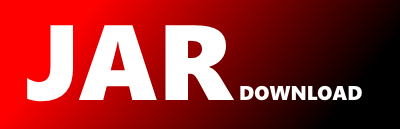
ucar.nc2.ft.point.remote.PointStreamProto Maven / Gradle / Ivy
The newest version!
// Generated by the protocol buffer compiler. DO NOT EDIT!
// source: pointStream.proto
package ucar.nc2.ft.point.remote;
public final class PointStreamProto {
private PointStreamProto() {}
public static void registerAllExtensions(
com.google.protobuf.ExtensionRegistryLite registry) {
}
public static void registerAllExtensions(
com.google.protobuf.ExtensionRegistry registry) {
registerAllExtensions(
(com.google.protobuf.ExtensionRegistryLite) registry);
}
public interface LocationOrBuilder extends
// @@protoc_insertion_point(interface_extends:Location)
com.google.protobuf.MessageOrBuilder {
/**
* double time = 1;
* @return The time.
*/
double getTime();
/**
* double lat = 2;
* @return The lat.
*/
double getLat();
/**
* double lon = 3;
* @return The lon.
*/
double getLon();
/**
* double alt = 4;
* @return The alt.
*/
double getAlt();
/**
* double nomTime = 5;
* @return The nomTime.
*/
double getNomTime();
}
/**
* Protobuf type {@code Location}
*/
public static final class Location extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:Location)
LocationOrBuilder {
private static final long serialVersionUID = 0L;
// Use Location.newBuilder() to construct.
private Location(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private Location() {
}
@java.lang.Override
@SuppressWarnings({"unused"})
protected java.lang.Object newInstance(
UnusedPrivateParameter unused) {
return new Location();
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return ucar.nc2.ft.point.remote.PointStreamProto.internal_static_Location_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return ucar.nc2.ft.point.remote.PointStreamProto.internal_static_Location_fieldAccessorTable
.ensureFieldAccessorsInitialized(
ucar.nc2.ft.point.remote.PointStreamProto.Location.class, ucar.nc2.ft.point.remote.PointStreamProto.Location.Builder.class);
}
public static final int TIME_FIELD_NUMBER = 1;
private double time_ = 0D;
/**
* double time = 1;
* @return The time.
*/
@java.lang.Override
public double getTime() {
return time_;
}
public static final int LAT_FIELD_NUMBER = 2;
private double lat_ = 0D;
/**
* double lat = 2;
* @return The lat.
*/
@java.lang.Override
public double getLat() {
return lat_;
}
public static final int LON_FIELD_NUMBER = 3;
private double lon_ = 0D;
/**
* double lon = 3;
* @return The lon.
*/
@java.lang.Override
public double getLon() {
return lon_;
}
public static final int ALT_FIELD_NUMBER = 4;
private double alt_ = 0D;
/**
* double alt = 4;
* @return The alt.
*/
@java.lang.Override
public double getAlt() {
return alt_;
}
public static final int NOMTIME_FIELD_NUMBER = 5;
private double nomTime_ = 0D;
/**
* double nomTime = 5;
* @return The nomTime.
*/
@java.lang.Override
public double getNomTime() {
return nomTime_;
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (java.lang.Double.doubleToRawLongBits(time_) != 0) {
output.writeDouble(1, time_);
}
if (java.lang.Double.doubleToRawLongBits(lat_) != 0) {
output.writeDouble(2, lat_);
}
if (java.lang.Double.doubleToRawLongBits(lon_) != 0) {
output.writeDouble(3, lon_);
}
if (java.lang.Double.doubleToRawLongBits(alt_) != 0) {
output.writeDouble(4, alt_);
}
if (java.lang.Double.doubleToRawLongBits(nomTime_) != 0) {
output.writeDouble(5, nomTime_);
}
getUnknownFields().writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (java.lang.Double.doubleToRawLongBits(time_) != 0) {
size += com.google.protobuf.CodedOutputStream
.computeDoubleSize(1, time_);
}
if (java.lang.Double.doubleToRawLongBits(lat_) != 0) {
size += com.google.protobuf.CodedOutputStream
.computeDoubleSize(2, lat_);
}
if (java.lang.Double.doubleToRawLongBits(lon_) != 0) {
size += com.google.protobuf.CodedOutputStream
.computeDoubleSize(3, lon_);
}
if (java.lang.Double.doubleToRawLongBits(alt_) != 0) {
size += com.google.protobuf.CodedOutputStream
.computeDoubleSize(4, alt_);
}
if (java.lang.Double.doubleToRawLongBits(nomTime_) != 0) {
size += com.google.protobuf.CodedOutputStream
.computeDoubleSize(5, nomTime_);
}
size += getUnknownFields().getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof ucar.nc2.ft.point.remote.PointStreamProto.Location)) {
return super.equals(obj);
}
ucar.nc2.ft.point.remote.PointStreamProto.Location other = (ucar.nc2.ft.point.remote.PointStreamProto.Location) obj;
if (java.lang.Double.doubleToLongBits(getTime())
!= java.lang.Double.doubleToLongBits(
other.getTime())) return false;
if (java.lang.Double.doubleToLongBits(getLat())
!= java.lang.Double.doubleToLongBits(
other.getLat())) return false;
if (java.lang.Double.doubleToLongBits(getLon())
!= java.lang.Double.doubleToLongBits(
other.getLon())) return false;
if (java.lang.Double.doubleToLongBits(getAlt())
!= java.lang.Double.doubleToLongBits(
other.getAlt())) return false;
if (java.lang.Double.doubleToLongBits(getNomTime())
!= java.lang.Double.doubleToLongBits(
other.getNomTime())) return false;
if (!getUnknownFields().equals(other.getUnknownFields())) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
hash = (37 * hash) + TIME_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(
java.lang.Double.doubleToLongBits(getTime()));
hash = (37 * hash) + LAT_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(
java.lang.Double.doubleToLongBits(getLat()));
hash = (37 * hash) + LON_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(
java.lang.Double.doubleToLongBits(getLon()));
hash = (37 * hash) + ALT_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(
java.lang.Double.doubleToLongBits(getAlt()));
hash = (37 * hash) + NOMTIME_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(
java.lang.Double.doubleToLongBits(getNomTime()));
hash = (29 * hash) + getUnknownFields().hashCode();
memoizedHashCode = hash;
return hash;
}
public static ucar.nc2.ft.point.remote.PointStreamProto.Location parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static ucar.nc2.ft.point.remote.PointStreamProto.Location parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static ucar.nc2.ft.point.remote.PointStreamProto.Location parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static ucar.nc2.ft.point.remote.PointStreamProto.Location parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static ucar.nc2.ft.point.remote.PointStreamProto.Location parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static ucar.nc2.ft.point.remote.PointStreamProto.Location parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static ucar.nc2.ft.point.remote.PointStreamProto.Location parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static ucar.nc2.ft.point.remote.PointStreamProto.Location parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static ucar.nc2.ft.point.remote.PointStreamProto.Location parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static ucar.nc2.ft.point.remote.PointStreamProto.Location parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static ucar.nc2.ft.point.remote.PointStreamProto.Location parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static ucar.nc2.ft.point.remote.PointStreamProto.Location parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(ucar.nc2.ft.point.remote.PointStreamProto.Location prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code Location}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:Location)
ucar.nc2.ft.point.remote.PointStreamProto.LocationOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return ucar.nc2.ft.point.remote.PointStreamProto.internal_static_Location_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return ucar.nc2.ft.point.remote.PointStreamProto.internal_static_Location_fieldAccessorTable
.ensureFieldAccessorsInitialized(
ucar.nc2.ft.point.remote.PointStreamProto.Location.class, ucar.nc2.ft.point.remote.PointStreamProto.Location.Builder.class);
}
// Construct using ucar.nc2.ft.point.remote.PointStreamProto.Location.newBuilder()
private Builder() {
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
}
@java.lang.Override
public Builder clear() {
super.clear();
bitField0_ = 0;
time_ = 0D;
lat_ = 0D;
lon_ = 0D;
alt_ = 0D;
nomTime_ = 0D;
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return ucar.nc2.ft.point.remote.PointStreamProto.internal_static_Location_descriptor;
}
@java.lang.Override
public ucar.nc2.ft.point.remote.PointStreamProto.Location getDefaultInstanceForType() {
return ucar.nc2.ft.point.remote.PointStreamProto.Location.getDefaultInstance();
}
@java.lang.Override
public ucar.nc2.ft.point.remote.PointStreamProto.Location build() {
ucar.nc2.ft.point.remote.PointStreamProto.Location result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public ucar.nc2.ft.point.remote.PointStreamProto.Location buildPartial() {
ucar.nc2.ft.point.remote.PointStreamProto.Location result = new ucar.nc2.ft.point.remote.PointStreamProto.Location(this);
if (bitField0_ != 0) { buildPartial0(result); }
onBuilt();
return result;
}
private void buildPartial0(ucar.nc2.ft.point.remote.PointStreamProto.Location result) {
int from_bitField0_ = bitField0_;
if (((from_bitField0_ & 0x00000001) != 0)) {
result.time_ = time_;
}
if (((from_bitField0_ & 0x00000002) != 0)) {
result.lat_ = lat_;
}
if (((from_bitField0_ & 0x00000004) != 0)) {
result.lon_ = lon_;
}
if (((from_bitField0_ & 0x00000008) != 0)) {
result.alt_ = alt_;
}
if (((from_bitField0_ & 0x00000010) != 0)) {
result.nomTime_ = nomTime_;
}
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof ucar.nc2.ft.point.remote.PointStreamProto.Location) {
return mergeFrom((ucar.nc2.ft.point.remote.PointStreamProto.Location)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(ucar.nc2.ft.point.remote.PointStreamProto.Location other) {
if (other == ucar.nc2.ft.point.remote.PointStreamProto.Location.getDefaultInstance()) return this;
if (other.getTime() != 0D) {
setTime(other.getTime());
}
if (other.getLat() != 0D) {
setLat(other.getLat());
}
if (other.getLon() != 0D) {
setLon(other.getLon());
}
if (other.getAlt() != 0D) {
setAlt(other.getAlt());
}
if (other.getNomTime() != 0D) {
setNomTime(other.getNomTime());
}
this.mergeUnknownFields(other.getUnknownFields());
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 9: {
time_ = input.readDouble();
bitField0_ |= 0x00000001;
break;
} // case 9
case 17: {
lat_ = input.readDouble();
bitField0_ |= 0x00000002;
break;
} // case 17
case 25: {
lon_ = input.readDouble();
bitField0_ |= 0x00000004;
break;
} // case 25
case 33: {
alt_ = input.readDouble();
bitField0_ |= 0x00000008;
break;
} // case 33
case 41: {
nomTime_ = input.readDouble();
bitField0_ |= 0x00000010;
break;
} // case 41
default: {
if (!super.parseUnknownField(input, extensionRegistry, tag)) {
done = true; // was an endgroup tag
}
break;
} // default:
} // switch (tag)
} // while (!done)
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.unwrapIOException();
} finally {
onChanged();
} // finally
return this;
}
private int bitField0_;
private double time_ ;
/**
* double time = 1;
* @return The time.
*/
@java.lang.Override
public double getTime() {
return time_;
}
/**
* double time = 1;
* @param value The time to set.
* @return This builder for chaining.
*/
public Builder setTime(double value) {
time_ = value;
bitField0_ |= 0x00000001;
onChanged();
return this;
}
/**
* double time = 1;
* @return This builder for chaining.
*/
public Builder clearTime() {
bitField0_ = (bitField0_ & ~0x00000001);
time_ = 0D;
onChanged();
return this;
}
private double lat_ ;
/**
* double lat = 2;
* @return The lat.
*/
@java.lang.Override
public double getLat() {
return lat_;
}
/**
* double lat = 2;
* @param value The lat to set.
* @return This builder for chaining.
*/
public Builder setLat(double value) {
lat_ = value;
bitField0_ |= 0x00000002;
onChanged();
return this;
}
/**
* double lat = 2;
* @return This builder for chaining.
*/
public Builder clearLat() {
bitField0_ = (bitField0_ & ~0x00000002);
lat_ = 0D;
onChanged();
return this;
}
private double lon_ ;
/**
* double lon = 3;
* @return The lon.
*/
@java.lang.Override
public double getLon() {
return lon_;
}
/**
* double lon = 3;
* @param value The lon to set.
* @return This builder for chaining.
*/
public Builder setLon(double value) {
lon_ = value;
bitField0_ |= 0x00000004;
onChanged();
return this;
}
/**
* double lon = 3;
* @return This builder for chaining.
*/
public Builder clearLon() {
bitField0_ = (bitField0_ & ~0x00000004);
lon_ = 0D;
onChanged();
return this;
}
private double alt_ ;
/**
* double alt = 4;
* @return The alt.
*/
@java.lang.Override
public double getAlt() {
return alt_;
}
/**
* double alt = 4;
* @param value The alt to set.
* @return This builder for chaining.
*/
public Builder setAlt(double value) {
alt_ = value;
bitField0_ |= 0x00000008;
onChanged();
return this;
}
/**
* double alt = 4;
* @return This builder for chaining.
*/
public Builder clearAlt() {
bitField0_ = (bitField0_ & ~0x00000008);
alt_ = 0D;
onChanged();
return this;
}
private double nomTime_ ;
/**
* double nomTime = 5;
* @return The nomTime.
*/
@java.lang.Override
public double getNomTime() {
return nomTime_;
}
/**
* double nomTime = 5;
* @param value The nomTime to set.
* @return This builder for chaining.
*/
public Builder setNomTime(double value) {
nomTime_ = value;
bitField0_ |= 0x00000010;
onChanged();
return this;
}
/**
* double nomTime = 5;
* @return This builder for chaining.
*/
public Builder clearNomTime() {
bitField0_ = (bitField0_ & ~0x00000010);
nomTime_ = 0D;
onChanged();
return this;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:Location)
}
// @@protoc_insertion_point(class_scope:Location)
private static final ucar.nc2.ft.point.remote.PointStreamProto.Location DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new ucar.nc2.ft.point.remote.PointStreamProto.Location();
}
public static ucar.nc2.ft.point.remote.PointStreamProto.Location getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public Location parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
Builder builder = newBuilder();
try {
builder.mergeFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(builder.buildPartial());
} catch (com.google.protobuf.UninitializedMessageException e) {
throw e.asInvalidProtocolBufferException().setUnfinishedMessage(builder.buildPartial());
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(e)
.setUnfinishedMessage(builder.buildPartial());
}
return builder.buildPartial();
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public ucar.nc2.ft.point.remote.PointStreamProto.Location getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface PointFeatureOrBuilder extends
// @@protoc_insertion_point(interface_extends:PointFeature)
com.google.protobuf.MessageOrBuilder {
/**
* .Location loc = 1;
* @return Whether the loc field is set.
*/
boolean hasLoc();
/**
* .Location loc = 1;
* @return The loc.
*/
ucar.nc2.ft.point.remote.PointStreamProto.Location getLoc();
/**
* .Location loc = 1;
*/
ucar.nc2.ft.point.remote.PointStreamProto.LocationOrBuilder getLocOrBuilder();
/**
*
* fixed length data
*
*
* bytes data = 3;
* @return The data.
*/
com.google.protobuf.ByteString getData();
/**
*
* Strings
*
*
* repeated string sdata = 4;
* @return A list containing the sdata.
*/
java.util.List
getSdataList();
/**
*
* Strings
*
*
* repeated string sdata = 4;
* @return The count of sdata.
*/
int getSdataCount();
/**
*
* Strings
*
*
* repeated string sdata = 4;
* @param index The index of the element to return.
* @return The sdata at the given index.
*/
java.lang.String getSdata(int index);
/**
*
* Strings
*
*
* repeated string sdata = 4;
* @param index The index of the value to return.
* @return The bytes of the sdata at the given index.
*/
com.google.protobuf.ByteString
getSdataBytes(int index);
}
/**
* Protobuf type {@code PointFeature}
*/
public static final class PointFeature extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:PointFeature)
PointFeatureOrBuilder {
private static final long serialVersionUID = 0L;
// Use PointFeature.newBuilder() to construct.
private PointFeature(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private PointFeature() {
data_ = com.google.protobuf.ByteString.EMPTY;
sdata_ =
com.google.protobuf.LazyStringArrayList.emptyList();
}
@java.lang.Override
@SuppressWarnings({"unused"})
protected java.lang.Object newInstance(
UnusedPrivateParameter unused) {
return new PointFeature();
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return ucar.nc2.ft.point.remote.PointStreamProto.internal_static_PointFeature_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return ucar.nc2.ft.point.remote.PointStreamProto.internal_static_PointFeature_fieldAccessorTable
.ensureFieldAccessorsInitialized(
ucar.nc2.ft.point.remote.PointStreamProto.PointFeature.class, ucar.nc2.ft.point.remote.PointStreamProto.PointFeature.Builder.class);
}
public static final int LOC_FIELD_NUMBER = 1;
private ucar.nc2.ft.point.remote.PointStreamProto.Location loc_;
/**
* .Location loc = 1;
* @return Whether the loc field is set.
*/
@java.lang.Override
public boolean hasLoc() {
return loc_ != null;
}
/**
* .Location loc = 1;
* @return The loc.
*/
@java.lang.Override
public ucar.nc2.ft.point.remote.PointStreamProto.Location getLoc() {
return loc_ == null ? ucar.nc2.ft.point.remote.PointStreamProto.Location.getDefaultInstance() : loc_;
}
/**
* .Location loc = 1;
*/
@java.lang.Override
public ucar.nc2.ft.point.remote.PointStreamProto.LocationOrBuilder getLocOrBuilder() {
return loc_ == null ? ucar.nc2.ft.point.remote.PointStreamProto.Location.getDefaultInstance() : loc_;
}
public static final int DATA_FIELD_NUMBER = 3;
private com.google.protobuf.ByteString data_ = com.google.protobuf.ByteString.EMPTY;
/**
*
* fixed length data
*
*
* bytes data = 3;
* @return The data.
*/
@java.lang.Override
public com.google.protobuf.ByteString getData() {
return data_;
}
public static final int SDATA_FIELD_NUMBER = 4;
@SuppressWarnings("serial")
private com.google.protobuf.LazyStringArrayList sdata_ =
com.google.protobuf.LazyStringArrayList.emptyList();
/**
*
* Strings
*
*
* repeated string sdata = 4;
* @return A list containing the sdata.
*/
public com.google.protobuf.ProtocolStringList
getSdataList() {
return sdata_;
}
/**
*
* Strings
*
*
* repeated string sdata = 4;
* @return The count of sdata.
*/
public int getSdataCount() {
return sdata_.size();
}
/**
*
* Strings
*
*
* repeated string sdata = 4;
* @param index The index of the element to return.
* @return The sdata at the given index.
*/
public java.lang.String getSdata(int index) {
return sdata_.get(index);
}
/**
*
* Strings
*
*
* repeated string sdata = 4;
* @param index The index of the value to return.
* @return The bytes of the sdata at the given index.
*/
public com.google.protobuf.ByteString
getSdataBytes(int index) {
return sdata_.getByteString(index);
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (loc_ != null) {
output.writeMessage(1, getLoc());
}
if (!data_.isEmpty()) {
output.writeBytes(3, data_);
}
for (int i = 0; i < sdata_.size(); i++) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 4, sdata_.getRaw(i));
}
getUnknownFields().writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (loc_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(1, getLoc());
}
if (!data_.isEmpty()) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(3, data_);
}
{
int dataSize = 0;
for (int i = 0; i < sdata_.size(); i++) {
dataSize += computeStringSizeNoTag(sdata_.getRaw(i));
}
size += dataSize;
size += 1 * getSdataList().size();
}
size += getUnknownFields().getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof ucar.nc2.ft.point.remote.PointStreamProto.PointFeature)) {
return super.equals(obj);
}
ucar.nc2.ft.point.remote.PointStreamProto.PointFeature other = (ucar.nc2.ft.point.remote.PointStreamProto.PointFeature) obj;
if (hasLoc() != other.hasLoc()) return false;
if (hasLoc()) {
if (!getLoc()
.equals(other.getLoc())) return false;
}
if (!getData()
.equals(other.getData())) return false;
if (!getSdataList()
.equals(other.getSdataList())) return false;
if (!getUnknownFields().equals(other.getUnknownFields())) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
if (hasLoc()) {
hash = (37 * hash) + LOC_FIELD_NUMBER;
hash = (53 * hash) + getLoc().hashCode();
}
hash = (37 * hash) + DATA_FIELD_NUMBER;
hash = (53 * hash) + getData().hashCode();
if (getSdataCount() > 0) {
hash = (37 * hash) + SDATA_FIELD_NUMBER;
hash = (53 * hash) + getSdataList().hashCode();
}
hash = (29 * hash) + getUnknownFields().hashCode();
memoizedHashCode = hash;
return hash;
}
public static ucar.nc2.ft.point.remote.PointStreamProto.PointFeature parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static ucar.nc2.ft.point.remote.PointStreamProto.PointFeature parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static ucar.nc2.ft.point.remote.PointStreamProto.PointFeature parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static ucar.nc2.ft.point.remote.PointStreamProto.PointFeature parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static ucar.nc2.ft.point.remote.PointStreamProto.PointFeature parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static ucar.nc2.ft.point.remote.PointStreamProto.PointFeature parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static ucar.nc2.ft.point.remote.PointStreamProto.PointFeature parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static ucar.nc2.ft.point.remote.PointStreamProto.PointFeature parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static ucar.nc2.ft.point.remote.PointStreamProto.PointFeature parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static ucar.nc2.ft.point.remote.PointStreamProto.PointFeature parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static ucar.nc2.ft.point.remote.PointStreamProto.PointFeature parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static ucar.nc2.ft.point.remote.PointStreamProto.PointFeature parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(ucar.nc2.ft.point.remote.PointStreamProto.PointFeature prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code PointFeature}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:PointFeature)
ucar.nc2.ft.point.remote.PointStreamProto.PointFeatureOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return ucar.nc2.ft.point.remote.PointStreamProto.internal_static_PointFeature_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return ucar.nc2.ft.point.remote.PointStreamProto.internal_static_PointFeature_fieldAccessorTable
.ensureFieldAccessorsInitialized(
ucar.nc2.ft.point.remote.PointStreamProto.PointFeature.class, ucar.nc2.ft.point.remote.PointStreamProto.PointFeature.Builder.class);
}
// Construct using ucar.nc2.ft.point.remote.PointStreamProto.PointFeature.newBuilder()
private Builder() {
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
}
@java.lang.Override
public Builder clear() {
super.clear();
bitField0_ = 0;
loc_ = null;
if (locBuilder_ != null) {
locBuilder_.dispose();
locBuilder_ = null;
}
data_ = com.google.protobuf.ByteString.EMPTY;
sdata_ =
com.google.protobuf.LazyStringArrayList.emptyList();
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return ucar.nc2.ft.point.remote.PointStreamProto.internal_static_PointFeature_descriptor;
}
@java.lang.Override
public ucar.nc2.ft.point.remote.PointStreamProto.PointFeature getDefaultInstanceForType() {
return ucar.nc2.ft.point.remote.PointStreamProto.PointFeature.getDefaultInstance();
}
@java.lang.Override
public ucar.nc2.ft.point.remote.PointStreamProto.PointFeature build() {
ucar.nc2.ft.point.remote.PointStreamProto.PointFeature result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public ucar.nc2.ft.point.remote.PointStreamProto.PointFeature buildPartial() {
ucar.nc2.ft.point.remote.PointStreamProto.PointFeature result = new ucar.nc2.ft.point.remote.PointStreamProto.PointFeature(this);
if (bitField0_ != 0) { buildPartial0(result); }
onBuilt();
return result;
}
private void buildPartial0(ucar.nc2.ft.point.remote.PointStreamProto.PointFeature result) {
int from_bitField0_ = bitField0_;
if (((from_bitField0_ & 0x00000001) != 0)) {
result.loc_ = locBuilder_ == null
? loc_
: locBuilder_.build();
}
if (((from_bitField0_ & 0x00000002) != 0)) {
result.data_ = data_;
}
if (((from_bitField0_ & 0x00000004) != 0)) {
sdata_.makeImmutable();
result.sdata_ = sdata_;
}
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof ucar.nc2.ft.point.remote.PointStreamProto.PointFeature) {
return mergeFrom((ucar.nc2.ft.point.remote.PointStreamProto.PointFeature)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(ucar.nc2.ft.point.remote.PointStreamProto.PointFeature other) {
if (other == ucar.nc2.ft.point.remote.PointStreamProto.PointFeature.getDefaultInstance()) return this;
if (other.hasLoc()) {
mergeLoc(other.getLoc());
}
if (other.getData() != com.google.protobuf.ByteString.EMPTY) {
setData(other.getData());
}
if (!other.sdata_.isEmpty()) {
if (sdata_.isEmpty()) {
sdata_ = other.sdata_;
bitField0_ |= 0x00000004;
} else {
ensureSdataIsMutable();
sdata_.addAll(other.sdata_);
}
onChanged();
}
this.mergeUnknownFields(other.getUnknownFields());
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 10: {
input.readMessage(
getLocFieldBuilder().getBuilder(),
extensionRegistry);
bitField0_ |= 0x00000001;
break;
} // case 10
case 26: {
data_ = input.readBytes();
bitField0_ |= 0x00000002;
break;
} // case 26
case 34: {
java.lang.String s = input.readStringRequireUtf8();
ensureSdataIsMutable();
sdata_.add(s);
break;
} // case 34
default: {
if (!super.parseUnknownField(input, extensionRegistry, tag)) {
done = true; // was an endgroup tag
}
break;
} // default:
} // switch (tag)
} // while (!done)
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.unwrapIOException();
} finally {
onChanged();
} // finally
return this;
}
private int bitField0_;
private ucar.nc2.ft.point.remote.PointStreamProto.Location loc_;
private com.google.protobuf.SingleFieldBuilderV3<
ucar.nc2.ft.point.remote.PointStreamProto.Location, ucar.nc2.ft.point.remote.PointStreamProto.Location.Builder, ucar.nc2.ft.point.remote.PointStreamProto.LocationOrBuilder> locBuilder_;
/**
* .Location loc = 1;
* @return Whether the loc field is set.
*/
public boolean hasLoc() {
return ((bitField0_ & 0x00000001) != 0);
}
/**
* .Location loc = 1;
* @return The loc.
*/
public ucar.nc2.ft.point.remote.PointStreamProto.Location getLoc() {
if (locBuilder_ == null) {
return loc_ == null ? ucar.nc2.ft.point.remote.PointStreamProto.Location.getDefaultInstance() : loc_;
} else {
return locBuilder_.getMessage();
}
}
/**
* .Location loc = 1;
*/
public Builder setLoc(ucar.nc2.ft.point.remote.PointStreamProto.Location value) {
if (locBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
loc_ = value;
} else {
locBuilder_.setMessage(value);
}
bitField0_ |= 0x00000001;
onChanged();
return this;
}
/**
* .Location loc = 1;
*/
public Builder setLoc(
ucar.nc2.ft.point.remote.PointStreamProto.Location.Builder builderForValue) {
if (locBuilder_ == null) {
loc_ = builderForValue.build();
} else {
locBuilder_.setMessage(builderForValue.build());
}
bitField0_ |= 0x00000001;
onChanged();
return this;
}
/**
* .Location loc = 1;
*/
public Builder mergeLoc(ucar.nc2.ft.point.remote.PointStreamProto.Location value) {
if (locBuilder_ == null) {
if (((bitField0_ & 0x00000001) != 0) &&
loc_ != null &&
loc_ != ucar.nc2.ft.point.remote.PointStreamProto.Location.getDefaultInstance()) {
getLocBuilder().mergeFrom(value);
} else {
loc_ = value;
}
} else {
locBuilder_.mergeFrom(value);
}
bitField0_ |= 0x00000001;
onChanged();
return this;
}
/**
* .Location loc = 1;
*/
public Builder clearLoc() {
bitField0_ = (bitField0_ & ~0x00000001);
loc_ = null;
if (locBuilder_ != null) {
locBuilder_.dispose();
locBuilder_ = null;
}
onChanged();
return this;
}
/**
* .Location loc = 1;
*/
public ucar.nc2.ft.point.remote.PointStreamProto.Location.Builder getLocBuilder() {
bitField0_ |= 0x00000001;
onChanged();
return getLocFieldBuilder().getBuilder();
}
/**
* .Location loc = 1;
*/
public ucar.nc2.ft.point.remote.PointStreamProto.LocationOrBuilder getLocOrBuilder() {
if (locBuilder_ != null) {
return locBuilder_.getMessageOrBuilder();
} else {
return loc_ == null ?
ucar.nc2.ft.point.remote.PointStreamProto.Location.getDefaultInstance() : loc_;
}
}
/**
* .Location loc = 1;
*/
private com.google.protobuf.SingleFieldBuilderV3<
ucar.nc2.ft.point.remote.PointStreamProto.Location, ucar.nc2.ft.point.remote.PointStreamProto.Location.Builder, ucar.nc2.ft.point.remote.PointStreamProto.LocationOrBuilder>
getLocFieldBuilder() {
if (locBuilder_ == null) {
locBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
ucar.nc2.ft.point.remote.PointStreamProto.Location, ucar.nc2.ft.point.remote.PointStreamProto.Location.Builder, ucar.nc2.ft.point.remote.PointStreamProto.LocationOrBuilder>(
getLoc(),
getParentForChildren(),
isClean());
loc_ = null;
}
return locBuilder_;
}
private com.google.protobuf.ByteString data_ = com.google.protobuf.ByteString.EMPTY;
/**
*
* fixed length data
*
*
* bytes data = 3;
* @return The data.
*/
@java.lang.Override
public com.google.protobuf.ByteString getData() {
return data_;
}
/**
*
* fixed length data
*
*
* bytes data = 3;
* @param value The data to set.
* @return This builder for chaining.
*/
public Builder setData(com.google.protobuf.ByteString value) {
if (value == null) { throw new NullPointerException(); }
data_ = value;
bitField0_ |= 0x00000002;
onChanged();
return this;
}
/**
*
* fixed length data
*
*
* bytes data = 3;
* @return This builder for chaining.
*/
public Builder clearData() {
bitField0_ = (bitField0_ & ~0x00000002);
data_ = getDefaultInstance().getData();
onChanged();
return this;
}
private com.google.protobuf.LazyStringArrayList sdata_ =
com.google.protobuf.LazyStringArrayList.emptyList();
private void ensureSdataIsMutable() {
if (!sdata_.isModifiable()) {
sdata_ = new com.google.protobuf.LazyStringArrayList(sdata_);
}
bitField0_ |= 0x00000004;
}
/**
*
* Strings
*
*
* repeated string sdata = 4;
* @return A list containing the sdata.
*/
public com.google.protobuf.ProtocolStringList
getSdataList() {
sdata_.makeImmutable();
return sdata_;
}
/**
*
* Strings
*
*
* repeated string sdata = 4;
* @return The count of sdata.
*/
public int getSdataCount() {
return sdata_.size();
}
/**
*
* Strings
*
*
* repeated string sdata = 4;
* @param index The index of the element to return.
* @return The sdata at the given index.
*/
public java.lang.String getSdata(int index) {
return sdata_.get(index);
}
/**
*
* Strings
*
*
* repeated string sdata = 4;
* @param index The index of the value to return.
* @return The bytes of the sdata at the given index.
*/
public com.google.protobuf.ByteString
getSdataBytes(int index) {
return sdata_.getByteString(index);
}
/**
*
* Strings
*
*
* repeated string sdata = 4;
* @param index The index to set the value at.
* @param value The sdata to set.
* @return This builder for chaining.
*/
public Builder setSdata(
int index, java.lang.String value) {
if (value == null) { throw new NullPointerException(); }
ensureSdataIsMutable();
sdata_.set(index, value);
bitField0_ |= 0x00000004;
onChanged();
return this;
}
/**
*
* Strings
*
*
* repeated string sdata = 4;
* @param value The sdata to add.
* @return This builder for chaining.
*/
public Builder addSdata(
java.lang.String value) {
if (value == null) { throw new NullPointerException(); }
ensureSdataIsMutable();
sdata_.add(value);
bitField0_ |= 0x00000004;
onChanged();
return this;
}
/**
*
* Strings
*
*
* repeated string sdata = 4;
* @param values The sdata to add.
* @return This builder for chaining.
*/
public Builder addAllSdata(
java.lang.Iterable values) {
ensureSdataIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(
values, sdata_);
bitField0_ |= 0x00000004;
onChanged();
return this;
}
/**
*
* Strings
*
*
* repeated string sdata = 4;
* @return This builder for chaining.
*/
public Builder clearSdata() {
sdata_ =
com.google.protobuf.LazyStringArrayList.emptyList();
bitField0_ = (bitField0_ & ~0x00000004);;
onChanged();
return this;
}
/**
*
* Strings
*
*
* repeated string sdata = 4;
* @param value The bytes of the sdata to add.
* @return This builder for chaining.
*/
public Builder addSdataBytes(
com.google.protobuf.ByteString value) {
if (value == null) { throw new NullPointerException(); }
checkByteStringIsUtf8(value);
ensureSdataIsMutable();
sdata_.add(value);
bitField0_ |= 0x00000004;
onChanged();
return this;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:PointFeature)
}
// @@protoc_insertion_point(class_scope:PointFeature)
private static final ucar.nc2.ft.point.remote.PointStreamProto.PointFeature DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new ucar.nc2.ft.point.remote.PointStreamProto.PointFeature();
}
public static ucar.nc2.ft.point.remote.PointStreamProto.PointFeature getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public PointFeature parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
Builder builder = newBuilder();
try {
builder.mergeFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(builder.buildPartial());
} catch (com.google.protobuf.UninitializedMessageException e) {
throw e.asInvalidProtocolBufferException().setUnfinishedMessage(builder.buildPartial());
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(e)
.setUnfinishedMessage(builder.buildPartial());
}
return builder.buildPartial();
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public ucar.nc2.ft.point.remote.PointStreamProto.PointFeature getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface PointFeatureMemberOrBuilder extends
// @@protoc_insertion_point(interface_extends:PointFeatureMember)
com.google.protobuf.MessageOrBuilder {
/**
* string name = 1;
* @return The name.
*/
java.lang.String getName();
/**
* string name = 1;
* @return The bytes for name.
*/
com.google.protobuf.ByteString
getNameBytes();
/**
* string desc = 2;
* @return The desc.
*/
java.lang.String getDesc();
/**
* string desc = 2;
* @return The bytes for desc.
*/
com.google.protobuf.ByteString
getDescBytes();
/**
* string units = 3;
* @return The units.
*/
java.lang.String getUnits();
/**
* string units = 3;
* @return The bytes for units.
*/
com.google.protobuf.ByteString
getUnitsBytes();
/**
* .DataType dataType = 4;
* @return The enum numeric value on the wire for dataType.
*/
int getDataTypeValue();
/**
* .DataType dataType = 4;
* @return The dataType.
*/
ucar.nc2.stream.NcStreamProto.DataType getDataType();
/**
* .Section section = 5;
* @return Whether the section field is set.
*/
boolean hasSection();
/**
* .Section section = 5;
* @return The section.
*/
ucar.nc2.stream.NcStreamProto.Section getSection();
/**
* .Section section = 5;
*/
ucar.nc2.stream.NcStreamProto.SectionOrBuilder getSectionOrBuilder();
}
/**
* Protobuf type {@code PointFeatureMember}
*/
public static final class PointFeatureMember extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:PointFeatureMember)
PointFeatureMemberOrBuilder {
private static final long serialVersionUID = 0L;
// Use PointFeatureMember.newBuilder() to construct.
private PointFeatureMember(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private PointFeatureMember() {
name_ = "";
desc_ = "";
units_ = "";
dataType_ = 0;
}
@java.lang.Override
@SuppressWarnings({"unused"})
protected java.lang.Object newInstance(
UnusedPrivateParameter unused) {
return new PointFeatureMember();
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return ucar.nc2.ft.point.remote.PointStreamProto.internal_static_PointFeatureMember_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return ucar.nc2.ft.point.remote.PointStreamProto.internal_static_PointFeatureMember_fieldAccessorTable
.ensureFieldAccessorsInitialized(
ucar.nc2.ft.point.remote.PointStreamProto.PointFeatureMember.class, ucar.nc2.ft.point.remote.PointStreamProto.PointFeatureMember.Builder.class);
}
public static final int NAME_FIELD_NUMBER = 1;
@SuppressWarnings("serial")
private volatile java.lang.Object name_ = "";
/**
* string name = 1;
* @return The name.
*/
@java.lang.Override
public java.lang.String getName() {
java.lang.Object ref = name_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
name_ = s;
return s;
}
}
/**
* string name = 1;
* @return The bytes for name.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getNameBytes() {
java.lang.Object ref = name_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
name_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int DESC_FIELD_NUMBER = 2;
@SuppressWarnings("serial")
private volatile java.lang.Object desc_ = "";
/**
* string desc = 2;
* @return The desc.
*/
@java.lang.Override
public java.lang.String getDesc() {
java.lang.Object ref = desc_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
desc_ = s;
return s;
}
}
/**
* string desc = 2;
* @return The bytes for desc.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getDescBytes() {
java.lang.Object ref = desc_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
desc_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int UNITS_FIELD_NUMBER = 3;
@SuppressWarnings("serial")
private volatile java.lang.Object units_ = "";
/**
* string units = 3;
* @return The units.
*/
@java.lang.Override
public java.lang.String getUnits() {
java.lang.Object ref = units_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
units_ = s;
return s;
}
}
/**
* string units = 3;
* @return The bytes for units.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getUnitsBytes() {
java.lang.Object ref = units_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
units_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int DATATYPE_FIELD_NUMBER = 4;
private int dataType_ = 0;
/**
* .DataType dataType = 4;
* @return The enum numeric value on the wire for dataType.
*/
@java.lang.Override public int getDataTypeValue() {
return dataType_;
}
/**
* .DataType dataType = 4;
* @return The dataType.
*/
@java.lang.Override public ucar.nc2.stream.NcStreamProto.DataType getDataType() {
ucar.nc2.stream.NcStreamProto.DataType result = ucar.nc2.stream.NcStreamProto.DataType.forNumber(dataType_);
return result == null ? ucar.nc2.stream.NcStreamProto.DataType.UNRECOGNIZED : result;
}
public static final int SECTION_FIELD_NUMBER = 5;
private ucar.nc2.stream.NcStreamProto.Section section_;
/**
* .Section section = 5;
* @return Whether the section field is set.
*/
@java.lang.Override
public boolean hasSection() {
return section_ != null;
}
/**
* .Section section = 5;
* @return The section.
*/
@java.lang.Override
public ucar.nc2.stream.NcStreamProto.Section getSection() {
return section_ == null ? ucar.nc2.stream.NcStreamProto.Section.getDefaultInstance() : section_;
}
/**
* .Section section = 5;
*/
@java.lang.Override
public ucar.nc2.stream.NcStreamProto.SectionOrBuilder getSectionOrBuilder() {
return section_ == null ? ucar.nc2.stream.NcStreamProto.Section.getDefaultInstance() : section_;
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(name_)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 1, name_);
}
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(desc_)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 2, desc_);
}
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(units_)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 3, units_);
}
if (dataType_ != ucar.nc2.stream.NcStreamProto.DataType.CHAR.getNumber()) {
output.writeEnum(4, dataType_);
}
if (section_ != null) {
output.writeMessage(5, getSection());
}
getUnknownFields().writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(name_)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(1, name_);
}
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(desc_)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(2, desc_);
}
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(units_)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(3, units_);
}
if (dataType_ != ucar.nc2.stream.NcStreamProto.DataType.CHAR.getNumber()) {
size += com.google.protobuf.CodedOutputStream
.computeEnumSize(4, dataType_);
}
if (section_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(5, getSection());
}
size += getUnknownFields().getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof ucar.nc2.ft.point.remote.PointStreamProto.PointFeatureMember)) {
return super.equals(obj);
}
ucar.nc2.ft.point.remote.PointStreamProto.PointFeatureMember other = (ucar.nc2.ft.point.remote.PointStreamProto.PointFeatureMember) obj;
if (!getName()
.equals(other.getName())) return false;
if (!getDesc()
.equals(other.getDesc())) return false;
if (!getUnits()
.equals(other.getUnits())) return false;
if (dataType_ != other.dataType_) return false;
if (hasSection() != other.hasSection()) return false;
if (hasSection()) {
if (!getSection()
.equals(other.getSection())) return false;
}
if (!getUnknownFields().equals(other.getUnknownFields())) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
hash = (37 * hash) + NAME_FIELD_NUMBER;
hash = (53 * hash) + getName().hashCode();
hash = (37 * hash) + DESC_FIELD_NUMBER;
hash = (53 * hash) + getDesc().hashCode();
hash = (37 * hash) + UNITS_FIELD_NUMBER;
hash = (53 * hash) + getUnits().hashCode();
hash = (37 * hash) + DATATYPE_FIELD_NUMBER;
hash = (53 * hash) + dataType_;
if (hasSection()) {
hash = (37 * hash) + SECTION_FIELD_NUMBER;
hash = (53 * hash) + getSection().hashCode();
}
hash = (29 * hash) + getUnknownFields().hashCode();
memoizedHashCode = hash;
return hash;
}
public static ucar.nc2.ft.point.remote.PointStreamProto.PointFeatureMember parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static ucar.nc2.ft.point.remote.PointStreamProto.PointFeatureMember parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static ucar.nc2.ft.point.remote.PointStreamProto.PointFeatureMember parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static ucar.nc2.ft.point.remote.PointStreamProto.PointFeatureMember parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static ucar.nc2.ft.point.remote.PointStreamProto.PointFeatureMember parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static ucar.nc2.ft.point.remote.PointStreamProto.PointFeatureMember parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static ucar.nc2.ft.point.remote.PointStreamProto.PointFeatureMember parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static ucar.nc2.ft.point.remote.PointStreamProto.PointFeatureMember parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static ucar.nc2.ft.point.remote.PointStreamProto.PointFeatureMember parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static ucar.nc2.ft.point.remote.PointStreamProto.PointFeatureMember parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static ucar.nc2.ft.point.remote.PointStreamProto.PointFeatureMember parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static ucar.nc2.ft.point.remote.PointStreamProto.PointFeatureMember parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(ucar.nc2.ft.point.remote.PointStreamProto.PointFeatureMember prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code PointFeatureMember}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:PointFeatureMember)
ucar.nc2.ft.point.remote.PointStreamProto.PointFeatureMemberOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return ucar.nc2.ft.point.remote.PointStreamProto.internal_static_PointFeatureMember_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return ucar.nc2.ft.point.remote.PointStreamProto.internal_static_PointFeatureMember_fieldAccessorTable
.ensureFieldAccessorsInitialized(
ucar.nc2.ft.point.remote.PointStreamProto.PointFeatureMember.class, ucar.nc2.ft.point.remote.PointStreamProto.PointFeatureMember.Builder.class);
}
// Construct using ucar.nc2.ft.point.remote.PointStreamProto.PointFeatureMember.newBuilder()
private Builder() {
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
}
@java.lang.Override
public Builder clear() {
super.clear();
bitField0_ = 0;
name_ = "";
desc_ = "";
units_ = "";
dataType_ = 0;
section_ = null;
if (sectionBuilder_ != null) {
sectionBuilder_.dispose();
sectionBuilder_ = null;
}
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return ucar.nc2.ft.point.remote.PointStreamProto.internal_static_PointFeatureMember_descriptor;
}
@java.lang.Override
public ucar.nc2.ft.point.remote.PointStreamProto.PointFeatureMember getDefaultInstanceForType() {
return ucar.nc2.ft.point.remote.PointStreamProto.PointFeatureMember.getDefaultInstance();
}
@java.lang.Override
public ucar.nc2.ft.point.remote.PointStreamProto.PointFeatureMember build() {
ucar.nc2.ft.point.remote.PointStreamProto.PointFeatureMember result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public ucar.nc2.ft.point.remote.PointStreamProto.PointFeatureMember buildPartial() {
ucar.nc2.ft.point.remote.PointStreamProto.PointFeatureMember result = new ucar.nc2.ft.point.remote.PointStreamProto.PointFeatureMember(this);
if (bitField0_ != 0) { buildPartial0(result); }
onBuilt();
return result;
}
private void buildPartial0(ucar.nc2.ft.point.remote.PointStreamProto.PointFeatureMember result) {
int from_bitField0_ = bitField0_;
if (((from_bitField0_ & 0x00000001) != 0)) {
result.name_ = name_;
}
if (((from_bitField0_ & 0x00000002) != 0)) {
result.desc_ = desc_;
}
if (((from_bitField0_ & 0x00000004) != 0)) {
result.units_ = units_;
}
if (((from_bitField0_ & 0x00000008) != 0)) {
result.dataType_ = dataType_;
}
if (((from_bitField0_ & 0x00000010) != 0)) {
result.section_ = sectionBuilder_ == null
? section_
: sectionBuilder_.build();
}
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof ucar.nc2.ft.point.remote.PointStreamProto.PointFeatureMember) {
return mergeFrom((ucar.nc2.ft.point.remote.PointStreamProto.PointFeatureMember)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(ucar.nc2.ft.point.remote.PointStreamProto.PointFeatureMember other) {
if (other == ucar.nc2.ft.point.remote.PointStreamProto.PointFeatureMember.getDefaultInstance()) return this;
if (!other.getName().isEmpty()) {
name_ = other.name_;
bitField0_ |= 0x00000001;
onChanged();
}
if (!other.getDesc().isEmpty()) {
desc_ = other.desc_;
bitField0_ |= 0x00000002;
onChanged();
}
if (!other.getUnits().isEmpty()) {
units_ = other.units_;
bitField0_ |= 0x00000004;
onChanged();
}
if (other.dataType_ != 0) {
setDataTypeValue(other.getDataTypeValue());
}
if (other.hasSection()) {
mergeSection(other.getSection());
}
this.mergeUnknownFields(other.getUnknownFields());
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 10: {
name_ = input.readStringRequireUtf8();
bitField0_ |= 0x00000001;
break;
} // case 10
case 18: {
desc_ = input.readStringRequireUtf8();
bitField0_ |= 0x00000002;
break;
} // case 18
case 26: {
units_ = input.readStringRequireUtf8();
bitField0_ |= 0x00000004;
break;
} // case 26
case 32: {
dataType_ = input.readEnum();
bitField0_ |= 0x00000008;
break;
} // case 32
case 42: {
input.readMessage(
getSectionFieldBuilder().getBuilder(),
extensionRegistry);
bitField0_ |= 0x00000010;
break;
} // case 42
default: {
if (!super.parseUnknownField(input, extensionRegistry, tag)) {
done = true; // was an endgroup tag
}
break;
} // default:
} // switch (tag)
} // while (!done)
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.unwrapIOException();
} finally {
onChanged();
} // finally
return this;
}
private int bitField0_;
private java.lang.Object name_ = "";
/**
* string name = 1;
* @return The name.
*/
public java.lang.String getName() {
java.lang.Object ref = name_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
name_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* string name = 1;
* @return The bytes for name.
*/
public com.google.protobuf.ByteString
getNameBytes() {
java.lang.Object ref = name_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
name_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* string name = 1;
* @param value The name to set.
* @return This builder for chaining.
*/
public Builder setName(
java.lang.String value) {
if (value == null) { throw new NullPointerException(); }
name_ = value;
bitField0_ |= 0x00000001;
onChanged();
return this;
}
/**
* string name = 1;
* @return This builder for chaining.
*/
public Builder clearName() {
name_ = getDefaultInstance().getName();
bitField0_ = (bitField0_ & ~0x00000001);
onChanged();
return this;
}
/**
* string name = 1;
* @param value The bytes for name to set.
* @return This builder for chaining.
*/
public Builder setNameBytes(
com.google.protobuf.ByteString value) {
if (value == null) { throw new NullPointerException(); }
checkByteStringIsUtf8(value);
name_ = value;
bitField0_ |= 0x00000001;
onChanged();
return this;
}
private java.lang.Object desc_ = "";
/**
* string desc = 2;
* @return The desc.
*/
public java.lang.String getDesc() {
java.lang.Object ref = desc_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
desc_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* string desc = 2;
* @return The bytes for desc.
*/
public com.google.protobuf.ByteString
getDescBytes() {
java.lang.Object ref = desc_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
desc_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* string desc = 2;
* @param value The desc to set.
* @return This builder for chaining.
*/
public Builder setDesc(
java.lang.String value) {
if (value == null) { throw new NullPointerException(); }
desc_ = value;
bitField0_ |= 0x00000002;
onChanged();
return this;
}
/**
* string desc = 2;
* @return This builder for chaining.
*/
public Builder clearDesc() {
desc_ = getDefaultInstance().getDesc();
bitField0_ = (bitField0_ & ~0x00000002);
onChanged();
return this;
}
/**
* string desc = 2;
* @param value The bytes for desc to set.
* @return This builder for chaining.
*/
public Builder setDescBytes(
com.google.protobuf.ByteString value) {
if (value == null) { throw new NullPointerException(); }
checkByteStringIsUtf8(value);
desc_ = value;
bitField0_ |= 0x00000002;
onChanged();
return this;
}
private java.lang.Object units_ = "";
/**
* string units = 3;
* @return The units.
*/
public java.lang.String getUnits() {
java.lang.Object ref = units_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
units_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* string units = 3;
* @return The bytes for units.
*/
public com.google.protobuf.ByteString
getUnitsBytes() {
java.lang.Object ref = units_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
units_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* string units = 3;
* @param value The units to set.
* @return This builder for chaining.
*/
public Builder setUnits(
java.lang.String value) {
if (value == null) { throw new NullPointerException(); }
units_ = value;
bitField0_ |= 0x00000004;
onChanged();
return this;
}
/**
* string units = 3;
* @return This builder for chaining.
*/
public Builder clearUnits() {
units_ = getDefaultInstance().getUnits();
bitField0_ = (bitField0_ & ~0x00000004);
onChanged();
return this;
}
/**
* string units = 3;
* @param value The bytes for units to set.
* @return This builder for chaining.
*/
public Builder setUnitsBytes(
com.google.protobuf.ByteString value) {
if (value == null) { throw new NullPointerException(); }
checkByteStringIsUtf8(value);
units_ = value;
bitField0_ |= 0x00000004;
onChanged();
return this;
}
private int dataType_ = 0;
/**
* .DataType dataType = 4;
* @return The enum numeric value on the wire for dataType.
*/
@java.lang.Override public int getDataTypeValue() {
return dataType_;
}
/**
* .DataType dataType = 4;
* @param value The enum numeric value on the wire for dataType to set.
* @return This builder for chaining.
*/
public Builder setDataTypeValue(int value) {
dataType_ = value;
bitField0_ |= 0x00000008;
onChanged();
return this;
}
/**
* .DataType dataType = 4;
* @return The dataType.
*/
@java.lang.Override
public ucar.nc2.stream.NcStreamProto.DataType getDataType() {
ucar.nc2.stream.NcStreamProto.DataType result = ucar.nc2.stream.NcStreamProto.DataType.forNumber(dataType_);
return result == null ? ucar.nc2.stream.NcStreamProto.DataType.UNRECOGNIZED : result;
}
/**
* .DataType dataType = 4;
* @param value The dataType to set.
* @return This builder for chaining.
*/
public Builder setDataType(ucar.nc2.stream.NcStreamProto.DataType value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000008;
dataType_ = value.getNumber();
onChanged();
return this;
}
/**
* .DataType dataType = 4;
* @return This builder for chaining.
*/
public Builder clearDataType() {
bitField0_ = (bitField0_ & ~0x00000008);
dataType_ = 0;
onChanged();
return this;
}
private ucar.nc2.stream.NcStreamProto.Section section_;
private com.google.protobuf.SingleFieldBuilderV3<
ucar.nc2.stream.NcStreamProto.Section, ucar.nc2.stream.NcStreamProto.Section.Builder, ucar.nc2.stream.NcStreamProto.SectionOrBuilder> sectionBuilder_;
/**
* .Section section = 5;
* @return Whether the section field is set.
*/
public boolean hasSection() {
return ((bitField0_ & 0x00000010) != 0);
}
/**
* .Section section = 5;
* @return The section.
*/
public ucar.nc2.stream.NcStreamProto.Section getSection() {
if (sectionBuilder_ == null) {
return section_ == null ? ucar.nc2.stream.NcStreamProto.Section.getDefaultInstance() : section_;
} else {
return sectionBuilder_.getMessage();
}
}
/**
* .Section section = 5;
*/
public Builder setSection(ucar.nc2.stream.NcStreamProto.Section value) {
if (sectionBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
section_ = value;
} else {
sectionBuilder_.setMessage(value);
}
bitField0_ |= 0x00000010;
onChanged();
return this;
}
/**
* .Section section = 5;
*/
public Builder setSection(
ucar.nc2.stream.NcStreamProto.Section.Builder builderForValue) {
if (sectionBuilder_ == null) {
section_ = builderForValue.build();
} else {
sectionBuilder_.setMessage(builderForValue.build());
}
bitField0_ |= 0x00000010;
onChanged();
return this;
}
/**
* .Section section = 5;
*/
public Builder mergeSection(ucar.nc2.stream.NcStreamProto.Section value) {
if (sectionBuilder_ == null) {
if (((bitField0_ & 0x00000010) != 0) &&
section_ != null &&
section_ != ucar.nc2.stream.NcStreamProto.Section.getDefaultInstance()) {
getSectionBuilder().mergeFrom(value);
} else {
section_ = value;
}
} else {
sectionBuilder_.mergeFrom(value);
}
bitField0_ |= 0x00000010;
onChanged();
return this;
}
/**
* .Section section = 5;
*/
public Builder clearSection() {
bitField0_ = (bitField0_ & ~0x00000010);
section_ = null;
if (sectionBuilder_ != null) {
sectionBuilder_.dispose();
sectionBuilder_ = null;
}
onChanged();
return this;
}
/**
* .Section section = 5;
*/
public ucar.nc2.stream.NcStreamProto.Section.Builder getSectionBuilder() {
bitField0_ |= 0x00000010;
onChanged();
return getSectionFieldBuilder().getBuilder();
}
/**
* .Section section = 5;
*/
public ucar.nc2.stream.NcStreamProto.SectionOrBuilder getSectionOrBuilder() {
if (sectionBuilder_ != null) {
return sectionBuilder_.getMessageOrBuilder();
} else {
return section_ == null ?
ucar.nc2.stream.NcStreamProto.Section.getDefaultInstance() : section_;
}
}
/**
* .Section section = 5;
*/
private com.google.protobuf.SingleFieldBuilderV3<
ucar.nc2.stream.NcStreamProto.Section, ucar.nc2.stream.NcStreamProto.Section.Builder, ucar.nc2.stream.NcStreamProto.SectionOrBuilder>
getSectionFieldBuilder() {
if (sectionBuilder_ == null) {
sectionBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
ucar.nc2.stream.NcStreamProto.Section, ucar.nc2.stream.NcStreamProto.Section.Builder, ucar.nc2.stream.NcStreamProto.SectionOrBuilder>(
getSection(),
getParentForChildren(),
isClean());
section_ = null;
}
return sectionBuilder_;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:PointFeatureMember)
}
// @@protoc_insertion_point(class_scope:PointFeatureMember)
private static final ucar.nc2.ft.point.remote.PointStreamProto.PointFeatureMember DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new ucar.nc2.ft.point.remote.PointStreamProto.PointFeatureMember();
}
public static ucar.nc2.ft.point.remote.PointStreamProto.PointFeatureMember getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public PointFeatureMember parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
Builder builder = newBuilder();
try {
builder.mergeFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(builder.buildPartial());
} catch (com.google.protobuf.UninitializedMessageException e) {
throw e.asInvalidProtocolBufferException().setUnfinishedMessage(builder.buildPartial());
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(e)
.setUnfinishedMessage(builder.buildPartial());
}
return builder.buildPartial();
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public ucar.nc2.ft.point.remote.PointStreamProto.PointFeatureMember getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface PointFeatureCollectionOrBuilder extends
// @@protoc_insertion_point(interface_extends:PointFeatureCollection)
com.google.protobuf.MessageOrBuilder {
/**
* string name = 1;
* @return The name.
*/
java.lang.String getName();
/**
* string name = 1;
* @return The bytes for name.
*/
com.google.protobuf.ByteString
getNameBytes();
/**
* string timeUnit = 2;
* @return The timeUnit.
*/
java.lang.String getTimeUnit();
/**
* string timeUnit = 2;
* @return The bytes for timeUnit.
*/
com.google.protobuf.ByteString
getTimeUnitBytes();
/**
* repeated .PointFeatureMember members = 3;
*/
java.util.List
getMembersList();
/**
* repeated .PointFeatureMember members = 3;
*/
ucar.nc2.ft.point.remote.PointStreamProto.PointFeatureMember getMembers(int index);
/**
* repeated .PointFeatureMember members = 3;
*/
int getMembersCount();
/**
* repeated .PointFeatureMember members = 3;
*/
java.util.List extends ucar.nc2.ft.point.remote.PointStreamProto.PointFeatureMemberOrBuilder>
getMembersOrBuilderList();
/**
* repeated .PointFeatureMember members = 3;
*/
ucar.nc2.ft.point.remote.PointStreamProto.PointFeatureMemberOrBuilder getMembersOrBuilder(
int index);
/**
* string altUnit = 4;
* @return The altUnit.
*/
java.lang.String getAltUnit();
/**
* string altUnit = 4;
* @return The bytes for altUnit.
*/
com.google.protobuf.ByteString
getAltUnitBytes();
}
/**
* Protobuf type {@code PointFeatureCollection}
*/
public static final class PointFeatureCollection extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:PointFeatureCollection)
PointFeatureCollectionOrBuilder {
private static final long serialVersionUID = 0L;
// Use PointFeatureCollection.newBuilder() to construct.
private PointFeatureCollection(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private PointFeatureCollection() {
name_ = "";
timeUnit_ = "";
members_ = java.util.Collections.emptyList();
altUnit_ = "";
}
@java.lang.Override
@SuppressWarnings({"unused"})
protected java.lang.Object newInstance(
UnusedPrivateParameter unused) {
return new PointFeatureCollection();
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return ucar.nc2.ft.point.remote.PointStreamProto.internal_static_PointFeatureCollection_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return ucar.nc2.ft.point.remote.PointStreamProto.internal_static_PointFeatureCollection_fieldAccessorTable
.ensureFieldAccessorsInitialized(
ucar.nc2.ft.point.remote.PointStreamProto.PointFeatureCollection.class, ucar.nc2.ft.point.remote.PointStreamProto.PointFeatureCollection.Builder.class);
}
public static final int NAME_FIELD_NUMBER = 1;
@SuppressWarnings("serial")
private volatile java.lang.Object name_ = "";
/**
* string name = 1;
* @return The name.
*/
@java.lang.Override
public java.lang.String getName() {
java.lang.Object ref = name_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
name_ = s;
return s;
}
}
/**
* string name = 1;
* @return The bytes for name.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getNameBytes() {
java.lang.Object ref = name_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
name_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int TIMEUNIT_FIELD_NUMBER = 2;
@SuppressWarnings("serial")
private volatile java.lang.Object timeUnit_ = "";
/**
* string timeUnit = 2;
* @return The timeUnit.
*/
@java.lang.Override
public java.lang.String getTimeUnit() {
java.lang.Object ref = timeUnit_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
timeUnit_ = s;
return s;
}
}
/**
* string timeUnit = 2;
* @return The bytes for timeUnit.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getTimeUnitBytes() {
java.lang.Object ref = timeUnit_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
timeUnit_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int MEMBERS_FIELD_NUMBER = 3;
@SuppressWarnings("serial")
private java.util.List members_;
/**
* repeated .PointFeatureMember members = 3;
*/
@java.lang.Override
public java.util.List getMembersList() {
return members_;
}
/**
* repeated .PointFeatureMember members = 3;
*/
@java.lang.Override
public java.util.List extends ucar.nc2.ft.point.remote.PointStreamProto.PointFeatureMemberOrBuilder>
getMembersOrBuilderList() {
return members_;
}
/**
* repeated .PointFeatureMember members = 3;
*/
@java.lang.Override
public int getMembersCount() {
return members_.size();
}
/**
* repeated .PointFeatureMember members = 3;
*/
@java.lang.Override
public ucar.nc2.ft.point.remote.PointStreamProto.PointFeatureMember getMembers(int index) {
return members_.get(index);
}
/**
* repeated .PointFeatureMember members = 3;
*/
@java.lang.Override
public ucar.nc2.ft.point.remote.PointStreamProto.PointFeatureMemberOrBuilder getMembersOrBuilder(
int index) {
return members_.get(index);
}
public static final int ALTUNIT_FIELD_NUMBER = 4;
@SuppressWarnings("serial")
private volatile java.lang.Object altUnit_ = "";
/**
* string altUnit = 4;
* @return The altUnit.
*/
@java.lang.Override
public java.lang.String getAltUnit() {
java.lang.Object ref = altUnit_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
altUnit_ = s;
return s;
}
}
/**
* string altUnit = 4;
* @return The bytes for altUnit.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getAltUnitBytes() {
java.lang.Object ref = altUnit_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
altUnit_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(name_)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 1, name_);
}
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(timeUnit_)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 2, timeUnit_);
}
for (int i = 0; i < members_.size(); i++) {
output.writeMessage(3, members_.get(i));
}
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(altUnit_)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 4, altUnit_);
}
getUnknownFields().writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(name_)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(1, name_);
}
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(timeUnit_)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(2, timeUnit_);
}
for (int i = 0; i < members_.size(); i++) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(3, members_.get(i));
}
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(altUnit_)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(4, altUnit_);
}
size += getUnknownFields().getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof ucar.nc2.ft.point.remote.PointStreamProto.PointFeatureCollection)) {
return super.equals(obj);
}
ucar.nc2.ft.point.remote.PointStreamProto.PointFeatureCollection other = (ucar.nc2.ft.point.remote.PointStreamProto.PointFeatureCollection) obj;
if (!getName()
.equals(other.getName())) return false;
if (!getTimeUnit()
.equals(other.getTimeUnit())) return false;
if (!getMembersList()
.equals(other.getMembersList())) return false;
if (!getAltUnit()
.equals(other.getAltUnit())) return false;
if (!getUnknownFields().equals(other.getUnknownFields())) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
hash = (37 * hash) + NAME_FIELD_NUMBER;
hash = (53 * hash) + getName().hashCode();
hash = (37 * hash) + TIMEUNIT_FIELD_NUMBER;
hash = (53 * hash) + getTimeUnit().hashCode();
if (getMembersCount() > 0) {
hash = (37 * hash) + MEMBERS_FIELD_NUMBER;
hash = (53 * hash) + getMembersList().hashCode();
}
hash = (37 * hash) + ALTUNIT_FIELD_NUMBER;
hash = (53 * hash) + getAltUnit().hashCode();
hash = (29 * hash) + getUnknownFields().hashCode();
memoizedHashCode = hash;
return hash;
}
public static ucar.nc2.ft.point.remote.PointStreamProto.PointFeatureCollection parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static ucar.nc2.ft.point.remote.PointStreamProto.PointFeatureCollection parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static ucar.nc2.ft.point.remote.PointStreamProto.PointFeatureCollection parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static ucar.nc2.ft.point.remote.PointStreamProto.PointFeatureCollection parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static ucar.nc2.ft.point.remote.PointStreamProto.PointFeatureCollection parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static ucar.nc2.ft.point.remote.PointStreamProto.PointFeatureCollection parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static ucar.nc2.ft.point.remote.PointStreamProto.PointFeatureCollection parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static ucar.nc2.ft.point.remote.PointStreamProto.PointFeatureCollection parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static ucar.nc2.ft.point.remote.PointStreamProto.PointFeatureCollection parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static ucar.nc2.ft.point.remote.PointStreamProto.PointFeatureCollection parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static ucar.nc2.ft.point.remote.PointStreamProto.PointFeatureCollection parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static ucar.nc2.ft.point.remote.PointStreamProto.PointFeatureCollection parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(ucar.nc2.ft.point.remote.PointStreamProto.PointFeatureCollection prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code PointFeatureCollection}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:PointFeatureCollection)
ucar.nc2.ft.point.remote.PointStreamProto.PointFeatureCollectionOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return ucar.nc2.ft.point.remote.PointStreamProto.internal_static_PointFeatureCollection_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return ucar.nc2.ft.point.remote.PointStreamProto.internal_static_PointFeatureCollection_fieldAccessorTable
.ensureFieldAccessorsInitialized(
ucar.nc2.ft.point.remote.PointStreamProto.PointFeatureCollection.class, ucar.nc2.ft.point.remote.PointStreamProto.PointFeatureCollection.Builder.class);
}
// Construct using ucar.nc2.ft.point.remote.PointStreamProto.PointFeatureCollection.newBuilder()
private Builder() {
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
}
@java.lang.Override
public Builder clear() {
super.clear();
bitField0_ = 0;
name_ = "";
timeUnit_ = "";
if (membersBuilder_ == null) {
members_ = java.util.Collections.emptyList();
} else {
members_ = null;
membersBuilder_.clear();
}
bitField0_ = (bitField0_ & ~0x00000004);
altUnit_ = "";
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return ucar.nc2.ft.point.remote.PointStreamProto.internal_static_PointFeatureCollection_descriptor;
}
@java.lang.Override
public ucar.nc2.ft.point.remote.PointStreamProto.PointFeatureCollection getDefaultInstanceForType() {
return ucar.nc2.ft.point.remote.PointStreamProto.PointFeatureCollection.getDefaultInstance();
}
@java.lang.Override
public ucar.nc2.ft.point.remote.PointStreamProto.PointFeatureCollection build() {
ucar.nc2.ft.point.remote.PointStreamProto.PointFeatureCollection result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public ucar.nc2.ft.point.remote.PointStreamProto.PointFeatureCollection buildPartial() {
ucar.nc2.ft.point.remote.PointStreamProto.PointFeatureCollection result = new ucar.nc2.ft.point.remote.PointStreamProto.PointFeatureCollection(this);
buildPartialRepeatedFields(result);
if (bitField0_ != 0) { buildPartial0(result); }
onBuilt();
return result;
}
private void buildPartialRepeatedFields(ucar.nc2.ft.point.remote.PointStreamProto.PointFeatureCollection result) {
if (membersBuilder_ == null) {
if (((bitField0_ & 0x00000004) != 0)) {
members_ = java.util.Collections.unmodifiableList(members_);
bitField0_ = (bitField0_ & ~0x00000004);
}
result.members_ = members_;
} else {
result.members_ = membersBuilder_.build();
}
}
private void buildPartial0(ucar.nc2.ft.point.remote.PointStreamProto.PointFeatureCollection result) {
int from_bitField0_ = bitField0_;
if (((from_bitField0_ & 0x00000001) != 0)) {
result.name_ = name_;
}
if (((from_bitField0_ & 0x00000002) != 0)) {
result.timeUnit_ = timeUnit_;
}
if (((from_bitField0_ & 0x00000008) != 0)) {
result.altUnit_ = altUnit_;
}
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof ucar.nc2.ft.point.remote.PointStreamProto.PointFeatureCollection) {
return mergeFrom((ucar.nc2.ft.point.remote.PointStreamProto.PointFeatureCollection)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(ucar.nc2.ft.point.remote.PointStreamProto.PointFeatureCollection other) {
if (other == ucar.nc2.ft.point.remote.PointStreamProto.PointFeatureCollection.getDefaultInstance()) return this;
if (!other.getName().isEmpty()) {
name_ = other.name_;
bitField0_ |= 0x00000001;
onChanged();
}
if (!other.getTimeUnit().isEmpty()) {
timeUnit_ = other.timeUnit_;
bitField0_ |= 0x00000002;
onChanged();
}
if (membersBuilder_ == null) {
if (!other.members_.isEmpty()) {
if (members_.isEmpty()) {
members_ = other.members_;
bitField0_ = (bitField0_ & ~0x00000004);
} else {
ensureMembersIsMutable();
members_.addAll(other.members_);
}
onChanged();
}
} else {
if (!other.members_.isEmpty()) {
if (membersBuilder_.isEmpty()) {
membersBuilder_.dispose();
membersBuilder_ = null;
members_ = other.members_;
bitField0_ = (bitField0_ & ~0x00000004);
membersBuilder_ =
com.google.protobuf.GeneratedMessageV3.alwaysUseFieldBuilders ?
getMembersFieldBuilder() : null;
} else {
membersBuilder_.addAllMessages(other.members_);
}
}
}
if (!other.getAltUnit().isEmpty()) {
altUnit_ = other.altUnit_;
bitField0_ |= 0x00000008;
onChanged();
}
this.mergeUnknownFields(other.getUnknownFields());
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 10: {
name_ = input.readStringRequireUtf8();
bitField0_ |= 0x00000001;
break;
} // case 10
case 18: {
timeUnit_ = input.readStringRequireUtf8();
bitField0_ |= 0x00000002;
break;
} // case 18
case 26: {
ucar.nc2.ft.point.remote.PointStreamProto.PointFeatureMember m =
input.readMessage(
ucar.nc2.ft.point.remote.PointStreamProto.PointFeatureMember.parser(),
extensionRegistry);
if (membersBuilder_ == null) {
ensureMembersIsMutable();
members_.add(m);
} else {
membersBuilder_.addMessage(m);
}
break;
} // case 26
case 34: {
altUnit_ = input.readStringRequireUtf8();
bitField0_ |= 0x00000008;
break;
} // case 34
default: {
if (!super.parseUnknownField(input, extensionRegistry, tag)) {
done = true; // was an endgroup tag
}
break;
} // default:
} // switch (tag)
} // while (!done)
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.unwrapIOException();
} finally {
onChanged();
} // finally
return this;
}
private int bitField0_;
private java.lang.Object name_ = "";
/**
* string name = 1;
* @return The name.
*/
public java.lang.String getName() {
java.lang.Object ref = name_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
name_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* string name = 1;
* @return The bytes for name.
*/
public com.google.protobuf.ByteString
getNameBytes() {
java.lang.Object ref = name_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
name_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* string name = 1;
* @param value The name to set.
* @return This builder for chaining.
*/
public Builder setName(
java.lang.String value) {
if (value == null) { throw new NullPointerException(); }
name_ = value;
bitField0_ |= 0x00000001;
onChanged();
return this;
}
/**
* string name = 1;
* @return This builder for chaining.
*/
public Builder clearName() {
name_ = getDefaultInstance().getName();
bitField0_ = (bitField0_ & ~0x00000001);
onChanged();
return this;
}
/**
* string name = 1;
* @param value The bytes for name to set.
* @return This builder for chaining.
*/
public Builder setNameBytes(
com.google.protobuf.ByteString value) {
if (value == null) { throw new NullPointerException(); }
checkByteStringIsUtf8(value);
name_ = value;
bitField0_ |= 0x00000001;
onChanged();
return this;
}
private java.lang.Object timeUnit_ = "";
/**
* string timeUnit = 2;
* @return The timeUnit.
*/
public java.lang.String getTimeUnit() {
java.lang.Object ref = timeUnit_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
timeUnit_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* string timeUnit = 2;
* @return The bytes for timeUnit.
*/
public com.google.protobuf.ByteString
getTimeUnitBytes() {
java.lang.Object ref = timeUnit_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
timeUnit_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* string timeUnit = 2;
* @param value The timeUnit to set.
* @return This builder for chaining.
*/
public Builder setTimeUnit(
java.lang.String value) {
if (value == null) { throw new NullPointerException(); }
timeUnit_ = value;
bitField0_ |= 0x00000002;
onChanged();
return this;
}
/**
* string timeUnit = 2;
* @return This builder for chaining.
*/
public Builder clearTimeUnit() {
timeUnit_ = getDefaultInstance().getTimeUnit();
bitField0_ = (bitField0_ & ~0x00000002);
onChanged();
return this;
}
/**
* string timeUnit = 2;
* @param value The bytes for timeUnit to set.
* @return This builder for chaining.
*/
public Builder setTimeUnitBytes(
com.google.protobuf.ByteString value) {
if (value == null) { throw new NullPointerException(); }
checkByteStringIsUtf8(value);
timeUnit_ = value;
bitField0_ |= 0x00000002;
onChanged();
return this;
}
private java.util.List members_ =
java.util.Collections.emptyList();
private void ensureMembersIsMutable() {
if (!((bitField0_ & 0x00000004) != 0)) {
members_ = new java.util.ArrayList(members_);
bitField0_ |= 0x00000004;
}
}
private com.google.protobuf.RepeatedFieldBuilderV3<
ucar.nc2.ft.point.remote.PointStreamProto.PointFeatureMember, ucar.nc2.ft.point.remote.PointStreamProto.PointFeatureMember.Builder, ucar.nc2.ft.point.remote.PointStreamProto.PointFeatureMemberOrBuilder> membersBuilder_;
/**
* repeated .PointFeatureMember members = 3;
*/
public java.util.List getMembersList() {
if (membersBuilder_ == null) {
return java.util.Collections.unmodifiableList(members_);
} else {
return membersBuilder_.getMessageList();
}
}
/**
* repeated .PointFeatureMember members = 3;
*/
public int getMembersCount() {
if (membersBuilder_ == null) {
return members_.size();
} else {
return membersBuilder_.getCount();
}
}
/**
* repeated .PointFeatureMember members = 3;
*/
public ucar.nc2.ft.point.remote.PointStreamProto.PointFeatureMember getMembers(int index) {
if (membersBuilder_ == null) {
return members_.get(index);
} else {
return membersBuilder_.getMessage(index);
}
}
/**
* repeated .PointFeatureMember members = 3;
*/
public Builder setMembers(
int index, ucar.nc2.ft.point.remote.PointStreamProto.PointFeatureMember value) {
if (membersBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureMembersIsMutable();
members_.set(index, value);
onChanged();
} else {
membersBuilder_.setMessage(index, value);
}
return this;
}
/**
* repeated .PointFeatureMember members = 3;
*/
public Builder setMembers(
int index, ucar.nc2.ft.point.remote.PointStreamProto.PointFeatureMember.Builder builderForValue) {
if (membersBuilder_ == null) {
ensureMembersIsMutable();
members_.set(index, builderForValue.build());
onChanged();
} else {
membersBuilder_.setMessage(index, builderForValue.build());
}
return this;
}
/**
* repeated .PointFeatureMember members = 3;
*/
public Builder addMembers(ucar.nc2.ft.point.remote.PointStreamProto.PointFeatureMember value) {
if (membersBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureMembersIsMutable();
members_.add(value);
onChanged();
} else {
membersBuilder_.addMessage(value);
}
return this;
}
/**
* repeated .PointFeatureMember members = 3;
*/
public Builder addMembers(
int index, ucar.nc2.ft.point.remote.PointStreamProto.PointFeatureMember value) {
if (membersBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureMembersIsMutable();
members_.add(index, value);
onChanged();
} else {
membersBuilder_.addMessage(index, value);
}
return this;
}
/**
* repeated .PointFeatureMember members = 3;
*/
public Builder addMembers(
ucar.nc2.ft.point.remote.PointStreamProto.PointFeatureMember.Builder builderForValue) {
if (membersBuilder_ == null) {
ensureMembersIsMutable();
members_.add(builderForValue.build());
onChanged();
} else {
membersBuilder_.addMessage(builderForValue.build());
}
return this;
}
/**
* repeated .PointFeatureMember members = 3;
*/
public Builder addMembers(
int index, ucar.nc2.ft.point.remote.PointStreamProto.PointFeatureMember.Builder builderForValue) {
if (membersBuilder_ == null) {
ensureMembersIsMutable();
members_.add(index, builderForValue.build());
onChanged();
} else {
membersBuilder_.addMessage(index, builderForValue.build());
}
return this;
}
/**
* repeated .PointFeatureMember members = 3;
*/
public Builder addAllMembers(
java.lang.Iterable extends ucar.nc2.ft.point.remote.PointStreamProto.PointFeatureMember> values) {
if (membersBuilder_ == null) {
ensureMembersIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(
values, members_);
onChanged();
} else {
membersBuilder_.addAllMessages(values);
}
return this;
}
/**
* repeated .PointFeatureMember members = 3;
*/
public Builder clearMembers() {
if (membersBuilder_ == null) {
members_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000004);
onChanged();
} else {
membersBuilder_.clear();
}
return this;
}
/**
* repeated .PointFeatureMember members = 3;
*/
public Builder removeMembers(int index) {
if (membersBuilder_ == null) {
ensureMembersIsMutable();
members_.remove(index);
onChanged();
} else {
membersBuilder_.remove(index);
}
return this;
}
/**
* repeated .PointFeatureMember members = 3;
*/
public ucar.nc2.ft.point.remote.PointStreamProto.PointFeatureMember.Builder getMembersBuilder(
int index) {
return getMembersFieldBuilder().getBuilder(index);
}
/**
* repeated .PointFeatureMember members = 3;
*/
public ucar.nc2.ft.point.remote.PointStreamProto.PointFeatureMemberOrBuilder getMembersOrBuilder(
int index) {
if (membersBuilder_ == null) {
return members_.get(index); } else {
return membersBuilder_.getMessageOrBuilder(index);
}
}
/**
* repeated .PointFeatureMember members = 3;
*/
public java.util.List extends ucar.nc2.ft.point.remote.PointStreamProto.PointFeatureMemberOrBuilder>
getMembersOrBuilderList() {
if (membersBuilder_ != null) {
return membersBuilder_.getMessageOrBuilderList();
} else {
return java.util.Collections.unmodifiableList(members_);
}
}
/**
* repeated .PointFeatureMember members = 3;
*/
public ucar.nc2.ft.point.remote.PointStreamProto.PointFeatureMember.Builder addMembersBuilder() {
return getMembersFieldBuilder().addBuilder(
ucar.nc2.ft.point.remote.PointStreamProto.PointFeatureMember.getDefaultInstance());
}
/**
* repeated .PointFeatureMember members = 3;
*/
public ucar.nc2.ft.point.remote.PointStreamProto.PointFeatureMember.Builder addMembersBuilder(
int index) {
return getMembersFieldBuilder().addBuilder(
index, ucar.nc2.ft.point.remote.PointStreamProto.PointFeatureMember.getDefaultInstance());
}
/**
* repeated .PointFeatureMember members = 3;
*/
public java.util.List
getMembersBuilderList() {
return getMembersFieldBuilder().getBuilderList();
}
private com.google.protobuf.RepeatedFieldBuilderV3<
ucar.nc2.ft.point.remote.PointStreamProto.PointFeatureMember, ucar.nc2.ft.point.remote.PointStreamProto.PointFeatureMember.Builder, ucar.nc2.ft.point.remote.PointStreamProto.PointFeatureMemberOrBuilder>
getMembersFieldBuilder() {
if (membersBuilder_ == null) {
membersBuilder_ = new com.google.protobuf.RepeatedFieldBuilderV3<
ucar.nc2.ft.point.remote.PointStreamProto.PointFeatureMember, ucar.nc2.ft.point.remote.PointStreamProto.PointFeatureMember.Builder, ucar.nc2.ft.point.remote.PointStreamProto.PointFeatureMemberOrBuilder>(
members_,
((bitField0_ & 0x00000004) != 0),
getParentForChildren(),
isClean());
members_ = null;
}
return membersBuilder_;
}
private java.lang.Object altUnit_ = "";
/**
* string altUnit = 4;
* @return The altUnit.
*/
public java.lang.String getAltUnit() {
java.lang.Object ref = altUnit_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
altUnit_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* string altUnit = 4;
* @return The bytes for altUnit.
*/
public com.google.protobuf.ByteString
getAltUnitBytes() {
java.lang.Object ref = altUnit_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
altUnit_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* string altUnit = 4;
* @param value The altUnit to set.
* @return This builder for chaining.
*/
public Builder setAltUnit(
java.lang.String value) {
if (value == null) { throw new NullPointerException(); }
altUnit_ = value;
bitField0_ |= 0x00000008;
onChanged();
return this;
}
/**
* string altUnit = 4;
* @return This builder for chaining.
*/
public Builder clearAltUnit() {
altUnit_ = getDefaultInstance().getAltUnit();
bitField0_ = (bitField0_ & ~0x00000008);
onChanged();
return this;
}
/**
* string altUnit = 4;
* @param value The bytes for altUnit to set.
* @return This builder for chaining.
*/
public Builder setAltUnitBytes(
com.google.protobuf.ByteString value) {
if (value == null) { throw new NullPointerException(); }
checkByteStringIsUtf8(value);
altUnit_ = value;
bitField0_ |= 0x00000008;
onChanged();
return this;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:PointFeatureCollection)
}
// @@protoc_insertion_point(class_scope:PointFeatureCollection)
private static final ucar.nc2.ft.point.remote.PointStreamProto.PointFeatureCollection DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new ucar.nc2.ft.point.remote.PointStreamProto.PointFeatureCollection();
}
public static ucar.nc2.ft.point.remote.PointStreamProto.PointFeatureCollection getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public PointFeatureCollection parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
Builder builder = newBuilder();
try {
builder.mergeFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(builder.buildPartial());
} catch (com.google.protobuf.UninitializedMessageException e) {
throw e.asInvalidProtocolBufferException().setUnfinishedMessage(builder.buildPartial());
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(e)
.setUnfinishedMessage(builder.buildPartial());
}
return builder.buildPartial();
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public ucar.nc2.ft.point.remote.PointStreamProto.PointFeatureCollection getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface StationOrBuilder extends
// @@protoc_insertion_point(interface_extends:Station)
com.google.protobuf.MessageOrBuilder {
/**
* string id = 1;
* @return The id.
*/
java.lang.String getId();
/**
* string id = 1;
* @return The bytes for id.
*/
com.google.protobuf.ByteString
getIdBytes();
/**
* double lat = 2;
* @return The lat.
*/
double getLat();
/**
* double lon = 3;
* @return The lon.
*/
double getLon();
/**
* double alt = 4;
* @return The alt.
*/
double getAlt();
/**
* string desc = 5;
* @return The desc.
*/
java.lang.String getDesc();
/**
* string desc = 5;
* @return The bytes for desc.
*/
com.google.protobuf.ByteString
getDescBytes();
/**
* string wmoId = 6;
* @return The wmoId.
*/
java.lang.String getWmoId();
/**
* string wmoId = 6;
* @return The bytes for wmoId.
*/
com.google.protobuf.ByteString
getWmoIdBytes();
}
/**
* Protobuf type {@code Station}
*/
public static final class Station extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:Station)
StationOrBuilder {
private static final long serialVersionUID = 0L;
// Use Station.newBuilder() to construct.
private Station(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private Station() {
id_ = "";
desc_ = "";
wmoId_ = "";
}
@java.lang.Override
@SuppressWarnings({"unused"})
protected java.lang.Object newInstance(
UnusedPrivateParameter unused) {
return new Station();
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return ucar.nc2.ft.point.remote.PointStreamProto.internal_static_Station_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return ucar.nc2.ft.point.remote.PointStreamProto.internal_static_Station_fieldAccessorTable
.ensureFieldAccessorsInitialized(
ucar.nc2.ft.point.remote.PointStreamProto.Station.class, ucar.nc2.ft.point.remote.PointStreamProto.Station.Builder.class);
}
public static final int ID_FIELD_NUMBER = 1;
@SuppressWarnings("serial")
private volatile java.lang.Object id_ = "";
/**
* string id = 1;
* @return The id.
*/
@java.lang.Override
public java.lang.String getId() {
java.lang.Object ref = id_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
id_ = s;
return s;
}
}
/**
* string id = 1;
* @return The bytes for id.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getIdBytes() {
java.lang.Object ref = id_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
id_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int LAT_FIELD_NUMBER = 2;
private double lat_ = 0D;
/**
* double lat = 2;
* @return The lat.
*/
@java.lang.Override
public double getLat() {
return lat_;
}
public static final int LON_FIELD_NUMBER = 3;
private double lon_ = 0D;
/**
* double lon = 3;
* @return The lon.
*/
@java.lang.Override
public double getLon() {
return lon_;
}
public static final int ALT_FIELD_NUMBER = 4;
private double alt_ = 0D;
/**
* double alt = 4;
* @return The alt.
*/
@java.lang.Override
public double getAlt() {
return alt_;
}
public static final int DESC_FIELD_NUMBER = 5;
@SuppressWarnings("serial")
private volatile java.lang.Object desc_ = "";
/**
* string desc = 5;
* @return The desc.
*/
@java.lang.Override
public java.lang.String getDesc() {
java.lang.Object ref = desc_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
desc_ = s;
return s;
}
}
/**
* string desc = 5;
* @return The bytes for desc.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getDescBytes() {
java.lang.Object ref = desc_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
desc_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int WMOID_FIELD_NUMBER = 6;
@SuppressWarnings("serial")
private volatile java.lang.Object wmoId_ = "";
/**
* string wmoId = 6;
* @return The wmoId.
*/
@java.lang.Override
public java.lang.String getWmoId() {
java.lang.Object ref = wmoId_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
wmoId_ = s;
return s;
}
}
/**
* string wmoId = 6;
* @return The bytes for wmoId.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getWmoIdBytes() {
java.lang.Object ref = wmoId_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
wmoId_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(id_)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 1, id_);
}
if (java.lang.Double.doubleToRawLongBits(lat_) != 0) {
output.writeDouble(2, lat_);
}
if (java.lang.Double.doubleToRawLongBits(lon_) != 0) {
output.writeDouble(3, lon_);
}
if (java.lang.Double.doubleToRawLongBits(alt_) != 0) {
output.writeDouble(4, alt_);
}
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(desc_)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 5, desc_);
}
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(wmoId_)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 6, wmoId_);
}
getUnknownFields().writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(id_)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(1, id_);
}
if (java.lang.Double.doubleToRawLongBits(lat_) != 0) {
size += com.google.protobuf.CodedOutputStream
.computeDoubleSize(2, lat_);
}
if (java.lang.Double.doubleToRawLongBits(lon_) != 0) {
size += com.google.protobuf.CodedOutputStream
.computeDoubleSize(3, lon_);
}
if (java.lang.Double.doubleToRawLongBits(alt_) != 0) {
size += com.google.protobuf.CodedOutputStream
.computeDoubleSize(4, alt_);
}
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(desc_)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(5, desc_);
}
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(wmoId_)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(6, wmoId_);
}
size += getUnknownFields().getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof ucar.nc2.ft.point.remote.PointStreamProto.Station)) {
return super.equals(obj);
}
ucar.nc2.ft.point.remote.PointStreamProto.Station other = (ucar.nc2.ft.point.remote.PointStreamProto.Station) obj;
if (!getId()
.equals(other.getId())) return false;
if (java.lang.Double.doubleToLongBits(getLat())
!= java.lang.Double.doubleToLongBits(
other.getLat())) return false;
if (java.lang.Double.doubleToLongBits(getLon())
!= java.lang.Double.doubleToLongBits(
other.getLon())) return false;
if (java.lang.Double.doubleToLongBits(getAlt())
!= java.lang.Double.doubleToLongBits(
other.getAlt())) return false;
if (!getDesc()
.equals(other.getDesc())) return false;
if (!getWmoId()
.equals(other.getWmoId())) return false;
if (!getUnknownFields().equals(other.getUnknownFields())) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
hash = (37 * hash) + ID_FIELD_NUMBER;
hash = (53 * hash) + getId().hashCode();
hash = (37 * hash) + LAT_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(
java.lang.Double.doubleToLongBits(getLat()));
hash = (37 * hash) + LON_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(
java.lang.Double.doubleToLongBits(getLon()));
hash = (37 * hash) + ALT_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(
java.lang.Double.doubleToLongBits(getAlt()));
hash = (37 * hash) + DESC_FIELD_NUMBER;
hash = (53 * hash) + getDesc().hashCode();
hash = (37 * hash) + WMOID_FIELD_NUMBER;
hash = (53 * hash) + getWmoId().hashCode();
hash = (29 * hash) + getUnknownFields().hashCode();
memoizedHashCode = hash;
return hash;
}
public static ucar.nc2.ft.point.remote.PointStreamProto.Station parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static ucar.nc2.ft.point.remote.PointStreamProto.Station parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static ucar.nc2.ft.point.remote.PointStreamProto.Station parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static ucar.nc2.ft.point.remote.PointStreamProto.Station parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static ucar.nc2.ft.point.remote.PointStreamProto.Station parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static ucar.nc2.ft.point.remote.PointStreamProto.Station parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static ucar.nc2.ft.point.remote.PointStreamProto.Station parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static ucar.nc2.ft.point.remote.PointStreamProto.Station parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static ucar.nc2.ft.point.remote.PointStreamProto.Station parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static ucar.nc2.ft.point.remote.PointStreamProto.Station parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static ucar.nc2.ft.point.remote.PointStreamProto.Station parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static ucar.nc2.ft.point.remote.PointStreamProto.Station parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(ucar.nc2.ft.point.remote.PointStreamProto.Station prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code Station}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:Station)
ucar.nc2.ft.point.remote.PointStreamProto.StationOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return ucar.nc2.ft.point.remote.PointStreamProto.internal_static_Station_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return ucar.nc2.ft.point.remote.PointStreamProto.internal_static_Station_fieldAccessorTable
.ensureFieldAccessorsInitialized(
ucar.nc2.ft.point.remote.PointStreamProto.Station.class, ucar.nc2.ft.point.remote.PointStreamProto.Station.Builder.class);
}
// Construct using ucar.nc2.ft.point.remote.PointStreamProto.Station.newBuilder()
private Builder() {
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
}
@java.lang.Override
public Builder clear() {
super.clear();
bitField0_ = 0;
id_ = "";
lat_ = 0D;
lon_ = 0D;
alt_ = 0D;
desc_ = "";
wmoId_ = "";
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return ucar.nc2.ft.point.remote.PointStreamProto.internal_static_Station_descriptor;
}
@java.lang.Override
public ucar.nc2.ft.point.remote.PointStreamProto.Station getDefaultInstanceForType() {
return ucar.nc2.ft.point.remote.PointStreamProto.Station.getDefaultInstance();
}
@java.lang.Override
public ucar.nc2.ft.point.remote.PointStreamProto.Station build() {
ucar.nc2.ft.point.remote.PointStreamProto.Station result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public ucar.nc2.ft.point.remote.PointStreamProto.Station buildPartial() {
ucar.nc2.ft.point.remote.PointStreamProto.Station result = new ucar.nc2.ft.point.remote.PointStreamProto.Station(this);
if (bitField0_ != 0) { buildPartial0(result); }
onBuilt();
return result;
}
private void buildPartial0(ucar.nc2.ft.point.remote.PointStreamProto.Station result) {
int from_bitField0_ = bitField0_;
if (((from_bitField0_ & 0x00000001) != 0)) {
result.id_ = id_;
}
if (((from_bitField0_ & 0x00000002) != 0)) {
result.lat_ = lat_;
}
if (((from_bitField0_ & 0x00000004) != 0)) {
result.lon_ = lon_;
}
if (((from_bitField0_ & 0x00000008) != 0)) {
result.alt_ = alt_;
}
if (((from_bitField0_ & 0x00000010) != 0)) {
result.desc_ = desc_;
}
if (((from_bitField0_ & 0x00000020) != 0)) {
result.wmoId_ = wmoId_;
}
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof ucar.nc2.ft.point.remote.PointStreamProto.Station) {
return mergeFrom((ucar.nc2.ft.point.remote.PointStreamProto.Station)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(ucar.nc2.ft.point.remote.PointStreamProto.Station other) {
if (other == ucar.nc2.ft.point.remote.PointStreamProto.Station.getDefaultInstance()) return this;
if (!other.getId().isEmpty()) {
id_ = other.id_;
bitField0_ |= 0x00000001;
onChanged();
}
if (other.getLat() != 0D) {
setLat(other.getLat());
}
if (other.getLon() != 0D) {
setLon(other.getLon());
}
if (other.getAlt() != 0D) {
setAlt(other.getAlt());
}
if (!other.getDesc().isEmpty()) {
desc_ = other.desc_;
bitField0_ |= 0x00000010;
onChanged();
}
if (!other.getWmoId().isEmpty()) {
wmoId_ = other.wmoId_;
bitField0_ |= 0x00000020;
onChanged();
}
this.mergeUnknownFields(other.getUnknownFields());
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 10: {
id_ = input.readStringRequireUtf8();
bitField0_ |= 0x00000001;
break;
} // case 10
case 17: {
lat_ = input.readDouble();
bitField0_ |= 0x00000002;
break;
} // case 17
case 25: {
lon_ = input.readDouble();
bitField0_ |= 0x00000004;
break;
} // case 25
case 33: {
alt_ = input.readDouble();
bitField0_ |= 0x00000008;
break;
} // case 33
case 42: {
desc_ = input.readStringRequireUtf8();
bitField0_ |= 0x00000010;
break;
} // case 42
case 50: {
wmoId_ = input.readStringRequireUtf8();
bitField0_ |= 0x00000020;
break;
} // case 50
default: {
if (!super.parseUnknownField(input, extensionRegistry, tag)) {
done = true; // was an endgroup tag
}
break;
} // default:
} // switch (tag)
} // while (!done)
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.unwrapIOException();
} finally {
onChanged();
} // finally
return this;
}
private int bitField0_;
private java.lang.Object id_ = "";
/**
* string id = 1;
* @return The id.
*/
public java.lang.String getId() {
java.lang.Object ref = id_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
id_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* string id = 1;
* @return The bytes for id.
*/
public com.google.protobuf.ByteString
getIdBytes() {
java.lang.Object ref = id_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
id_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* string id = 1;
* @param value The id to set.
* @return This builder for chaining.
*/
public Builder setId(
java.lang.String value) {
if (value == null) { throw new NullPointerException(); }
id_ = value;
bitField0_ |= 0x00000001;
onChanged();
return this;
}
/**
* string id = 1;
* @return This builder for chaining.
*/
public Builder clearId() {
id_ = getDefaultInstance().getId();
bitField0_ = (bitField0_ & ~0x00000001);
onChanged();
return this;
}
/**
* string id = 1;
* @param value The bytes for id to set.
* @return This builder for chaining.
*/
public Builder setIdBytes(
com.google.protobuf.ByteString value) {
if (value == null) { throw new NullPointerException(); }
checkByteStringIsUtf8(value);
id_ = value;
bitField0_ |= 0x00000001;
onChanged();
return this;
}
private double lat_ ;
/**
* double lat = 2;
* @return The lat.
*/
@java.lang.Override
public double getLat() {
return lat_;
}
/**
* double lat = 2;
* @param value The lat to set.
* @return This builder for chaining.
*/
public Builder setLat(double value) {
lat_ = value;
bitField0_ |= 0x00000002;
onChanged();
return this;
}
/**
* double lat = 2;
* @return This builder for chaining.
*/
public Builder clearLat() {
bitField0_ = (bitField0_ & ~0x00000002);
lat_ = 0D;
onChanged();
return this;
}
private double lon_ ;
/**
* double lon = 3;
* @return The lon.
*/
@java.lang.Override
public double getLon() {
return lon_;
}
/**
* double lon = 3;
* @param value The lon to set.
* @return This builder for chaining.
*/
public Builder setLon(double value) {
lon_ = value;
bitField0_ |= 0x00000004;
onChanged();
return this;
}
/**
* double lon = 3;
* @return This builder for chaining.
*/
public Builder clearLon() {
bitField0_ = (bitField0_ & ~0x00000004);
lon_ = 0D;
onChanged();
return this;
}
private double alt_ ;
/**
* double alt = 4;
* @return The alt.
*/
@java.lang.Override
public double getAlt() {
return alt_;
}
/**
* double alt = 4;
* @param value The alt to set.
* @return This builder for chaining.
*/
public Builder setAlt(double value) {
alt_ = value;
bitField0_ |= 0x00000008;
onChanged();
return this;
}
/**
* double alt = 4;
* @return This builder for chaining.
*/
public Builder clearAlt() {
bitField0_ = (bitField0_ & ~0x00000008);
alt_ = 0D;
onChanged();
return this;
}
private java.lang.Object desc_ = "";
/**
* string desc = 5;
* @return The desc.
*/
public java.lang.String getDesc() {
java.lang.Object ref = desc_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
desc_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* string desc = 5;
* @return The bytes for desc.
*/
public com.google.protobuf.ByteString
getDescBytes() {
java.lang.Object ref = desc_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
desc_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* string desc = 5;
* @param value The desc to set.
* @return This builder for chaining.
*/
public Builder setDesc(
java.lang.String value) {
if (value == null) { throw new NullPointerException(); }
desc_ = value;
bitField0_ |= 0x00000010;
onChanged();
return this;
}
/**
* string desc = 5;
* @return This builder for chaining.
*/
public Builder clearDesc() {
desc_ = getDefaultInstance().getDesc();
bitField0_ = (bitField0_ & ~0x00000010);
onChanged();
return this;
}
/**
* string desc = 5;
* @param value The bytes for desc to set.
* @return This builder for chaining.
*/
public Builder setDescBytes(
com.google.protobuf.ByteString value) {
if (value == null) { throw new NullPointerException(); }
checkByteStringIsUtf8(value);
desc_ = value;
bitField0_ |= 0x00000010;
onChanged();
return this;
}
private java.lang.Object wmoId_ = "";
/**
* string wmoId = 6;
* @return The wmoId.
*/
public java.lang.String getWmoId() {
java.lang.Object ref = wmoId_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
wmoId_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* string wmoId = 6;
* @return The bytes for wmoId.
*/
public com.google.protobuf.ByteString
getWmoIdBytes() {
java.lang.Object ref = wmoId_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
wmoId_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* string wmoId = 6;
* @param value The wmoId to set.
* @return This builder for chaining.
*/
public Builder setWmoId(
java.lang.String value) {
if (value == null) { throw new NullPointerException(); }
wmoId_ = value;
bitField0_ |= 0x00000020;
onChanged();
return this;
}
/**
* string wmoId = 6;
* @return This builder for chaining.
*/
public Builder clearWmoId() {
wmoId_ = getDefaultInstance().getWmoId();
bitField0_ = (bitField0_ & ~0x00000020);
onChanged();
return this;
}
/**
* string wmoId = 6;
* @param value The bytes for wmoId to set.
* @return This builder for chaining.
*/
public Builder setWmoIdBytes(
com.google.protobuf.ByteString value) {
if (value == null) { throw new NullPointerException(); }
checkByteStringIsUtf8(value);
wmoId_ = value;
bitField0_ |= 0x00000020;
onChanged();
return this;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:Station)
}
// @@protoc_insertion_point(class_scope:Station)
private static final ucar.nc2.ft.point.remote.PointStreamProto.Station DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new ucar.nc2.ft.point.remote.PointStreamProto.Station();
}
public static ucar.nc2.ft.point.remote.PointStreamProto.Station getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public Station parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
Builder builder = newBuilder();
try {
builder.mergeFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(builder.buildPartial());
} catch (com.google.protobuf.UninitializedMessageException e) {
throw e.asInvalidProtocolBufferException().setUnfinishedMessage(builder.buildPartial());
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(e)
.setUnfinishedMessage(builder.buildPartial());
}
return builder.buildPartial();
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public ucar.nc2.ft.point.remote.PointStreamProto.Station getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface StationListOrBuilder extends
// @@protoc_insertion_point(interface_extends:StationList)
com.google.protobuf.MessageOrBuilder {
/**
* repeated .Station stations = 1;
*/
java.util.List
getStationsList();
/**
* repeated .Station stations = 1;
*/
ucar.nc2.ft.point.remote.PointStreamProto.Station getStations(int index);
/**
* repeated .Station stations = 1;
*/
int getStationsCount();
/**
* repeated .Station stations = 1;
*/
java.util.List extends ucar.nc2.ft.point.remote.PointStreamProto.StationOrBuilder>
getStationsOrBuilderList();
/**
* repeated .Station stations = 1;
*/
ucar.nc2.ft.point.remote.PointStreamProto.StationOrBuilder getStationsOrBuilder(
int index);
}
/**
* Protobuf type {@code StationList}
*/
public static final class StationList extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:StationList)
StationListOrBuilder {
private static final long serialVersionUID = 0L;
// Use StationList.newBuilder() to construct.
private StationList(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private StationList() {
stations_ = java.util.Collections.emptyList();
}
@java.lang.Override
@SuppressWarnings({"unused"})
protected java.lang.Object newInstance(
UnusedPrivateParameter unused) {
return new StationList();
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return ucar.nc2.ft.point.remote.PointStreamProto.internal_static_StationList_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return ucar.nc2.ft.point.remote.PointStreamProto.internal_static_StationList_fieldAccessorTable
.ensureFieldAccessorsInitialized(
ucar.nc2.ft.point.remote.PointStreamProto.StationList.class, ucar.nc2.ft.point.remote.PointStreamProto.StationList.Builder.class);
}
public static final int STATIONS_FIELD_NUMBER = 1;
@SuppressWarnings("serial")
private java.util.List stations_;
/**
* repeated .Station stations = 1;
*/
@java.lang.Override
public java.util.List getStationsList() {
return stations_;
}
/**
* repeated .Station stations = 1;
*/
@java.lang.Override
public java.util.List extends ucar.nc2.ft.point.remote.PointStreamProto.StationOrBuilder>
getStationsOrBuilderList() {
return stations_;
}
/**
* repeated .Station stations = 1;
*/
@java.lang.Override
public int getStationsCount() {
return stations_.size();
}
/**
* repeated .Station stations = 1;
*/
@java.lang.Override
public ucar.nc2.ft.point.remote.PointStreamProto.Station getStations(int index) {
return stations_.get(index);
}
/**
* repeated .Station stations = 1;
*/
@java.lang.Override
public ucar.nc2.ft.point.remote.PointStreamProto.StationOrBuilder getStationsOrBuilder(
int index) {
return stations_.get(index);
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
for (int i = 0; i < stations_.size(); i++) {
output.writeMessage(1, stations_.get(i));
}
getUnknownFields().writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
for (int i = 0; i < stations_.size(); i++) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(1, stations_.get(i));
}
size += getUnknownFields().getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof ucar.nc2.ft.point.remote.PointStreamProto.StationList)) {
return super.equals(obj);
}
ucar.nc2.ft.point.remote.PointStreamProto.StationList other = (ucar.nc2.ft.point.remote.PointStreamProto.StationList) obj;
if (!getStationsList()
.equals(other.getStationsList())) return false;
if (!getUnknownFields().equals(other.getUnknownFields())) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
if (getStationsCount() > 0) {
hash = (37 * hash) + STATIONS_FIELD_NUMBER;
hash = (53 * hash) + getStationsList().hashCode();
}
hash = (29 * hash) + getUnknownFields().hashCode();
memoizedHashCode = hash;
return hash;
}
public static ucar.nc2.ft.point.remote.PointStreamProto.StationList parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static ucar.nc2.ft.point.remote.PointStreamProto.StationList parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static ucar.nc2.ft.point.remote.PointStreamProto.StationList parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static ucar.nc2.ft.point.remote.PointStreamProto.StationList parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static ucar.nc2.ft.point.remote.PointStreamProto.StationList parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static ucar.nc2.ft.point.remote.PointStreamProto.StationList parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static ucar.nc2.ft.point.remote.PointStreamProto.StationList parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static ucar.nc2.ft.point.remote.PointStreamProto.StationList parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static ucar.nc2.ft.point.remote.PointStreamProto.StationList parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static ucar.nc2.ft.point.remote.PointStreamProto.StationList parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static ucar.nc2.ft.point.remote.PointStreamProto.StationList parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static ucar.nc2.ft.point.remote.PointStreamProto.StationList parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(ucar.nc2.ft.point.remote.PointStreamProto.StationList prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code StationList}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:StationList)
ucar.nc2.ft.point.remote.PointStreamProto.StationListOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return ucar.nc2.ft.point.remote.PointStreamProto.internal_static_StationList_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return ucar.nc2.ft.point.remote.PointStreamProto.internal_static_StationList_fieldAccessorTable
.ensureFieldAccessorsInitialized(
ucar.nc2.ft.point.remote.PointStreamProto.StationList.class, ucar.nc2.ft.point.remote.PointStreamProto.StationList.Builder.class);
}
// Construct using ucar.nc2.ft.point.remote.PointStreamProto.StationList.newBuilder()
private Builder() {
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
}
@java.lang.Override
public Builder clear() {
super.clear();
bitField0_ = 0;
if (stationsBuilder_ == null) {
stations_ = java.util.Collections.emptyList();
} else {
stations_ = null;
stationsBuilder_.clear();
}
bitField0_ = (bitField0_ & ~0x00000001);
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return ucar.nc2.ft.point.remote.PointStreamProto.internal_static_StationList_descriptor;
}
@java.lang.Override
public ucar.nc2.ft.point.remote.PointStreamProto.StationList getDefaultInstanceForType() {
return ucar.nc2.ft.point.remote.PointStreamProto.StationList.getDefaultInstance();
}
@java.lang.Override
public ucar.nc2.ft.point.remote.PointStreamProto.StationList build() {
ucar.nc2.ft.point.remote.PointStreamProto.StationList result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public ucar.nc2.ft.point.remote.PointStreamProto.StationList buildPartial() {
ucar.nc2.ft.point.remote.PointStreamProto.StationList result = new ucar.nc2.ft.point.remote.PointStreamProto.StationList(this);
buildPartialRepeatedFields(result);
if (bitField0_ != 0) { buildPartial0(result); }
onBuilt();
return result;
}
private void buildPartialRepeatedFields(ucar.nc2.ft.point.remote.PointStreamProto.StationList result) {
if (stationsBuilder_ == null) {
if (((bitField0_ & 0x00000001) != 0)) {
stations_ = java.util.Collections.unmodifiableList(stations_);
bitField0_ = (bitField0_ & ~0x00000001);
}
result.stations_ = stations_;
} else {
result.stations_ = stationsBuilder_.build();
}
}
private void buildPartial0(ucar.nc2.ft.point.remote.PointStreamProto.StationList result) {
int from_bitField0_ = bitField0_;
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof ucar.nc2.ft.point.remote.PointStreamProto.StationList) {
return mergeFrom((ucar.nc2.ft.point.remote.PointStreamProto.StationList)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(ucar.nc2.ft.point.remote.PointStreamProto.StationList other) {
if (other == ucar.nc2.ft.point.remote.PointStreamProto.StationList.getDefaultInstance()) return this;
if (stationsBuilder_ == null) {
if (!other.stations_.isEmpty()) {
if (stations_.isEmpty()) {
stations_ = other.stations_;
bitField0_ = (bitField0_ & ~0x00000001);
} else {
ensureStationsIsMutable();
stations_.addAll(other.stations_);
}
onChanged();
}
} else {
if (!other.stations_.isEmpty()) {
if (stationsBuilder_.isEmpty()) {
stationsBuilder_.dispose();
stationsBuilder_ = null;
stations_ = other.stations_;
bitField0_ = (bitField0_ & ~0x00000001);
stationsBuilder_ =
com.google.protobuf.GeneratedMessageV3.alwaysUseFieldBuilders ?
getStationsFieldBuilder() : null;
} else {
stationsBuilder_.addAllMessages(other.stations_);
}
}
}
this.mergeUnknownFields(other.getUnknownFields());
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 10: {
ucar.nc2.ft.point.remote.PointStreamProto.Station m =
input.readMessage(
ucar.nc2.ft.point.remote.PointStreamProto.Station.parser(),
extensionRegistry);
if (stationsBuilder_ == null) {
ensureStationsIsMutable();
stations_.add(m);
} else {
stationsBuilder_.addMessage(m);
}
break;
} // case 10
default: {
if (!super.parseUnknownField(input, extensionRegistry, tag)) {
done = true; // was an endgroup tag
}
break;
} // default:
} // switch (tag)
} // while (!done)
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.unwrapIOException();
} finally {
onChanged();
} // finally
return this;
}
private int bitField0_;
private java.util.List stations_ =
java.util.Collections.emptyList();
private void ensureStationsIsMutable() {
if (!((bitField0_ & 0x00000001) != 0)) {
stations_ = new java.util.ArrayList(stations_);
bitField0_ |= 0x00000001;
}
}
private com.google.protobuf.RepeatedFieldBuilderV3<
ucar.nc2.ft.point.remote.PointStreamProto.Station, ucar.nc2.ft.point.remote.PointStreamProto.Station.Builder, ucar.nc2.ft.point.remote.PointStreamProto.StationOrBuilder> stationsBuilder_;
/**
* repeated .Station stations = 1;
*/
public java.util.List getStationsList() {
if (stationsBuilder_ == null) {
return java.util.Collections.unmodifiableList(stations_);
} else {
return stationsBuilder_.getMessageList();
}
}
/**
* repeated .Station stations = 1;
*/
public int getStationsCount() {
if (stationsBuilder_ == null) {
return stations_.size();
} else {
return stationsBuilder_.getCount();
}
}
/**
* repeated .Station stations = 1;
*/
public ucar.nc2.ft.point.remote.PointStreamProto.Station getStations(int index) {
if (stationsBuilder_ == null) {
return stations_.get(index);
} else {
return stationsBuilder_.getMessage(index);
}
}
/**
* repeated .Station stations = 1;
*/
public Builder setStations(
int index, ucar.nc2.ft.point.remote.PointStreamProto.Station value) {
if (stationsBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureStationsIsMutable();
stations_.set(index, value);
onChanged();
} else {
stationsBuilder_.setMessage(index, value);
}
return this;
}
/**
* repeated .Station stations = 1;
*/
public Builder setStations(
int index, ucar.nc2.ft.point.remote.PointStreamProto.Station.Builder builderForValue) {
if (stationsBuilder_ == null) {
ensureStationsIsMutable();
stations_.set(index, builderForValue.build());
onChanged();
} else {
stationsBuilder_.setMessage(index, builderForValue.build());
}
return this;
}
/**
* repeated .Station stations = 1;
*/
public Builder addStations(ucar.nc2.ft.point.remote.PointStreamProto.Station value) {
if (stationsBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureStationsIsMutable();
stations_.add(value);
onChanged();
} else {
stationsBuilder_.addMessage(value);
}
return this;
}
/**
* repeated .Station stations = 1;
*/
public Builder addStations(
int index, ucar.nc2.ft.point.remote.PointStreamProto.Station value) {
if (stationsBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureStationsIsMutable();
stations_.add(index, value);
onChanged();
} else {
stationsBuilder_.addMessage(index, value);
}
return this;
}
/**
* repeated .Station stations = 1;
*/
public Builder addStations(
ucar.nc2.ft.point.remote.PointStreamProto.Station.Builder builderForValue) {
if (stationsBuilder_ == null) {
ensureStationsIsMutable();
stations_.add(builderForValue.build());
onChanged();
} else {
stationsBuilder_.addMessage(builderForValue.build());
}
return this;
}
/**
* repeated .Station stations = 1;
*/
public Builder addStations(
int index, ucar.nc2.ft.point.remote.PointStreamProto.Station.Builder builderForValue) {
if (stationsBuilder_ == null) {
ensureStationsIsMutable();
stations_.add(index, builderForValue.build());
onChanged();
} else {
stationsBuilder_.addMessage(index, builderForValue.build());
}
return this;
}
/**
* repeated .Station stations = 1;
*/
public Builder addAllStations(
java.lang.Iterable extends ucar.nc2.ft.point.remote.PointStreamProto.Station> values) {
if (stationsBuilder_ == null) {
ensureStationsIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(
values, stations_);
onChanged();
} else {
stationsBuilder_.addAllMessages(values);
}
return this;
}
/**
* repeated .Station stations = 1;
*/
public Builder clearStations() {
if (stationsBuilder_ == null) {
stations_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000001);
onChanged();
} else {
stationsBuilder_.clear();
}
return this;
}
/**
* repeated .Station stations = 1;
*/
public Builder removeStations(int index) {
if (stationsBuilder_ == null) {
ensureStationsIsMutable();
stations_.remove(index);
onChanged();
} else {
stationsBuilder_.remove(index);
}
return this;
}
/**
* repeated .Station stations = 1;
*/
public ucar.nc2.ft.point.remote.PointStreamProto.Station.Builder getStationsBuilder(
int index) {
return getStationsFieldBuilder().getBuilder(index);
}
/**
* repeated .Station stations = 1;
*/
public ucar.nc2.ft.point.remote.PointStreamProto.StationOrBuilder getStationsOrBuilder(
int index) {
if (stationsBuilder_ == null) {
return stations_.get(index); } else {
return stationsBuilder_.getMessageOrBuilder(index);
}
}
/**
* repeated .Station stations = 1;
*/
public java.util.List extends ucar.nc2.ft.point.remote.PointStreamProto.StationOrBuilder>
getStationsOrBuilderList() {
if (stationsBuilder_ != null) {
return stationsBuilder_.getMessageOrBuilderList();
} else {
return java.util.Collections.unmodifiableList(stations_);
}
}
/**
* repeated .Station stations = 1;
*/
public ucar.nc2.ft.point.remote.PointStreamProto.Station.Builder addStationsBuilder() {
return getStationsFieldBuilder().addBuilder(
ucar.nc2.ft.point.remote.PointStreamProto.Station.getDefaultInstance());
}
/**
* repeated .Station stations = 1;
*/
public ucar.nc2.ft.point.remote.PointStreamProto.Station.Builder addStationsBuilder(
int index) {
return getStationsFieldBuilder().addBuilder(
index, ucar.nc2.ft.point.remote.PointStreamProto.Station.getDefaultInstance());
}
/**
* repeated .Station stations = 1;
*/
public java.util.List
getStationsBuilderList() {
return getStationsFieldBuilder().getBuilderList();
}
private com.google.protobuf.RepeatedFieldBuilderV3<
ucar.nc2.ft.point.remote.PointStreamProto.Station, ucar.nc2.ft.point.remote.PointStreamProto.Station.Builder, ucar.nc2.ft.point.remote.PointStreamProto.StationOrBuilder>
getStationsFieldBuilder() {
if (stationsBuilder_ == null) {
stationsBuilder_ = new com.google.protobuf.RepeatedFieldBuilderV3<
ucar.nc2.ft.point.remote.PointStreamProto.Station, ucar.nc2.ft.point.remote.PointStreamProto.Station.Builder, ucar.nc2.ft.point.remote.PointStreamProto.StationOrBuilder>(
stations_,
((bitField0_ & 0x00000001) != 0),
getParentForChildren(),
isClean());
stations_ = null;
}
return stationsBuilder_;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:StationList)
}
// @@protoc_insertion_point(class_scope:StationList)
private static final ucar.nc2.ft.point.remote.PointStreamProto.StationList DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new ucar.nc2.ft.point.remote.PointStreamProto.StationList();
}
public static ucar.nc2.ft.point.remote.PointStreamProto.StationList getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public StationList parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
Builder builder = newBuilder();
try {
builder.mergeFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(builder.buildPartial());
} catch (com.google.protobuf.UninitializedMessageException e) {
throw e.asInvalidProtocolBufferException().setUnfinishedMessage(builder.buildPartial());
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(e)
.setUnfinishedMessage(builder.buildPartial());
}
return builder.buildPartial();
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public ucar.nc2.ft.point.remote.PointStreamProto.StationList getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_Location_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_Location_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_PointFeature_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_PointFeature_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_PointFeatureMember_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_PointFeatureMember_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_PointFeatureCollection_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_PointFeatureCollection_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_Station_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_Station_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_StationList_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_StationList_fieldAccessorTable;
public static com.google.protobuf.Descriptors.FileDescriptor
getDescriptor() {
return descriptor;
}
private static com.google.protobuf.Descriptors.FileDescriptor
descriptor;
static {
java.lang.String[] descriptorData = {
"\n\021pointStream.proto\032\016ncStream.proto\"P\n\010L" +
"ocation\022\014\n\004time\030\001 \001(\001\022\013\n\003lat\030\002 \001(\001\022\013\n\003lo" +
"n\030\003 \001(\001\022\013\n\003alt\030\004 \001(\001\022\017\n\007nomTime\030\005 \001(\001\"C\n" +
"\014PointFeature\022\026\n\003loc\030\001 \001(\0132\t.Location\022\014\n" +
"\004data\030\003 \001(\014\022\r\n\005sdata\030\004 \003(\t\"w\n\022PointFeatu" +
"reMember\022\014\n\004name\030\001 \001(\t\022\014\n\004desc\030\002 \001(\t\022\r\n\005" +
"units\030\003 \001(\t\022\033\n\010dataType\030\004 \001(\0162\t.DataType" +
"\022\031\n\007section\030\005 \001(\0132\010.Section\"o\n\026PointFeat" +
"ureCollection\022\014\n\004name\030\001 \001(\t\022\020\n\010timeUnit\030" +
"\002 \001(\t\022$\n\007members\030\003 \003(\0132\023.PointFeatureMem" +
"ber\022\017\n\007altUnit\030\004 \001(\t\"Y\n\007Station\022\n\n\002id\030\001 " +
"\001(\t\022\013\n\003lat\030\002 \001(\001\022\013\n\003lon\030\003 \001(\001\022\013\n\003alt\030\004 \001" +
"(\001\022\014\n\004desc\030\005 \001(\t\022\r\n\005wmoId\030\006 \001(\t\")\n\013Stati" +
"onList\022\032\n\010stations\030\001 \003(\0132\010.StationB,\n\030uc" +
"ar.nc2.ft.point.remoteB\020PointStreamProto" +
"b\006proto3"
};
descriptor = com.google.protobuf.Descriptors.FileDescriptor
.internalBuildGeneratedFileFrom(descriptorData,
new com.google.protobuf.Descriptors.FileDescriptor[] {
ucar.nc2.stream.NcStreamProto.getDescriptor(),
});
internal_static_Location_descriptor =
getDescriptor().getMessageTypes().get(0);
internal_static_Location_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_Location_descriptor,
new java.lang.String[] { "Time", "Lat", "Lon", "Alt", "NomTime", });
internal_static_PointFeature_descriptor =
getDescriptor().getMessageTypes().get(1);
internal_static_PointFeature_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_PointFeature_descriptor,
new java.lang.String[] { "Loc", "Data", "Sdata", });
internal_static_PointFeatureMember_descriptor =
getDescriptor().getMessageTypes().get(2);
internal_static_PointFeatureMember_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_PointFeatureMember_descriptor,
new java.lang.String[] { "Name", "Desc", "Units", "DataType", "Section", });
internal_static_PointFeatureCollection_descriptor =
getDescriptor().getMessageTypes().get(3);
internal_static_PointFeatureCollection_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_PointFeatureCollection_descriptor,
new java.lang.String[] { "Name", "TimeUnit", "Members", "AltUnit", });
internal_static_Station_descriptor =
getDescriptor().getMessageTypes().get(4);
internal_static_Station_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_Station_descriptor,
new java.lang.String[] { "Id", "Lat", "Lon", "Alt", "Desc", "WmoId", });
internal_static_StationList_descriptor =
getDescriptor().getMessageTypes().get(5);
internal_static_StationList_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_StationList_descriptor,
new java.lang.String[] { "Stations", });
ucar.nc2.stream.NcStreamProto.getDescriptor();
}
// @@protoc_insertion_point(outer_class_scope)
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy