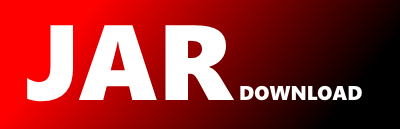
ucar.units.Prefix Maven / Gradle / Ivy
The newest version!
/*
* Copyright (c) 1998-2018 University Corporation for Atmospheric Research/Unidata
* See LICENSE for license information.
*/
package ucar.units;
import javax.annotation.concurrent.Immutable;
/**
* Provides support for unit prefixes (e.g. "centi", "c").
*
* Instances of this class are immutable.
*
* @author Steven R. Emmerson
*/
@Immutable
public abstract class Prefix implements Comparable
© 2015 - 2025 Weber Informatics LLC | Privacy Policy