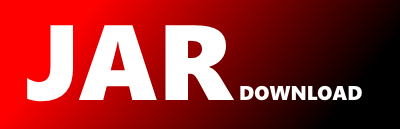
templates.intTable.stg Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of fulib Show documentation
Show all versions of fulib Show documentation
Fulib is a Java-code generating library.
intTable(packageName,primitiveType,objectType) ::=
<<
package ;
import java.util.ArrayList;
import java.util.Collections;
import java.util.LinkedHashMap;
public class Table
{
private ArrayList\ > table = new ArrayList\<>();
public ArrayList\ > getTable()
{
return table;
}
public void setTable(ArrayList\ > table)
{
this.table = table;
}
private String columnName = null;
public String getColumnName()
{
return columnName;
}
public void setColumnName(String columnName)
{
this.columnName = columnName;
}
LinkedHashMap\ columnMap = new LinkedHashMap\<>();
public void setColumnMap(LinkedHashMap\ columnMap)
{
this.columnMap = columnMap;
}
public Table(... start)
{
columnName = "A";
columnMap.put(columnName, 0);
for ( current : start)
{
ArrayList\
© 2015 - 2025 Weber Informatics LLC | Privacy Policy