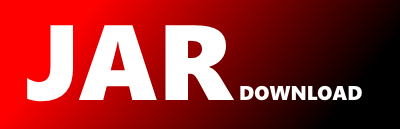
fj.control.db.DB Maven / Gradle / Ivy
package fj.control.db;
import fj.F;
import fj.Function;
import java.sql.Connection;
import java.sql.SQLException;
import java.util.concurrent.Callable;
/**
* The DB monad represents a database action, or a value within the context of a database connection.
*/
public abstract class DB {
/**
* Executes the database action, given a database connection.
*
* @param c The connection against which to execute the action.
* @return The result of the action.
* @throws SQLException if a database error occurred.
*/
public abstract A run(final Connection c) throws SQLException;
/**
* Constructs a database action as a function from a database connection to a value.
*
* @param f A function from a database connection to a value.
* @return A database action representing the given function.
*/
public static DB db(final F f) {
return new DB() {
public A run(final Connection c) {
return f.f(c);
}
};
}
/**
* Returns the callable-valued function projection of this database action.
*
* @return The callable-valued function which is isomorphic to this database action.
*/
public final F> asFunction() {
return c -> () -> run(c);
}
/**
* Map a function over the result of this action.
*
* @param f The function to map over the result.
* @return A new database action that applies the given function to the result of this action.
*/
public final DB map(final F f) {
return new DB() {
public B run(final Connection c) throws SQLException {
return f.f(DB.this.run(c));
}
};
}
/**
* Promotes any given function so that it transforms between values in the database.
*
* @param f The function to promote.
* @return A function equivalent to the given one, which operates on values in the database.
*/
public static F, DB> liftM(final F f) {
return a -> a.map(f);
}
/**
* Constructs a database action that returns the given value completely intact.
*
* @param a A value to be wrapped in a database action.
* @return A new database action that returns the given value.
*/
public static DB unit(final A a) {
return new DB() {
public A run(final Connection c) {
return a;
}
};
}
/**
* Binds the given action across the result of this database action.
*
* @param f The function to bind across the result of this database action.
* @return A new database action equivalent to applying the given function to the result of this action.
*/
public final DB bind(final F> f) {
return new DB() {
public B run(final Connection c) throws SQLException {
return f.f(DB.this.run(c)).run(c);
}
};
}
/**
* Removes one layer of monadic structure.
*
* @param a A database action that results in another.
* @return A new database action equivalent to the result of the given action.
*/
public static DB join(final DB> a) {
return a.bind(Function.>identity());
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy