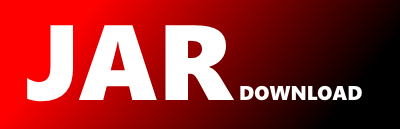
org.w3.owl.configuration.OwlConfigurationComponent Maven / Gradle / Ivy
/*
* Copyright (c) 2012 - 2024 Data In Motion and others.
* All rights reserved.
*
* This program and the accompanying materials are made
* available under the terms of the Eclipse Public License 2.0
* which is available at https://www.eclipse.org/legal/epl-2.0/
*
* SPDX-License-Identifier: EPL-2.0
*
* Contributors:
* Mark Hoffmann - initial API and implementation
*/
package org.w3.owl.configuration;
import java.util.Hashtable;
import org.eclipse.emf.ecore.EFactory;
import org.eclipse.emf.ecore.EPackage;
import org.eclipse.emf.ecore.resource.Resource.Factory;
import org.gecko.emf.osgi.configurator.EPackageConfigurator;
import org.osgi.annotation.bundle.Capability;
import org.osgi.framework.BundleContext;
import org.osgi.framework.ServiceRegistration;
import org.osgi.service.component.annotations.Activate;
import org.osgi.service.component.annotations.Component;
import org.osgi.service.component.annotations.Deactivate;
import org.osgi.service.condition.Condition;
import org.w3.owl.OwlFactory;
import org.w3.owl.OwlPackage;
import org.w3.owl.impl.OwlPackageImpl;
import org.w3.owl.util.OwlResourceFactoryImpl;
/**
* The PackageConfiguration for the model.
* The package will be registered into a OSGi base model registry.
*
* @generated
*/
@Component(name = "OwlConfigurator")
@Capability( namespace = "osgi.service", attribute = { "objectClass:List=\"org.w3.owl.util.OwlResourceFactoryImpl, org.eclipse.emf.ecore.resource.Resource$Factory\"" , "uses:=\"org.eclipse.emf.ecore.resource,org.w3.owl.util\"" })
@Capability( namespace = "osgi.service", attribute = { "objectClass:List=\"org.w3.owl.OwlFactory, org.eclipse.emf.ecore.EFactory\"" , "uses:=\"org.eclipse.emf.ecore,org.w3.owl\"" })
@Capability( namespace = "osgi.service", attribute = { "objectClass:List=\"org.w3.owl.OwlPackage, org.eclipse.emf.ecore.EPackage\"" , "uses:=\"org.eclipse.emf.ecore,org.w3.owl\"" })
@Capability( namespace = "osgi.service", attribute = { "objectClass:List=\"org.gecko.emf.osgi.configurator.EPackageConfigurator\"" , "uses:=\"org.eclipse.emf.ecore,org.w3.owl\"" })
@Capability( namespace = "osgi.service", attribute = { "objectClass:List=\"org.osgi.service.condition.Condition\"" , "uses:=org.osgi.service.condition" })
public class OwlConfigurationComponent {
private ServiceRegistration> packageRegistration = null;
private ServiceRegistration ePackageConfiguratorRegistration = null;
private ServiceRegistration> eFactoryRegistration = null;
private ServiceRegistration> conditionRegistration = null;
private ServiceRegistration> resourceFactoryRegistration = null;
/**
* Activates the Configuration Component.
*
* @generated
*/
@Activate
public void activate(BundleContext ctx) {
OwlPackage ePackage = OwlPackageImpl.eINSTANCE;
OwlEPackageConfigurator packageConfigurator = registerEPackageConfiguratorService(ePackage, ctx);
registerResourceFactoryService(ctx);
registerEPackageService(ePackage, packageConfigurator, ctx);
registerEFactoryService(ePackage, packageConfigurator, ctx);
registerConditionService(packageConfigurator, ctx);
}
/**
* Registers the OwlEPackageConfigurator as a service.
*
* @generated
*/
private OwlEPackageConfigurator registerEPackageConfiguratorService(OwlPackage ePackage, BundleContext ctx){
OwlEPackageConfigurator packageConfigurator = new OwlEPackageConfigurator(ePackage);
// register the EPackageConfigurator
Hashtable properties = new Hashtable();
properties.putAll(packageConfigurator.getServiceProperties());
ePackageConfiguratorRegistration = ctx.registerService(EPackageConfigurator.class, packageConfigurator, properties);
return packageConfigurator;
}
/**
* Registers the OwlResourceFactoryImpl as a service.
*
* @generated
*/
private void registerResourceFactoryService(BundleContext ctx){
OwlResourceFactoryImpl factory = new OwlResourceFactoryImpl();
Hashtable properties = new Hashtable();
properties.putAll(factory.getServiceProperties());
String[] serviceClasses = new String[] {OwlResourceFactoryImpl.class.getName(), Factory.class.getName()};
resourceFactoryRegistration = ctx.registerService(serviceClasses, factory, properties);
}
/**
* Registers the OwlPackage as a service.
*
* @generated
*/
private void registerEPackageService(OwlPackage ePackage, OwlEPackageConfigurator packageConfigurator, BundleContext ctx){
Hashtable properties = new Hashtable();
properties.putAll(packageConfigurator.getServiceProperties());
String[] serviceClasses = new String[] {OwlPackage.class.getName(), EPackage.class.getName()};
packageRegistration = ctx.registerService(serviceClasses, ePackage, properties);
}
/**
* Registers the OwlFactory as a service.
*
* @generated
*/
private void registerEFactoryService(OwlPackage ePackage, OwlEPackageConfigurator packageConfigurator, BundleContext ctx){
Hashtable properties = new Hashtable();
properties.putAll(packageConfigurator.getServiceProperties());
String[] serviceClasses = new String[] {OwlFactory.class.getName(), EFactory.class.getName()};
eFactoryRegistration = ctx.registerService(serviceClasses, ePackage.getOwlFactory(), properties);
}
private void registerConditionService(OwlEPackageConfigurator packageConfigurator, BundleContext ctx){
// register the EPackage
Hashtable properties = new Hashtable();
properties.putAll(packageConfigurator.getServiceProperties());
properties.put(Condition.CONDITION_ID, OwlPackage.eNS_URI);
conditionRegistration = ctx.registerService(Condition.class, Condition.INSTANCE, properties);
}
/**
* Deactivates and unregisters everything.
*
* @generated
*/
@Deactivate
public void deactivate() {
conditionRegistration.unregister();
eFactoryRegistration.unregister();
packageRegistration.unregister();
resourceFactoryRegistration.unregister();
ePackageConfiguratorRegistration.unregister();
EPackage.Registry.INSTANCE.remove(OwlPackage.eNS_URI);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy