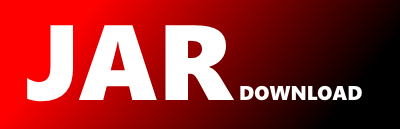
org.fuin.srcgen4j.commons.ParserConfig Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of srcgen4j-commons Show documentation
Show all versions of srcgen4j-commons Show documentation
Source code generation for Java (Commons)
The newest version!
/**
* Copyright (C) 2015 Michael Schnell. All rights reserved.
* http://www.fuin.org/
*
* This library is free software; you can redistribute it and/or modify it under
* the terms of the GNU Lesser General Public License as published by the Free
* Software Foundation; either version 3 of the License, or (at your option) any
* later version.
*
* This library is distributed in the hope that it will be useful, but WITHOUT
* ANY WARRANTY; without even the implied warranty of MERCHANTABILITY or FITNESS
* FOR A PARTICULAR PURPOSE. See the GNU Lesser General Public License for more
* details.
*
* You should have received a copy of the GNU Lesser General Public License
* along with this library. If not, see http://www.gnu.org/licenses/.
*/
package org.fuin.srcgen4j.commons;
import static org.fuin.utils4j.Utils4J.replaceVars;
import java.util.Map;
import javax.annotation.Nullable;
import javax.validation.Valid;
import javax.validation.constraints.NotEmpty;
import javax.validation.constraints.NotNull;
import javax.xml.bind.annotation.XmlAccessType;
import javax.xml.bind.annotation.XmlAccessorType;
import javax.xml.bind.annotation.XmlAttribute;
import javax.xml.bind.annotation.XmlElement;
import javax.xml.bind.annotation.XmlRootElement;
import javax.xml.bind.annotation.XmlType;
import org.fuin.objects4j.common.Contract;
import org.fuin.utils4j.Utils4J;
import org.fuin.xmlcfg4j.AbstractNamedElement;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
/**
* Represents a model parser.
*/
@XmlAccessorType(XmlAccessType.FIELD)
@XmlRootElement(name = "parser")
@XmlType(propOrder = { "config", "className" })
public class ParserConfig extends AbstractNamedElement implements InitializableElement {
private static final Logger LOG = LoggerFactory.getLogger(ParserConfig.class);
@NotEmpty
@XmlAttribute(name = "class")
private String className;
@Nullable
@Valid
@XmlElement(name = "config")
private Config config;
@Nullable
private transient SrcGen4JContext context;
@Nullable
private transient Parsers parent;
@Nullable
private transient Parser
© 2015 - 2024 Weber Informatics LLC | Privacy Policy