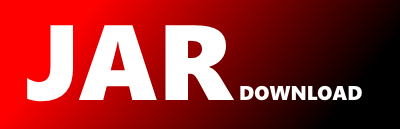
fj.P5 Maven / Gradle / Ivy
package fj;
/**
* A product-5.
*
* @version %build.number%
*/
public abstract class P5 {
/**
* Access the first element of the product.
*
* @return The first element of the product.
*/
public abstract A _1();
/**
* Access the second element of the product.
*
* @return The second element of the product.
*/
public abstract B _2();
/**
* Access the third element of the product.
*
* @return The third element of the product.
*/
public abstract C _3();
/**
* Access the fourth element of the product.
*
* @return The fourth element of the product.
*/
public abstract D _4();
/**
* Access the fifth element of the product.
*
* @return The fifth element of the product.
*/
public abstract E _5();
/**
* Map the first element of the product.
*
* @param f The function to map with.
* @return A product with the given function applied.
*/
public final P5 map1(final F f) {
return new P5() {
public X _1() {
return f.f(P5.this._1());
}
public B _2() {
return P5.this._2();
}
public C _3() {
return P5.this._3();
}
public D _4() {
return P5.this._4();
}
public E _5() {
return P5.this._5();
}
};
}
/**
* Map the second element of the product.
*
* @param f The function to map with.
* @return A product with the given function applied.
*/
public final P5 map2(final F f) {
return new P5() {
public A _1() {
return P5.this._1();
}
public X _2() {
return f.f(P5.this._2());
}
public C _3() {
return P5.this._3();
}
public D _4() {
return P5.this._4();
}
public E _5() {
return P5.this._5();
}
};
}
/**
* Map the third element of the product.
*
* @param f The function to map with.
* @return A product with the given function applied.
*/
public final P5 map3(final F f) {
return new P5() {
public A _1() {
return P5.this._1();
}
public B _2() {
return P5.this._2();
}
public X _3() {
return f.f(P5.this._3());
}
public D _4() {
return P5.this._4();
}
public E _5() {
return P5.this._5();
}
};
}
/**
* Map the fourth element of the product.
*
* @param f The function to map with.
* @return A product with the given function applied.
*/
public final P5 map4(final F f) {
return new P5() {
public A _1() {
return P5.this._1();
}
public B _2() {
return P5.this._2();
}
public C _3() {
return P5.this._3();
}
public X _4() {
return f.f(P5.this._4());
}
public E _5() {
return P5.this._5();
}
};
}
/**
* Map the fifth element of the product.
*
* @param f The function to map with.
* @return A product with the given function applied.
*/
public final P5 map5(final F f) {
return new P5() {
public A _1() {
return P5.this._1();
}
public B _2() {
return P5.this._2();
}
public C _3() {
return P5.this._3();
}
public D _4() {
return P5.this._4();
}
public X _5() {
return f.f(P5.this._5());
}
};
}
/**
* Returns the 1-product projection over the first element.
*
* @return the 1-product projection over the first element.
*/
public final P1 _1_() {
return F1Functions.lazy(P5.__1()).f(this);
}
/**
* Returns the 1-product projection over the second element.
*
* @return the 1-product projection over the second element.
*/
public final P1 _2_() {
return F1Functions.lazy(P5.__2()).f(this);
}
/**
* Returns the 1-product projection over the third element.
*
* @return the 1-product projection over the third element.
*/
public final P1 _3_() {
return F1Functions.lazy(P5.__3()).f(this);
}
/**
* Returns the 1-product projection over the fourth element.
*
* @return the 1-product projection over the fourth element.
*/
public final P1 _4_() {
return F1Functions.lazy(P5.__4()).f(this);
}
/**
* Returns the 1-product projection over the fifth element.
*
* @return the 1-product projection over the fifth element.
*/
public final P1 _5_() {
return F1Functions.lazy(P5.__5()).f(this);
}
/**
* Provides a memoising P5 that remembers its values.
*
* @return A P5 that calls this P5 once for any given element and remembers the value for subsequent calls.
*/
public final P5 memo() {
P5 self = this;
return new P5() {
private final P1 a = P1.memo(u -> self._1());
private final P1 b = P1.memo(u -> self._2());
private final P1 c = P1.memo(u -> self._3());
private final P1 d = P1.memo(u -> self._4());
private final P1 e = P1.memo(u -> self._5());
public A _1() {
return a._1();
}
public B _2() {
return b._1();
}
public C _3() {
return c._1();
}
public D _4() {
return d._1();
}
public E _5() {
return e._1();
}
};
}
/**
* Returns a function that returns the first element of a product.
*
* @return A function that returns the first element of a product.
*/
public static F, A> __1() {
return p -> p._1();
}
/**
* Returns a function that returns the second element of a product.
*
* @return A function that returns the second element of a product.
*/
public static F, B> __2() {
return p -> p._2();
}
/**
* Returns a function that returns the third element of a product.
*
* @return A function that returns the third element of a product.
*/
public static F, C> __3() {
return p -> p._3();
}
/**
* Returns a function that returns the fourth element of a product.
*
* @return A function that returns the fourth element of a product.
*/
public static F, D> __4() {
return p -> p._4();
}
/**
* Returns a function that returns the fifth element of a product.
*
* @return A function that returns the fifth element of a product.
*/
public static F, E> __5() {
return p -> p._5();
}
@Override
public String toString() {
return Show.p5Show(Show.anyShow(), Show.anyShow(), Show.anyShow(), Show.anyShow(), Show.anyShow()).showS(this);
}
@Override
public boolean equals(Object other) {
return Equal.equals0(P5.class, this, other,
() -> Equal.p5Equal(Equal.anyEqual(), Equal.anyEqual(), Equal.anyEqual(), Equal.anyEqual(), Equal.anyEqual()));
}
@Override
public int hashCode() {
return Hash.p5Hash(Hash.anyHash(), Hash.anyHash(), Hash.anyHash(), Hash.anyHash(), Hash.anyHash()).hash(this);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy