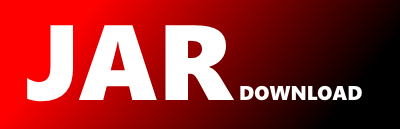
org.fudaa.ebli.graphe.BGrapheController Maven / Gradle / Ivy
The newest version!
/*
* @creation 12 d?c. 2003
* @modification $Date: 2006-09-19 14:55:45 $
* @license GNU General Public License 2
* @copyright (c)1998-2001 CETMEF 2 bd Gambetta F-60231 Compiegne
* @mail [email protected]
*/
package org.fudaa.ebli.graphe;
import java.awt.Color;
import java.awt.Component;
import java.awt.Font;
import java.awt.Graphics;
import java.awt.event.ActionEvent;
import java.awt.print.PageFormat;
import javax.swing.Action;
import javax.swing.DefaultListSelectionModel;
import javax.swing.JColorChooser;
import javax.swing.JComponent;
import javax.swing.JTable;
import javax.swing.ListSelectionModel;
import javax.swing.table.TableCellEditor;
import javax.swing.table.TableCellRenderer;
import org.fudaa.ctulu.gui.CtuluCellBooleanEditor;
import org.fudaa.ctulu.gui.CtuluCellBooleanRenderer;
import org.fudaa.ctulu.gui.CtuluCellButtonEditor;
import org.fudaa.ctulu.gui.CtuluCellTextRenderer;
import org.fudaa.ebli.commun.EbliActionInterface;
import org.fudaa.ebli.commun.EbliActionSimple;
import org.fudaa.ebli.commun.EbliLib;
import org.fudaa.ebli.ressource.EbliResource;
import org.fudaa.ebli.trace.TraceIcon;
/**
* Une classe qui permet de gerer un graphe avec une liste des courbes utilis?es.
*
* @author deniger
* @version $Id: BGrapheController.java,v 1.13 2006-09-19 14:55:45 deniger Exp $
*/
public class BGrapheController {
private BGraphe bgraphe_;
private Graphe graphe_;
private BGrapheComponentTableModel grapheModel_;
private ListSelectionModel selectionModel_;
private EbliActionInterface colorAction_;
/**
*
*/
public BGrapheController() {
this(true, true);
}
public BGrapheController(final boolean _legend, final boolean _copyright) {
bgraphe_= new BGraphe();
graphe_= new Graphe();
graphe_.legende_= _legend;
graphe_.copyright_= _copyright;
bgraphe_.setGraphe(graphe_);
}
public void updateModel(){
if(grapheModel_!=null) {
grapheModel_.fireTableDataChanged();
}
}
public void updateAxes(){
final double[] minMax=graphe_.getMinMaxXY();
double pas=(minMax[1]-minMax[0])/10;
Axe a=graphe_.getHorizontalAxe();
if(a!=null) {
a.pas_=(int)pas;
}
pas=(minMax[3]-minMax[2])/10;
a=graphe_.getFirstVerticalAxe();
if(a!=null) {
a.pas_=(int)pas;
}
}
public void setLegende(final boolean _b) {
graphe_.legende_= _b;
bgraphe_.fullRepaint();
}
public void setInteractif(final boolean _b) {
bgraphe_.setInteractif(_b);
}
public EbliActionInterface createChangeCouleurAction() {
if (colorAction_ == null) {
colorAction_=
new EbliActionSimple(
EbliResource.EBLI.getString("Couleur de trac?"),
EbliResource.EBLI.getIcon("avantplan"),
"FOREGROUND_COLOR") {
@Override
public void actionPerformed(final ActionEvent _e) {
changeAspectCouleur();
}
};
colorAction_.putValue(
Action.SHORT_DESCRIPTION,
EbliResource.EBLI.getString(
"Modifier la couleur de trac? des courbes s?lectionn?es"));
}
return colorAction_;
}
public void restaurer(){
bgraphe_.restaurer();
}
public void changeAspectCouleur() {
if (selectionModel_ == null) {
return;
}
final int min= selectionModel_.getMinSelectionIndex();
if (min >= 0) {
final int max= selectionModel_.getMaxSelectionIndex();
Color init= null;
if (max == min) {
init= graphe_.getGrapheComponent(min).getAspectContour();
}
final Color newColor=
JColorChooser.showDialog(
bgraphe_,
EbliLib.getS("Couleur du trac?"),
init);
if (newColor != null) {
boolean c= false;
for (int i= min; i <= max; i++) {
if (selectionModel_.isSelectedIndex(i)) {
c |= graphe_.getGrapheComponent(i).setAspectContour(newColor);
}
}
if (c) {
bgraphe_.fullRepaint();
}
}
}
}
public void setCopyright(final boolean _b) {
graphe_.copyright_= _b;
bgraphe_.fullRepaint();
}
public JComponent createGrapheComponent() {
return bgraphe_;
}
public JTable createTable() {
final JTable r= new JTable(getGrapheTableModel());
grapheModel_.setRendererAndEditor(r.getColumnModel());
r.setSelectionModel(getTableSelectionModel());
return r;
}
public ListSelectionModel getTableSelectionModel() {
if (selectionModel_ == null) {
selectionModel_= new DefaultListSelectionModel();
}
return selectionModel_;
}
public BGrapheComponentTableModel getGrapheTableModel() {
if (grapheModel_ == null) {
grapheModel_= createGrapheTableModel();
}
return grapheModel_;
}
protected BGrapheComponentTableModel createGrapheTableModel() {
return new BGrapheComponentTableModel(bgraphe_);
}
public void ajoute(final Axe _a) {
graphe_.ajoute(_a);
bgraphe_.repaint();
}
public void ajouteComponent(final GrapheComponent _c) {
graphe_.ajouteComponent(_c);
if (grapheModel_ != null) {
grapheModel_.fireTableDataChanged();
}
}
/**
*
*/
public void setFtLegend(final Font _font) {
graphe_.setFtLegend(_font);
bgraphe_.repaint();
}
public int print(final Graphics _g, final PageFormat _format, final int _numPage) {
return bgraphe_.print(_g, _format, _numPage);
}
/**
*
*/
public void setFtSousTitre(final Font _font) {
graphe_.setFtSousTitre(_font);
bgraphe_.repaint();
}
public static GrapheVisibleCellEditor createVisibleCellEditor() {
return new GrapheVisibleCellEditor();
}
public static TableCellRenderer createVisibleCellRenderer() {
return new GrapheVisibleCellRenderer();
}
public static TableCellEditor createContourColorEditor() {
return new GrapheColorContourCellEditor();
}
public static TableCellRenderer createNameContourRenderer() {
return new GrapheNameContourRenderer();
}
public static TableCellRenderer createContourColorRenderer() {
return new GrapheColorContourCellRenderer();
}
/**
*
*/
public void setFtTitre(final Font _font) {
graphe_.setFtTitre(_font);
bgraphe_.repaint();
}
/**
* @author ??
* @version $Id: BGrapheController.java,v 1.13 2006-09-19 14:55:45 deniger Exp $
*/
public static class GrapheVisibleCellRenderer
extends CtuluCellBooleanRenderer {
@Override
public void setValue(final Object _value) {
setSelected(((GrapheComponent)_value).isVisible());
}
}
/**
* @author ??
* @version $Id: BGrapheController.java,v 1.13 2006-09-19 14:55:45 deniger Exp $
*/
public static class GrapheVisibleCellEditor extends CtuluCellBooleanEditor {
@Override
public boolean getBooleanValue(final Object _o) {
return ((GrapheComponent)_o).isVisible();
}
}
/**
* @author ??
* @version $Id: BGrapheController.java,v 1.13 2006-09-19 14:55:45 deniger Exp $
*/
public static class GrapheNameContourRenderer extends CtuluCellTextRenderer {
public GrapheNameContourRenderer() {
setIcon(new TraceIcon(TraceIcon.CARRE_PLEIN, 6));
}
@Override
protected void setValue(final Object _value) {
final GrapheComponent c= (GrapheComponent)_value;
setText(c.getTitre());
final TraceIcon ic= (TraceIcon)getIcon();
ic.setCouleur(c.getAspectContour());
}
}
/**
* @author ??
* @version $Id: BGrapheController.java,v 1.13 2006-09-19 14:55:45 deniger Exp $
*/
public static class GrapheColorContourCellRenderer
extends CtuluCellTextRenderer {
public GrapheColorContourCellRenderer() {
setOpaque(true);
}
@Override
public Component getTableCellRendererComponent(
final JTable _table,
final Object _value,
final boolean _isSelected,
final boolean _hasFocus,
final int _row,
final int _column) {
setBackground(((GrapheComponent)_value).getAspectContour());
return this;
}
}
public static class GrapheColorContourCellEditor
extends CtuluCellButtonEditor {
public GrapheColorContourCellEditor() {
super(null);
setOpaque(true);
}
@Override
public Component getTableCellEditorComponent(
final JTable _table,
final Object _value,
final boolean _isSelected,
final int _row,
final int _column) {
value_= ((GrapheComponent)_value).getAspectContour();
setBackground((Color)value_);
return this;
}
/**
*
*/
@Override
protected void doAction() {
Color c= (Color)value_;
c=
JColorChooser.showDialog(
this,
EbliResource.EBLI.getString("Couleur du trac?"),
c);
if (c != null) {
value_= c;
setBackground(c);
}
}
}
/**
*
*/
public GrapheInteractionSuiviSouris activeSuiviSouris() {
return bgraphe_.activeSuiviSouris();
}
/**
*
*/
public void unactiveSuiviSouris() {
bgraphe_.unactiveSuiviSouris();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy