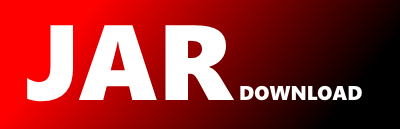
org.w3.owl.OwlFactory Maven / Gradle / Ivy
/*
* Copyright (c) 2012 - 2024 Data In Motion and others.
* All rights reserved.
*
* This program and the accompanying materials are made
* available under the terms of the Eclipse Public License 2.0
* which is available at https://www.eclipse.org/legal/epl-2.0/
*
* SPDX-License-Identifier: EPL-2.0
*
* Contributors:
* Mark Hoffmann - initial API and implementation
*/
package org.w3.owl;
import org.eclipse.emf.ecore.EFactory;
import org.osgi.annotation.versioning.ProviderType;
/**
*
* The Factory for the model.
* It provides a create method for each non-abstract class of the model.
*
* @see org.w3.owl.OwlPackage
* @generated
*/
@ProviderType
public interface OwlFactory extends EFactory {
/**
* The singleton instance of the factory.
*
*
* @generated
*/
OwlFactory eINSTANCE = org.w3.owl.impl.OwlFactoryImpl.init();
/**
* Returns a new object of class 'Nothing'.
*
*
* @return a new object of class 'Nothing'.
* @generated
*/
Nothing createNothing();
/**
* Returns a new object of class 'Thing'.
*
*
* @return a new object of class 'Thing'.
* @generated
*/
Thing createThing();
/**
* Returns a new object of class 'Typed Thing'.
*
*
* @return a new object of class 'Typed Thing'.
* @generated
*/
TypedThing createTypedThing();
/**
* Returns a new object of class 'Annotation'.
*
*
* @return a new object of class 'Annotation'.
* @generated
*/
Annotation createAnnotation();
/**
* Returns a new object of class 'Data Range'.
*
*
* @return a new object of class 'Data Range'.
* @generated
*/
DataRange createDataRange();
/**
* Returns a new object of class 'Object Property'.
*
*
* @return a new object of class 'Object Property'.
* @generated
*/
ObjectProperty createObjectProperty();
/**
* Returns a new object of class 'Named Individual'.
*
*
* @return a new object of class 'Named Individual'.
* @generated
*/
NamedIndividual createNamedIndividual();
/**
* Returns a new object of class 'Individual'.
*
*
* @return a new object of class 'Individual'.
* @generated
*/
Individual createIndividual();
/**
* Returns a new object of class 'real'.
*
*
* @return a new object of class 'real'.
* @generated
*/
real createreal();
/**
* Returns a new object of class 'Ontology Property'.
*
*
* @return a new object of class 'Ontology Property'.
* @generated
*/
OntologyProperty createOntologyProperty();
/**
* Returns a new object of class 'Class'.
*
*
* @return a new object of class 'Class'.
* @generated
*/
Class createClass();
/**
* Returns a new object of class 'Restriction'.
*
*
* @return a new object of class 'Restriction'.
* @generated
*/
Restriction createRestriction();
/**
* Returns a new object of class 'Asymmetric Property'.
*
*
* @return a new object of class 'Asymmetric Property'.
* @generated
*/
AsymmetricProperty createAsymmetricProperty();
/**
* Returns a new object of class 'Anonymous Individual'.
*
*
* @return a new object of class 'Anonymous Individual'.
* @generated
*/
AnonymousIndividual createAnonymousIndividual();
/**
* Returns a new object of class 'Transitive Property'.
*
*
* @return a new object of class 'Transitive Property'.
* @generated
*/
TransitiveProperty createTransitiveProperty();
/**
* Returns a new object of class 'rational'.
*
*
* @return a new object of class 'rational'.
* @generated
*/
rational createrational();
/**
* Returns a new object of class 'Reflexive Property'.
*
*
* @return a new object of class 'Reflexive Property'.
* @generated
*/
ReflexiveProperty createReflexiveProperty();
/**
* Returns a new object of class 'Ontology'.
*
*
* @return a new object of class 'Ontology'.
* @generated
*/
Ontology createOntology();
/**
* Returns a new object of class 'Symmetric Property'.
*
*
* @return a new object of class 'Symmetric Property'.
* @generated
*/
SymmetricProperty createSymmetricProperty();
/**
* Returns a new object of class 'All Disjoint Properties'.
*
*
* @return a new object of class 'All Disjoint Properties'.
* @generated
*/
AllDisjointProperties createAllDisjointProperties();
/**
* Returns a new object of class 'Axiom'.
*
*
* @return a new object of class 'Axiom'.
* @generated
*/
Axiom createAxiom();
/**
* Returns a new object of class 'Datatype Property'.
*
*
* @return a new object of class 'Datatype Property'.
* @generated
*/
DatatypeProperty createDatatypeProperty();
/**
* Returns a new object of class 'Datatype'.
*
*
* @return a new object of class 'Datatype'.
* @generated
*/
Datatype createDatatype();
/**
* Returns a new object of class 'Negative Property Assertion'.
*
*
* @return a new object of class 'Negative Property Assertion'.
* @generated
*/
NegativePropertyAssertion createNegativePropertyAssertion();
/**
* Returns a new object of class 'Functional Property'.
*
*
* @return a new object of class 'Functional Property'.
* @generated
*/
FunctionalProperty createFunctionalProperty();
/**
* Returns a new object of class 'All Disjoint Classes'.
*
*
* @return a new object of class 'All Disjoint Classes'.
* @generated
*/
AllDisjointClasses createAllDisjointClasses();
/**
* Returns a new object of class 'All Different'.
*
*
* @return a new object of class 'All Different'.
* @generated
*/
AllDifferent createAllDifferent();
/**
* Returns a new object of class 'Irreflexive Property'.
*
*
* @return a new object of class 'Irreflexive Property'.
* @generated
*/
IrreflexiveProperty createIrreflexiveProperty();
/**
* Returns a new object of class 'Annotation Property'.
*
*
* @return a new object of class 'Annotation Property'.
* @generated
*/
AnnotationProperty createAnnotationProperty();
/**
* Returns a new object of class 'Inverse Functional Property'.
*
*
* @return a new object of class 'Inverse Functional Property'.
* @generated
*/
InverseFunctionalProperty createInverseFunctionalProperty();
/**
* Returns a new object of class 'Deprecated Class'.
*
*
* @return a new object of class 'Deprecated Class'.
* @generated
*/
DeprecatedClass createDeprecatedClass();
/**
* Returns a new object of class 'Deprecated Property'.
*
*
* @return a new object of class 'Deprecated Property'.
* @generated
*/
DeprecatedProperty createDeprecatedProperty();
/**
* Returns a new object of class 'Property Chain Axiom'.
*
*
* @return a new object of class 'Property Chain Axiom'.
* @generated
*/
PropertyChainAxiom createPropertyChainAxiom();
/**
* Returns the package supported by this factory.
*
*
* @return the package supported by this factory.
* @generated
*/
OwlPackage getOwlPackage();
} //OwlFactory
© 2015 - 2025 Weber Informatics LLC | Privacy Policy