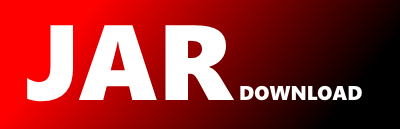
fj.Ord Maven / Gradle / Ivy
package fj;
import fj.data.Array;
import fj.data.Either;
import fj.data.List;
import fj.data.Natural;
import fj.data.NonEmptyList;
import fj.data.Option;
import fj.data.Set;
import fj.data.Stream;
import fj.data.Validation;
import java.math.BigDecimal;
import java.math.BigInteger;
import java.util.Comparator;
import static fj.Function.curry;
/**
* Tests for ordering between two objects.
*
* @version %build.number%
*/
public final class Ord {
private final F> f;
private Ord(final F> f) {
this.f = f;
}
/**
* First-class ordering.
*
* @return A function that returns an ordering for its arguments.
*/
public F> compare() {
return f;
}
/**
* Returns an ordering for the given arguments.
*
* @param a1 An instance to compare for ordering to another.
* @param a2 An instance to compare for ordering to another.
* @return An ordering for the given arguments.
*/
public Ordering compare(final A a1, final A a2) {
return f.f(a1).f(a2);
}
/**
* Returns true
if the given arguments are equal, false
otherwise.
*
* @param a1 An instance to compare for equality to another.
* @param a2 An instance to compare for equality to another.
* @return true
if the given arguments are equal, false
otherwise.
*/
public boolean eq(final A a1, final A a2) {
return compare(a1, a2) == Ordering.EQ;
}
/**
* Returns an Equal
for this order.
*
* @return An Equal
for this order.
*/
public Equal equal() {
return Equal.equal(curry(this::eq));
}
/**
* Maps the given function across this ord as a contra-variant functor.
*
* @param f The function to map.
* @return A new ord.
*/
public Ord contramap(final F f) {
return ord(F1Functions.o(F1Functions.o(F1Functions.andThen(f), this.f), f));
}
/**
* Returns true
if the first given argument is less than the second given argument,
* false
otherwise.
*
* @param a1 An instance to compare for ordering to another.
* @param a2 An instance to compare for ordering to another.
* @return true
if the first given argument is less than the second given argument,
* false
otherwise.
*/
public boolean isLessThan(final A a1, final A a2) {
return compare(a1, a2) == Ordering.LT;
}
/**
* Returns true
if the first given argument is greater than the second given
* argument, false
otherwise.
*
* @param a1 An instance to compare for ordering to another.
* @param a2 An instance to compare for ordering to another.
* @return true
if the first given argument is greater than the second given
* argument, false
otherwise.
*/
public boolean isGreaterThan(final A a1, final A a2) {
return compare(a1, a2) == Ordering.GT;
}
/**
* Returns a function that returns true if its argument is less than the argument to this method.
*
* @param a A value to compare against.
* @return A function that returns true if its argument is less than the argument to this method.
*/
public F isLessThan(final A a) {
return a2 -> compare(a2, a) == Ordering.LT;
}
/**
* Returns a function that returns true if its argument is greater than than the argument to this method.
*
* @param a A value to compare against.
* @return A function that returns true if its argument is greater than the argument to this method.
*/
public F isGreaterThan(final A a) {
return a2 -> compare(a2, a) == Ordering.GT;
}
/**
* Returns the greater of its two arguments.
*
* @param a1 A value to compare with another.
* @param a2 A value to compare with another.
* @return The greater of the two values.
*/
public A max(final A a1, final A a2) {
return isGreaterThan(a1, a2) ? a1 : a2;
}
/**
* Returns the lesser of its two arguments.
*
* @param a1 A value to compare with another.
* @param a2 A value to compare with another.
* @return The lesser of the two values.
*/
public A min(final A a1, final A a2) {
return isLessThan(a1, a2) ? a1 : a2;
}
/**
* A function that returns the greater of its two arguments.
*/
public final F> max = curry((a, a1) -> max(a, a1));
/**
* A function that returns the lesser of its two arguments.
*/
public final F> min = curry((a, a1) -> min(a, a1));
public final Ord reverse() { return ord(Function.flip(f)); }
/**
* Returns an order instance that uses the given equality test and ordering function.
*
* @param f The order function.
* @return An order instance.
*/
public static Ord ord(final F> f) {
return new Ord(f);
}
/**
* An order instance for the boolean
type.
*/
public static final Ord booleanOrd = ord(
a1 -> a2 -> {
final int x = a1.compareTo(a2);
return x < 0 ? Ordering.LT : x == 0 ? Ordering.EQ : Ordering.GT;
});
/**
* An order instance for the byte
type.
*/
public static final Ord byteOrd = ord(
a1 -> a2 -> {
final int x = a1.compareTo(a2);
return x < 0 ? Ordering.LT : x == 0 ? Ordering.EQ : Ordering.GT;
});
/**
* An order instance for the char
type.
*/
public static final Ord charOrd = ord(
a1 -> a2 -> {
final int x = a1.compareTo(a2);
return x < 0 ? Ordering.LT : x == 0 ? Ordering.EQ : Ordering.GT;
});
/**
* An order instance for the double
type.
*/
public static final Ord doubleOrd = ord(
a1 -> a2 -> {
final int x = a1.compareTo(a2);
return x < 0 ? Ordering.LT : x == 0 ? Ordering.EQ : Ordering.GT;
});
/**
* An order instance for the float
type.
*/
public static final Ord floatOrd = ord(
a1 -> a2 -> {
final int x = a1.compareTo(a2);
return x < 0 ? Ordering.LT : x == 0 ? Ordering.EQ : Ordering.GT;
});
/**
* An order instance for the int
type.
*/
public static final Ord intOrd = ord(
a1 -> a2 -> {
final int x = a1.compareTo(a2);
return x < 0 ? Ordering.LT : x == 0 ? Ordering.EQ : Ordering.GT;
});
/**
* An order instance for the BigInteger
type.
*/
public static final Ord bigintOrd = ord(
a1 -> a2 -> {
final int x = a1.compareTo(a2);
return x < 0 ? Ordering.LT : x == 0 ? Ordering.EQ : Ordering.GT;
});
/**
* An order instance for the BigDecimal
type.
*/
public static final Ord bigdecimalOrd = ord(
a1 -> a2 -> {
final int x = a1.compareTo(a2);
return x < 0 ? Ordering.LT : x == 0 ? Ordering.EQ : Ordering.GT;
});
/**
* An order instance for the long
type.
*/
public static final Ord longOrd = ord(
a1 -> a2 -> {
final int x = a1.compareTo(a2);
return x < 0 ? Ordering.LT : x == 0 ? Ordering.EQ : Ordering.GT;
});
/**
* An order instance for the short
type.
*/
public static final Ord shortOrd = ord(
a1 -> a2 -> {
final int x = a1.compareTo(a2);
return x < 0 ? Ordering.LT : x == 0 ? Ordering.EQ : Ordering.GT;
});
/**
* An order instance for the {@link Ordering} type.
*/
public static final Ord orderingOrd = Ord.ord(curry((o1, o2) -> o1 == o2 ?
Ordering.EQ :
o1 == Ordering.LT ?
Ordering.LT :
o2 == Ordering.LT ?
Ordering.GT :
o1 == Ordering.EQ ?
Ordering.LT :
Ordering.GT));
/**
* An order instance for the {@link String} type.
*/
public static final Ord stringOrd = ord(
a1 -> a2 -> {
final int x = a1.compareTo(a2);
return x < 0 ? Ordering.LT : x == 0 ? Ordering.EQ : Ordering.GT;
});
/**
* An order instance for the {@link StringBuffer} type.
*/
public static final Ord stringBufferOrd =
ord(a1 -> a2 -> stringOrd.compare(a1.toString(), a2.toString()));
/**
* An order instance for the {@link StringBuffer} type.
*/
public static final Ord stringBuilderOrd =
ord(a1 -> a2 -> stringOrd.compare(a1.toString(), a2.toString()));
/**
* An order instance for the {@link Option} type.
*
* @param oa Order across the element of the option.
* @return An order instance for the {@link Option} type.
*/
public static Ord
© 2015 - 2025 Weber Informatics LLC | Privacy Policy