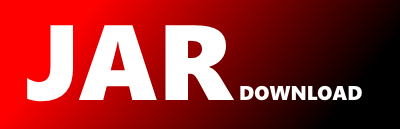
fj.F Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of functionaljava Show documentation
Show all versions of functionaljava Show documentation
Functional Java is an open source library that supports closures for the Java programming language
The newest version!
package fj;
import fj.control.parallel.Actor;
import fj.control.parallel.Promise;
import fj.control.parallel.Strategy;
import fj.data.*;
import java.util.ArrayList;
import java.util.LinkedList;
import java.util.TreeSet;
import java.util.concurrent.*;
import java.util.function.Function;
import static fj.data.Option.some;
import static fj.data.Stream.iterableStream;
import static fj.data.Zipper.fromStream;
/**
* A transformation or function from A
to B
.
*/
@FunctionalInterface
public interface F extends Function {
/**
* Transform A
to B
.
*
* @param a The A
to transform.
* @return The result of the transformation.
*/
B f(A a);
default B apply(A a) {
return f(a);
}
/**
* Function composition
*
* @param g A function to compose with this one.
* @return The composed function such that this function is applied last.
*/
default F o(final F g) {
return c -> f(g.f(c));
}
/**
* First-class function composition
*
* @return A function that composes this function with another.
*/
default F, F> o() {
return g -> o(g);
}
/**
* Function composition flipped.
*
* @param g A function with which to compose this one.
* @return The composed function such that this function is applied first.
*/
@SuppressWarnings("unchecked")
default F andThen(final F g) {
return g.o(this);
}
/**
* First-class composition flipped.
*
* @return A function that invokes this function and then a given function on the result.
*/
default F, F> andThen() {
return g -> andThen(g);
}
/**
* Binds a given function across this function (Reader Monad).
*
* @param g A function that takes the return value of this function as an argument, yielding a new function.
* @return A function that invokes this function on its argument and then the given function on the result.
*/
default F bind(final F> g) {
return a -> g.f(f(a)).f(a);
}
/**
* First-class function binding.
*
* @return A function that binds another function across this function.
*/
default F>, F> bind() {
return g -> bind(g);
}
/**
* Function application in an environment (Applicative Functor).
*
* @param g A function with the same argument type as this function, yielding a function that takes the return
* value of this function.
* @return A new function that invokes the given function on its argument, yielding a new function that is then
* applied to the result of applying this function to the argument.
*/
default F apply(final F> g) {
return a -> g.f(a).f(f(a));
}
/**
* First-class function application in an environment.
*
* @return A function that applies a given function within the environment of this function.
*/
default F>, F> apply() {
return g -> apply(g);
}
/**
* Applies this function over the arguments of another function.
*
* @param g The function over whose arguments to apply this function.
* @return A new function that invokes this function on its arguments before invoking the given function.
*/
default F> on(final F> g) {
return a1 -> a2 -> g.f(f(a1)).f(f(a2));
}
/**
* Applies this function over the arguments of another function.
*
* @return A function that applies this function over the arguments of another function.
*/
default F>, F>> on() {
return g -> on(g);
}
/**
* Promotes this function so that it returns its result in a product-1. Kleisli arrow for P1.
*
* @return This function promoted to return its result in a product-1.
*/
default F> lazy() {
return a -> P.lazy(() -> f(a));
}
/**
* Partial application.
*
* @param a The A
to which to apply this function.
* @return The function partially applied to the given argument to return a lazy value.
*/
default P1 partial(final A a) {
return P.lazy(() -> f(a));
}
/**
* Promotes this function to map over a product-1.
*
* @return This function promoted to map over a product-1.
*/
default F, P1> mapP1() {
return p -> p.map(this);
}
/**
* Promotes this function so that it returns its result in an Option. Kleisli arrow for Option.
*
* @return This function promoted to return its result in an Option.
*/
default F> optionK() {
return a -> some(f(a));
}
/**
* Promotes this function to map over an optional value.
*
* @return This function promoted to map over an optional value.
*/
default F
© 2015 - 2025 Weber Informatics LLC | Privacy Policy