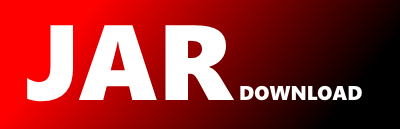
org.fxmisc.wellbehaved.event.EventPattern Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of WellBehavedFX Show documentation
Show all versions of WellBehavedFX Show documentation
Composable event handlers and skin skeletons for JavaFX controls.
The newest version!
package org.fxmisc.wellbehaved.event;
import static javafx.scene.input.KeyEvent.*;
import java.util.Optional;
import java.util.function.Predicate;
import javafx.event.Event;
import javafx.event.EventType;
import javafx.scene.input.KeyCharacterCombination;
import javafx.scene.input.KeyCode;
import javafx.scene.input.KeyCodeCombination;
import javafx.scene.input.KeyCombination;
import javafx.scene.input.KeyEvent;
@FunctionalInterface
public interface EventPattern {
Optional match(T event);
default EventPattern andThen(EventPattern super U, V> next) {
return t -> match(t).flatMap(next::match);
}
default EventPattern and(Predicate super U> condition) {
return t -> match(t).map(u -> condition.test(u) ? u : null);
}
static EventPattern eventTypePattern(EventType extends T> eventType) {
return event -> {
EventType extends Event> actualType = event.getEventType();
do {
if(actualType.equals(eventType)) {
@SuppressWarnings("unchecked")
T res = (T) event;
return Optional.of(res);
}
actualType = actualType.getSuperType();
} while(actualType != null);
return Optional.empty();
};
}
static EventPattern keyPressed() {
return eventTypePattern(KeyEvent.KEY_PRESSED);
}
static EventPattern keyPressed(KeyCombination combination) {
return keyPressed().and(combination::match);
}
static EventPattern keyPressed(KeyCode code, KeyCombination.Modifier... modifiers) {
return keyPressed(new KeyCodeCombination(code, modifiers));
}
static EventPattern keyPressed(String character, KeyCombination.Modifier... modifiers) {
return keyPressed(new KeyCharacterCombination(character, modifiers));
}
static EventPattern keyReleased() {
return eventTypePattern(KEY_RELEASED);
}
static EventPattern keyReleased(KeyCombination combination) {
return keyReleased().and(combination::match);
}
static EventPattern keyReleased(KeyCode code, KeyCombination.Modifier... modifiers) {
return keyReleased(new KeyCodeCombination(code, modifiers));
}
static EventPattern keyReleased(String character, KeyCombination.Modifier... modifiers) {
return keyReleased(new KeyCharacterCombination(character, modifiers));
}
static EventPattern keyTyped() {
return eventTypePattern(KEY_TYPED);
}
static EventPattern keyTyped(String character, KeyCombination.Modifier... modifiers) {
KeyTypedCombination combination = new KeyTypedCombination(character, modifiers);
return keyTyped().and(combination::match);
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy