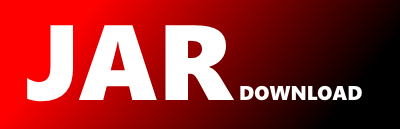
fj.F2Functions Maven / Gradle / Ivy
package fj;
import fj.control.parallel.Promise;
import fj.data.*;
import static fj.P.p;
import static fj.data.IterableW.wrap;
import static fj.data.Set.iterableSet;
import static fj.data.Tree.node;
import static fj.data.TreeZipper.treeZipper;
import static fj.data.Zipper.zipper;
/**
* Created by MarkPerry on 6/04/2014.
*/
public final class F2Functions {
private F2Functions() {
}
/**
* Partial application.
*
* @param a The A
to which to apply this function.
* @return The function partially applied to the given argument.
*/
public static F f(final F2 f, final A a) {
return b -> f.f(a, b);
}
/**
* Curries this wrapped function to a wrapped function of arity-1 that returns another wrapped function.
*
* @return a wrapped function of arity-1 that returns another wrapped function.
*/
public static F> curry(final F2 f) {
return a -> b -> f.f(a, b);
}
/**
* Flips the arguments of this function.
*
* @return A new function with the arguments of this function flipped.
*/
public static F2 flip(final F2 f) {
return (b, a) -> f.f(a, b);
}
/**
* Uncurries this function to a function on tuples.
*
* @return A new function that calls this function with the elements of a given tuple.
*/
public static F, C> tuple(final F2 f) {
return p -> f.f(p._1(), p._2());
}
/**
* Promotes this function to a function on Arrays.
*
* @return This function promoted to transform Arrays.
*/
public static F2, Array, Array> arrayM(final F2 f) {
return (a, b) -> a.bind(b, curry(f));
}
/**
* Promotes this function to a function on Promises.
*
* @return This function promoted to transform Promises.
*/
public static F2, Promise, Promise> promiseM(final F2 f) {
return (a, b) -> a.bind(b, curry(f));
}
/**
* Promotes this function to a function on Iterables.
*
* @return This function promoted to transform Iterables.
*/
public static F2, Iterable, IterableW> iterableM(final F2 f) {
return (a, b) -> IterableW.liftM2(curry(f)).f(a).f(b);
}
/**
* Promotes this function to a function on Lists.
*
* @return This function promoted to transform Lists.
*/
public static F2, List, List> listM(final F2 f) {
return (a, b) -> List.liftM2(curry(f)).f(a).f(b);
}
/**
* Promotes this function to a function on non-empty lists.
*
* @return This function promoted to transform non-empty lists.
*/
public static F2, NonEmptyList, NonEmptyList> nelM(final F2 f) {
return (as, bs) -> NonEmptyList.fromList(as.toList().bind(bs.toList(), f)).some();
}
/**
* Promotes this function to a function on Options.
*
* @return This function promoted to transform Options.
*/
public static F2
© 2015 - 2025 Weber Informatics LLC | Privacy Policy