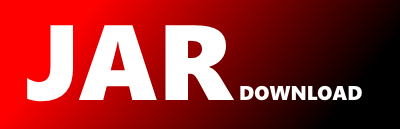
geb.navigator.Locator.groovy Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of geb-core Show documentation
Show all versions of geb-core Show documentation
Geb (pronounced "jeb") is a browser automation solution. It brings together the power of WebDriver, the elegance of jQuery content selection, the robustness of Page Object modelling and the expressiveness of the Groovy language.
The newest version!
/*
* Copyright 2014 the original author or authors.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package geb.navigator
import org.openqa.selenium.By
/**
* Allows to create {@link geb.navigator.Navigator}s by selecting {@link org.openqa.selenium.WebElement}s
* using different criteria (CSS selectors, attribute maps, indexes, ranges and {@link org.openqa.selenium.By} selectors).
*/
interface Locator extends BasicLocator {
public static final String MATCH_ALL_SELECTOR = "*"
/**
* Shorthand for find(null, selector, null)
*
* @return new Navigator
*/
Navigator find(String selector)
/**
* Shorthand for find(selector)
*
* @return new Navigator
* @see #find(java.lang.String)
*/
Navigator $(String selector)
/**
* Creates a new Navigator instance containing the elements whose attributes match the specified values or patterns.
* The key 'text' can be used to match the text contained in elements. Regular expression Pattern objects may be
* used as values.
* Examples:
*
* - find(name: "firstName")
* - selects all elements with the name "firstName"
* - find(name: "firstName", readonly: "readonly")
* - selects all elements with the name "firstName" that are read-only
* - find(text: "I can has cheezburger")
* - selects all elements containing the exact text
* - find(text: ~/I can has.+/)
* - selects all elements whose text matches a regular expression
*
* @param predicates a Map with keys representing attributes and values representing required values or patterns
* @return a new Navigator instance containing the matched elements
*/
Navigator find(Map attributes)
/**
* Shorthand for find(selector)[indexOfElement]
.
* @param selector a CSS selector
* @param index index of the required element in the selection
* @return new Navigator instance containing a single element
*/
Navigator find(String selector, int index)
/**
* Shorthand for find(null, selector, range)
*
* @param selector The css selector
* @return new Navigator
*/
Navigator find(String selector, Range range)
/**
* Shorthand for find(selector, index)
.
*
* @param selector a CSS selector
* @param index index of the required element in the selection
* @return new Navigator instance containing a single element
* @see #find(java.lang.String, int)
*/
Navigator $(String selector, int index)
/**
* Shorthand for find(selector, range)
*
* @param selector The css selector
* @return new Navigator
*/
Navigator $(String selector, Range range)
/**
* Shorthand for find(predicates, bySelector)
*
* @param bySelector a WebDriver By selector
* @param predicates a Map with keys representing attributes and values representing required values or patterns
* @return a new Navigator instance containing the matched elements
*/
Navigator $(Map attributes, By bySelector)
/**
* Shorthand for find(predicates, bySelector, index)
*
* @param bySelector a WebDriver By selector
* @return new Navigator
*/
Navigator $(Map attributes, By bySelector, int index)
/**
* Shorthand for find(predicates, bySelector, range)
*
* @param bySelector a WebDriver By selector
* @return new Navigator instance containing the matched elements
*/
Navigator $(Map attributes, By bySelector, Range range)
/**
* Shorthand for find(bySelector)
*
* @param bySelector a WebDriver By selector
* @return new Navigator
* @see BasicLocator#find(org.openqa.selenium.By)
*/
Navigator $(By bySelector)
/**
* Shorthand for find(bySelector, index)
.
*
* @param bySelector a WebDriver By selector
* @param index index of the required element in the selection
* @return new Navigator instance containing a single element
* @see BasicLocator#find(org.openqa.selenium.By, int)
*/
Navigator $(By bySelector, int index)
/**
* Shorthand for find(bySelector, range)
*
* @param bySelector a WebDriver By selector
* @return new Navigator
*/
Navigator $(By bySelector, Range range)
/**
* Shorthand for find(null, bySelector, range)
*
* @param bySelector a WebDriver By selector
* @return new Navigator
*/
Navigator find(By bySelector, Range range)
/**
* Shorthand for find(predicates)
*
* @param predicates a Map with keys representing attributes and values representing required values or patterns
* @return a new Navigator instance containing the matched elements
*/
Navigator $(Map attributes)
/**
* Shorthand for find(predicates, index)
*
* @return new Navigator
*/
Navigator $(Map attributes, int index)
/**
* Shorthand for find(predicates, range)
*
* @param predicates attribute predicates
* @param predicates range the range of matches to select
* @return new Navigator
*/
Navigator $(Map attributes, Range range)
/**
* Shorthand for find(predicates, selector)
*
* @param selector a CSS selector
* @param predicates a Map with keys representing attributes and values representing required values or patterns
* @return a new Navigator instance containing the matched elements
*/
Navigator $(Map attributes, String selector)
/**
* Shorthand for find(predicates, selector, index)
*
* @return new Navigator
*/
Navigator $(Map attributes, String selector, int index)
/**
* Shorthand for find(predicates, selector, range)
*
* @param selector a CSS selector
* @return new Navigator instance containing the matched elements
*/
Navigator $(Map attributes, String selector, Range range)
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy