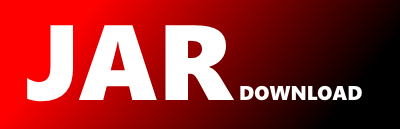
org.fudaa.ebli.graphe.Graphe Maven / Gradle / Ivy
The newest version!
/*
* @file Graphe.java
* @creation 1999-07-29
* @modification $Date: 2006-09-19 14:55:45 $
* @license GNU General Public License 2
* @copyright (c)1998-2001 CETMEF 2 bd Gambetta F-60231 Compiegne
* @mail [email protected]
*/
package org.fudaa.ebli.graphe;
import java.applet.Applet;
import java.awt.*;
import java.awt.image.ImageObserver;
import java.util.ArrayList;
import java.util.List;
import java.util.Vector;
import org.fudaa.ctulu.CtuluLibMessage;
import org.fudaa.ctulu.CtuluLibString;
/**
* Graphe.
*
* @version $Revision: 1.18 $ $Date: 2006-09-19 14:55:45 $ by $Author: deniger $
* @author Guillaume Desnoix
*/
public class Graphe {
public String titre_;
public String soustitre_;
public boolean legende_;
public boolean animation_;
public int vitesse_;
public Marges marges_;
private Vector grComponents_;
private Vector courbes_;
private Vector axesVertical_;
public Image fond_;
private Axe hAxe_;
private Axe firstAxe_;
private Font ftLegend_;
private Font ftTitre_;
private Font ftSousTitre_;
public boolean copyright_;
public boolean visible_;
public Graphe() {
titre_ = CtuluLibString.EMPTY_STRING;
soustitre_ = titre_;
legende_ = true;
animation_ = false;
vitesse_ = 1000;
marges_ = new Marges();
grComponents_ = new Vector(10);
axesVertical_ = new Vector(3);
courbes_ = new Vector(20);
hAxe_ = new Axe();
fond_ = null;
copyright_ = true;
visible_ = true;
final String type = "SansSerif";
ftTitre_ = new Font(type, Font.BOLD, 14);
ftSousTitre_ = new Font(type, Font.PLAIN, 12);
ftLegend_ = new Font(type, Font.ITALIC, 10);
}
public Vector getCourbes() {
return courbes_;
}
public Axe getHorizontalAxe() {
return hAxe_;
}
public double[] getMinMaxXY() {
final double[] minMax = new double[4];
boolean init = false;
double xmin = 0;
double xmax = 0;
double ymin = 0;
double ymax = 0;
final List r = new ArrayList(grComponents_.size() + courbes_.size());
r.addAll(grComponents_);
r.addAll(courbes_);
for (int i = r.size() - 1; i >= 0; i--) {
if (((GrapheComponent) r.get(i)).getMinMax(minMax)) {
if (!init) {
xmin = minMax[0];
xmax = minMax[1];
ymin = minMax[2];
ymax = minMax[3];
init = true;
} else {
if (minMax[0] < xmin) {
xmin = minMax[0];
}
if (minMax[1] > xmax) {
xmax = minMax[1];
}
if (minMax[2] < ymin) {
ymin = minMax[2];
}
if (minMax[3] > ymax) {
ymax = minMax[3];
}
}
}
}
minMax[0] = xmin;
minMax[1] = xmax;
minMax[2] = ymin;
minMax[3] = ymax;
return minMax;
}
public boolean restaurer() {
final double[] minMax = getMinMaxXY();
boolean r = false;
if (hAxe_.maximum_ != minMax[1]) {
hAxe_.maximum_ = minMax[1];
r = true;
}
if (hAxe_.minimum_ != minMax[0]) {
hAxe_.minimum_ = minMax[0];
r = true;
}
final Axe a = getFirstVerticalAxe();
if (a.maximum_ != minMax[3]) {
r = true;
a.maximum_ = minMax[3];
}
if (a.minimum_ != minMax[2]) {
a.minimum_ = minMax[2];
r = true;
}
return r;
}
public Axe getFirstVerticalAxe() {
return firstAxe_;
}
public static Graphe parse(final Lecteur _lin, final Applet _applet) {
final Graphe r = new Graphe();
String t = _lin.get();
if (t.equals("graphe")) {
t = _lin.get();
}
if (!t.equals("{")) {
System.err.println("Erreur de syntaxe: " + t);
}
t = _lin.get();
while (!t.equals("") && !t.equals("}")) {
final String oui = "oui";
if (t.equals("titre")) {
r.titre_ = _lin.get();
