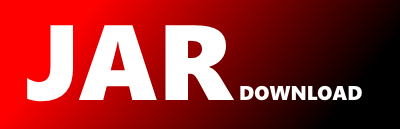
templates.tablesSelectColumns.stg Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of fulib Show documentation
Show all versions of fulib Show documentation
Fulib is a Java-code generating library.
selectColumns(className) ::=
<<
public Table selectColumns(String... columnNames)
{
LinkedHashMap\ oldColumnMap = (LinkedHashMap\) this.columnMap.clone();
this.columnMap.clear();
int i = 0;
for (String name : columnNames)
{
if (oldColumnMap.get(name) == null)
throw new IllegalArgumentException("unknown column name: " + name);
this.columnMap.put(name, i);
i++;
}
ArrayList\ > oldTable = (ArrayList\ >) this.table.clone();
this.table.clear();
LinkedHashSet\ > rowSet = new LinkedHashSet\<>();
for (ArrayList row : oldTable)
{
ArrayList newRow = new ArrayList();
for (String name : columnNames)
{
Object value = row.get(oldColumnMap.get(name));
newRow.add(value);
}
if (rowSet.add(newRow))
this.table.add(newRow);
}
return this;
}
>>
dropColumns(className) ::=
<<
public Table dropColumns(String... columnNames)
{
LinkedHashMap\ oldColumnMap = (LinkedHashMap\) this.columnMap.clone();
this.columnMap.clear();
LinkedHashSet\ dropNames = new LinkedHashSet\<>();
dropNames.addAll(Arrays.asList(columnNames));
int i = 0;
for (String name : oldColumnMap.keySet())
{
if ( ! dropNames.contains(name))
{
this.columnMap.put(name, i);
i++;
}
}
ArrayList\ > oldTable = (ArrayList\ >) this.table.clone();
this.table.clear();
LinkedHashSet\ > rowSet = new LinkedHashSet\<>();
for (ArrayList row : oldTable)
{
ArrayList newRow = new ArrayList();
for (String name : this.columnMap.keySet())
{
Object value = row.get(oldColumnMap.get(name));
newRow.add(value);
}
if (rowSet.add(newRow))
this.table.add(newRow);
}
return this;
}
>>
addColumn(className) ::=
<<
public void addColumn(String columnName, java.util.function.Function\,Object> function)
{
int newColumnNumber = this.table.size() > 0 ? this.table.get(0).size() : 0;
for (ArrayList\
© 2015 - 2025 Weber Informatics LLC | Privacy Policy