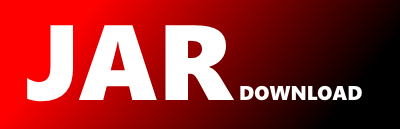
org.eclipse.paho.mqttv5.client.IMqttAsyncClient Maven / Gradle / Ivy
/*******************************************************************************
* Copyright (c) 2009, 2018 IBM Corp.
*
* All rights reserved. This program and the accompanying materials
* are made available under the terms of the Eclipse Public License v2.0
* and Eclipse Distribution License v1.0 which accompany this distribution.
*
* The Eclipse Public License is available at
* https://www.eclipse.org/legal/epl-2.0
* and the Eclipse Distribution License is available at
* https://www.eclipse.org/org/documents/edl-v10.php
*
* Contributors:
* James Sutton - MQTT V5 support
*/
package org.eclipse.paho.mqttv5.client;
import org.eclipse.paho.mqttv5.client.util.Debug;
import org.eclipse.paho.mqttv5.common.MqttException;
import org.eclipse.paho.mqttv5.common.MqttMessage;
import org.eclipse.paho.mqttv5.common.MqttPersistenceException;
import org.eclipse.paho.mqttv5.common.MqttSecurityException;
import org.eclipse.paho.mqttv5.common.MqttSubscription;
import org.eclipse.paho.mqttv5.common.packet.MqttProperties;
public interface IMqttAsyncClient {
/**
* Connects to an MQTT server using the default options.
*
* The default options are specified in {@link MqttConnectionOptions} class.
*
*
* @param userContext
* optional object used to pass context to the callback. Use null if
* not required.
* @param callback
* optional listener that will be notified when the connect
* completes. Use null if not required.
* @throws MqttSecurityException
* for security related problems
* @throws MqttException
* for non security related problems
* @return token used to track and wait for the connect to complete. The token
* will be passed to any callback that has been set.
* @see #connect(MqttConnectionOptions, Object, MqttActionListener)
*/
public IMqttToken connect(Object userContext, MqttActionListener callback) throws MqttException, MqttSecurityException;
/**
* Connects to an MQTT server using the default options.
*
* The default options are specified in {@link MqttConnectionOptions} class.
*
*
* @throws MqttSecurityException
* for security related problems
* @throws MqttException
* for non security related problems
* @return token used to track and wait for the connect to complete. The token
* will be passed to the callback methods if a callback is set.
* @see #connect(MqttConnectionOptions, Object, MqttActionListener)
*/
public IMqttToken connect() throws MqttException, MqttSecurityException;
/**
* Connects to an MQTT server using the provided connect options.
*
* The connection will be established using the options specified in the
* {@link MqttConnectionOptions} parameter.
*
*
* @param options
* a set of connection parameters that override the defaults.
* @throws MqttSecurityException
* for security related problems
* @throws MqttException
* for non security related problems
* @return token used to track and wait for the connect to complete. The token
* will be passed to any callback that has been set.
* @see #connect(MqttConnectionOptions, Object, MqttActionListener)
*/
public IMqttToken connect(MqttConnectionOptions options) throws MqttException, MqttSecurityException;
/**
* Connects to an MQTT server using the specified options.
*
* The server to connect to is specified on the constructor. It is recommended
* to call {@link #setCallback(MqttCallback)} prior to connecting in order that
* messages destined for the client can be accepted as soon as the client is
* connected.
*
*
* The method returns control before the connect completes. Completion can be
* tracked by:
*
*
* - Waiting on the returned token {@link IMqttToken#waitForCompletion()}
* or
* - Passing in a callback {@link MqttActionListener}
*
*
* @param options
* a set of connection parameters that override the defaults.
* @param userContext
* optional object for used to pass context to the callback. Use null
* if not required.
* @param callback
* optional listener that will be notified when the connect
* completes. Use null if not required.
* @return token used to track and wait for the connect to complete. The token
* will be passed to any callback that has been set.
* @throws MqttSecurityException
* for security related problems
* @throws MqttException
* for non security related problems including communication errors
*/
public IMqttToken connect(MqttConnectionOptions options, Object userContext, MqttActionListener callback)
throws MqttException, MqttSecurityException;
/**
* Disconnects from the server.
*
* An attempt is made to quiesce the client allowing outstanding work to
* complete before disconnecting. It will wait for a maximum of 30 seconds for
* work to quiesce before disconnecting. This method must not be called from
* inside {@link MqttCallback} methods.
*
*
* @param userContext
* optional object used to pass context to the callback. Use null if
* not required.
* @param callback
* optional listener that will be notified when the disconnect
* completes. Use null if not required.
* @return token used to track and wait for the disconnect to complete. The
* token will be passed to any callback that has been set.
* @throws MqttException
* for problems encountered while disconnecting
* @see #disconnect(long, Object, MqttActionListener, int, MqttProperties)
*
*/
public IMqttToken disconnect(Object userContext, MqttActionListener callback) throws MqttException;
/**
* Disconnects from the server.
*
* An attempt is made to quiesce the client allowing outstanding work to
* complete before disconnecting. It will wait for a maximum of 30 seconds for
* work to quiesce before disconnecting. This method must not be called from
* inside {@link MqttCallback} methods.
*
*
* @return token used to track and wait for disconnect to complete. The token
* will be passed to any callback that has been set.
* @throws MqttException
* for problems encountered while disconnecting
* @see #disconnect(long, Object, MqttActionListener, int, MqttProperties)
*/
public IMqttToken disconnect() throws MqttException;
/**
* Disconnects from the server.
*
* An attempt is made to quiesce the client allowing outstanding work to
* complete before disconnecting. It will wait for a maximum of the specified
* quiesce time for work to complete before disconnecting. This method must not
* be called from inside {@link MqttCallback} methods.
*
*
* @param quiesceTimeout
* the amount of time in milliseconds to allow for existing work to
* finish before disconnecting. A value of zero or less means the
* client will not quiesce.
* @return token used to track and wait for disconnect to complete. The token
* will be passed to the callback methods if a callback is set.
* @throws MqttException
* for problems encountered while disconnecting
* @see #disconnect(long, Object, MqttActionListener, int, MqttProperties)
*/
public IMqttToken disconnect(long quiesceTimeout) throws MqttException;
/**
* Disconnects from the server.
*
* The client will wait for {@link MqttCallback} methods to complete. It will
* then wait for up to the quiesce timeout to allow for work which has already
* been initiated to complete. For instance when a QoS 2 message has started
* flowing to the server but the QoS 2 flow has not completed.It prevents new
* messages being accepted and does not send any messages that have been
* accepted but not yet started delivery across the network to the server. When
* work has completed or after the quiesce timeout, the client will disconnect
* from the server. If the cleanStart flag was set to false and is set to
* false the next time a connection is made QoS 1 and 2 messages that were not
* previously delivered will be delivered.
*
*
* This method must not be called from inside {@link MqttCallback} methods.
*
*
* The method returns control before the disconnect completes. Completion can be
* tracked by:
*
*
* - Waiting on the returned token {@link IMqttToken#waitForCompletion()}
* or
* - Passing in a callback {@link MqttActionListener}
*
*
* @param quiesceTimeout
* the amount of time in milliseconds to allow for existing work to
* finish before disconnecting. A value of zero or less means the
* client will not quiesce.
* @param userContext
* optional object used to pass context to the callback. Use null if
* not required.
* @param callback
* optional listener that will be notified when the disconnect
* completes. Use null if not required.
* @param reasonCode
* the disconnection reason code.
* @param disconnectProperties
* The {@link MqttProperties} to be sent.
* @return token used to track and wait for the connect to complete. The token
* will be passed to any callback that has been set.
* @throws MqttException
* for problems encountered while disconnecting
*/
public IMqttToken disconnect(long quiesceTimeout, Object userContext, MqttActionListener callback, int reasonCode,
MqttProperties disconnectProperties) throws MqttException;
/**
* Disconnects from the server forcibly to reset all the states. Could be useful
* when disconnect attempt failed.
*
* Because the client is able to establish the TCP/IP connection to a none MQTT
* server and it will certainly fail to send the disconnect packet. It will wait
* for a maximum of 30 seconds for work to quiesce before disconnecting and wait
* for a maximum of 10 seconds for sending the disconnect packet to server.
*
* @throws MqttException
* if any unexpected error
* @since 0.4.1
*/
public void disconnectForcibly() throws MqttException;
/**
* Disconnects from the server forcibly to reset all the states. Could be useful
* when disconnect attempt failed.
*
* Because the client is able to establish the TCP/IP connection to a none MQTT
* server and it will certainly fail to send the disconnect packet. It will wait
* for a maximum of 30 seconds for work to quiesce before disconnecting.
*
* @param disconnectTimeout
* the amount of time in milliseconds to allow send disconnect packet
* to server.
* @throws MqttException
* if any unexpected error
* @since 0.4.1
*/
public void disconnectForcibly(long disconnectTimeout) throws MqttException;
/**
* Disconnects from the server forcibly to reset all the states. Could be useful
* when disconnect attempt failed.
