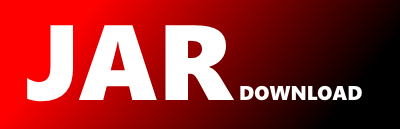
org.fuin.utils4j.jaxb.JaxbUtils Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of utils4j Show documentation
Show all versions of utils4j Show documentation
A small Java library that contains several helpful utility classes.
The newest version!
/**
* Copyright (C) 2015 Michael Schnell. All rights reserved.
* http://www.fuin.org/
*
* This library is free software; you can redistribute it and/or modify it under
* the terms of the GNU Lesser General Public License as published by the Free
* Software Foundation; either version 3 of the License, or (at your option) any
* later version.
*
* This library is distributed in the hope that it will be useful, but WITHOUT
* ANY WARRANTY; without even the implied warranty of MERCHANTABILITY or FITNESS
* FOR A PARTICULAR PURPOSE. See the GNU Lesser General Public License for more
* details.
*
* You should have received a copy of the GNU Lesser General Public License
* along with this library. If not, see http://www.gnu.org/licenses/.
*/
package org.fuin.utils4j.jaxb;
import jakarta.xml.bind.JAXBContext;
import jakarta.xml.bind.JAXBException;
import jakarta.xml.bind.Marshaller;
import jakarta.xml.bind.Unmarshaller;
import jakarta.xml.bind.annotation.adapters.XmlAdapter;
import org.fuin.utils4j.Utils4J;
import javax.xml.stream.XMLStreamWriter;
import java.io.*;
/**
* JAXB releated functions.
*/
public final class JaxbUtils {
private static final String ERROR_MARSHALLING_TEST_DATA = "Error marshalling test data";
/** Standard XML prefix with UTF-8 encoding. */
public static final String XML_PREFIX = "";
private JaxbUtils() {
throw new UnsupportedOperationException("It's not allowed to create an instance of a utility class");
}
/**
* Marshals the given data. A null
data argument returns null
.
*
* @param data
* Data to serialize or null
.
* @param classesToBeBound
* List of java classes to be recognized by the {@link JAXBContext} - Cannot be null
.
*
* @return XML data or null
.
*
* @param
* Type of the data.
* @deprecated Use method {@link #marshal(Marshaller, Object)} together with {@link MarshallerBuilder} instead
*/
public static String marshal(final T data, final Class>... classesToBeBound) {
return marshal(data, null, classesToBeBound);
}
/**
* Marshals the given data. A null
data argument returns null
.
*
* @param data
* Data to serialize or null
.
* @param adapters
* Adapters to associate with the marshaller or null
.
* @param classesToBeBound
* List of java classes to be recognized by the {@link JAXBContext} - Cannot be null
.
*
* @return XML data or null
.
*
* @param
* Type of the data.
* @deprecated Use method {@link #marshal(Marshaller, Object)} together with {@link MarshallerBuilder} instead
*/
public static String marshal(final T data, final XmlAdapter, ?>[] adapters, final Class>... classesToBeBound) {
if (data == null) {
return null;
}
try {
final JAXBContext ctx = JAXBContext.newInstance(classesToBeBound);
return marshal(ctx, data, adapters);
} catch (final JAXBException ex) {
throw new RuntimeException(ERROR_MARSHALLING_TEST_DATA, ex);
}
}
/**
* Marshals the given data using a given context. A null
data argument returns null
.
*
* @param ctx
* Context to use - Cannot be null
.
* @param data
* Data to serialize or null
.
*
* @return XML data or null
.
*
* @param
* Type of the data.
* @deprecated Use method {@link #marshal(Marshaller, Object)} together with {@link MarshallerBuilder} instead
*/
public static String marshal(final JAXBContext ctx, final T data) {
return marshal(ctx, data, null);
}
/**
* Marshals the given data using a given context. A null
data argument returns null
.
*
* @param ctx
* Context to use - Cannot be null
.
* @param data
* Data to serialize or null
.
* @param adapters
* Adapters to associate with the marshaller or null
.
*
* @return XML data or null
.
*
* @param
* Type of the data.
* @deprecated Use method {@link #marshal(Marshaller, Object)} together with {@link MarshallerBuilder} instead
*/
public static String marshal(final JAXBContext ctx, final T data, final XmlAdapter, ?>[] adapters) {
if (data == null) {
return null;
}
final StringWriter writer = new StringWriter();
marshal(ctx, data, adapters, writer);
return writer.toString();
}
/**
* Marshals the given data using a given context. A null
data argument returns null
.
*
* @param ctx
* Context to use - Cannot be null
.
* @param data
* Data to serialize or null
.
* @param adapters
* Adapters to associate with the marshaller or null
.
* @param writer
* Writer to use.
*
* @param
* Type of the data to write.
* @deprecated Use method {@link #marshal(Marshaller, Object, Writer)} together with {@link MarshallerBuilder} instead
*/
public static void marshal(final JAXBContext ctx, final T data, final XmlAdapter, ?>[] adapters, final Writer writer) {
if (data == null) {
return;
}
try {
final Marshaller marshaller = ctx.createMarshaller();
if (adapters != null) {
for (final XmlAdapter, ?> adapter : adapters) {
marshaller.setAdapter(adapter);
}
}
marshaller.marshal(data, writer);
} catch (final JAXBException ex) {
throw new RuntimeException(ERROR_MARSHALLING_TEST_DATA, ex);
}
}
/**
* Marshals the given data using a given context. A null
data argument returns null
.
*
* @param ctx
* Context to use - Cannot be null
.
* @param data
* Data to serialize or null
.
* @param adapters
* Adapters to associate with the marshaller or null
.
* @param writer
* Writer to use.
*
* @param
* Type of the data to write.
* @deprecated Use method {@link #marshal(Marshaller, Object, XMLStreamWriter)} together with {@link MarshallerBuilder} instead
*/
public static void marshal(final JAXBContext ctx, final T data, final XmlAdapter, ?>[] adapters, final XMLStreamWriter writer) {
if (data == null) {
return;
}
try {
final Marshaller marshaller = ctx.createMarshaller();
if (adapters != null) {
for (final XmlAdapter, ?> adapter : adapters) {
marshaller.setAdapter(adapter);
}
}
marshaller.marshal(data, writer);
} catch (final JAXBException ex) {
throw new RuntimeException(ERROR_MARSHALLING_TEST_DATA, ex);
}
}
/**
* Unmarshals the given data. A null
XML data argument returns null
.
*
* @param xmlData
* XML data or null
.
* @param classesToBeBound
* List of java classes to be recognized by the {@link JAXBContext} - Cannot be null
.
*
* @return Data or null
.
*
* @param
* Type of the expected data.
*
* @deprecated Use method {@link #unmarshal(Unmarshaller, String)} together with {@link UnmarshallerBuilder} instead
*/
public static T unmarshal(final String xmlData, final Class>... classesToBeBound) {
final UnmarshallerBuilder builder = new UnmarshallerBuilder();
if (classesToBeBound != null) {
builder.addClassesToBeBound(classesToBeBound);
}
builder.withHandler(event -> { return false; });
return unmarshal(builder.build(), xmlData);
}
/**
* Unmarshals the given data. A null
XML data argument returns null
.
*
* @param xmlData
* XML data or null
.
* @param adapters
* Adapters to associate with the unmarshaller or null
.
* @param classesToBeBound
* List of java classes to be recognized by the {@link JAXBContext} - Cannot be null
.
*
* @return Data or null
.
*
* @param
* Type of the expected data.
*
* @deprecated Use method {@link #unmarshal(Unmarshaller, String)} together with {@link UnmarshallerBuilder} instead
*/
public static T unmarshal(final String xmlData, final XmlAdapter, ?>[] adapters, final Class>... classesToBeBound) {
final UnmarshallerBuilder builder = new UnmarshallerBuilder();
if (classesToBeBound != null) {
builder.addClassesToBeBound(classesToBeBound);
}
builder.addAdapters(adapters);
builder.withHandler(event -> { return false; });
return unmarshal(builder.build(), xmlData);
}
/**
* Unmarshals the given data using a given context. A null
XML data argument returns null
.
*
* @param ctx
* Context to use - Cannot be null
.
* @param xmlData
* XML data or null
.
* @param adapters
* Adapters to associate with the unmarshaller or null
.
*
* @return Data or null
.
*
* @param
* Type of the expected data.
*
* @deprecated Use method {@link #unmarshal(Unmarshaller, String)} together with {@link UnmarshallerBuilder} instead
*/
public static T unmarshal(final JAXBContext ctx, final String xmlData, final XmlAdapter, ?>[] adapters) {
final UnmarshallerBuilder builder = new UnmarshallerBuilder().withContext(ctx);
if (adapters != null) {
builder.addAdapters(adapters);
}
builder.withHandler(event -> { return false; });
return unmarshal(builder.build(), xmlData);
}
/**
* Unmarshals the given data using a given context. A null
XML data argument returns null
.
*
* @param ctx
* Context to use - Cannot be null
.
* @param reader
* Reader with XML data.
* @param adapters
* Adapters to associate with the unmarshaller or null
.
*
* @return Data or null
.
*
* @param
* Type of the expected data.
*
* @deprecated Use method {@link #unmarshal(Unmarshaller, Reader)} together with {@link UnmarshallerBuilder} instead
*/
public static T unmarshal(final JAXBContext ctx, final Reader reader, final XmlAdapter, ?>[] adapters) {
final UnmarshallerBuilder builder = new UnmarshallerBuilder().withContext(ctx);
if (adapters != null) {
builder.addAdapters(adapters);
}
builder.withHandler(event -> { return false; });
return unmarshal(builder.build(), reader);
}
/**
* Convenience method to unmarshal an object from a string.
*
* @param unmarshaller
* Unmarshaller to use.
* @param xml
* XML source to parse or null
.
*
* @return Unmarshalled object or null
(in case the provided XML source argument was null).
*
* @param
* Type returned.
*/
@SuppressWarnings("unchecked")
public static T unmarshal(final Unmarshaller unmarshaller, final String xml) {
Utils4J.checkNotNull("unmarshaller", unmarshaller);
if (xml == null) {
return null;
}
try {
return (T) unmarshaller.unmarshal(new StringReader(xml));
} catch (final JAXBException ex) {
throw new RuntimeException("Error unmarshalling from XML: " + xml, ex);
}
}
/**
* Convenience method to unmarshal an object from a reader.
*
* @param unmarshaller
* Unmarshaller to use.
* @param reader
* Reader with XML source to parse.
*
* @return Unmarshalled object.
*
* @param
* Type returned.
*/
@SuppressWarnings("unchecked")
public static T unmarshal(final Unmarshaller unmarshaller, final Reader reader) {
Utils4J.checkNotNull("unmarshaller", unmarshaller);
Utils4J.checkNotNull("reader", reader);
try {
return (T) unmarshaller.unmarshal(reader);
} catch (final JAXBException ex) {
throw new RuntimeException("Error unmarshalling from reader", ex);
}
}
/**
* Convenience method to unmarshal an object from a file.
*
* @param unmarshaller
* Unmarshaller to use.
* @param file
* File with XML source to parse. Cannot be null
and must be an existing file.
*
* @return Unmarshalled object.
*
* @param
* Type returned.
*/
@SuppressWarnings("unchecked")
public static T unmarshal(final Unmarshaller unmarshaller, final File file) {
Utils4J.checkNotNull("unmarshaller", unmarshaller);
Utils4J.checkNotNull("file", file);
Utils4J.checkValidFile(file);
try (final FileReader reader = new FileReader(file)) {
return (T) unmarshaller.unmarshal(reader);
} catch (final JAXBException | IOException ex) {
throw new RuntimeException("Error unmarshalling from file: " + file, ex);
}
}
/**
* Convenience method to marshals the given data to string. A null
data argument returns null
.
*
* @param marshaller
* Marshaller to use - Cannot be null
.
* @param data
* Data to serialize or null
.
*
* @return XML data or null
.
*
* @param
* Type of the data.
*/
public static String marshal(final Marshaller marshaller, final T data) {
Utils4J.checkNotNull("marshaller", marshaller);
if (data == null) {
return null;
}
final StringWriter writer = new StringWriter();
marshal(marshaller, data, writer);
return writer.toString();
}
/**
* Convenience method to marshals the given data using a writer. A null
data argument returns null
.
*
* @param marshaller
* Marshaller to use - Cannot be null
.
* @param data
* Data to serialize or null
.
* @param writer
* Writer to use.
*
* @param
* Type of the data to write.
*/
public static void marshal(final Marshaller marshaller, final T data, final Writer writer) {
Utils4J.checkNotNull("marshaller", marshaller);
Utils4J.checkNotNull("writer", writer);
if (data == null) {
return;
}
try {
marshaller.marshal(data, writer);
} catch (final JAXBException ex) {
throw new RuntimeException(ERROR_MARSHALLING_TEST_DATA, ex);
}
}
/**
* Convenience method to marshals the given data using an XML stream writer. A null
data argument returns null
.
*
* @param marshaller
* Marshaller to use - Cannot be null
.
* @param data
* Data to serialize or null
.
* @param writer
* Writer to use.
*
* @param
* Type of the data to write.
*/
public static void marshal(final Marshaller marshaller, final T data, final XMLStreamWriter writer) {
Utils4J.checkNotNull("marshaller", marshaller);
Utils4J.checkNotNull("writer", writer);
if (data == null) {
return;
}
try {
marshaller.marshal(data, writer);
} catch (final JAXBException ex) {
throw new RuntimeException(ERROR_MARSHALLING_TEST_DATA, ex);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy