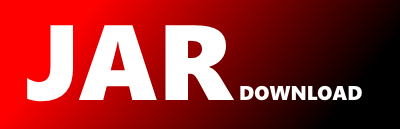
org.gecko.emf.rest.common.AbstractEMFAnnotationHandler Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of org.gecko.emf.rest.jakartars Show documentation
Show all versions of org.gecko.emf.rest.jakartars Show documentation
Extension to load and save EMF EObjects using a MessageBodyReader or -Writer
/**
* Copyright (c) 2012 - 2023 Data In Motion and others.
* All rights reserved.
*
* This program and the accompanying materials are made available under the terms of the
* Eclipse Public License v2.0 which accompanies this distribution, and is available at
* http://www.eclipse.org/legal/epl-v20.html
*
* Contributors:
* Data In Motion - initial API and implementation
*/
package org.gecko.emf.rest.common;
import java.lang.annotation.Annotation;
import java.text.SimpleDateFormat;
import java.util.LinkedList;
import java.util.List;
import java.util.Map;
import org.eclipse.emf.common.util.Diagnostic;
import org.eclipse.emf.common.util.URI;
import org.eclipse.emf.ecore.EClass;
import org.eclipse.emf.ecore.resource.Resource;
import org.eclipse.emf.ecore.resource.ResourceSet;
import org.gecko.emf.rest.annotations.AnnotationConverter;
import org.gecko.emf.rest.annotations.ContentNotEmpty;
import org.gecko.emf.rest.annotations.EMFResourceOptions;
import org.gecko.emf.rest.annotations.ResourceEClass;
import org.gecko.emf.rest.annotations.ResourceOption;
import org.gecko.emf.rest.annotations.ValidateContent;
/**
* Base class to handle EMF resource annotation and turn them into EMF load or
* save options
*
* @author Mark Hoffmann
*/
public abstract class AbstractEMFAnnotationHandler {
protected List annotationConverters = new LinkedList<>();
/**
* Reads the annotations for {@link EMFResourceOptions} and adds them to the
* given {@link Map}
*
* @param annotations the {@link Annotation} Array to parse
* @param options the options {@link Map} to add the annotations content to
* @param resourceSet the {@link ResourceSet} to use
*/
protected void handleAnnotedOptions(Annotation[] annotations, Map
© 2015 - 2024 Weber Informatics LLC | Privacy Policy