} else if (t.equals("sous-titre")) {
r.soustitre_ = _lin.get();
} else if (t.equals("legende")) {
r.legende_ = _lin.get().equals(oui);
} else if (t.equals("animation")) {
r.animation_ = _lin.get().equals(oui);
} else if (t.equals("copyright")) {
r.copyright_ = _lin.get().equals(oui);
} else if (t.equals("vitesse")) {
r.vitesse_ = Integer.valueOf(_lin.get()).intValue();
} else if (t.equals("fond")) {
;
} else if (t.equals("marges")) {
r.marges_ = Marges.parse(_lin);
} else if (t.equals("axe")) {
r.ajoute(Axe.parse(_lin));
} else if (t.equals("courbe")) {
r.grComponents_.add(CourbeDefault.parse(_lin));
} else if (t.equals("repartition")) {
r.grComponents_.add(Repartition.parse(_lin));
} else if (t.equals("contrainte")) {
r.grComponents_.add(Contrainte.parse(_lin));
} else if (t.equals("visible")) {
r.visible_ = _lin.get().equals(oui);
} else {
System.err.println("Erreur de syntaxe: " + t);
}
t = _lin.get();
}
return r;
}
public static Graphe parseXY(final Lecteur _lin) {
final Graphe r = new Graphe();
final CourbeDefault c = CourbeDefault.parseXY(_lin);
final Axe v = new Axe();
v.vertical_ = true;
v.minimum_ = c.getMinY();
v.maximum_ = c.getMaxY();
v.titre_ = "Y";
final Axe h = new Axe();
h.minimum_ = c.getMinX();
h.titre_ = "X";
h.maximum_ = c.getMaxX();
r.axesVertical_.addElement(v);
r.axesVertical_.addElement(h);
r.grComponents_.add(c);
return r;
}
public final void ajouteComponent(final GrapheComponent _c) {
if (!grComponents_.contains(_c)) {
grComponents_.add(_c);
}
}
public final int getNbGrapheComponent() {
return grComponents_.size();
}
public final GrapheComponent getGrapheComponent(final int _i) {
return (GrapheComponent) grComponents_.get(_i);
}
public final boolean setAllVisible(final boolean _b) {
boolean r = false;
final int n = grComponents_.size() - 1;
for (int i = n; i >= 0; i--) {
r |= ((GrapheComponent) grComponents_.get(i)).setVisible(_b);
}
return r;
}
public final boolean setOnlyOneVisible(final GrapheComponent _c) {
boolean r = false;
final int n = grComponents_.size() - 1;
for (int i = n; i >= 0; i--) {
final GrapheComponent c = getGrapheComponent(i);
r |= c.setVisible(c == _c);
}
return r;
}
public void ajoute(final Axe _a) {
if (_a == null) {
return;
}
if (_a.vertical_) {
if (!axesVertical_.contains(_a)) {
axesVertical_.add(_a);
}
if (firstAxe_ == null) {
firstAxe_ = _a;
}
} else {
hAxe_ = _a;
}
}
public void ajoute(final Courbe _c) {
if (_c != null) {
courbes_.add(_c);
}
}
public void ajoute(final Repartition _c) {
ajouteComponent(_c);
}
public void ajoute(final Contrainte _c) {
ajouteComponent(_c);
}
public void ajoute(final Object _el) {
if (_el instanceof Axe) {
ajoute((Axe) _el);
} else if (_el instanceof Courbe) {
ajoute((Courbe) _el);
} else if (_el instanceof GrapheComponent) {
ajouteComponent((GrapheComponent) _el);
} else {
CtuluLibMessage.error("?l?ment " + _el.getClass().getName() + " inconnu");
}
}
public void enleve(final Object _el) {
if (!courbes_.remove(_el)) {
if (!grComponents_.removeElement(_el)) {
axesVertical_.remove(_el);
if (_el == firstAxe_) {
firstAxe_ = null;
}
}
}
}
public Object[] getElementsParType(final Class _c) {
final List cs = new ArrayList();
if (_c.equals(Axe.class)) {
cs.add(hAxe_);
cs.addAll(axesVertical_);
return cs.toArray();
}
for (int i = 0; i < grComponents_.size(); i++) {
if (grComponents_.get(i).getClass() == _c) {
cs.add(grComponents_.get(i));
}
}
for (int i = 0; i < this.courbes_.size(); i++) {
if (courbes_.get(i).getClass() == _c) {
cs.add(courbes_.get(i));
}
}
return cs.toArray();
}
public void dessine(final Graphics _g, final int _x, final int _y, final int _w, final int _h, final int _nr, final ImageObserver _applet) {
if (!visible_) {
return;
}
final Graphics2D g2d = (Graphics2D) _g;
if ((fond_ != null) && (fond_.getWidth(_applet) > 0)) {
g2d.drawImage(fond_, _x, _y, _w, _h, _applet);
} else {
g2d.setColor(Color.white);
g2d.fillRect(_x, _y, _w, _h);
}
Font f;
FontMetrics fm;
f = ftTitre_;
g2d.setFont(f);
g2d.setColor(Color.black);
fm = g2d.getFontMetrics();
int legendeYShift = _y;
g2d.drawString(titre_, _x + (_w - fm.stringWidth(titre_)) / 2, _y + (marges_.haut_ + f.getSize()) / 2);
if ((titre_ != null) && (titre_.length() > 0)) {
final int max = (_y + (marges_.haut_ + f.getSize()) / 2);
if (max > legendeYShift) {
legendeYShift = max;
}
}
f = ftSousTitre_;
g2d.setFont(f);
g2d.setColor(Color.black);
fm = g2d.getFontMetrics();
g2d.drawString(soustitre_, _x + (_w - fm.stringWidth(soustitre_)) / 2, _y + marges_.haut_ - 2);
legendeYShift = printCopyright(_x, _y, _w, g2d, legendeYShift);
g2d.setColor(Color.black);
f = new Font("SansSerif", Font.PLAIN, 10);
g2d.setFont(f);
final int nbElement = grComponents_.size();
final int nbCourbe = courbes_.size();
final int nbAxe = axesVertical_.size();
final int hMarge = _h - marges_.haut_ - marges_.bas_;
final int wMarge = _w - marges_.gauche_ - marges_.droite_;
final int xMarge = _x + marges_.gauche_;
final int yMarge = _y + marges_.haut_;
for (int i = 0; i < nbAxe; i++) {
final Axe a = (Axe) axesVertical_.get(i);
a.dessine(g2d, xMarge - i * a.ecart_, yMarge, wMarge + i * a.ecart_, hMarge);
}
// dessine axe horizontal
hAxe_.dessine(g2d, xMarge, yMarge + hMarge, wMarge, hMarge);
Axe aayDefault = null;
final Shape oldClip = g2d.getClip();
g2d.clip(new Rectangle(xMarge, yMarge, wMarge, hMarge));
aayDefault = dessineAxes(g2d, nbElement, hMarge, wMarge, xMarge, yMarge, aayDefault);
for (int i = 0; i < nbCourbe; i++) {
if (animation_ && (i != _nr)) {
return;
}
final Courbe o = (Courbe) courbes_.get(i);
final int axe = o.getAxeId();
Axe aay = null;
if (axe >= 0) {
if (axe >= axesVertical_.size()) {
if (aayDefault == null) {
aayDefault = new Axe();
}
aay = aayDefault;
} else {
aay = (Axe) axesVertical_.get(axe);
}
}
o.dessine(g2d, xMarge, yMarge, wMarge, hMarge, hAxe_, aay, true);
}
g2d.setClip(oldClip);
dessineLegende(_x, _w, g2d, legendeYShift, nbElement, nbCourbe);
}
private void dessineLegende(final int _x, final int _w, final Graphics2D _g2d, final int _legendeYShift, final int _nbElement, final int _nbCourbe) {
Font f;
FontMetrics fm;
if (legende_ && !animation_) {
f = ftLegend_;
_g2d.setFont(f);
// on dessine a partir de 15 du haut.
int maxWidthForLegend = 0;
fm = _g2d.getFontMetrics();
maxWidthForLegend = dessineLegendeComponents(_nbElement, fm, maxWidthForLegend);
maxWidthForLegend = dessineLegendeCourbes(_nbCourbe, fm, maxWidthForLegend);
if (maxWidthForLegend == 0) {
return;
}
final int wRect = 12;
final int hRect = 4;
final int wTrait = 11;
final int hTrait = hRect / 2;
final int xLeft = _x + _w - 15 - maxWidthForLegend - (marges_.droite_ / 2) - wRect;
final int xTitre = xLeft + wRect + 15;
int hLine = fm.getHeight();
// Au cas ou la hauteur de ligne soit inferieur a la hauteur du carre.
if (hLine < (hRect + 2)) {
hLine = hRect + 2;
}
int yl = _legendeYShift + 10 + hLine;
final Color old = _g2d.getColor();
for (int i = 0; i < _nbElement; i++) {
final GrapheComponent c = (GrapheComponent) grComponents_.get(i);
if (c.isLegendAvailable() && c.isVisible() && (c.getTitre() != null) && (c.getTitre().length() > 0)) {
_g2d.setColor(c.getAspectSurface());
_g2d.fillRect(xLeft, yl - hRect, wRect, hRect);
_g2d.setColor(c.getAspectContour());
_g2d.fillRect(xLeft, yl - ((hRect - hTrait) / 2) - hTrait, wTrait, hTrait);
_g2d.drawString(c.getTitre(), xTitre, yl);
}
yl += hLine;
}
for (int i = 0; i < _nbCourbe; i++) {
final GrapheComponent c = (GrapheComponent) courbes_.get(i);
if (c.isLegendAvailable() && c.isVisible() && (c.getTitre() != null) && (c.getTitre().length() > 0)) {
_g2d.setColor(c.getAspectSurface());
_g2d.fillRect(xLeft, yl - hRect, wRect, hRect);
_g2d.setColor(c.getAspectContour());
_g2d.fillRect(xLeft, yl - ((hRect - hTrait) / 2) - hTrait, wTrait, hTrait);
_g2d.drawString(c.getTitre(), xTitre, yl);
}
yl += hLine;
}
_g2d.setColor(old);
}
}
private int dessineLegendeCourbes(final int _nbCourbe, final FontMetrics _fm, final int _maxWidthForLegend) {
int res = _maxWidthForLegend;
for (int i = _nbCourbe - 1; i >= 0; i--) {
int temp;
final GrapheComponent c = (GrapheComponent) courbes_.get(i);
if (c.isLegendAvailable() && c.isVisible()) {
temp = _fm.stringWidth(c.getTitre());
if (temp > res) {
res = temp;
}
}
}
return res;
}
private int dessineLegendeComponents(final int _nbElement, final FontMetrics _fm, final int _maxWidthForLegend) {
int res = _maxWidthForLegend;
for (int i = _nbElement - 1; i >= 0; i--) {
int temp;
final GrapheComponent c = (GrapheComponent) grComponents_.get(i);
if (c.isLegendAvailable() && c.isVisible()) {
temp = _fm.stringWidth(c.getTitre());
if (temp > res) {
res = temp;
}
}
}
return res;
}
private Axe dessineAxes(final Graphics2D _g2d, final int _nbElement, final int _hMarge, final int _wMarge, final int _xMarge, final int _yMarge,
final Axe _aayDefault) {
Axe res = _aayDefault;
for (int i = 0; i < _nbElement; i++) {
final GrapheComponent o = (GrapheComponent) grComponents_.get(i);
final int axe = o.getAxeId();
Axe aay = null;
if (axe >= 0) {
if (axe >= axesVertical_.size()) {
if (res == null) {
res = new Axe();
}
aay = res;
} else {
aay = (Axe) axesVertical_.get(axe);
}
}
o.dessine(_g2d, _xMarge, _yMarge, _wMarge, _hMarge, hAxe_, aay, true);
}
return res;
}
private int printCopyright(final int _x, final int _y, final int _w, final Graphics2D _g2d, final int _legendeYShift) {
Font f;
FontMetrics fm;
int res=_legendeYShift;
if (copyright_) {
_g2d.setColor(Color.orange);
f = new Font("SansSerif", Font.BOLD, 8);
_g2d.setFont(f);
final String t = "Ebli Graphique 1D - CETMEF 1997/2002";
fm = _g2d.getFontMetrics();
_g2d.drawString(t, _x + _w - 2 - fm.stringWidth(t), _y + f.getSize() + 5);
final int max = _y + f.getSize() + 5;
if (max > res) {
res = max;
}
}
return res;
}
public Font getFtLegend() {
return ftLegend_;
}
public Font getFtSousTitre() {
return ftSousTitre_;
}
public Font getFtTitre() {
return ftTitre_;
}
public void setFtLegend(final Font _font) {
ftLegend_ = _font;
}
public void setFtSousTitre(final Font _font) {
ftSousTitre_ = _font;
}
public void setFtTitre(final Font _font) {
ftTitre_ = _font;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy