String determineHeureString(final int _nbSecondes) {
/*
* String s2=""+_nbSecondes; int nbS=Integer.parseInt(s2);
*/
final int heure = _nbSecondes / 3600;
final int minute = _nbSecondes / 60 - heure * 60;
String s = "";
if (minute > 9) {
s = heure + CtuluLibString.VIR + minute;
} else {
s = heure + ",0" + minute;
}
return s;
}
/** fin ajout benmaneu* */
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy