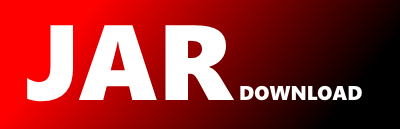
org.fudaa.ebli.graphe.Contrainte Maven / Gradle / Ivy
The newest version!
/*
* @creation 1999-07-29
* @modification $Date: 2006-09-19 14:55:45 $
* @license GNU General Public License 2
* @copyright (c)1998-2001 CETMEF 2 bd Gambetta F-60231 Compiegne
* @mail [email protected]
*/
package org.fudaa.ebli.graphe;
import java.awt.Color;
import java.awt.FontMetrics;
import java.awt.Graphics2D;
import com.memoire.fu.FuLog;
/**
* Contrainte.
*
* @version $Id: Contrainte.java,v 1.10 2006-09-19 14:55:45 deniger Exp $
* @author Guillaume Desnoix
*/
public class Contrainte implements GrapheComponent {
public String titre_;
public boolean visible_;
public String type_;
public int axe_;
public boolean vertical_;
public double s_;
public double v_;
public Color couleur_;
public Contrainte() {
titre_ = "";
visible_ = true;
type_ = "";
axe_ = 0;
vertical_ = true;
v_ = 0.;
s_ = Double.NaN;
couleur_ = Color.blue;
}
public static Contrainte parse(final Lecteur _lin) {
final Contrainte r = new Contrainte();
String t = _lin.get();
if ("contrainte".equals(t)) {
t = _lin.get();
}
if (!"{".equals(t)) {
System.err.println("Erreur de syntaxe: " + t);
}
t = _lin.get();
while (!"".equals(t) && !"}".equals(t)) {
if ("titre".equals(t)) {
r.titre_ = _lin.get();
} else if ("type".equals(t)) {
r.type_ = _lin.get();
} else if ("orientation".equals(t)) {
r.vertical_ = _lin.get().equals("vertical");
} else if ("axe".equals(t)) {
try {
r.axe_ = Integer.parseInt(_lin.get());
} catch (final NumberFormatException _e) {FuLog.error(_e);}
} else if (t.equals("valeur")) {
r.v_ = Double.valueOf(_lin.get()).doubleValue();
} else if (t.equals("position")) {
r.s_ = Double.valueOf(_lin.get()).doubleValue();
} else if (t.equals("couleur")) {
r.couleur_ = new Color(Integer.valueOf(_lin.get(), 16).intValue());
} else if (t.equals("visible")) {
r.visible_ = _lin.get().equals("oui");
} else {
FuLog.error("EGR: Erreur de syntaxe: " + t);
}
t = _lin.get();
}
return r;
}
@Override
public void dessine(final Graphics2D _g, final int _x, final int _y, final int _w, final int _h, final Axe _ax, final Axe _ay, final boolean _b) {
if (!visible_) {
return;
}
final FontMetrics fm = _g.getFontMetrics();
_g.setColor(couleur_);
if (vertical_) {
final int xe = _x + (int) (_w * ((s_ - _ax.minimum_) / (_ax.maximum_ - _ax.minimum_)));
final int ye = _y + _h - (int) (_h * ((v_ - _ay.minimum_) / (_ay.maximum_ - _ay.minimum_)));
if (type_.equals("min")) {
if (Double.isNaN(s_)) {
_g.drawLine(_x, ye, _x + _w - 1, ye);
_g.drawLine(_x + _w - 4, ye, _x + _w - 4, ye + 7);
_g.drawString(titre_, _x + _w - 6 - fm.stringWidth(titre_), ye + 11);
} else {
_g.drawLine(xe - 3, ye, xe + 3, ye);
_g.drawLine(xe, ye, xe, ye + 7);
_g.drawString(titre_, xe + 3, ye + 11);
}
} else if (type_.equals("max")) {
if (Double.isNaN(s_)) {
_g.drawLine(_x, ye, _x + _w - 1, ye);
_g.drawLine(_x + _w - 4, ye, _x + _w - 4, ye - 7);
_g.drawString(titre_, _x + _w - 6 - fm.stringWidth(titre_), ye - 3);
} else {
_g.drawLine(xe - 3, ye, xe + 3, ye);
_g.drawLine(xe, ye, xe, ye - 7);
_g.drawString(titre_, xe + 3, ye - 4);
}
} else {
_g.drawRect(xe - 1, ye - 1, 2, 2);
_g.drawString(titre_, xe + 2, ye + 4);
}
} else {
final int xe = _x + (int) (_w * ((v_ - _ax.minimum_) / (_ax.maximum_ - _ax.minimum_)));
final int ye = _y + _h - (int) (_h * ((s_ - _ay.minimum_) / (_ay.maximum_ - _ay.minimum_)));
if (type_.equals("min")) {
if (Double.isNaN(s_)) {
_g.drawLine(xe, _y, xe, _y + _h - 1);
_g.drawLine(xe - 7, _y + _h - 13, xe, _y + _h - 13);
_g.drawString(titre_, xe - 2 - fm.stringWidth(titre_), _y + _h - 3);
} else {
_g.drawLine(xe, ye - 3, xe, ye + 3);
_g.drawLine(xe - 7, ye, xe, ye);
_g.drawString(titre_, xe - 2 - fm.stringWidth(titre_), ye + 12);
}
} else if (type_.equals("max")) {
if (Double.isNaN(s_)) {
_g.drawLine(xe, _y, xe, _y + _h - 1);
_g.drawLine(xe, _y + 13, xe + 7, _y + 13);
_g.drawString(titre_, xe + 3, _y + 10);
} else {
_g.drawLine(xe, ye - 3, xe, ye + 3);
_g.drawLine(xe, ye, xe + 7, ye);
_g.drawString(titre_, xe + 3, ye - 2);
}
} else {
_g.drawRect(xe - 1, ye - 1, 2, 2);
_g.drawString(titre_, xe + 2, ye + 4);
}
}
}
public final boolean isCourbe() {
return false;
}
/**
*
*/
@Override
public String getTitre() {
return titre_;
}
/**
*
*/
@Override
public boolean isVisible() {
return visible_;
}
/**
*
*/
@Override
public int getAxeId() {
return axe_;
}
/**
*
*/
@Override
public Color getAspectContour() {
return Color.white;
}
/**
*
*/
@Override
public Color getAspectSurface() {
return Color.white;
}
/**
*
*/
@Override
public Color getAspectTexte() {
return couleur_;
}
/**
*
*/
@Override
public final boolean isLegendAvailable() {
return false;
}
@Override
public boolean setVisible(final boolean _r) {
if (visible_ != _r) {
visible_ = _r;
return true;
}
return false;
}
@Override
public boolean setAspectContour(final Color _c) {
if (_c == null) {
return false;
}
if ((couleur_ == _c) || (_c.equals(couleur_))) {
return false;
}
couleur_ = _c;
return true;
}
@Override
public boolean getMinMax(final double[] _xminXmaxYminYmax) {
return false;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy