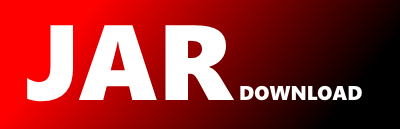
org.fudaa.ebli.graphe.BGraphePersonnaliseur Maven / Gradle / Ivy
The newest version!
/*
* @creation 2000-06-19
* @modification $Date: 2006-09-19 14:55:45 $
* @license GNU General Public License 2
* @copyright (c)1998-2001 CETMEF 2 bd Gambetta F-60231 Compiegne
* @mail [email protected]
*/
package org.fudaa.ebli.graphe;
import java.awt.Color;
import java.awt.Dimension;
import java.awt.FlowLayout;
import java.awt.Image;
import java.awt.MediaTracker;
import java.awt.event.ActionEvent;
import java.awt.event.ActionListener;
import java.beans.PropertyChangeEvent;
import java.beans.PropertyChangeListener;
import java.io.File;
import java.util.Vector;
import javax.swing.*;
import javax.swing.border.TitledBorder;
import javax.swing.event.ListSelectionEvent;
import javax.swing.event.ListSelectionListener;
import com.memoire.bu.BuColorIcon;
import com.memoire.bu.BuGridLayout;
import com.memoire.bu.BuLabel;
import com.memoire.bu.BuPanel;
import com.memoire.bu.BuTextField;
/**
* Un composant pour personnaliser les graphes.
*
* @version $Revision: 1.12 $ $Date: 2006-09-19 14:55:45 $ by $Author: deniger $
* @author Axel von Arnim
*/
public class BGraphePersonnaliseur
extends JTabbedPane
implements ActionListener, ListSelectionListener, PropertyChangeListener {
public static final int OK= 1;
public final static int CANCEL= 2;
Graphe graphe_;
int response_;
Vector listeners_;
BuTextField tfGrapheTitre_;
BuTextField tfGrapheSousTitre_;
JCheckBox cbGrapheLegende_;
BuTextField tfGrapheMargeD_,
tfGrapheMargeG_,
tfGrapheMargeB_,
tfGrapheMargeH_;
JButton btGrapheFond_;
Object[] axes_;
JList lsAxes_;
BuTextField tfAxeTitre_;
JCheckBox cbAxeVisible_;
JCheckBox cbAxeGraduations_;
JCheckBox cbAxeGrille_;
BuTextField tfAxePas_;
BuTextField tfAxeEcart_;
JButton btAxeCouleur_;
Object[] courbes_;
JList lsCourbes_;
BuTextField tfCourbeTitre_;
JCheckBox cbCourbeVisible_;
JCheckBox cbCourbeMarqueurs_;
JButton btCourbeCouleur_;
public BGraphePersonnaliseur(final Graphe _p) {
graphe_= _p;
axes_= graphe_.getElementsParType(new Axe().getClass());
courbes_= graphe_.getElementsParType(new CourbeDefault().getClass());
listeners_= new Vector();
int nGraphe= 0;
final BuPanel pnGrapheEd= new BuPanel();
BuGridLayout lo= new BuGridLayout(2, 5, 5, false, true);
lo.setCfilled(false);
lo.setXAlign(0);
pnGrapheEd.setLayout(lo);
pnGrapheEd.setBorder(new TitledBorder("Graphe"));
createGrapheTitre();
pnGrapheEd.add(new BuLabel("Titre "), nGraphe++);
pnGrapheEd.add(tfGrapheTitre_, nGraphe++);
createSousTitre();
pnGrapheEd.add(new BuLabel("Sous-titre "), nGraphe++);
pnGrapheEd.add(tfGrapheSousTitre_, nGraphe++);
createCbLegende();
pnGrapheEd.add(new BuLabel("L?gende "), nGraphe++);
pnGrapheEd.add(cbGrapheLegende_, nGraphe++);
createBtChanger();
pnGrapheEd.add(new BuLabel("Fond "), nGraphe++);
pnGrapheEd.add(btGrapheFond_, nGraphe++);
final BuPanel pnMargesGD= new BuPanel();
pnMargesGD.setLayout(new FlowLayout(FlowLayout.LEFT));
createMargeDr();
final BuLabel lbMargeD= new BuLabel("droite:");
pnMargesGD.add(lbMargeD);
pnMargesGD.add(tfGrapheMargeD_);
createMargeG();
final BuLabel lbMargeG= new BuLabel("gauche:");
pnMargesGD.add(lbMargeG);
pnMargesGD.add(tfGrapheMargeG_);
pnGrapheEd.add(new BuLabel("Marges "), nGraphe++);
pnGrapheEd.add(pnMargesGD, nGraphe++);
final BuPanel pnMargesHB= new BuPanel();
pnMargesHB.setLayout(new FlowLayout(FlowLayout.LEFT));
createMargeH();
final BuLabel lbMargeH= new BuLabel("haut:");
lbMargeH.setPreferredSize(lbMargeD.getPreferredSize());
pnMargesHB.add(lbMargeH);
pnMargesHB.add(tfGrapheMargeH_);
createMargeB();
final BuLabel lbMargeB= new BuLabel("bas:");
lbMargeB.setPreferredSize(lbMargeG.getPreferredSize());
pnMargesHB.add(lbMargeB);
pnMargesHB.add(tfGrapheMargeB_);
pnGrapheEd.add(new BuLabel(" "), nGraphe++);
pnGrapheEd.add(pnMargesHB, nGraphe++);
final String[] itAxes = createAxes();
final JScrollPane spAxes = createAxeList(itAxes);
spAxes.setPreferredSize(new Dimension(100, 200));
int nAxe= 0;
final BuPanel pnAxeEd= new BuPanel();
lo= new BuGridLayout(2, 5, 5, false, true);
lo.setCfilled(false);
lo.setXAlign(0);
pnAxeEd.setLayout(lo);
createAxeTitre();
pnAxeEd.add(new BuLabel("Titre "), nAxe++);
pnAxeEd.add(tfAxeTitre_, nAxe++);
createAxeVisible();
pnAxeEd.add(new BuLabel("Visible "), nAxe++);
pnAxeEd.add(cbAxeVisible_, nAxe++);
cbAxeGraduations_= new JCheckBox();
cbAxeGraduations_.setActionCommand("AXEGRADUATIONS");
if (axes_.length > 0) {
cbAxeGraduations_.setSelected(((Axe)axes_[0]).graduations_);
}
cbAxeGraduations_.addActionListener(this);
pnAxeEd.add(new BuLabel("Graduations "), nAxe++);
pnAxeEd.add(cbAxeGraduations_, nAxe++);
createAxeGrille();
pnAxeEd.add(new BuLabel("Grille "), nAxe++);
pnAxeEd.add(cbAxeGrille_, nAxe++);
createAxePas();
pnAxeEd.add(new BuLabel("Pas "), nAxe++);
pnAxeEd.add(tfAxePas_, nAxe++);
createAxeEcart();
pnAxeEd.add(new BuLabel("Ecart "), nAxe++);
pnAxeEd.add(tfAxeEcart_, nAxe++);
final Color back=pnAxeEd.getBackground();
createAxeCouleur(back);
pnAxeEd.add(new BuLabel("Couleur "), nAxe++);
pnAxeEd.add(btAxeCouleur_, nAxe++);
final BuPanel pnAxe= new BuPanel();
pnAxe.setLayout(new FlowLayout(FlowLayout.LEFT));
pnAxe.setBorder(new TitledBorder("Axes"));
pnAxe.add(spAxes);
pnAxe.add(pnAxeEd);
final String[] itCourbes = createCourbes();
final JScrollPane spCourbes = createListCourbes(itCourbes);
int nCourbe= 0;
final BuPanel pnCourbeEd= new BuPanel();
lo= new BuGridLayout(2, 5, 5, false, true);
lo.setCfilled(false);
lo.setXAlign(0);
pnCourbeEd.setLayout(lo);
createCourbeTitre();
pnCourbeEd.add(new BuLabel("Titre "), nCourbe++);
pnCourbeEd.add(tfCourbeTitre_, nCourbe++);
createCourbeVisible();
pnCourbeEd.add(new BuLabel("Visible "), nCourbe++);
pnCourbeEd.add(cbCourbeVisible_, nCourbe++);
createCourbeMarqueurs();
pnCourbeEd.add(new BuLabel("Marqueurs "), nCourbe++);
pnCourbeEd.add(cbCourbeMarqueurs_, nCourbe++);
createCourbeCouleur(pnCourbeEd);
pnCourbeEd.add(new BuLabel("Couleur "), nCourbe++);
pnCourbeEd.add(btCourbeCouleur_, nCourbe++);
final BuPanel pnCourbe= new BuPanel();
pnCourbe.setLayout(new FlowLayout(FlowLayout.LEFT));
pnCourbe.setBorder(new TitledBorder("Courbes"));
pnCourbe.add(spCourbes);
pnCourbe.add(pnCourbeEd);
addTab("Graphe", pnGrapheEd);
addTab("Axes", pnAxe);
addTab("Courbes", pnCourbe);
doLayout();
}
private void createCourbeCouleur(final BuPanel _pnCourbeEd) {
Icon icon;
btCourbeCouleur_= new JButton();
icon= new BuColorIcon(_pnCourbeEd.getBackground());
btCourbeCouleur_.setIcon(icon);
if (courbes_.length > 0) {
btCourbeCouleur_.setIcon(
new BuColorIcon(((CourbeDefault)courbes_[0]).aspect_.contour_));
}
btCourbeCouleur_.setActionCommand("COURBECOULEUR");
btCourbeCouleur_.addActionListener(this);
}
private void createCourbeMarqueurs() {
cbCourbeMarqueurs_= new JCheckBox();
cbCourbeMarqueurs_.setActionCommand("COURBEMARQUEURS");
if (courbes_.length > 0) {
cbCourbeMarqueurs_.setSelected(((CourbeDefault)courbes_[0]).marqueurs_);
}
cbCourbeMarqueurs_.addActionListener(this);
}
private void createCourbeVisible() {
cbCourbeVisible_= new JCheckBox();
cbCourbeVisible_.setActionCommand("COURBEVISIBLE");
if (courbes_.length > 0) {
cbCourbeVisible_.setSelected(((CourbeDefault)courbes_[0]).visible_);
}
cbCourbeVisible_.addActionListener(this);
}
private void createCourbeTitre() {
tfCourbeTitre_= new BuTextField();
tfCourbeTitre_.setColumns(8);
if (courbes_.length > 0) {
tfCourbeTitre_.setText(((CourbeDefault)courbes_[0]).titre_);
}
tfCourbeTitre_.setActionCommand("COURBETITRE");
tfCourbeTitre_.addActionListener(this);
}
private JScrollPane createListCourbes(final String[] _itCourbes) {
lsCourbes_= new JList(_itCourbes);
lsCourbes_.setSelectedIndex(0);
lsCourbes_.addListSelectionListener(this);
final JScrollPane spCourbes= new JScrollPane(lsCourbes_);
spCourbes.setPreferredSize(new Dimension(100, 200));
return spCourbes;
}
private String[] createCourbes() {
final String[] itCourbes= new String[courbes_.length];
for (int i= 0; i < courbes_.length; i++) {
itCourbes[i]= ((CourbeDefault)courbes_[i]).titre_;
if ((itCourbes[i] == null) || (itCourbes[i].trim().equals(""))) {
itCourbes[i]= "courbe " + (i + 1);
}
}
return itCourbes;
}
private void createAxeCouleur(final Color _back) {
btAxeCouleur_= new JButton();
final Icon icon= new BuColorIcon(_back);
btAxeCouleur_.setIcon(icon);
if (axes_.length > 0) {
btAxeCouleur_.setIcon(new BuColorIcon(((Axe)axes_[0]).aspect_.contour_));
}
btAxeCouleur_.setActionCommand("AXECOULEUR");
btAxeCouleur_.addActionListener(this);
}
private void createAxeEcart() {
tfAxeEcart_= BuTextField.createIntegerField();
tfAxeEcart_.setColumns(8);
tfAxeEcart_.setEnabled(false);
if (axes_.length > 0) {
tfAxeEcart_.setValue(new Integer(((Axe)axes_[0]).ecart_));
}
tfAxeEcart_.setActionCommand("AXEECART");
tfAxeEcart_.addActionListener(this);
}
private void createAxePas() {
tfAxePas_= BuTextField.createDoubleField();
tfAxePas_.setColumns(8);
if (axes_.length > 0) {
tfAxePas_.setValue(new Double(((Axe)axes_[0]).pas_));
}
tfAxePas_.setActionCommand("AXEPAS");
tfAxePas_.addActionListener(this);
}
private void createAxeGrille() {
cbAxeGrille_= new JCheckBox();
cbAxeGrille_.setActionCommand("AXEGRILLE");
if (axes_.length > 0) {
cbAxeGrille_.setSelected(((Axe)axes_[0]).grille_);
}
cbAxeGrille_.addActionListener(this);
}
private void createAxeVisible() {
cbAxeVisible_= new JCheckBox();
cbAxeVisible_.setActionCommand("AXEVISIBLE");
if (axes_.length > 0) {
cbAxeVisible_.setSelected(((Axe)axes_[0]).visible_);
}
cbAxeVisible_.addActionListener(this);
}
private void createAxeTitre() {
tfAxeTitre_= new BuTextField();
tfAxeTitre_.setColumns(8);
if (axes_.length > 0) {
tfAxeTitre_.setText(((Axe)axes_[0]).titre_);
}
tfAxeTitre_.setActionCommand("AXETITRE");
tfAxeTitre_.addActionListener(this);
}
private JScrollPane createAxeList(final String[] _itAxes) {
lsAxes_= new JList(_itAxes);
lsAxes_.setSelectedIndex(0);
lsAxes_.addListSelectionListener(this);
final JScrollPane spAxes= new JScrollPane(lsAxes_);
return spAxes;
}
private String[] createAxes() {
final String[] itAxes= new String[axes_.length];
for (int i= 0; i < axes_.length; i++) {
itAxes[i]= ((Axe)axes_[i]).titre_;
if ((itAxes[i] == null) || (itAxes[i].trim().equals(""))) {
itAxes[i]= "axe " + (i + 1);
}
}
return itAxes;
}
private void createMargeB() {
tfGrapheMargeB_= BuTextField.createIntegerField();
tfGrapheMargeB_.setColumns(3);
tfGrapheMargeB_.setValue(new Integer(graphe_.marges_.gauche_));
tfGrapheMargeB_.setActionCommand("GRAPHEMARGEB");
tfGrapheMargeB_.addActionListener(this);
}
private void createMargeH() {
tfGrapheMargeH_= BuTextField.createIntegerField();
tfGrapheMargeH_.setColumns(3);
tfGrapheMargeH_.setValue(new Integer(graphe_.marges_.haut_));
tfGrapheMargeH_.setActionCommand("GRAPHEMARGEH");
tfGrapheMargeH_.addActionListener(this);
}
private void createMargeG() {
tfGrapheMargeG_= BuTextField.createIntegerField();
tfGrapheMargeG_.setColumns(3);
tfGrapheMargeG_.setValue(new Integer(graphe_.marges_.gauche_));
tfGrapheMargeG_.setActionCommand("GRAPHEMARGEG");
tfGrapheMargeG_.addActionListener(this);
}
private void createMargeDr() {
tfGrapheMargeD_= BuTextField.createIntegerField();
tfGrapheMargeD_.setColumns(3);
tfGrapheMargeD_.setValue(new Integer(graphe_.marges_.droite_));
tfGrapheMargeD_.setActionCommand("GRAPHEMARGED");
tfGrapheMargeD_.addActionListener(this);
}
private void createBtChanger() {
btGrapheFond_= new JButton("Changer");
btGrapheFond_.setActionCommand("GRAPHEFOND");
btGrapheFond_.addActionListener(this);
}
private void createCbLegende() {
cbGrapheLegende_= new JCheckBox();
cbGrapheLegende_.setActionCommand("GRAPHELEGENDE");
cbGrapheLegende_.setSelected(graphe_.legende_);
cbGrapheLegende_.addActionListener(this);
}
private void createSousTitre() {
tfGrapheSousTitre_= new BuTextField();
tfGrapheSousTitre_.setColumns(8);
tfGrapheSousTitre_.setText(graphe_.soustitre_);
tfGrapheSousTitre_.setActionCommand("GRAPHESOUSTITRE");
tfGrapheSousTitre_.addActionListener(this);
}
private void createGrapheTitre() {
tfGrapheTitre_= new BuTextField();
tfGrapheTitre_.setColumns(8);
tfGrapheTitre_.setText(graphe_.titre_);
tfGrapheTitre_.setActionCommand("GRAPHETITRE");
tfGrapheTitre_.addActionListener(this);
}
@Override
public void valueChanged(final ListSelectionEvent _evt) {
final JList src= (JList)_evt.getSource();
final int ind= src.getSelectedIndex();
if (src == lsAxes_) {
if ((ind < 0) || (ind >= axes_.length)) {
return;
}
final Axe a= (Axe)axes_[ind];
tfAxeTitre_.setText(a.titre_);
cbAxeVisible_.setSelected(a.visible_);
cbAxeGraduations_.setSelected(a.graduations_);
cbAxeGrille_.setSelected(a.grille_);
tfAxePas_.setValue(new Double(a.pas_));
tfAxeEcart_.setValue(new Integer(a.ecart_));
if (a.vertical_) {
tfAxeEcart_.setEnabled(true);
}
btAxeCouleur_.setIcon(new BuColorIcon(a.aspect_.contour_));
} else if (src == lsCourbes_) {
if ((ind < 0) || (ind >= courbes_.length)) {
return;
}
final CourbeDefault c= (CourbeDefault)courbes_[ind];
tfCourbeTitre_.setText(c.titre_);
cbCourbeVisible_.setSelected(c.visible_);
cbCourbeMarqueurs_.setSelected(c.marqueurs_);
btCourbeCouleur_.setIcon(new BuColorIcon(c.aspect_.contour_));
}
}
@Override
public void propertyChange(final PropertyChangeEvent _evt) {
final String prop= _evt.getPropertyName();
if (prop.equals("GRAPHE_LABEL")) {
final Graphe g= (Graphe)_evt.getNewValue();
cbGrapheLegende_.setSelected(g.legende_);
} else if (prop.equals("GRAPHE_TITRE")) {
final String titre= (String)_evt.getNewValue();
tfGrapheTitre_.setText(titre);
} else if (prop.startsWith("AXE")) {
if (prop.endsWith("LABEL")) {
axes_= graphe_.getElementsParType((new Axe()).getClass());
lsAxes_.removeAll();
final String[] itAxes= new String[axes_.length];
for (int i= 0; i < axes_.length; i++) {
itAxes[i]= ((Axe)axes_[i]).titre_;
if ((itAxes[i] == null) || (itAxes[i].trim().equals(""))) {
itAxes[i]= "axe " + (i + 1);
}
}
lsAxes_.setListData(itAxes);
lsAxes_.setSelectedIndex(0);
}
final Axe a= (Axe)_evt.getNewValue();
if (lsAxes_.getSelectedValue().equals(a.titre_)) {
tfAxeTitre_.setText(a.titre_);
cbAxeVisible_.setSelected(a.visible_);
cbAxeGraduations_.setSelected(a.graduations_);
cbAxeGrille_.setSelected(a.grille_);
tfAxePas_.setValue(new Double(a.pas_));
tfAxeEcart_.setValue(new Integer(a.ecart_));
if (a.vertical_) {
tfAxeEcart_.setEnabled(true);
}
btAxeCouleur_.setIcon(new BuColorIcon(a.aspect_.contour_));
}
} else if (prop.equals("MARGE")) {
final Marges m= (Marges)_evt.getNewValue();
tfGrapheMargeB_.setValue(new Integer(m.bas_));
tfGrapheMargeD_.setValue(new Integer(m.droite_));
tfGrapheMargeG_.setValue(new Integer(m.gauche_));
tfGrapheMargeH_.setValue(new Integer(m.haut_));
} else if (prop.equals("COURBES")) {
courbes_= graphe_.getElementsParType(new CourbeDefault().getClass());
lsCourbes_.removeAll();
final String[] itCourbes= new String[courbes_.length];
for (int i= 0; i < courbes_.length; i++) {
itCourbes[i]= ((CourbeDefault)courbes_[i]).titre_;
if ((itCourbes[i] == null) || (itCourbes[i].trim().equals(""))) {
itCourbes[i]= "courbe " + (i + 1);
}
}
lsCourbes_.setListData(itCourbes);
lsCourbes_.setSelectedIndex(0);
}
}
@Override
public void actionPerformed(final ActionEvent _e) {
final String cmd= _e.getActionCommand();
// Object src=e.getSource();
final String marges = "grapheMarges";
if (cmd.equals("GRAPHETITRE")) {
final String text= tfGrapheTitre_.getText();
graphe_.titre_= text;
firePropertyChange("grapheTitre", null, null);
} else if (cmd.equals("GRAPHESOUSTITRE")) {
doGrapheSsTitre();
} else if (cmd.equals("GRAPHELEGENDE")) {
doGrapheLegende();
} else if (cmd.equals("GRAPHEMARGEG")) {
doMargeG(marges);
} else if (cmd.equals("GRAPHEMARGED")) {
doMargeD(marges);
} else if (cmd.equals("GRAPHEMARGEH")) {
doMargeH(marges);
} else if (cmd.equals("GRAPHEMARGEB")) {
doMargesB(marges);
} else if (cmd.equals("GRAPHEFOND")) {
doGrapheFond();
} else if (cmd.equals("COURBETITRE")) {
doCourbeTitre();
} else if (cmd.equals("COURBEVISIBLE")) {
doCourbeVisible();
} else if (cmd.equals("COURBEMARQUEURS")) {
doCourbeMarqueurs();
} else if (cmd.equals("COURBECOULEUR")) {
doCourbeCouleur();
} else if (cmd.equals("AXETITRE")) {
doAxeTitre();
} else if (cmd.equals("AXEVISIBLE")) {
doAxeVisible();
} else if (cmd.equals("AXEGRADUATIONS")) {
doAxeGraduation();
} else if (cmd.equals("AXEGRILLE")) {
doAxeGrille();
} else if (cmd.equals("AXEPAS")) {
doAxePas();
} else if (cmd.equals("AXEECART")) {
doAxeEcart();
} else if (cmd.equals("AXECOULEUR")) {
doAxeCouleur();
}
}
private void doAxeCouleur() {
final Axe a= (Axe)axes_[lsAxes_.getSelectedIndex()];
final Color oldc= a.aspect_.contour_;
final Color c= JColorChooser.showDialog(this, "Couleur", oldc);
btAxeCouleur_.setIcon(new BuColorIcon(c));
a.aspect_.contour_= c;
firePropertyChange("axeCouleur", oldc, c);
repaint();
}
private void doAxeEcart() {
final Object ecart= tfAxeEcart_.getValue();
if (ecart != null){
final Axe a= (Axe)axes_[lsAxes_.getSelectedIndex()];
a.ecart_= ((Integer)ecart).intValue();
firePropertyChange("axeEcart", null, null);
}
}
private void doAxePas() {
final Object pas= tfAxePas_.getValue();
if (pas != null){
final Axe a= (Axe)axes_[lsAxes_.getSelectedIndex()];
a.pas_= ((Double)pas).doubleValue();
firePropertyChange("axePas", null, null);
}
}
private void doAxeGrille() {
final boolean selected= cbAxeGrille_.isSelected();
final Axe a= (Axe)axes_[lsAxes_.getSelectedIndex()];
a.grille_= selected;
firePropertyChange("axeGrille", null, null);
}
private void doAxeGraduation() {
final boolean selected= cbAxeGraduations_.isSelected();
final Axe a= (Axe)axes_[lsAxes_.getSelectedIndex()];
a.graduations_= selected;
firePropertyChange("axeGraduations", null, null);
}
private void doAxeVisible() {
final boolean selected= cbAxeVisible_.isSelected();
final Axe a= (Axe)axes_[lsAxes_.getSelectedIndex()];
a.visible_= selected;
firePropertyChange("axeVisible", null, null);
}
private void doAxeTitre() {
final String text= tfAxeTitre_.getText();
final int ind= lsAxes_.getSelectedIndex();
final Axe a= (Axe)axes_[ind];
a.titre_= text;
final String[] itAxes= new String[axes_.length];
for (int i= 0; i < axes_.length; i++) {
itAxes[i]= ((Axe)axes_[i]).titre_;
if ((itAxes[i] == null) || (itAxes[i].trim().equals(""))) {
itAxes[i]= "axe " + (i + 1);
}
}
lsAxes_.setListData(itAxes);
lsAxes_.setSelectedIndex(ind);
firePropertyChange("axeTitre", null, null);
}
private void doCourbeCouleur() {
// String s=(String)lsCourbes.getSelectedValue();
final CourbeDefault crb= (CourbeDefault)courbes_[lsCourbes_.getSelectedIndex()];
final Color oldc= crb.aspect_.contour_;
final Color c= JColorChooser.showDialog(this, "Couleur", oldc);
btCourbeCouleur_.setIcon(new BuColorIcon(c));
crb.aspect_.contour_= c;
firePropertyChange("courbeCouleur", oldc, c);
repaint();
}
private void doCourbeMarqueurs() {
final boolean selected= cbCourbeMarqueurs_.isSelected();
final CourbeDefault c= (CourbeDefault)courbes_[lsCourbes_.getSelectedIndex()];
c.marqueurs_= selected;
firePropertyChange("courbeMarqueurs", null, null);
}
private void doCourbeVisible() {
final boolean selected= cbCourbeVisible_.isSelected();
final CourbeDefault c= (CourbeDefault)courbes_[lsCourbes_.getSelectedIndex()];
c.visible_= selected;
firePropertyChange("courbeVisible", null, null);
}
private void doCourbeTitre() {
final String text= tfCourbeTitre_.getText();
final int ind= lsCourbes_.getSelectedIndex();
final CourbeDefault c= (CourbeDefault)courbes_[ind];
c.titre_= text;
final String[] itCourbes= new String[courbes_.length];
for (int i= 0; i < courbes_.length; i++) {
itCourbes[i]= ((CourbeDefault)courbes_[i]).titre_;
if ((itCourbes[i] == null) || (itCourbes[i].trim().equals(""))) {
itCourbes[i]= "courbe " + (i + 1);
}
}
lsCourbes_.setListData(itCourbes);
lsCourbes_.setSelectedIndex(ind);
firePropertyChange("courbeTitre", null, null);
}
private void doGrapheFond() {
final JFileChooser fc = createFileChooser();
final int returnVal= fc.showOpenDialog(this);
if (returnVal == JFileChooser.APPROVE_OPTION) {
final String file= fc.getSelectedFile().getAbsolutePath();
final Image img= getToolkit().createImage(file);
final MediaTracker tracker= new MediaTracker(this);
tracker.addImage(img, 0);
try {
tracker.waitForID(0);
} catch (final InterruptedException ie) {}
graphe_.fond_= img;
firePropertyChange("grapheFond", null, null);
}
}
private void doMargesB(final String _marges) {
final Object v= tfGrapheMargeB_.getValue();
if (v != null){
graphe_.marges_.bas_= ((Integer)v).intValue();
firePropertyChange(_marges, null, null);
}
}
private void doMargeH(final String _marges) {
final Object v= tfGrapheMargeH_.getValue();
if (v != null){
graphe_.marges_.haut_= ((Integer)v).intValue();
firePropertyChange(_marges, null, null);
}
}
private void doMargeD(final String _marges) {
final Object v= tfGrapheMargeD_.getValue();
if (v != null){
graphe_.marges_.droite_= ((Integer)v).intValue();
firePropertyChange(_marges, null, null);
}
}
private void doMargeG(final String _marges) {
final Object v= tfGrapheMargeG_.getValue();
if (v != null){
graphe_.marges_.gauche_= ((Integer)v).intValue();
firePropertyChange(_marges, null, null);
}
}
private void doGrapheLegende() {
final boolean selected= cbGrapheLegende_.isSelected();
graphe_.legende_= selected;
firePropertyChange("grapheLegende", null, null);
}
private void doGrapheSsTitre() {
final String text= tfGrapheSousTitre_.getText();
graphe_.soustitre_= text;
firePropertyChange("grapheSousTitre", null, null);
}
private JFileChooser createFileChooser() {
final JFileChooser fc= new JFileChooser();
fc.setFileFilter(new javax.swing.filechooser.FileFilter() {
@Override
public boolean accept(final File _f) {
return _f.isDirectory()
|| _f.getName().endsWith(".gif")
|| _f.getName().endsWith(".GIF")
|| _f.getName().endsWith(".jpg")
|| _f.getName().endsWith(".JPG")
|| _f.getName().endsWith(".png")
|| _f.getName().endsWith(".PNG");
}
@Override
public String getDescription() {
return "Images GIF,JPG,PNG";
}
});
return fc;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy