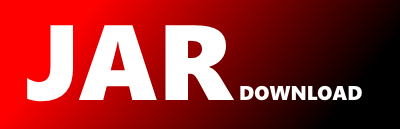
org.w3.rdfs.impl.SeeAlsoImpl Maven / Gradle / Ivy
/*
* Copyright (c) 2012 - 2024 Data In Motion and others.
* All rights reserved.
*
* This program and the accompanying materials are made
* available under the terms of the Eclipse Public License 2.0
* which is available at https://www.eclipse.org/legal/epl-2.0/
*
* SPDX-License-Identifier: EPL-2.0
*
* Contributors:
* Mark Hoffmann - initial API and implementation
*/
package org.w3.rdfs.impl;
import org.eclipse.emf.common.notify.Notification;
import org.eclipse.emf.common.notify.NotificationChain;
import org.eclipse.emf.ecore.EClass;
import org.eclipse.emf.ecore.InternalEObject;
import org.eclipse.emf.ecore.impl.ENotificationImpl;
import org.eclipse.emf.ecore.impl.MinimalEObjectImpl;
import org.w3.rdfs.RDFResource;
import org.w3.rdfs.RdfsPackage;
import org.w3.rdfs.SeeAlso;
/**
*
* An implementation of the model object 'See Also'.
*
*
* The following features are implemented:
*
*
* - {@link org.w3.rdfs.impl.SeeAlsoImpl#getResource Resource}
* - {@link org.w3.rdfs.impl.SeeAlsoImpl#getSeeAlso See Also}
*
*
* @generated
*/
public class SeeAlsoImpl extends MinimalEObjectImpl.Container implements SeeAlso {
/**
* The cached value of the '{@link #getResource() Resource}' reference.
*
*
* @see #getResource()
* @generated
* @ordered
*/
protected RDFResource resource;
/**
* The cached value of the '{@link #getSeeAlso() See Also}' reference.
*
*
* @see #getSeeAlso()
* @generated
* @ordered
*/
protected RDFResource seeAlso;
/**
*
*
* @generated
*/
protected SeeAlsoImpl() {
super();
}
/**
*
*
* @generated
*/
@Override
protected EClass eStaticClass() {
return RdfsPackage.Literals.SEE_ALSO;
}
/**
*
*
* @generated
*/
@Override
public RDFResource getResource() {
if (resource != null && resource.eIsProxy()) {
InternalEObject oldResource = (InternalEObject)resource;
resource = (RDFResource)eResolveProxy(oldResource);
if (resource != oldResource) {
if (eNotificationRequired())
eNotify(new ENotificationImpl(this, Notification.RESOLVE, RdfsPackage.SEE_ALSO__RESOURCE, oldResource, resource));
}
}
return resource;
}
/**
*
*
* @generated
*/
public RDFResource basicGetResource() {
return resource;
}
/**
*
*
* @generated
*/
public NotificationChain basicSetResource(RDFResource newResource, NotificationChain msgs) {
RDFResource oldResource = resource;
resource = newResource;
if (eNotificationRequired()) {
ENotificationImpl notification = new ENotificationImpl(this, Notification.SET, RdfsPackage.SEE_ALSO__RESOURCE, oldResource, newResource);
if (msgs == null) msgs = notification; else msgs.add(notification);
}
return msgs;
}
/**
*
*
* @generated
*/
@Override
public void setResource(RDFResource newResource) {
if (newResource != resource) {
NotificationChain msgs = null;
if (resource != null)
msgs = ((InternalEObject)resource).eInverseRemove(this, RdfsPackage.RDF_RESOURCE__SEE_ALSO, RDFResource.class, msgs);
if (newResource != null)
msgs = ((InternalEObject)newResource).eInverseAdd(this, RdfsPackage.RDF_RESOURCE__SEE_ALSO, RDFResource.class, msgs);
msgs = basicSetResource(newResource, msgs);
if (msgs != null) msgs.dispatch();
}
else if (eNotificationRequired())
eNotify(new ENotificationImpl(this, Notification.SET, RdfsPackage.SEE_ALSO__RESOURCE, newResource, newResource));
}
/**
*
*
* @generated
*/
@Override
public RDFResource getSeeAlso() {
if (seeAlso != null && seeAlso.eIsProxy()) {
InternalEObject oldSeeAlso = (InternalEObject)seeAlso;
seeAlso = (RDFResource)eResolveProxy(oldSeeAlso);
if (seeAlso != oldSeeAlso) {
if (eNotificationRequired())
eNotify(new ENotificationImpl(this, Notification.RESOLVE, RdfsPackage.SEE_ALSO__SEE_ALSO, oldSeeAlso, seeAlso));
}
}
return seeAlso;
}
/**
*
*
* @generated
*/
public RDFResource basicGetSeeAlso() {
return seeAlso;
}
/**
*
*
* @generated
*/
@Override
public void setSeeAlso(RDFResource newSeeAlso) {
RDFResource oldSeeAlso = seeAlso;
seeAlso = newSeeAlso;
if (eNotificationRequired())
eNotify(new ENotificationImpl(this, Notification.SET, RdfsPackage.SEE_ALSO__SEE_ALSO, oldSeeAlso, seeAlso));
}
/**
*
*
* @generated
*/
@Override
public NotificationChain eInverseAdd(InternalEObject otherEnd, int featureID, NotificationChain msgs) {
switch (featureID) {
case RdfsPackage.SEE_ALSO__RESOURCE:
if (resource != null)
msgs = ((InternalEObject)resource).eInverseRemove(this, RdfsPackage.RDF_RESOURCE__SEE_ALSO, RDFResource.class, msgs);
return basicSetResource((RDFResource)otherEnd, msgs);
}
return super.eInverseAdd(otherEnd, featureID, msgs);
}
/**
*
*
* @generated
*/
@Override
public NotificationChain eInverseRemove(InternalEObject otherEnd, int featureID, NotificationChain msgs) {
switch (featureID) {
case RdfsPackage.SEE_ALSO__RESOURCE:
return basicSetResource(null, msgs);
}
return super.eInverseRemove(otherEnd, featureID, msgs);
}
/**
*
*
* @generated
*/
@Override
public Object eGet(int featureID, boolean resolve, boolean coreType) {
switch (featureID) {
case RdfsPackage.SEE_ALSO__RESOURCE:
if (resolve) return getResource();
return basicGetResource();
case RdfsPackage.SEE_ALSO__SEE_ALSO:
if (resolve) return getSeeAlso();
return basicGetSeeAlso();
}
return super.eGet(featureID, resolve, coreType);
}
/**
*
*
* @generated
*/
@Override
public void eSet(int featureID, Object newValue) {
switch (featureID) {
case RdfsPackage.SEE_ALSO__RESOURCE:
setResource((RDFResource)newValue);
return;
case RdfsPackage.SEE_ALSO__SEE_ALSO:
setSeeAlso((RDFResource)newValue);
return;
}
super.eSet(featureID, newValue);
}
/**
*
*
* @generated
*/
@Override
public void eUnset(int featureID) {
switch (featureID) {
case RdfsPackage.SEE_ALSO__RESOURCE:
setResource((RDFResource)null);
return;
case RdfsPackage.SEE_ALSO__SEE_ALSO:
setSeeAlso((RDFResource)null);
return;
}
super.eUnset(featureID);
}
/**
*
*
* @generated
*/
@Override
public boolean eIsSet(int featureID) {
switch (featureID) {
case RdfsPackage.SEE_ALSO__RESOURCE:
return resource != null;
case RdfsPackage.SEE_ALSO__SEE_ALSO:
return seeAlso != null;
}
return super.eIsSet(featureID);
}
} //SeeAlsoImpl
© 2015 - 2025 Weber Informatics LLC | Privacy Policy