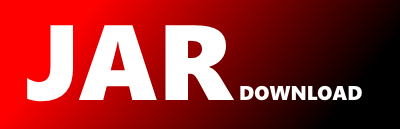
fj.parser.Result Maven / Gradle / Ivy
package fj.parser;
import fj.F;
import fj.F2;
import static fj.Function.curry;
import java.util.Iterator;
import java.util.NoSuchElementException;
/**
* A parse result made up of a value (A) and the remainder of the parse input (I).
*
* @version %build.number%
*/
public final class Result implements Iterable {
private final I i;
private final A a;
private Result(final I i, final A a) {
this.i = i;
this.a = a;
}
/**
* The remainder of the parse input.
*
* @return The remainder of the parse input.
*/
public I rest() {
return i;
}
/**
* The parsed value.
*
* @return The parsed value.
*/
public A value() {
return a;
}
/**
* Maps the given function across the remainder of the parse input.
*
* @param f The function to map with.
* @return A result with a different parse input.
*/
public Result mapRest(final F f) {
return result(f.f(i), a);
}
/**
* First-class function mapping across the remainder of the parse input.
*
* @return A first-class function mapping across the remainder of the parse input.
*/
public F, Result> mapRest() {
return f -> mapRest(f);
}
/**
* Maps the given function across the parse value.
*
* @param f The function to map with.
* @return A result with a different parse value.
*/
public Result mapValue(final F f) {
return result(i, f.f(a));
}
/**
* First-class function mapping across the parse value.
*
* @return A first-class function mapping across the parse value.
*/
public F, Result> mapValue() {
return f -> mapValue(f);
}
/**
* A bifunctor map across both the remainder of the parse input and the parse value.
*
* @param f The function to map the remainder of the parse input with.
* @param g The function to map the parse value with.
* @return A result with a different parse input and parse value.
*/
public Result bimap(final F f, final F g) {
return mapRest(f).mapValue(g);
}
/**
* First-class bifunctor map.
*
* @return A first-class bifunctor map.
*/
public F, F, Result>> bimap() {
return curry((f, g) -> bimap(f, g));
}
/**
* Returns an iterator over the parse value. This method exists to permit the use in a for
-each loop.
*
* @return An iterator over the parse value.
*/
public Iterator iterator() {
return new Iterator() {
private boolean r;
public boolean hasNext() {
return !r;
}
public A next() {
if (r)
throw new NoSuchElementException();
else {
r = true;
return a;
}
}
public void remove() {
throw new UnsupportedOperationException();
}
};
}
/**
* Construct a result with the given remainder of the parse input and parse value.
*
* @param i The remainder of the parse input.
* @param a The parse value.
* @return A result with the given remainder of the parse input and parse value.
*/
public static Result result(final I i, final A a) {
return new Result(i, a);
}
/**
* First-class construction of a result.
*
* @return A first-class function for construction of a result.
*/
public static F>> result() {
return curry((i, a) -> result(i, a));
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy