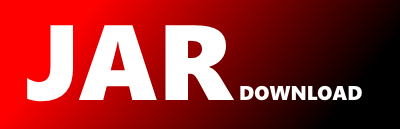
org.gannacademy.cdf.turtlelogo.Terrarium Maven / Gradle / Ivy
Show all versions of turtle-logo Show documentation
package org.gannacademy.cdf.turtlelogo;
import javax.swing.*;
import java.awt.*;
import java.util.ArrayList;
import java.util.List;
/**
* A {@link Turtle} lives (and draws) inside a Terrarium
.
*
* 
*
* A terrarium can be resized using the {@link #setSize(int, int)} method and repositioned on the screen using the
* {@link #setPosition(int, int)}. The terrarium can also have a custom background color (set via the
* {@link #setBackground(Color)} method — it defaults to white.
*
* 
*
* Initially, there is only a single terrarium, into which all new turtles are added. However, it is possible to
* instantiate additional terraria, and to direct Turtles to them using the {@link Turtle#setTerrarium(Terrarium)}
* method. From a technical standpoint, the initial terrarium is a quasi-singleton, and will continue to be treated as
* a singleton by any new turtles as they are instantiated. The singleton terrarium instance can be accessed statically
* via the {@link #getInstance()} method. When additional terraria have been instantiated, they may also be accessed
* statically via their index (in instantiation order) using the {@link #getInstance(int)} method.
*
* @author Seth Battis
*/
public class Terrarium extends JPanel {
/**
* The parts of the terrarium that are "under the surface" are not meant to be used by students. This mechanism
* (inspired by this awesome Stack Overflow answer) recreates a
* version of the C++ friend
concept: a public method that is only available to some other
* objects, rather than all other objects.
*
* @author Seth Battis
*/
public static final class UnderTheSurface {
private UnderTheSurface() {
}
}
protected static final UnderTheSurface UNDER_THE_SURFACE = new UnderTheSurface();
/**
* 600 pixels
*/
public static final int DEFAULT_WIDTH = 600;
/**
* 400 pixels
*/
public static final int DEFAULT_HEIGHT = 400;
/**
* {@link Color#WHITE}
*/
public static final Color DEFAULT_BACKGROUND = Color.WHITE;
private static List terraria;
private JFrame frame;
private List turtles;
private List