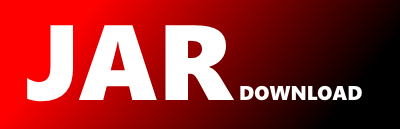
org.fudaa.ebli.graphe.BGrapheEditeurAxes Maven / Gradle / Ivy
The newest version!
/*
* @creation 1999-12-13
* @modification $Date: 2007-04-16 16:35:09 $
* @license GNU General Public License 2
* @copyright (c)1998-2001 CETMEF 2 bd Gambetta F-60231 Compiegne
* @mail [email protected]
*/
package org.fudaa.ebli.graphe;
import java.awt.Color;
import java.awt.Dimension;
import java.awt.Graphics;
import java.awt.Point;
import java.awt.event.*;
import java.beans.PropertyChangeEvent;
import java.beans.PropertyChangeListener;
import java.text.NumberFormat;
import java.util.Locale;
import java.util.Vector;
import javax.swing.JToggleButton;
import javax.swing.event.ChangeEvent;
import javax.swing.event.ChangeListener;
import com.memoire.bu.*;
import org.fudaa.ctulu.CtuluLib;
import org.fudaa.ebli.commun.EbliLib;
import org.fudaa.ebli.controle.BMolette;
import org.fudaa.ebli.graphe.Axe;
import org.fudaa.ebli.graphe.BGraphe;
import org.fudaa.ebli.graphe.Graphe;
import org.fudaa.ebli.graphe.Valeur;
/**
* @version $Revision: 1.11 $ $Date: 2007-04-16 16:35:09 $ by $Author: deniger $
* @author Axel von Arnim
*/
public class BGrapheEditeurAxes extends BuPanel implements ActionListener, KeyListener, ChangeListener, MouseListener,
MouseMotionListener, PropertyChangeListener {
private static final Color MOD_COLOR = Color.green.darker();
BuTextField tfEchX1_, tfEchX2_, tfEchZ1_, tfEchZ2_;
BMolette mlTrX_, mlTrZ_;
BuButton btReset_;
JToggleButton btZoom_;
Vector listeners_;
int sensibilite_;
BGraphe bgraphe_;
boolean gInteractif_;
ContexteCoordonnees coordContext_, coordContextP_;
final BuLabel lbOrd;
final BuLabel lbAbs;
public BGrapheEditeurAxes(final BGraphe _b) {
super();
bgraphe_ = _b;
coordContext_ = null;
coordContextP_ = null;
listeners_ = new Vector();
sensibilite_ = 100;
final NumberFormat nf2 = NumberFormat.getInstance(Locale.US);
nf2.setMaximumFractionDigits(3);
nf2.setGroupingUsed(false);
this.setLayout(new BuGridLayout(2, 5, 5, true, true));
final BuPanel pnCentr = new BuPanel();
pnCentr.setLayout(new BuVerticalLayout(5, true, false));
int m = 0;
final BuPanel pnEchX = new BuPanel();
pnEchX.setLayout(new BuHorizontalLayout(5, false, false));
lbAbs = new BuLabel(EbliLib.getS("Abscisse"));
pnEchX.add(lbAbs, m++);
tfEchX1_ = BuTextField.createDoubleField();
// tfEchX1_.setDisplayFormat(nf2);
tfEchX1_.addActionListener(this);
tfEchX1_.addKeyListener(this);
tfEchX1_.setColumns(5);
pnEchX.add(tfEchX1_, m++);
tfEchX2_ = BuTextField.createDoubleField();
// tfEchX2_.setDisplayFormat(nf2);
tfEchX2_.addActionListener(this);
tfEchX2_.addKeyListener(this);
tfEchX2_.setColumns(5);
pnEchX.add(tfEchX2_, m++);
m = 0;
final BuPanel pnEchZ = new BuPanel();
pnEchZ.setLayout(new BuHorizontalLayout(5, false, false));
lbOrd = new BuLabel(EbliLib.getS("Ordonn?e"));
lbOrd.setPreferredSize(lbAbs.getPreferredSize());
pnEchZ.add(lbOrd, m++);
tfEchZ1_ = BuTextField.createDoubleField();
// tfEchZ1_.setDisplayFormat(nf2);
tfEchZ1_.addActionListener(this);
tfEchZ1_.addKeyListener(this);
tfEchZ1_.setColumns(5);
pnEchZ.add(tfEchZ1_, m++);
tfEchZ2_ = BuTextField.createDoubleField();
// tfEchZ2_.setDisplayFormat(nf2);
tfEchZ2_.addActionListener(this);
tfEchZ2_.addKeyListener(this);
tfEchZ2_.setColumns(5);
pnEchZ.add(tfEchZ2_, m++);
final BuPanel pnBoutons = new BuPanel();
pnBoutons.setLayout(new BuHorizontalLayout(0, true, false));
btZoom_ = new JToggleButton(EbliLib.getS("Zoom"));
btZoom_.addActionListener(this);
btReset_ = new BuButton(EbliLib.getS("Restaurer"));
btReset_.addActionListener(this);
pnBoutons.add(btZoom_, 0);
pnBoutons.add(btReset_, 1);
mlTrX_ = new BMolette();
mlTrX_.setOrientation(BMolette.HORIZONTAL);
mlTrX_.addChangeListener(this);
pnCentr.add(pnEchX, 0);
pnCentr.add(pnEchZ, 1);
pnCentr.add(pnBoutons, 2);
pnCentr.doLayout();
mlTrZ_ = new BMolette();
mlTrZ_.setMinimumSize(new Dimension(0, 0));
final Dimension d = mlTrZ_.getPreferredSize();
d.height = pnCentr.getPreferredSize().height;
mlTrZ_.setPreferredSize(d);
mlTrZ_.setOrientation(BMolette.VERTICAL);
mlTrZ_.addChangeListener(this);
this.add(pnCentr, 0);
this.add(mlTrZ_, 1);
this.add(mlTrX_, 2);
}
public Double[][] getAxes() {
final Double[][] axes = new Double[2][2];
axes[0][0] = (Double) tfEchX1_.getValue();
axes[0][1] = (Double) tfEchX2_.getValue();
axes[1][0] = (Double) tfEchZ1_.getValue();
axes[1][1] = (Double) tfEchZ2_.getValue();
return axes;
}
public void setAxes(final Double[][] _a) {
if (_a[0][0] != null) {
tfEchX1_.setValue(_a[0][0]);
}
if (_a[0][1] != null) {
tfEchX2_.setValue(_a[0][1]);
}
if (_a[1][0] != null) {
tfEchZ1_.setValue(_a[1][0]);
}
if (_a[1][1] != null) {
tfEchZ2_.setValue(_a[1][1]);
}
}
@Override
public void keyPressed(final KeyEvent _evt) {}
@Override
public void keyReleased(final KeyEvent _evt) {}
@Override
public void keyTyped(final KeyEvent _evt) {
final Object src = _evt.getSource();
if (src == tfEchX1_) {
tfEchX1_.setForeground(MOD_COLOR);
tfEchX1_.repaint();
} else if (src == tfEchX2_) {
tfEchX2_.setForeground(MOD_COLOR);
tfEchX2_.repaint();
} else if (src == tfEchZ1_) {
tfEchZ1_.setForeground(MOD_COLOR);
tfEchZ1_.repaint();
} else if (src == tfEchZ2_) {
tfEchZ2_.setForeground(MOD_COLOR);
tfEchZ2_.repaint();
}
}
public int getSensibilite() {
return sensibilite_;
}
public void setSensibilite(final int _sensibilite) {
if (sensibilite_ != _sensibilite) {
final int vp = sensibilite_;
sensibilite_ = _sensibilite;
firePropertyChange("sensibilite", vp, sensibilite_);
}
}
@Override
public void stateChanged(final ChangeEvent _evt) {
final BMolette src = (BMolette) _evt.getSource();
int dir = 1;
if (src.getDirection() == BMolette.MOINS) {
dir = -1;
}
final Double[][] axes = getAxes();
if (src == mlTrX_) {
if ((axes[0][0] == null) || (axes[0][1] == null)) {
return;
}
final double x1 = axes[0][0].doubleValue();
final double x2 = axes[0][1].doubleValue();
final double dx = (x2 - x1) / sensibilite_;
axes[0][0] = new Double(x1 + dir * dx);
axes[0][1] = new Double(x2 + dir * dx);
}
if (src == mlTrZ_) {
if ((axes[1][0] == null) || (axes[1][1] == null)) {
return;
}
final double z1 = axes[1][0].doubleValue();
final double z2 = axes[1][1].doubleValue();
final double dz = (z2 - z1) / sensibilite_;
axes[1][0] = new Double(z1 + dir * dz);
axes[1][1] = new Double(z2 + dir * dz);
}
setAxes(axes);
if (!src.getValueIsAdjusting()) {
fireActionPerformed(new ActionEvent(this, ActionEvent.ACTION_PERFORMED, getName()));
}
}
@Override
public void propertyChange(final PropertyChangeEvent _evt) {
if (_evt.getPropertyName().equals("AXEX")) {
final Axe axeX = (Axe) _evt.getNewValue();
tfEchX1_.setValue(new Double(axeX.minimum_));
tfEchX2_.setValue(new Double(axeX.maximum_));
} else if (_evt.getPropertyName().equals("AXEY")) {
final Axe axeY = (Axe) _evt.getNewValue();
tfEchZ1_.setValue(new Double(axeY.minimum_));
tfEchZ2_.setValue(new Double(axeY.maximum_));
}
}
@Override
public void actionPerformed(final ActionEvent _evt) {
final Object src = _evt.getSource();
if (src == btZoom_) {
if (btZoom_.isSelected()) {
gInteractif_ = bgraphe_.isInteractif();
if (gInteractif_) {
bgraphe_.setInteractif(false);
}
bgraphe_.addMouseListener(this);
bgraphe_.addMouseMotionListener(this);
} else {
bgraphe_.removeMouseListener(this);
bgraphe_.removeMouseMotionListener(this);
if (gInteractif_) {
bgraphe_.setInteractif(true);
}
}
return;
}
if (((src == tfEchX1_) || (src == tfEchX2_) || (src == tfEchZ1_) || (src == tfEchZ2_)) && (!verifieBornes())) {
return;
}
Color fg = javax.swing.UIManager.getColor("textfield.foreground");
if (fg == null) {
fg = Color.black;
}
tfEchX1_.setForeground(fg);
tfEchX1_.repaint();
tfEchX2_.setForeground(fg);
tfEchX2_.repaint();
tfEchZ1_.setForeground(fg);
tfEchZ1_.repaint();
tfEchZ2_.setForeground(fg);
tfEchZ2_.repaint();
if (src == btReset_) {
tfEchX1_.setValue(null);
tfEchX2_.setValue(null);
tfEchZ1_.setValue(null);
tfEchZ2_.setValue(null);
}
fireActionPerformed(new ActionEvent(this, _evt.getID(), getName(), _evt.getModifiers()));
}
public void addActionListener(final ActionListener _l) {
if (!listeners_.contains(_l)) {
listeners_.add(_l);
}
}
public void removeActionListener(final ActionListener _l) {
if (listeners_.contains(_l)) {
listeners_.remove(_l);
}
}
private void fireActionPerformed(final ActionEvent _evt) {
for (int i = 0; i < listeners_.size(); i++) {
((ActionListener) listeners_.get(i)).actionPerformed(_evt);
}
}
@Override
public void mousePressed(final MouseEvent _evt) {
if (coordContext_ == null) {
coordContext_ = new ContexteCoordonnees();
coordContextP_ = new ContexteCoordonnees();
final Graphe g = bgraphe_.getGraphe();
coordContext_.axes_ = g.getElementsParType(new Axe().getClass());
/*
* coordContextP_.g_ = g; coordContext_.g_ = g;
*/
coordContextP_.x_ = g.marges_.gauche_;
coordContext_.x_ = g.marges_.gauche_;
coordContextP_.y_ = g.marges_.haut_;
coordContext_.y_ = g.marges_.haut_;
coordContextP_.w_ = bgraphe_.getWidth() - g.marges_.gauche_ - g.marges_.droite_;
coordContext_.w_ = coordContextP_.w_;
coordContextP_.h_ = bgraphe_.getHeight() - g.marges_.haut_ - g.marges_.bas_;
coordContext_.h_ = coordContextP_.h_;
// recherche des axes
for (int ia = 0; ia < coordContext_.axes_.length; ia++) {
final Axe a = (Axe) coordContext_.axes_[ia];
if (a.vertical_) {
coordContextP_.ay_ = a;
coordContext_.ay_ = a;
} else {
coordContextP_.ax_ = a;
coordContext_.ax_ = a;
}
}
}
coordContext_.pointDep_ = new Point(_evt.getX(), _evt.getY());
coordContextP_.pointFin_ = coordContext_.pointDep_;
}
@Override
public void mouseEntered(final MouseEvent _evt) {}
@Override
public void mouseExited(final MouseEvent _evt) {}
@Override
public void mouseClicked(final MouseEvent _evt) {}
@Override
public void mouseReleased(final MouseEvent _evt) {
if (coordContextP_.pointFin_ == coordContext_.pointDep_) {
coordContextP_ = null;
coordContext_ = null;
return;
}
final Graphics gr = bgraphe_.getGraphics();
gr.setXORMode(bgraphe_.getBackground());
gr.setColor(Color.black);
final int xi = coordContext_.pointDep_.x;
final int yi = coordContext_.pointDep_.y;
final int xfp = coordContextP_.pointFin_.x;
final int yfp = coordContextP_.pointFin_.y;
gr.drawRect(xi, yi, xfp - xi, yfp - yi);
coordContext_.pointFin_ = new Point(_evt.getX(), _evt.getY());
final Valeur vDep = versReel(coordContext_.pointDep_, coordContext_);
final Valeur vFin = versReel(coordContext_.pointFin_, coordContext_);
tfEchX1_.setValue(CtuluLib.getDouble(vDep.s_));
tfEchX2_.setValue(CtuluLib.getDouble(vFin.s_));
tfEchZ1_.setValue(CtuluLib.getDouble(vFin.v_));
tfEchZ2_.setValue(CtuluLib.getDouble(vDep.v_));
coordContextP_ = null;
coordContext_ = null;
fireActionPerformed(new ActionEvent(this, ActionEvent.ACTION_PERFORMED, getName()));
}
@Override
public void mouseDragged(final MouseEvent _evt) {
final Graphics gr = bgraphe_.getGraphics();
gr.setXORMode(bgraphe_.getBackground());
gr.setColor(Color.black);
final int xi = coordContext_.pointDep_.x;
final int yi = coordContext_.pointDep_.y;
final int xfp = coordContextP_.pointFin_.x;
final int yfp = coordContextP_.pointFin_.y;
final int xf = _evt.getX();
final int yf = _evt.getY();
final Point p = new Point(xf, yf);
if ((p.x < coordContext_.pointDep_.x) || (p.y < coordContext_.pointDep_.y)) {
return;
}
coordContextP_.pointFin_ = p;
gr.drawRect(xi, yi, xfp - xi, yfp - yi);
gr.drawRect(xi, yi, xf - xi, yf - yi);
}
@Override
public void mouseMoved(final MouseEvent _e) {}
private Valeur versReel(final Point _p, final ContexteCoordonnees _cx) {
final double yr = _cx.ay_.minimum_ + (_cx.y_ + _cx.h_ - _p.y) * (_cx.ay_.maximum_ - _cx.ay_.minimum_) / (_cx.h_);
final double xr = _cx.ax_.minimum_ + (_p.x - _cx.x_) * (_cx.ax_.maximum_ - _cx.ax_.minimum_) / (_cx.w_);
final Valeur res = new Valeur();
res.s_ = xr;
res.v_ = yr;
return res;
}
private boolean verifieBornes() {
final Double[][] axes = getAxes();
if ((axes[0][0] != null) && (axes[0][1] != null) && (axes[0][0].equals(axes[0][1]))) {
return false;
}
if ((axes[1][0] != null) && (axes[1][1] != null) && (axes[1][0].equals(axes[1][1]))) {
return false;
}
return true;
}
static class ContexteCoordonnees {
// Graphe g_;
Object[] axes_;
int x_;
int y_;
int w_;
int h_;
Axe ax_;
Axe ay_;
Point pointDep_;
Point pointFin_;
}
public BuButton getBtReset_() {
return btReset_;
}
public void setBtReset_(BuButton btReset_) {
this.btReset_ = btReset_;
}
public JToggleButton getBtZoom_() {
return btZoom_;
}
public void setBtZoom_(JToggleButton btZoom_) {
this.btZoom_ = btZoom_;
}
public BuLabel getLbOrd() {
return lbOrd;
}
public BuLabel getLbAbs() {
return lbAbs;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy