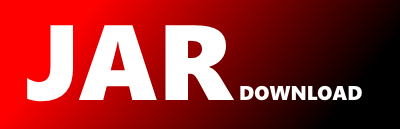
org.fudaa.ebli.graphe.BGraphe Maven / Gradle / Ivy
The newest version!
/**
* @creation 24/02/99
* @modification $Date: 2007-02-13 08:00:59 $
* @license GNU General Public License 2
* @copyright (c)1998-2001 CETMEF 2 bd Gambetta F-60231 Compiegne
*/
package org.fudaa.ebli.graphe;
import java.awt.*;
import java.awt.event.ComponentEvent;
import java.awt.event.ComponentListener;
import java.awt.geom.Point2D;
import java.awt.image.BufferedImage;
import java.awt.print.PageFormat;
import java.awt.print.Printable;
import java.io.InputStream;
import java.io.InputStreamReader;
import java.io.Reader;
import java.text.NumberFormat;
import java.util.ArrayList;
import java.util.Arrays;
import java.util.List;
import java.util.Map;
import javax.swing.JComponent;
import com.memoire.bu.BuInformationsDocument;
import com.memoire.bu.BuInformationsSoftware;
import com.memoire.bu.BuPrinter;
import com.memoire.bu.BuResource;
import com.memoire.fu.Fu;
import com.memoire.fu.FuLog;
import org.fudaa.ctulu.CtuluLibImage;
import org.fudaa.ctulu.CtuluLibMessage;
import org.fudaa.ctulu.CtuluLibString;
import org.fudaa.ctulu.image.CtuluImageProducer;
import org.fudaa.ebli.impression.EbliPrinter;
/**
* Un composant pour afficher les graphes et pour les imprimer.
*
* @version $Id: BGraphe.java,v 1.22 2007-02-13 08:00:59 deniger Exp $
* @author Axel von Arnim
*/
public class BGraphe extends JComponent implements ComponentListener, CtuluImageProducer {
/**
* Si true
, affiche des infos utiles au debugage.
*/
public static final boolean DEBUG = false;
/**
* le Graphe.
*/
protected Graphe graphe_;
/**
* Graphe interactif.
*/
boolean interactif_;
/**
* L'image servant de cache et evitant le recalcul du graphique.
*/
Image cache_;
GrapheInteractionSuiviSouris suiviSouris_;
/**
* Le rectangle d'impression.
*/
// private Rectangle clipPrec_;
private transient BGrapheInteraction interaction_;
public BGraphe() {
super();
graphe_ = null;
interaction_ = null;
// clipPrec_ = new Rectangle();
addComponentListener(this);
}
public GrapheInteractionSuiviSouris activeSuiviSouris() {
if (suiviSouris_ == null) {
suiviSouris_ = new GrapheInteractionSuiviSouris(this);
addMouseMotionListener(suiviSouris_);
}
return suiviSouris_;
}
public void unactiveSuiviSouris() {
if (suiviSouris_ != null) {
removeMouseMotionListener(suiviSouris_);
suiviSouris_ = null;
}
}
public boolean versReel(final double _vx, double _vy, final Point2D _p) {
if (graphe_ == null) {
return false;
}
final Marges m = graphe_.marges_;
Axe a = graphe_.getHorizontalAxe();
final double x = (_vx - m.gauche_) * (a.maximum_ - a.minimum_) / (getWidth() - m.gauche_ - m.droite_) + a.minimum_;
a = graphe_.getFirstVerticalAxe();
final double y = (-_vy + getHeight() - m.bas_) * (a.maximum_ - a.minimum_) / (getHeight() - m.bas_ - m.haut_)
+ a.minimum_;
_p.setLocation(x, y);
return true;
}
public BuInformationsDocument getInformationsDocument() {
final BuInformationsDocument id = new BuInformationsDocument();
if (graphe_ != null) {
id.name = graphe_.titre_;
}
return id;
}
public BuInformationsSoftware getInformationsSoftware() {
final BuInformationsSoftware is = new BuInformationsSoftware();
is.name = "inconnu";
return is;
}
/**
* Affecte la valeur Graphe de BGraphe object.
*
* @param _gr La nouvelle valeur Graphe
*/
public void setGraphe(final Graphe _gr) {
if (_gr == graphe_) {
return;
}
graphe_ = _gr;
fullRepaint();
}
/**
* Affecte la valeur Interactif de BGraphe object.
*
* @param _i La nouvelle valeur Interactif
*/
public void setInteractif(final boolean _i) {
interactif_ = _i;
if (_i) {
if (interaction_ == null) {
interaction_ = new BGrapheInteraction(this);
}
addMouseListener(interaction_);
addMouseMotionListener(interaction_);
} else {
if (interaction_ != null) {
removeMouseListener(interaction_);
removeMouseMotionListener(interaction_);
}
interaction_ = null;
}
}
public void setFluxDonnees(final InputStream _data) {
setFluxDonnees(new InputStreamReader(_data));
}
/**
* Affecte la valeur FluxDonnees de BGraphe object.
*
* @param _data La nouvelle valeur FluxDonnees
*/
public void setFluxDonnees(final Reader _data) {
final Lecteur lin = new Lecteur(_data);
String t = lin.get();
while (!t.equals("")) {
if (t.equals("graphe")) {
graphe_ = Graphe.parse(lin, null);
} else {
FuLog.error("Erreur de syntaxe: " + t);
}
t = lin.get();
}
fullRepaint();
}
/**
* retourne la valeur Graphe de BGraphe object.
*
* @return La valeur Graphe
*/
public Graphe getGraphe() {
return graphe_;
}
/**
* retourne la valeur Interactif de BGraphe object.
*
* @return La valeur Interactif
*/
public boolean isInteractif() {
return interactif_;
}
/**
* retourne la valeur ImageCache de BGraphe object.
*
* @return La valeur ImageCache
*/
public Image getImageCache() {
Image cache = cache_;
final Dimension size = getSize();
if (cache == null) {
cache = createImage(size.width, size.height);
if (cache != null) {
final Graphics cg = cache.getGraphics();
graphe_.dessine(cg, 0, 0, size.width, size.height, 0, this);
}
}
return cache;
}
// Component events
/**
* ....
*
* @param _evt
*/
@Override
public void componentHidden(final ComponentEvent _evt) {
// clipPrec_ = new Rectangle();
repaint();
}
@Override
public void componentMoved(final ComponentEvent _evt) {
// clipPrec_ = new Rectangle();
repaint();
}
@Override
public void componentResized(final ComponentEvent _evt) {
fullRepaint();
}
@Override
public void componentShown(final ComponentEvent _evt) {
// clipPrec_ = new Rectangle();
repaint();
}
public void fullRepaint() {
if (DEBUG) {
FuLog.error("BGraphe : fullRepaint");
}
cache_ = null;
if (isVisible()) {
repaint();
}
}
public void restaurer() {
if (graphe_.restaurer()) {
fullRepaint();
}
}
@Override
public boolean imageUpdate(final Image _img, final int _infoflags, final int _x, final int _y, final int _width,
final int _height) {
if (Fu.DEBUG && FuLog.isDebug()) {
FuLog.debug("EGR: imageUpdate : " + _infoflags + CtuluLibString.ESPACE + _x + CtuluLibString.VIR + _y
+ CtuluLibString.ESPACE + _width + "x" + _height + CtuluLibString.ESPACE + _img);
}
return super.imageUpdate(_img, _infoflags, _x, _y, _width, _height);
}
/**
* Utiliser EbliPrinter.
*
* @param _job
* @param _g
* @deprecated
*/
public void print(final PrintJob _job, final Graphics _g) {
BuPrinter.INFO_DOC = new BuInformationsDocument();
BuPrinter.INFO_DOC.name = getName();
BuPrinter.INFO_DOC.logo = BuResource.BU.getIcon("graphe");
if (cache_ == null) {
FuLog.error("BGraphe: printing component");
BuPrinter.printComponent(_job, _g, this);
} else {
FuLog.error("BGraphe: printing cache image");
BuPrinter.printImage(_job, _g, cache_);
}
}
/**
* @return l'image produite dans la taille courante du composant.
*/
@Override
public BufferedImage produceImage(Map _params) {
return CtuluLibImage.produceImageForComponent(this, _params);
}
@Override
public BufferedImage produceImage(int _w, int _h, Map _params) {
return CtuluLibImage.produceImageForComponent(this, _w, _h, _params);
}
@Override
public Dimension getDefaultImageDimension() {
return getSize();
}
protected String[] formatData(final double[][] _axes, final double[] _xData, final double[][] _yData,
final boolean _optimize, final NumberFormat _nf) {
FuLog.error("formatData");
final Dimension scSize = Toolkit.getDefaultToolkit().getScreenSize();
final double x1Pixel = Math.abs((_axes[0][1] - _axes[0][0]) / scSize.width);
final double y1Pixel = Math.abs((_axes[1][1] - _axes[1][0]) / scSize.height);
FuLog.error(" x1pix=" + x1Pixel + ", y1pix=" + y1Pixel);
final double maxAbsVal = _axes[0][1];
final double minAbsVal = _axes[0][0];
final double maxCotVal = _axes[1][1];
final double minCotVal = _axes[1][0];
final String[] crb = new String[_yData.length];
for (int n = 0; n < _yData.length; n++) {
crb[n] = "";
}
if (_xData.length > 1) {
double xPrec = 0.;
double x = 0.;
double xSuiv = 0.;
double dx = 0.;
final double[] yPrec = new double[_yData.length];
final double[] y = new double[_yData.length];
final double[] ySuiv = new double[_yData.length];
final double[] dy = new double[_yData.length];
xPrec = Double.POSITIVE_INFINITY;
for (int n = 0; n < _yData.length; n++) {
yPrec[n] = Double.POSITIVE_INFINITY;
}
final int[] ny = new int[_yData.length];
boolean xPrecDedans = true;
boolean xDedans = true;
boolean xSuivDedans = true;
final boolean[] yPrecDedans = new boolean[_yData.length];
final boolean[] yDedans = new boolean[_yData.length];
final boolean[] ySuivDedans = new boolean[_yData.length];
final boolean[] yEchOk = new boolean[_yData.length];
Arrays.fill(yPrecDedans, true);
Arrays.fill(yDedans, true);
Arrays.fill(ySuivDedans, true);
Arrays.fill(yEchOk, true);
// Premier point
x = _xData[0];
xSuiv = _xData[1];
for (int n = 0; n < _yData.length; n++) {
y[n] = _yData[n][0];
ySuiv[n] = _yData[n][1];
}
xDedans = (x >= minAbsVal) && (x <= maxAbsVal);
xSuivDedans = (xSuiv >= minAbsVal) && (xSuiv <= maxAbsVal);
if (xDedans || xSuivDedans) {
for (int n = 0; n < _yData.length; n++) {
yDedans[n] = (y[n] >= minCotVal) && (y[n] <= maxCotVal);
ySuivDedans[n] = (ySuiv[n] >= minCotVal) && (ySuiv[n] <= maxCotVal);
if (yDedans[n] || ySuivDedans[n]) {
crb[n] += _nf.format(x) + CtuluLibString.ESPACE + _nf.format(y[n]) + CtuluLibString.LINE_SEP;
ny[n]++;
}
}
}
// Points 1 -> N-1
for (int i = 1; i < _xData.length - 1; i++) {
xPrec = x;
x = xSuiv;
xSuiv = _xData[i + 1];
xPrecDedans = xDedans;
xDedans = xSuivDedans;
xSuivDedans = (xSuiv >= minAbsVal) && (xSuiv <= maxAbsVal);
if (xPrecDedans || xDedans || xSuivDedans) {
for (int n = 0; n < _yData.length; n++) {
yPrec[n] = y[n];
y[n] = ySuiv[n];
ySuiv[n] = _yData[n][i + 1];
yPrecDedans[n] = yDedans[n];
yDedans[n] = ySuivDedans[n];
ySuivDedans[n] = (ySuiv[n] >= minCotVal) && (ySuiv[n] <= maxCotVal);
if (_optimize) {
dx = x - xPrec;
dy[n] = y[n] - yPrec[n];
dx = dx < 0 ? -dx : dx;
dy[n] = dy[n] < 0 ? -dy[n] : dy[n];
yEchOk[n] = ((dx > x1Pixel) || (dy[n] > y1Pixel));
}
if ((yPrecDedans[n] || yDedans[n] || ySuivDedans[n]) && yEchOk[n]) {
crb[n] += _nf.format(x) + CtuluLibString.ESPACE + _nf.format(y[n]) + CtuluLibString.LINE_SEP;
ny[n]++;
}
}
}
}
// Dernier point
xPrec = x;
x = xSuiv;
xPrecDedans = xDedans;
xDedans = xSuivDedans;
if (xPrecDedans || xDedans) {
for (int n = 0; n < _yData.length; n++) {
yPrec[n] = y[n];
y[n] = ySuiv[n];
yPrecDedans[n] = yDedans[n];
yDedans[n] = ySuivDedans[n];
if (yPrecDedans[n] || yDedans[n]) {
crb[n] += _nf.format(x) + CtuluLibString.ESPACE + _nf.format(y[n]) + CtuluLibString.LINE_SEP;
ny[n]++;
}
}
}
for (int n = 0; n < _yData.length; n++) {
FuLog.error("EGR: y[" + n + "]: " + ny[n] + " points");
}
}
return crb;
}
protected List[] formatBinData(final double[][] _axes, final double[] _xData, final double[][] _yData,
final boolean _optimize) {
FuLog.error("formatBinData");
Valeur v = null;
final Dimension scSize = Toolkit.getDefaultToolkit().getScreenSize();
final double x1Pixel = Math.abs((_axes[0][1] - _axes[0][0]) / scSize.width);
final double y1Pixel = Math.abs((_axes[1][1] - _axes[1][0]) / scSize.height);
FuLog.error(" x1pix=" + x1Pixel + ", y1pix=" + y1Pixel);
final double maxAbsVal = _axes[0][1];
final double minAbsVal = _axes[0][0];
final double maxCotVal = _axes[1][1];
final double minCotVal = _axes[1][0];
final List[] crb = new List[_yData.length];
for (int n = 0; n < _yData.length; n++) {
crb[n] = new ArrayList();
}
if (_xData.length > 1) {
double xPrec = 0.;
double x = 0.;
double xSuiv = 0.;
double dx = 0.;
final double[] yPrec = new double[_yData.length];
final double[] y = new double[_yData.length];
final double[] ySuiv = new double[_yData.length];
final double[] dy = new double[_yData.length];
xPrec = Double.POSITIVE_INFINITY;
for (int n = 0; n < _yData.length; n++) {
yPrec[n] = Double.POSITIVE_INFINITY;
}
final int[] ny = new int[_yData.length];
boolean xPrecDedans = true;
boolean xDedans = true;
boolean xSuivDedans = true;
final boolean[] yPrecDedans = new boolean[_yData.length];
final boolean[] yDedans = new boolean[_yData.length];
final boolean[] ySuivDedans = new boolean[_yData.length];
final boolean[] yEchOk = new boolean[_yData.length];
for (int n = 0; n < _yData.length; n++) {
yPrecDedans[n] = true;
yDedans[n] = true;
ySuivDedans[n] = true;
yEchOk[n] = true;
}
// Premier point
x = _xData[0];
xSuiv = _xData[1];
for (int n = 0; n < _yData.length; n++) {
y[n] = _yData[n][0];
ySuiv[n] = _yData[n][1];
}
xDedans = (x >= minAbsVal) && (x <= maxAbsVal);
xSuivDedans = (xSuiv >= minAbsVal) && (xSuiv <= maxAbsVal);
if (xDedans || xSuivDedans) {
for (int n = 0; n < _yData.length; n++) {
yDedans[n] = (y[n] >= minCotVal) && (y[n] <= maxCotVal);
ySuivDedans[n] = (ySuiv[n] >= minCotVal) && (ySuiv[n] <= maxCotVal);
if (yDedans[n] || ySuivDedans[n]) {
v = new Valeur();
v.s_ = x;
v.v_ = y[n];
crb[n].add(v);
ny[n]++;
}
}
}
// Points 1 -> N-1
for (int i = 1; i < _xData.length - 1; i++) {
xPrec = x;
x = xSuiv;
xSuiv = _xData[i + 1];
xPrecDedans = xDedans;
xDedans = xSuivDedans;
xSuivDedans = (xSuiv >= minAbsVal) && (xSuiv <= maxAbsVal);
if (xPrecDedans || xDedans || xSuivDedans) {
for (int n = 0; n < _yData.length; n++) {
yPrec[n] = y[n];
y[n] = ySuiv[n];
ySuiv[n] = _yData[n][i + 1];
yPrecDedans[n] = yDedans[n];
yDedans[n] = ySuivDedans[n];
ySuivDedans[n] = (ySuiv[n] >= minCotVal) && (ySuiv[n] <= maxCotVal);
if (_optimize) {
dx = x - xPrec;
dy[n] = y[n] - yPrec[n];
dx = dx < 0 ? -dx : dx;
dy[n] = dy[n] < 0 ? -dy[n] : dy[n];
yEchOk[n] = ((dx > x1Pixel) || (dy[n] > y1Pixel));
}
if ((yPrecDedans[n] || yDedans[n] || ySuivDedans[n]) && yEchOk[n]) {
v = new Valeur();
v.s_ = x;
v.v_ = y[n];
crb[n].add(v);
ny[n]++;
}
}
}
}
// Dernier point
xPrec = x;
x = xSuiv;
xPrecDedans = xDedans;
xDedans = xSuivDedans;
if (xPrecDedans || xDedans) {
for (int n = 0; n < _yData.length; n++) {
yPrec[n] = y[n];
y[n] = ySuiv[n];
yPrecDedans[n] = yDedans[n];
yDedans[n] = ySuivDedans[n];
if (yPrecDedans[n] || yDedans[n]) {
v = new Valeur();
v.s_ = x;
v.v_ = y[n];
crb[n].add(v);
ny[n]++;
}
}
}
for (int n = 0; n < _yData.length; n++) {
FuLog.error(" y[" + n + "]: " + ny[n] + " points");
}
}
return crb;
}
/**
* ....
*
* @param _g
*/
@Override
protected void paintComponent(final Graphics _g) {
if (graphe_ == null) {
return;
}
// DEBUG: cette ligne faisait s'effacer le composant a chaque repaint!!
// if( g.getClipBounds().equals(clipPrec_) ) return;
// clipPrec_ = g.getClipBounds();
final Dimension size = getSize();
Graphics cg;
if (cache_ == null) {
try {
cache_ = createImage(size.width, size.height);
cg = cache_.getGraphics();
} catch (final NullPointerException e) {
cache_ = null;
cg = _g;
}
if (CtuluLibMessage.DEBUG) {
FuLog.error("BGraphe : FULL");
}
graphe_.dessine(cg, 0, 0, size.width, size.height, 0, this);
if (cg != _g) {
if (DEBUG) {
FuLog.error("BGaphe : drawImage " + cache_.getWidth(this) + "x" + cache_.getHeight(this));
}
if (cache_ != null) {
_g.drawImage(cache_, 0, 0, this);
}
}
} else {
if (DEBUG) {
FuLog.error("BGraphe : CACH");
}
_g.drawImage(cache_, 0, 0, this);
}
}
public int getNumberOfPages() {
return 1;
}
public int print(final Graphics _g, final PageFormat _format, final int _numPage) {
if (_numPage != 0) {
return Printable.NO_SUCH_PAGE;
}
final Graphics2D g2d = (Graphics2D) _g;
final Color initColor = g2d.getColor();
final Font initFont = g2d.getFont();
g2d.setColor(Color.black);
g2d.setFont(EbliPrinter.FONTBASE);
// les dimensions du document
final int h = getHeight();
final int w = getWidth();
// calcule le facteur d'echelle maximal
final double facteur = EbliPrinter.echelle(_format, w, h);
final int wDessin = (int) Math.ceil(w * facteur);
final int hDessin = (int) Math.ceil(h * facteur);
// recuperation du point superieur gauche de la zone imprimable
final int x = (int) Math.round(_format.getImageableX() + (_format.getImageableWidth() - wDessin) / 2);
final int y = (int) Math.round(_format.getImageableY() + (_format.getImageableHeight() - hDessin) / 2);
// sauvegarde pour restauration finale
final Shape s = g2d.getClip();
// dessin...
g2d.clip(new Rectangle.Double(x, y, wDessin, hDessin));
graphe_.dessine(g2d, x, y, wDessin, hDessin, 0, this);
g2d.setClip(s);
g2d.setColor(initColor);
g2d.setFont(initFont);
return Printable.PAGE_EXISTS;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy