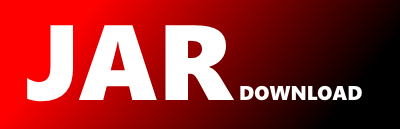
fj.function.BigIntegers Maven / Gradle / Ivy
package fj.function;
import fj.F;
import fj.F2;
import fj.Monoid;
import fj.data.List;
import static fj.Function.curry;
import java.math.BigInteger;
/**
* Curried functions over Integers.
*
* @version %build.number%
*/
public final class BigIntegers {
private BigIntegers() {
throw new UnsupportedOperationException();
}
/**
* Curried Integer addition.
*/
public static final F> add =
curry((a1, a2) -> a1.add(a2));
/**
* Curried Integer multiplication.
*/
public static final F> multiply =
curry((a1, a2) -> a1.multiply(a2));
/**
* Curried Integer subtraction.
*/
public static final F> subtract =
curry((a1, a2) -> a1.subtract(a2));
/**
* Negation.
*/
public static final F negate = i -> i.negate();
/**
* Absolute value.
*/
public static final F abs = i -> i.abs();
/**
* Remainder.
*/
public static final F> remainder =
curry((a1, a2) -> a1.remainder(a2));
/**
* Power.
*/
public static final F> power = curry((a1, a2) -> a1.pow(a2));
/**
* Sums a list of big integers.
*
* @param ints A list of big integers to sum.
* @return The sum of the big integers in the list.
*/
public static BigInteger sum(final List ints) {
return Monoid.bigintAdditionMonoid.sumLeft(ints);
}
/**
* Returns the product of a list of big integers.
*
* @param ints A list of big integers to multiply together.
* @return The product of the big integers in the list.
*/
public static BigInteger product(final List ints) {
return Monoid.bigintMultiplicationMonoid.sumLeft(ints);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy