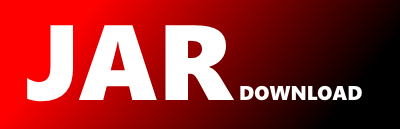
fj.F1Functions Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of functionaljava Show documentation
Show all versions of functionaljava Show documentation
Functional Java is an open source library that supports closures for the Java programming language
package fj;
import fj.control.parallel.Actor;
import fj.control.parallel.Promise;
import fj.control.parallel.Strategy;
import fj.data.*;
import java.util.ArrayList;
import java.util.LinkedList;
import java.util.PriorityQueue;
import java.util.TreeSet;
import java.util.concurrent.*;
import static fj.data.Option.some;
import static fj.data.Stream.iterableStream;
import static fj.data.Zipper.fromStream;
/**
* Created by MarkPerry on 6/04/2014.
*/
public class F1Functions {
/**
* Function composition
*
* @param g A function to compose with this one.
* @return The composed function such that this function is applied last.
*/
static public F o(final F f, final F g) {
return c -> f.f(g.f(c));
}
/**
* First-class function composition
*
* @return A function that composes this function with another.
*/
static public F, F> o(final F f) {
return g -> o(f, g);
}
/**
* Function composition flipped.
*
* @param g A function with which to compose this one.
* @return The composed function such that this function is applied first.
*/
@SuppressWarnings({"unchecked"})
static public F andThen(final F f, final F g) {
return o(g, f);
}
/**
* First-class composition flipped.
*
* @return A function that invokes this function and then a given function on the result.
*/
static public F, F> andThen(final F f) {
return g -> andThen(f, g);
}
/**
* Binds a given function across this function (Reader Monad).
*
* @param g A function that takes the return value of this function as an argument, yielding a new function.
* @return A function that invokes this function on its argument and then the given function on the result.
*/
static public F bind(final F f, final F> g) {
return a -> g.f(f.f(a)).f(a);
}
/**
* First-class function binding.
*
* @return A function that binds another function across this function.
*/
static public F>, F> bind(final F f) {
return g -> bind(f, g);
}
/**
* Function application in an environment (Applicative Functor).
*
* @param g A function with the same argument type as this function, yielding a function that takes the return
* value of this function.
* @return A new function that invokes the given function on its argument, yielding a new function that is then
* applied to the result of applying this function to the argument.
*/
static public F apply(final F f, final F> g) {
return a -> g.f(a).f(f.f(a));
}
/**
* First-class function application in an environment.
*
* @return A function that applies a given function within the environment of this function.
*/
static public F>, F> apply(final F f) {
return g -> apply(f, g);
}
/**
* Applies this function over the arguments of another function.
*
* @param g The function over whose arguments to apply this function.
* @return A new function that invokes this function on its arguments before invoking the given function.
*/
static public F> on(final F f, final F> g) {
return a1 -> a2 -> g.f(f.f(a1)).f(f.f(a2));
}
/**
* Applies this function over the arguments of another function.
*
* @return A function that applies this function over the arguments of another function.
*/
static public F>, F>> on(final F f) {
return g -> on(f, g);
}
/**
* Promotes this function so that it returns its result in a product-1. Kleisli arrow for P1.
*
* @return This function promoted to return its result in a product-1.
*/
static public F> lazy(final F f) {
return a -> P.lazy(() -> f.f(a));
}
/**
* Partial application.
*
* @param a The A
to which to apply this function.
* @return The function partially applied to the given argument to return a lazy value.
*/
static public P1 f(final F f, final A a) {
return P.lazy(() -> f.f(a));
}
/**
* Promotes this function to map over a product-1.
*
* @return This function promoted to map over a product-1.
*/
static public F, P1> mapP1(final F f) {
return p -> p.map(f);
}
/**
* Promotes this function so that it returns its result in an Option. Kleisli arrow for Option.
*
* @return This function promoted to return its result in an Option.
*/
static public F> optionK(final F f) {
return a -> some(f.f(a));
}
/**
* Promotes this function to map over an optional value.
*
* @return This function promoted to map over an optional value.
*/
static public F
© 2015 - 2025 Weber Informatics LLC | Privacy Policy