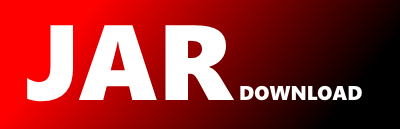
fj.Show Maven / Gradle / Ivy
package fj;
import fj.data.*;
import fj.data.hamt.BitSet;
import fj.data.hamt.HashArrayMappedTrie;
import fj.data.fingertrees.FingerTree;
import fj.data.hlist.HList;
import fj.data.vector.V2;
import fj.data.vector.V3;
import fj.data.vector.V4;
import fj.data.vector.V5;
import fj.data.vector.V6;
import fj.data.vector.V7;
import fj.data.vector.V8;
import fj.parser.Result;
import java.math.BigDecimal;
import java.math.BigInteger;
import static fj.Function.compose;
import static fj.P.p;
import static fj.Unit.unit;
import static fj.data.Stream.cons;
import static fj.data.Stream.fromString;
import static fj.data.Stream.join;
import static fj.data.Stream.single;
/**
* Renders an object for display.
*
* @version %build.number%
*/
public final class Show {
private final F> f;
private Show(final F> f) {
this.f = f;
}
/**
* Maps the given function across this show as a contra-variant functor.
*
* @param f The function to map.
* @return A new show.
*/
public Show contramap(final F f) {
return show(compose(this.f, f));
}
/**
* Returns the display rendering of the given argument.
*
* @param a The argument to display.
* @return The display rendering of the given argument.
*/
public Stream show(final A a) {
return f.f(a);
}
/**
* Returns the display rendering of the given argument.
*
* @param a The argument to display.
* @return The display rendering of the given argument.
*/
public List showl(final A a) {
return show(a).toList();
}
/**
* Returns the display rendering of the given argument as a String
.
*
* @param a The argument to display.
* @return The display rendering of the given argument as a String
.
*/
public String showS(final A a) {
return Stream.asString(show(a));
}
/**
* Returns the transformation equivalent to this show.
*
* @return the transformation equivalent to this show.
*/
public F showS_() {
return this::showS;
}
/**
* Returns the transformation equivalent to this show.
*
* @return the transformation equivalent to this show.
*/
public F> show_() {
return f;
}
/**
* Prints the given argument to the standard output stream with a new line.
*
* @param a The argument to print.
* @return The unit value.
*/
public Unit println(final A a) {
print(a);
System.out.println();
return unit();
}
/**
* Prints the given argument to the standard output stream.
*
* @param a The argument to print.
* @return The unit value.
*/
public Unit print(final A a) {
final char[] buffer = new char[8192];
int c = 0;
for (Stream cs = show(a); cs.isNotEmpty(); cs = cs.tail()._1()) {
buffer[c] = cs.head();
c++;
if (c == 8192) {
System.out.print(buffer);
c = 0;
}
}
System.out.print(Array.copyOfRange(buffer, 0, c));
return unit();
}
/**
* Prints the given argument to the standard error stream with a new line.
*
* @param a The argument to print.
*/
public void printlnE(final A a) {
System.err.println(showS(a));
}
/**
* Returns a show instance using the given function.
*
* @param f The function to use for the returned show instance.
* @return A show instance.
*/
public static Show show(final F> f) {
return new Show<>(f);
}
/**
* Returns a show instance using the given function.
*
* @param f The function to use for the returned show instance.
* @return A show instance.
*/
public static Show showS(final F f) {
return show(a -> fromString(f.f(a)));
}
/**
* Returns a show instance that uses {@link Object#toString()} to perform the display rendering.
*
* @return A show instance that uses {@link Object#toString()} to perform the display rendering.
*/
public static Show anyShow() {
return show(a -> fromString((a == null) ? "null" : a.toString()));
}
/**
* A show instance for the boolean
type.
*/
public static final Show booleanShow = anyShow();
/**
* A show instance for the byte
type.
*/
public static final Show byteShow = anyShow();
/**
* A show instance for the char
type.
*/
public static final Show charShow = anyShow();
/**
* A show instance for the double
type.
*/
public static final Show doubleShow = anyShow();
/**
* A show instance for the float
type.
*/
public static final Show floatShow = anyShow();
/**
* A show instance for the int
type.
*/
public static final Show intShow = anyShow();
/**
* A show instance for the BigInteger
type.
*/
public static final Show bigintShow = anyShow();
/**
* A show instance for the BigDecimal
type.
*/
public static final Show bigdecimalShow = anyShow();
/**
* A show instance for the long
type.
*/
public static final Show longShow = anyShow();
/**
* A show instance for the short
type.
*/
public static final Show shortShow = anyShow();
/**
* A show instance for the {@link String} type.
*/
public static final Show stringShow = anyShow();
/**
* A show instance for the {@link StringBuffer} type.
*/
public static final Show stringBufferShow = anyShow();
/**
* A show instance for the {@link StringBuilder} type.
*/
public static final Show stringBuilderShow = anyShow();
/**
* A show instance for the {@link Option} type.
*
* @param sa Show for the element of the option.
* @return A show instance for the {@link Option} type.
*/
public static Show
© 2015 - 2025 Weber Informatics LLC | Privacy Policy