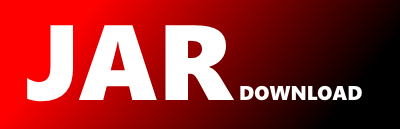
org.fuin.utils4j.JaxbUtils Maven / Gradle / Ivy
/**
* Copyright (C) 2015 Michael Schnell. All rights reserved.
* http://www.fuin.org/
*
* This library is free software; you can redistribute it and/or modify it under
* the terms of the GNU Lesser General Public License as published by the Free
* Software Foundation; either version 3 of the License, or (at your option) any
* later version.
*
* This library is distributed in the hope that it will be useful, but WITHOUT
* ANY WARRANTY; without even the implied warranty of MERCHANTABILITY or FITNESS
* FOR A PARTICULAR PURPOSE. See the GNU Lesser General Public License for more
* details.
*
* You should have received a copy of the GNU Lesser General Public License
* along with this library. If not, see http://www.gnu.org/licenses/.
*/
package org.fuin.utils4j;
import java.io.StringReader;
import java.io.StringWriter;
import javax.xml.bind.JAXBContext;
import javax.xml.bind.JAXBException;
import javax.xml.bind.Marshaller;
import javax.xml.bind.Unmarshaller;
import javax.xml.bind.ValidationEvent;
import javax.xml.bind.ValidationEventHandler;
import javax.xml.bind.annotation.adapters.XmlAdapter;
/**
* JAXB releated functions.
*/
public final class JaxbUtils {
/** Standard XML prefix with UTF-8 encoding. */
public static final String XML_PREFIX = "";
private JaxbUtils() {
throw new UnsupportedOperationException("It's not allowed to create an instance of a utility class");
}
/**
* Marshals the given data. A null
data argument returns null
.
*
* @param data
* Data to serialize or null
.
* @param classesToBeBound
* List of java classes to be recognized by the {@link JAXBContext} - Cannot be
* null
.
*
* @return XML data or null
.
*
* @param
* Type of the data.
*/
public static String marshal(final T data, final Class>... classesToBeBound) {
return marshal(data, null, classesToBeBound);
}
/**
* Marshals the given data. A null
data argument returns null
.
*
* @param data
* Data to serialize or null
.
* @param adapters
* Adapters to associate with the marshaller or null
.
* @param classesToBeBound
* List of java classes to be recognized by the {@link JAXBContext} - Cannot be
* null
.
*
* @return XML data or null
.
*
* @param
* Type of the data.
*/
public static String marshal(final T data, final XmlAdapter, ?>[] adapters,
final Class>... classesToBeBound) {
if (data == null) {
return null;
}
try {
final JAXBContext ctx = JAXBContext.newInstance(classesToBeBound);
return marshal(ctx, data, adapters);
} catch (final JAXBException ex) {
throw new RuntimeException("Error marshalling test data", ex);
}
}
/**
* Marshals the given data using a given context. A null
data argument returns
* null
.
*
* @param ctx
* Context to use - Cannot be null
.
* @param data
* Data to serialize or null
.
*
* @return XML data or null
.
*
* @param
* Type of the data.
*/
public static String marshal(final JAXBContext ctx, final T data) {
return marshal(ctx, data, null);
}
/**
* Marshals the given data using a given context. A null
data argument returns
* null
.
*
* @param ctx
* Context to use - Cannot be null
.
* @param data
* Data to serialize or null
.
* @param adapters
* Adapters to associate with the marshaller or null
.
*
* @return XML data or null
.
*
* @param
* Type of the data.
*/
public static String marshal(final JAXBContext ctx, final T data, final XmlAdapter, ?>[] adapters) {
if (data == null) {
return null;
}
try {
final Marshaller marshaller = ctx.createMarshaller();
if (adapters != null) {
for (final XmlAdapter, ?> adapter : adapters) {
marshaller.setAdapter(adapter);
}
}
final StringWriter writer = new StringWriter();
marshaller.marshal(data, writer);
return writer.toString();
} catch (final JAXBException ex) {
throw new RuntimeException("Error marshalling test data", ex);
}
}
/**
* Unmarshals the given data. A null
XML data argument returns null
.
*
* @param xmlData
* XML data or null
.
* @param classesToBeBound
* List of java classes to be recognized by the {@link JAXBContext} - Cannot be
* null
.
*
* @return Data or null
.
*
* @param
* Type of the expected data.
*/
public static T unmarshal(final String xmlData, final Class>... classesToBeBound) {
return unmarshal(xmlData, null, classesToBeBound);
}
/**
* Unmarshals the given data. A null
XML data argument returns null
.
*
* @param xmlData
* XML data or null
.
* @param adapters
* Adapters to associate with the unmarshaller or null
.
* @param classesToBeBound
* List of java classes to be recognized by the {@link JAXBContext} - Cannot be
* null
.
*
* @return Data or null
.
*
* @param
* Type of the expected data.
*/
public static T unmarshal(final String xmlData, final XmlAdapter, ?>[] adapters,
final Class>... classesToBeBound) {
if (xmlData == null) {
return null;
}
try {
final JAXBContext ctx = JAXBContext.newInstance(classesToBeBound);
return unmarshal(ctx, xmlData, adapters);
} catch (final JAXBException ex) {
throw new RuntimeException("Error unmarshalling test data", ex);
}
}
/**
* Unmarshals the given data using a given context. A null
XML data argument returns
* null
.
*
* @param ctx
* Context to use - Cannot be null
.
* @param xmlData
* XML data or null
.
* @param adapters
* Adapters to associate with the unmarshaller or null
.
*
* @return Data or null
.
*
* @param
* Type of the expected data.
*/
@SuppressWarnings("unchecked")
public static T unmarshal(final JAXBContext ctx, final String xmlData,
final XmlAdapter, ?>[] adapters) {
if (xmlData == null) {
return null;
}
try {
final Unmarshaller unmarshaller = ctx.createUnmarshaller();
if (adapters != null) {
for (final XmlAdapter, ?> adapter : adapters) {
unmarshaller.setAdapter(adapter);
}
}
unmarshaller.setEventHandler(new ValidationEventHandler() {
@Override
public boolean handleEvent(final ValidationEvent event) {
if (event.getSeverity() > 0) {
final Throwable ex = event.getLinkedException();
if (ex == null) {
throw new RuntimeException("Error unmarshalling the data: " + event.getMessage());
}
throw new RuntimeException("Error unmarshalling the data", ex);
}
return true;
}
});
return (T) unmarshaller.unmarshal(new StringReader(xmlData));
} catch (final JAXBException ex) {
throw new RuntimeException("Error unmarshalling test data", ex);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy