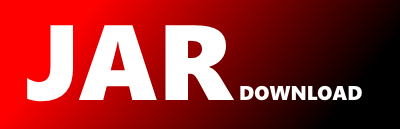
org.gatein.common.util.CollectionMap Maven / Gradle / Ivy
/******************************************************************************
* JBoss, a division of Red Hat *
* Copyright 2009, Red Hat Middleware, LLC, and individual *
* contributors as indicated by the @authors tag. See the *
* copyright.txt in the distribution for a full listing of *
* individual contributors. *
* *
* This is free software; you can redistribute it and/or modify it *
* under the terms of the GNU Lesser General Public License as *
* published by the Free Software Foundation; either version 2.1 of *
* the License, or (at your option) any later version. *
* *
* This software is distributed in the hope that it will be useful, *
* but WITHOUT ANY WARRANTY; without even the implied warranty of *
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU *
* Lesser General Public License for more details. *
* *
* You should have received a copy of the GNU Lesser General Public *
* License along with this software; if not, write to the Free *
* Software Foundation, Inc., 51 Franklin St, Fifth Floor, Boston, MA *
* 02110-1301 USA, or see the FSF site: http://www.fsf.org. *
******************************************************************************/
package org.gatein.common.util;
import java.io.Serializable;
import java.util.Collection;
import java.util.Collections;
import java.util.Comparator;
import java.util.HashMap;
import java.util.Iterator;
import java.util.Map;
import java.util.Set;
/**
* A map of collections.
*
* @author Julien Viet
* @version $Revision: 1.1 $
*/
@SuppressWarnings("serial")
public abstract class CollectionMap implements Serializable
{
/** The underlying map. */
private final Map> map;
/** An optional comparator. */
protected Comparator comparator;
public CollectionMap()
{
map = init(null);
}
public CollectionMap(CollectionMap other) throws IllegalArgumentException
{
if (other == null)
{
throw new IllegalArgumentException("Cannot copy null argument");
}
map = init(other);
}
public CollectionMap(CollectionMap other, Comparator comparator) throws IllegalArgumentException
{
this(other);
initComparator(comparator);
}
public CollectionMap(Comparator comparator)
{
this();
initComparator(comparator);
}
private void initComparator(Comparator comparator)
{
//
if (comparator == null)
{
throw new IllegalArgumentException("No null comparator allowed");
}
//
this.comparator = comparator;
}
/**
* Add an object in the set keyed under the specified key.
*
* @throws NullPointerException if the key is null
*/
public final void put(K key, V o) throws NullPointerException
{
if (key == null)
{
throw new NullPointerException("No null key");
}
//
Collection collection = map.get(key);
if (collection == null)
{
collection = newCollection();
map.put(key, collection);
}
add(collection, o);
}
/** Return the set of keys. */
public final Set keySet()
{
return map.keySet();
}
/**
* Remove the entire set of objects specified by the key.
*
* @throws NullPointerException if the key is null
*/
public final void remove(K key) throws NullPointerException
{
if (key == null)
{
throw new NullPointerException("No null key");
}
//
map.remove(key);
}
/**
* Remove an object in the set keyed under the specified key.
*
* @throws NullPointerException if the key is null
*/
public final void remove(K key, Object o) throws NullPointerException
{
if (key == null)
{
throw new NullPointerException("No null key");
}
//
Collection collection = map.get(key);
//
if (collection != null)
{
remove(collection, o);
//
if (collection.isEmpty())
{
map.remove(key);
}
}
}
/**
* Return true if the specified set contains the object o.
*
* @throws NullPointerException if the key is null
*/
public final boolean contains(K key, Object o) throws NullPointerException
{
if (key == null)
{
throw new NullPointerException("No null key");
}
//
Collection collection = map.get(key);
//
if (collection == null)
{
return false;
}
else
{
return collection.contains(o);
}
}
/** Return the collection specified by the key. */
public Collection get(K key)
{
return map.get(key);
}
/**
* Return an iterator over the values in the set specified by the key.
*
* @throws NullPointerException if the key is null
*/
public final Iterator iterator(final K key)
{
if (key == null)
{
throw new NullPointerException("No null key");
}
//
Collection set = map.get(key);
//
if (set == null)
{
Set tmp = Collections.emptySet();
return tmp.iterator();
}
else
{
final Iterator iterator = set.iterator();
return new Iterator()
{
public boolean hasNext()
{
return iterator.hasNext();
}
public V next()
{
return iterator.next();
}
public void remove()
{
iterator.remove();
if (!iterator.hasNext())
{
map.remove(key);
}
}
};
}
}
private Map> init(CollectionMap other)
{
Map> map = new HashMap>();
//
if (other != null)
{
for (Map.Entry> entry : other.map.entrySet())
{
K key = entry.getKey();
Collection value = entry.getValue();
map.put(key, newCollection(value));
}
}
//
return map;
}
protected abstract void add(Collection c, V o);
/**
* Removes an object from the collection. The type of the object to remove is intentionnally Object
and
* not the parameterized type
because the Collection interface is designed that way.
*
* @param c the collection to remove from
* @param o the object to remove
*/
protected abstract void remove(Collection c, Object o);
protected abstract Collection newCollection();
protected abstract Collection newCollection(Collection other);
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy