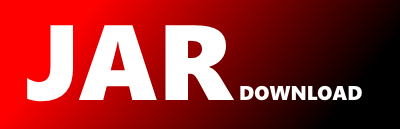
fj.data.Enumerator Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of functionaljava Show documentation
Show all versions of functionaljava Show documentation
Functional Java is an open source library that supports closures for the Java programming language
Please wait ...
© 2015 - 2025 Weber Informatics LLC | Privacy Policy