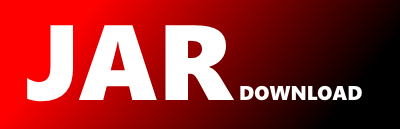
org.fxmisc.flowless.Cell Maven / Gradle / Ivy
Show all versions of Flowless Show documentation
package org.fxmisc.flowless;
import java.util.function.Consumer;
import java.util.function.IntConsumer;
import javafx.scene.Node;
/**
* Provides efficient memory usage by wrapping a {@link Node} within this object and reusing it when
* {@link #isReusable()} is true.
*/
@FunctionalInterface
public interface Cell {
static Cell wrapNode(N node) {
return new Cell() {
@Override
public N getNode() { return node; }
@Override
public String toString() { return node.toString(); }
};
}
N getNode();
/**
* Indicates whether this cell can be reused to display different items.
*
* Default implementation returns {@code false}.
*/
default boolean isReusable() {
return false;
}
/**
* If this cell is reusable (as indicated by {@link #isReusable()}),
* this method is called to display a different item. {@link #reset()}
* will have been called before a call to this method.
*
*
The default implementation throws
* {@link UnsupportedOperationException}.
*
* @param item the new item to display
*/
default void updateItem(T item) {
throw new UnsupportedOperationException();
}
/**
* Called to update index of a visible cell.
*
*
Default implementation does nothing.
*/
default void updateIndex(int index) {
// do nothing by default
}
/**
* Called when this cell is no longer used to display its item.
* If this cell is reusable, it may later be asked to display a different
* item by a call to {@link #updateItem(Object)}.
*
*
Default implementation does nothing.
*/
default void reset() {
// do nothing by default
}
/**
* Called when this cell is no longer going to be used at all.
* {@link #reset()} will have been called before this method is invoked.
*
*
Default implementation does nothing.
*/
default void dispose() {
// do nothing by default
}
default Cell beforeDispose(Runnable action) {
return CellWrapper.beforeDispose(this, action);
}
default Cell afterDispose(Runnable action) {
return CellWrapper.afterDispose(this, action);
}
default Cell beforeReset(Runnable action) {
return CellWrapper.beforeReset(this, action);
}
default Cell afterReset(Runnable action) {
return CellWrapper.afterReset(this, action);
}
default Cell beforeUpdateItem(Consumer super T> action) {
return CellWrapper.beforeUpdateItem(this, action);
}
default Cell afterUpdateItem(Consumer super T> action) {
return CellWrapper.afterUpdateItem(this, action);
}
default Cell beforeUpdateIndex(IntConsumer action) {
return CellWrapper.beforeUpdateIndex(this, action);
}
default Cell afterUpdateIndex(IntConsumer action) {
return CellWrapper.afterUpdateIndex(this, action);
}
}