*
* Because the client is able to establish the TCP/IP connection to a none MQTT
* server and it will certainly fail to send the disconnect packet.
*
* @param quiesceTimeout
* the amount of time in milliseconds to allow for existing work to
* finish before disconnecting. A value of zero or less means the
* client will not quiesce.
* @param disconnectTimeout
* the amount of time in milliseconds to allow send disconnect packet
* to server.
* @param reasonCode
* the disconnection reason code.
* @param disconnectProperties
* The {@link MqttProperties} to be sent.
* @throws MqttException
* if any unexpected error
* @since 0.4.1
*/
public void disconnectForcibly(long quiesceTimeout, long disconnectTimeout, int reasonCode,
MqttProperties disconnectProperties) throws MqttException;
/**
* Disconnects from the server forcibly to reset all the states. Could be useful
* when disconnect attempt failed.
*
* Because the client is able to establish the TCP/IP connection to a none MQTT
* server and it will certainly fail to send the disconnect packet.
*
* @param quiesceTimeout
* the amount of time in milliseconds to allow for existing work to
* finish before disconnecting. A value of zero or less means the
* client will not quiesce.
* @param disconnectTimeout
* the amount of time in milliseconds to allow send disconnect packet
* to server.
* @param sendDisconnectPacket
* if true, will send the disconnect packet to the server
* @throws MqttException
* if any unexpected error
*/
public void disconnectForcibly(long quiesceTimeout, long disconnectTimeout, boolean sendDisconnectPacket)
throws MqttException;
/**
* Determines if this client is currently connected to the server.
*
* @return true
if connected, false
otherwise.
*/
public boolean isConnected();
/**
* Returns the client ID used by this client.
*
* All clients connected to the same server or server farm must have a unique
* ID.
*
*
* @return the client ID used by this client.
*/
public String getClientId();
public void setClientId(String clientId);
/**
* Returns the address of the server used by this client.
*
* The format of the returned String is the same as that used on the
* constructor.
*
*
* @return the server's address, as a URI String.
* @see MqttAsyncClient#MqttAsyncClient(String, String)
*/
public String getServerURI();
/**
* Returns the currently connected Server URI Implemented due to:
* https://bugs.eclipse.org/bugs/show_bug.cgi?id=481097
*
* Where getServerURI only returns the URI that was provided in
* MqttAsyncClient's constructor, getCurrentServerURI returns the URI of the
* Server that the client is currently connected to. This would be different in
* scenarios where multiple server URIs have been provided to the
* MqttConnectOptions.
*
* @return the currently connected server URI
*/
public String getCurrentServerURI();
/*
* (non-Javadoc) Check and send a ping if needed. By default, client sends
* PingReq to server to keep the connection to server. For some platforms which
* cannot use this mechanism, such as Android, developer needs to handle the
* ping request manually with this method.
*
* @throws MqttException for other errors encountered while publishing the
* message.
*/
public IMqttToken checkPing(Object userContext, MqttActionListener callback) throws MqttException;
/**
* Subscribe to a topic, which may include wildcards.
*
* @see #subscribe(MqttSubscription[], Object, MqttActionListener,
* MqttProperties) throws MqttException
*
* @param topicFilter
* the topic to subscribe to, which can include wildcards.
* @param qos
* the maximum quality of service at which to subscribe. Messages
* published at a lower quality of service will be received at the
* published QoS. Messages published at a higher quality of service
* will be received using the QoS specified on the subscribe.
* @param userContext
* optional object used to pass context to the callback. Use null if
* not required.
* @param callback
* optional listener that will be notified when subscribe has
* completed
* @return token used to track and wait for the subscribe to complete. The token
* will be passed to callback methods if set.
* @throws MqttException
* if there was an error registering the subscription.
*/
public IMqttToken subscribe(String topicFilter, int qos, Object userContext, MqttActionListener callback)
throws MqttException;
public IMqttToken subscribe(String[] topicFilters, int[] qos, Object userContext, MqttActionListener callback)
throws MqttException;
/**
* Subscribe to a topic, which may include wildcards.
*
* @see #subscribe(MqttSubscription[], Object, MqttActionListener,
* MqttProperties)
*
* @param topicFilter
* the topic to subscribe to, which can include wildcards.
* @param qos
* the maximum quality of service at which to subscribe. Messages
* published at a lower quality of service will be received at the
* published QoS. Messages published at a higher quality of service
* will be received using the QoS specified on the subscribe.
* @return token used to track and wait for the subscribe to complete. The token
* will be passed to callback methods if set.
* @throws MqttException
* if there was an error registering the subscription.
*/
public IMqttToken subscribe(String topicFilter, int qos) throws MqttException;
public IMqttToken subscribe(String[] topicFilters, int[] qos) throws MqttException;
/**
* Subscribe to multiple topics, each of which may include wildcards.
*
*
* Provides an optimized way to subscribe to multiple topics compared to
* subscribing to each one individually.
*
*
* @see #subscribe(MqttSubscription[], Object, MqttActionListener,
* MqttProperties)
*
* @param subscriptions
* one or more {@link MqttSubscription} defining the subscription to
* be made.
* @return token used to track and wait for the subscribe to complete. The token
* will be passed to callback methods if set.
* @throws MqttException
* if there was an error registering the subscription.
*/
public IMqttToken subscribe(MqttSubscription[] subscriptions) throws MqttException;
/**
* Subscribes to multiple topics, each of which may include wildcards.
*
* Provides an optimized way to subscribe to multiple topics compared to
* subscribing to each one individually.
*
*
* The {@link #setCallback(MqttCallback)} method should be called before this
* method, otherwise any received messages will be discarded.
*
*
* If (@link MqttConnectOptions#setCleanStart(boolean)} was set to true when
* when connecting to the server then the subscription remains in place until
* either:
*
*
*
* - The client disconnects
* - An unsubscribe method is called to un-subscribe the topic
*
*
*
* If (@link MqttConnectOptions#setCleanStart(boolean)} was set to false when
* connecting to the server then the subscription remains in place until either:
*
*
* - An unsubscribe method is called to unsubscribe the topic
* - The next time the client connects with cleanStart set to true
*
*
* With cleanStart set to false the MQTT server will store messages on behalf
* of the client when the client is not connected. The next time the client
* connects with the same client ID the server will deliver the stored
* messages to the client.
*
*
*
* The "topic filter" string used when subscribing may contain special
* characters, which allow you to subscribe to multiple topics at once.
*
*
* The topic level separator is used to introduce structure into the topic, and
* can therefore be specified within the topic for that purpose. The multi-level
* wildcard and single-level wildcard can be used for subscriptions, but they
* cannot be used within a topic by the publisher of a message.
*
* - Topic level separator
* - The forward slash (/) is used to separate each level within a topic tree
* and provide a hierarchical structure to the topic space. The use of the topic
* level separator is significant when the two wildcard characters are
* encountered in topics specified by subscribers.
*
* - Multi-level wildcard
* -
*
* The number sign (#) is a wildcard character that matches any number of levels
* within a topic. For example, if you subscribe to
* finance/stock/ibm/#, you receive
* messages on these topics:
*
*
* - finance/stock/ibm
* - finance/stock/ibm/closingprice
* - finance/stock/ibm/currentprice
*
*
* The multi-level wildcard can represent zero or more levels. Therefore,
* finance/# can also match the singular finance, where
* # represents zero levels. The topic level separator is meaningless
* in this context, because there are no levels to separate.
*
*
*
* The multi-level wildcard can be specified only on its own or
* next to the topic level separator character. Therefore, # and
* finance/# are both valid, but finance# is not valid.
* The multi-level wildcard must be the last character used within the
* topic tree. For example, finance/# is valid but
* finance/#/closingprice is not valid.
*
*
*
* - Single-level wildcard
* -
*
* The plus sign (+) is a wildcard character that matches only one topic level.
* For example, finance/stock/+ matches finance/stock/ibm and
* finance/stock/xyz, but not finance/stock/ibm/closingprice.
* Also, because the single-level wildcard matches only a single level,
* finance/+ does not match finance.
*
*
*
* Use the single-level wildcard at any level in the topic tree, and in
* conjunction with the multilevel wildcard. Specify the single-level wildcard
* next to the topic level separator, except when it is specified on its own.
* Therefore, + and finance/+ are both valid, but
* finance+ is not valid. The single-level wildcard can be used
* at the end of the topic tree or within the topic tree. For example,
* finance/+ and finance/+/ibm are both valid.
*
*
*
*
* The method returns control before the subscribe completes. Completion can be
* tracked by:
*
*
* - Waiting on the supplied token {@link MqttToken#waitForCompletion()}
* or
* - Passing in a callback {@link MqttActionListener} to this method
*
*
* @param subscriptions
* one or more {@link MqttSubscription} defining the subscription to
* be made.
* @param userContext
* optional object used to pass context to the callback. Use null if
* not required.
* @param callback
* optional listener that will be notified when subscribe has
* completed
* @param subscriptionProperties
* The {@link MqttProperties} to be sent.
* @return token used to track and wait for the subscribe to complete. The token
* will be passed to callback methods if set.
* @throws MqttException
* if there was an error registering the subscription.
* @throws IllegalArgumentException
* if the two supplied arrays are not the same size.
*/
public IMqttToken subscribe(MqttSubscription[] subscriptions, Object userContext, MqttActionListener callback,
MqttProperties subscriptionProperties) throws MqttException;
/**
* Subscribe to a topic, which may include wildcards.
*
* @see #subscribe(MqttSubscription[], Object, MqttActionListener,
* MqttProperties)
*
* @param mqttSubscription
* a {@link MqttSubscription} defining the subscription to be made.
* @param userContext
* optional object used to pass context to the callback. Use null if
* not required.
* @param callback
* optional listener that will be notified when subscribe has
* completed
* @param messageListener
* a callback to handle incoming messages
* @param subscriptionProperties
* The {@link MqttProperties} to be sent.
* @return token used to track and wait for the subscribe to complete. The token
* will be passed to callback methods if set.
* @throws MqttException
* if there was an error registering the subscription.
*/
public IMqttToken subscribe(MqttSubscription mqttSubscription, Object userContext, MqttActionListener callback,
IMqttMessageListener messageListener, MqttProperties subscriptionProperties) throws MqttException;
/**
* Subscribe to a topic, which may include wildcards.
*
* @see #subscribe(MqttSubscription[], Object, MqttActionListener,
* MqttProperties)
*
* @param subscription
* a {@link MqttSubscription} defining the subscription to be made.
* @param messageListener
* a callback to handle incoming messages
* @return token used to track and wait for the subscribe to complete. The token
* will be passed to callback methods if set.
* @throws MqttException
* if there was an error registering the subscription.
*/
public IMqttToken subscribe(MqttSubscription subscription, IMqttMessageListener messageListener) throws MqttException;
/**
* Subscribe to multiple topics, each of which may include wildcards.
*
*
* Provides an optimized way to subscribe to multiple topics compared to
* subscribing to each one individually.
*
*
* @see #subscribe(MqttSubscription[], Object, MqttActionListener,
* MqttProperties)
*
** @param subscriptions
* one or more {@link MqttSubscription} defining the subscription to
* be made.
* @param messageListener
* a callback to handle incoming messages
* @return token used to track and wait for the subscribe to complete. The token
* will be passed to callback methods if set.
* @throws MqttException
* if there was an error registering the subscription.
*/
public IMqttToken subscribe(MqttSubscription[] subscriptions, IMqttMessageListener messageListener) throws MqttException;
/**
* Subscribe to multiple topics, each of which may include wildcards.
*
*
* Provides an optimized way to subscribe to multiple topics compared to
* subscribing to each one individually.
*
*
* @see #subscribe(MqttSubscription[], Object, MqttActionListener,
* MqttProperties)
*
* @param subscriptions
* one or more {@link MqttSubscription} defining the subscription to
* be made.
* @param userContext
* optional object used to pass context to the callback. Use null if
* not required.
* @param callback
* optional listener that will be notified when subscribe has
* completed
* @param messageListeners
* one or more callbacks to handle incoming messages.
* @param subscriptionProperties
* The {@link MqttProperties} to be sent.
* @return token used to track and wait for the subscribe to complete. The token
* will be passed to callback methods if set.
* @throws MqttException
* if there was an error registering the subscription.
*/
public IMqttToken subscribe(MqttSubscription[] subscriptions, Object userContext, MqttActionListener callback,
IMqttMessageListener[] messageListeners, MqttProperties subscriptionProperties) throws MqttException;
/**
* Subscribe to multiple topics, each of which may include wildcards.
*
*
* Provides an optimized way to subscribe to multiple topics compared to
* subscribing to each one individually.
*
*
* @see #subscribe(MqttSubscription[], Object, MqttActionListener,
* MqttProperties)
*
* @param subscriptions
* one or more {@link MqttSubscription} defining the subscription to
* be made.
* @param userContext
* optional object used to pass context to the callback. Use null if
* not required.
* @param callback
* optional listener that will be notified when subscribe has
* completed
* @param messageListener
* a callback to handle incoming messages.
* @param subscriptionProperties
* The {@link MqttProperties} to be sent.
* @return token used to track and wait for the subscribe to complete. The token
* will be passed to callback methods if set.
* @throws MqttException
* if there was an error registering the subscription.
*/
public IMqttToken subscribe(MqttSubscription[] subscriptions, Object userContext, MqttActionListener callback,
IMqttMessageListener messageListener, MqttProperties subscriptionProperties) throws MqttException;
/**
* Requests the server unsubscribe the client from a topics.
*
* @see #unsubscribe(String[], Object, MqttActionListener, MqttProperties)
*
* @param topicFilter
* the topic to unsubscribe from. It must match a topicFilter
* specified on an earlier subscribe.
* @param userContext
* optional object used to pass context to the callback. Use null if
* not required.
* @param callback
* optional listener that will be notified when unsubscribe has
* completed
* @return token used to track and wait for the unsubscribe to complete. The
* token will be passed to callback methods if set.
* @throws MqttException
* if there was an error unregistering the subscription.
*/
public IMqttToken unsubscribe(String topicFilter, Object userContext, MqttActionListener callback) throws MqttException;
/**
* Requests the server unsubscribe the client from a topic.
*
* @see #unsubscribe(String[], Object, MqttActionListener, MqttProperties)
* @param topicFilter
* the topic to unsubscribe from. It must match a topicFilter
* specified on an earlier subscribe.
* @return token used to track and wait for the unsubscribe to complete. The
* token will be passed to callback methods if set.
* @throws MqttException
* if there was an error unregistering the subscription.
*/
public IMqttToken unsubscribe(String topicFilter) throws MqttException;
/**
* Requests the server unsubscribe the client from one or more topics.
*
* @see #unsubscribe(String[], Object, MqttActionListener, MqttProperties)
*
* @param topicFilters
* one or more topics to unsubscribe from. Each topicFilter must
* match one specified on an earlier subscribe. *
* @return token used to track and wait for the unsubscribe to complete. The
* token will be passed to callback methods if set.
* @throws MqttException
* if there was an error unregistering the subscription.
*/
public IMqttToken unsubscribe(String[] topicFilters) throws MqttException;
/**
* Requests the server unsubscribe the client from one or more topics.
*
* Unsubcribing is the opposite of subscribing. When the server receives the
* unsubscribe request it looks to see if it can find a matching subscription
* for the client and then removes it. After this point the server will send no
* more messages to the client for this subscription.
*
*
* The topic(s) specified on the unsubscribe must match the topic(s) specified
* in the original subscribe request for the unsubscribe to succeed
*
*
* The method returns control before the unsubscribe completes. Completion can
* be tracked by:
*
*
*
* - Waiting on the returned token {@link MqttToken#waitForCompletion()}
* or
* - Passing in a callback {@link MqttActionListener} to this method
*
*
* @param topicFilters
* one or more topics to unsubscribe from. Each topicFilter must
* match one specified on an earlier subscribe.
* @param userContext
* optional object used to pass context to the callback. Use null if
* not required.
* @param callback
* optional listener that will be notified when unsubscribe has
* completed
* @param unsubscribeProperties
* The {@link MqttProperties} to be sent.
* @return token used to track and wait for the unsubscribe to complete. The
* token will be passed to callback methods if set.
* @throws MqttException
* if there was an error unregistering the subscription.
*/
public IMqttToken unsubscribe(String[] topicFilters, Object userContext, MqttActionListener callback,
MqttProperties unsubscribeProperties) throws MqttException;
/**
* Sets a callback listener to use for events that happen asynchronously.
*
* There are a number of events that the listener will be notified about. These
* include:
*
*
* - A new message has arrived and is ready to be processed
* - The connection to the server has been lost
* - Delivery of a message to the server has completed
*
*
* Other events that track the progress of an individual operation such as
* connect and subscribe can be tracked using the {@link MqttToken} returned
* from each non-blocking method or using setting a {@link MqttActionListener}
* on the non-blocking method.
*
*
* @see MqttCallback
* @param callback
* which will be invoked for certain asynchronous events
*/
public void setCallback(MqttCallback callback);
/**
* If manualAcks is set to true, then on completion of the messageArrived
* callback the MQTT acknowledgements are not sent. You must call
* messageArrivedComplete to send those acknowledgements. This allows finer
* control over when the acks are sent. The default behaviour, when manualAcks
* is false, is to send the MQTT acknowledgements automatically at the
* successful completion of the messageArrived callback method.
*
* @param manualAcks
* if set to true MQTT acknowledgements are not sent
*/
public void setManualAcks(boolean manualAcks);
/**
* Indicate that the application has completed processing the message with id
* messageId. This will cause the MQTT acknowledgement to be sent to the server.
*
* @param messageId
* the MQTT message id to be acknowledged
* @param qos
* the MQTT QoS of the message to be acknowledged
* @throws MqttException
* if there was a problem sending the acknowledgement
*/
public void messageArrivedComplete(int messageId, int qos) throws MqttException;
/**
* Returns the delivery tokens for any outstanding publish operations.
*
* If a client has been restarted and there are messages that were in the
* process of being delivered when the client stopped this method returns a
* token for each in-flight message enabling the delivery to be tracked
* Alternately the {@link MqttCallback#deliveryComplete(IMqttToken)}
* callback can be used to track the delivery of outstanding messages.
*
*
* If a client connects with cleanStart true then there will be no delivery
* tokens as the cleanStart option deletes all earlier state. For state to be
* remembered the client must connect with cleanStart set to false
*
*
* @return zero or more delivery tokens
*/
public IMqttToken[] getPendingTokens();
/**
* Publishes a message to a topic on the server.
*
* A convenience method, which will create a new {@link MqttMessage} object with
* a byte array payload and the specified QoS, and then publish it.
*
*
* @param topic
* to deliver the message to, for example "finance/stock/ibm".
* @param payload
* the byte array to use as the payload
* @param qos
* the Quality of Service to deliver the message at. Valid values are
* 0, 1 or 2.
* @param retained
* whether or not this message should be retained by the server.
* @param userContext
* optional object used to pass context to the callback. Use null if
* not required.
* @param callback
* optional listener that will be notified when message delivery hsa
* completed to the requested quality of service
* @return token used to track and wait for the publish to complete. The token
* will be passed to any callback that has been set.
* @throws MqttPersistenceException
* when a problem occurs storing the message
* @throws IllegalArgumentException
* if value of QoS is not 0, 1 or 2.
* @throws MqttException
* for other errors encountered while publishing the message. For
* instance client not connected.
* @see #publish(String, MqttMessage, Object, MqttActionListener)
* @see MqttMessage#setQos(int)
* @see MqttMessage#setRetained(boolean)
*/
public IMqttToken publish(String topic, byte[] payload, int qos, boolean retained, Object userContext,
MqttActionListener callback) throws MqttException, MqttPersistenceException;
/**
* Publishes a message to a topic on the server.
*
* A convenience method, which will create a new {@link MqttMessage} object with
* a byte array payload and the specified QoS, and then publish it.
*
*
* @param topic
* to deliver the message to, for example "finance/stock/ibm".
* @param payload
* the byte array to use as the payload
* @param qos
* the Quality of Service to deliver the message at. Valid values are
* 0, 1 or 2.
* @param retained
* whether or not this message should be retained by the server.
* @return token used to track and wait for the publish to complete. The token
* will be passed to any callback that has been set.
* @throws MqttPersistenceException
* when a problem occurs storing the message
* @throws IllegalArgumentException
* if value of QoS is not 0, 1 or 2.
* @throws MqttException
* for other errors encountered while publishing the message. For
* instance if too many messages are being processed.
* @see #publish(String, MqttMessage, Object, MqttActionListener)
* @see MqttMessage#setQos(int)
* @see MqttMessage#setRetained(boolean)
*/
public IMqttToken publish(String topic, byte[] payload, int qos, boolean retained)
throws MqttException, MqttPersistenceException;
/**
* Publishes a message to a topic on the server. Takes an {@link MqttMessage}
* message and delivers it to the server at the requested quality of service.
*
* @param topic
* to deliver the message to, for example "finance/stock/ibm".
* @param message
* to deliver to the server
* @return token used to track and wait for the publish to complete. The token
* will be passed to any callback that has been set.
* @throws MqttPersistenceException
* when a problem occurs storing the message
* @throws IllegalArgumentException
* if value of QoS is not 0, 1 or 2.
* @throws MqttException
* for other errors encountered while publishing the message. For
* instance client not connected.
* @see #publish(String, MqttMessage, Object, MqttActionListener)
*/
public IMqttToken publish(String topic, MqttMessage message) throws MqttException, MqttPersistenceException;
/**
* Publishes a message to a topic on the server.
*
* Once this method has returned cleanly, the message has been accepted for
* publication by the client and will be delivered on a background thread. In
* the event the connection fails or the client stops. Messages will be
* delivered to the requested quality of service once the connection is
* re-established to the server on condition that:
*
*
* - The connection is re-established with the same clientID
*
- The original connection was made with (@link
* MqttConnectOptions#setCleanStart(boolean)} set to false
*
- The connection is re-established with (@link
* MqttConnectOptions#setCleanStart(boolean)} set to false
*
- Depending when the failure occurs QoS 0 messages may not be delivered.
*
*
*
* When building an application, the design of the topic tree should take into
* account the following principles of topic name syntax and semantics:
*
*
*
* - A topic must be at least one character long.
* - Topic names are case sensitive. For example, ACCOUNTS and
* Accounts are two different topics.
* - Topic names can include the space character. For example, Accounts
* payable is a valid topic.
* - A leading "/" creates a distinct topic. For example, /finance is
* different from finance. /finance matches "+/+" and "/+",
* but not "+".
* - Do not include the null character (Unicode
*
*
* \x0000
*
*
* ) in any topic.
*
*
*
* The following principles apply to the construction and content of a topic
* tree:
*
*
* - The length is limited to 64k but within that there are no limits to the
* number of levels in a topic tree.
* - There can be any number of root nodes; that is, there can be any number
* of topic trees.
*
*
*
* The method returns control before the publish completes. Completion can be
* tracked by:
*
*
* - Setting an {@link IMqttAsyncClient#setCallback(MqttCallback)} where the
* {@link MqttCallback#deliveryComplete(IMqttToken)} method will be
* called.
* - Waiting on the returned token {@link MqttToken#waitForCompletion()}
* or
* - Passing in a callback {@link MqttActionListener} to this method
*
*
* @param topic
* to deliver the message to, for example "finance/stock/ibm".
* @param message
* to deliver to the server
* @param userContext
* optional object used to pass context to the callback. Use null if
* not required.
* @param callback
* optional listener that will be notified when message delivery has
* completed to the requested quality of service.
* @return token used to track and wait for the publish to complete. The token
* will be passed to callback methods if set.
* @throws MqttPersistenceException
* when a problem occurs storing the message
* @throws IllegalArgumentException
* if value of QoS is not 0, 1 or 2.
* @throws MqttException
* for other errors encountered while publishing the message. For
* instance client not connected.
* @see MqttMessage
*/
public IMqttToken publish(String topic, MqttMessage message, Object userContext, MqttActionListener callback)
throws MqttException, MqttPersistenceException;
/**
* An AUTH Packet is sent from Client to Server or Server to Client as part of
* an extended authentication exchange, such as challenge / response
* authentication. It is a protocol error for the Client or Server to send an
* AUTH packet if the CONNECT packet did not contain the same Authentication
* Method.
*
* @param reasonCode
* The Reason code, can be Success (0), Continue authentication (24)
* or Re-authenticate (25).
* @param userContext
* optional object used to pass context to the callback. Use null if
* not required.
* @param properties
* The {@link MqttProperties} to be sent, containing the
* Authentication Method, Authentication Data and any required User
* Defined Properties.
* @return token used to track and wait for the authentication to complete.
* @throws MqttException if an exception occurs whilst sending the authenticate packet.
*/
public IMqttToken authenticate(int reasonCode, Object userContext, MqttProperties properties) throws MqttException;
/**
* User triggered attempt to reconnect
*
* @throws MqttException
* if there is an issue with reconnecting
*/
public void reconnect() throws MqttException;
/**
* Sets the DisconnectedBufferOptions for this client
*
* @param bufferOpts
* the {@link DisconnectedBufferOptions}
*/
public void setBufferOpts(DisconnectedBufferOptions bufferOpts);
/**
* Returns the number of messages in the Disconnected Message Buffer
*
* @return Count of messages in the buffer
*/
public int getBufferedMessageCount();
/**
* Returns a message from the Disconnected Message Buffer
*
* @param bufferIndex
* the index of the message to be retrieved.
* @return the message located at the bufferIndex
*/
public MqttMessage getBufferedMessage(int bufferIndex);
/**
* Deletes a message from the Disconnected Message Buffer
*
* @param bufferIndex
* the index of the message to be deleted.
*/
public void deleteBufferedMessage(int bufferIndex);
/**
* Returns the current number of outgoing in-flight messages being sent by the
* client. Note that this number cannot be guaranteed to be 100% accurate as
* some messages may have been sent or queued in the time taken for this method
* to return.
*
* @return the current number of in-flight messages.
*/
public int getInFlightMessageCount();
/**
* Close the client Releases all resource associated with the client. After the
* client has been closed it cannot be reused. For instance attempts to connect
* will fail.
*
* @throws MqttException
* if the client is not disconnected.
*/
public void close() throws MqttException;
/**
* Close the client Releases all resource associated with the client. After the
* client has been closed it cannot be reused. For instance attempts to connect
* will fail.
*
* @param force
* - Will force the connection to close.
*
* @throws MqttException
* if the client is not disconnected.
*/
public void close(boolean force) throws MqttException;
/**
* Return a debug object that can be used to help solve problems.
*
* @return the {@link Debug} object
*/
public Debug getDebug();
public IMqttToken subscribe(MqttSubscription subscription) throws MqttException;
}