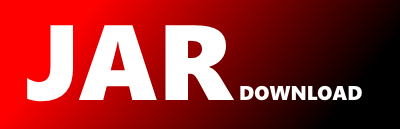
org.fujion.model.IBinder Maven / Gradle / Ivy
/*
* #%L
* fujion
* %%
* Copyright (C) 2018 Fujion Framework
* %%
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*
* #L%
*/
package org.fujion.model;
import java.util.function.Function;
/**
* Interface for implementing model bindings. The following binding types are supported:
*
* - Read - A property change is read from the model and written to the target.
* - Write - A property change is read from the target and written to the model.
* - Dual - Property changes are synchronized between the model and the target.
*
*
* @param The type of model object.
*/
public interface IBinder {
/**
* Supports use of format strings as type converters.
*/
class TemplateConverter implements Function
© 2015 - 2025 Weber Informatics LLC | Privacy Policy