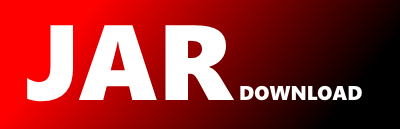
org.gecko.emf.osgi.helper.ServicePropertiesHelper Maven / Gradle / Ivy
The newest version!
/**
* Copyright (c) 2012 - 2022 Data In Motion and others.
* All rights reserved.
*
* This program and the accompanying materials are made
* available under the terms of the Eclipse Public License 2.0
* which is available at https://www.eclipse.org/legal/epl-2.0/
*
* SPDX-License-Identifier: EPL-2.0
*
* Contributors:
* Data In Motion - initial API and implementation
*/
package org.gecko.emf.osgi.helper;
import static java.util.Objects.isNull;
import static java.util.Objects.nonNull;
import static java.util.Objects.requireNonNull;
import java.util.Arrays;
import java.util.Collection;
import java.util.Collections;
import java.util.Dictionary;
import java.util.HashSet;
import java.util.Map;
import java.util.Map.Entry;
import java.util.Objects;
import java.util.Optional;
import java.util.Set;
import java.util.stream.Collectors;
import org.osgi.framework.Constants;
/**
* A Helper to update the given Service Properties
* @author Juergen Albert
* @since 22 Feb 2022
*/
public class ServicePropertiesHelper {
private ServicePropertiesHelper() {
}
/**
* Merges the source map into the target map and appends the values
* @param source the source map, must not be null
* @param target the map to merge into, must not be null
*/
public static void mergeNameMap(Map> source, Map> target) {
requireNonNull(source);
requireNonNull(target);
source.forEach((key, value)->appendNameMap(target, value, key, true));
}
/**
* Updates the name set for a given service id and appends the new names
* @param nameMap the map to update
* @param newNames the new properties
* @param serviceId the service id the name set belongs to
* @param append set to true
to append the values instead of replacing them
*/
public static void appendNameMap(Map> nameMap, Set newNames, Long serviceId, boolean append) {
requireNonNull(nameMap);
requireNonNull(newNames);
requireNonNull(serviceId);
Set nameSet = nameMap.get(serviceId);
if (isNull(nameSet)) {
nameSet = new HashSet<>();
nameMap.put(serviceId, nameSet);
}
if (!append) {
nameSet.clear();
}
nameSet.addAll(newNames);
}
/**
* Updates the name set for a given service id
* @param nameMap the map to update
* @param newNames the new properties
* @param serviceId the service id the name set belongs to
*/
public static void updateNameMap(Map> nameMap, Set newNames, Long serviceId) {
appendNameMap(nameMap, newNames, serviceId, false);
}
/**
* Return all values of all map entries as array
* @param nameMap the map to return values from
* @return a string array
*/
public static String[] getNamesArray(Map> nameMap) {
return nameMap.entrySet()
.stream()
.filter(e->Objects.nonNull(e.getValue()))
.map(Entry::getValue)
.flatMap(Collection::stream)
.collect(Collectors.toSet())
.toArray(new String[0]);
}
/**
* returns a String+ value and recognizes Object, Object[] and Collection
© 2015 - 2024 Weber Informatics LLC | Privacy Policy