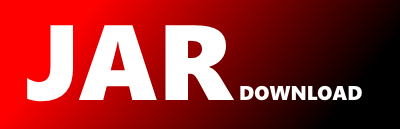
ucar.units.StandardUnitFormatTokenManager Maven / Gradle / Ivy
The newest version!
/*
* Copyright (c) 1998-2018 University Corporation for Atmospheric Research/Unidata
* See LICENSE for license information.
*/
/* Generated By:JavaCC: Do not edit this line. StandardUnitFormatTokenManager.java */
package ucar.units;
/** Token Manager. */
public class StandardUnitFormatTokenManager implements StandardUnitFormatConstants {
/** Debug output. */
public java.io.PrintStream debugStream = System.out;
/** Set debug output. */
public void setDebugStream(java.io.PrintStream ds) {
debugStream = ds;
}
private final int jjStopStringLiteralDfa_0(int pos, long active0) {
switch (pos) {
default:
return -1;
}
}
private final int jjStartNfa_0(int pos, long active0) {
return jjMoveNfa_0(jjStopStringLiteralDfa_0(pos, active0), pos + 1);
}
private int jjStopAtPos(int pos, int kind) {
jjmatchedKind = kind;
jjmatchedPos = pos;
return pos + 1;
}
private int jjMoveStringLiteralDfa0_0() {
switch (curChar) {
case 40:
return jjStopAtPos(0, 8);
case 41:
return jjStopAtPos(0, 9);
case 42:
return jjStopAtPos(0, 13);
case 43:
return jjStopAtPos(0, 2);
case 45:
return jjStopAtPos(0, 3);
case 46:
return jjStopAtPos(0, 12);
case 58:
return jjStopAtPos(0, 4);
case 84:
return jjStartNfaWithStates_0(0, 17, 49);
case 94:
return jjStopAtPos(0, 11);
case 116:
return jjStartNfaWithStates_0(0, 17, 49);
default:
return jjMoveNfa_0(1, 0);
}
}
private int jjStartNfaWithStates_0(int pos, int kind, int state) {
jjmatchedKind = kind;
jjmatchedPos = pos;
try {
curChar = input_stream.readChar();
} catch (java.io.IOException e) {
return pos + 1;
}
return jjMoveNfa_0(state, pos + 1);
}
private int jjMoveNfa_0(int startState, int curPos) {
int startsAt = 0;
jjnewStateCnt = 49;
int i = 1;
jjstateSet[0] = startState;
int kind = 0x7fffffff;
for (;;) {
if (++jjround == 0x7fffffff)
ReInitRounds();
if (curChar < 64) {
long l = 1L << curChar;
do {
switch (jjstateSet[--i]) {
case 1:
if ((0x3ff000000000000L & l) != 0L) {
if (kind > 5)
kind = 5;
jjCheckNAdd(0);
} else if ((0x100002600L & l) != 0L) {
if (kind > 1)
kind = 1;
jjCheckNAddStates(0, 7);
} else if ((0xa400000000L & l) != 0L) {
if (kind > 16)
kind = 16;
} else if (curChar == 47) {
if (kind > 14)
kind = 14;
}
break;
case 49:
case 9:
if ((0x3ff000000000000L & l) != 0L)
jjCheckNAddTwoStates(9, 10);
break;
case 0:
if ((0x3ff000000000000L & l) == 0L)
break;
if (kind > 5)
kind = 5;
jjCheckNAdd(0);
break;
case 2:
if ((0x280000000000L & l) != 0L)
jjCheckNAdd(3);
break;
case 3:
if ((0x3ff000000000000L & l) == 0L)
break;
if (kind > 10)
kind = 10;
jjCheckNAdd(3);
break;
case 4:
if (curChar == 47)
kind = 14;
break;
case 6:
if ((0x100002600L & l) == 0L)
break;
if (kind > 15)
kind = 15;
jjstateSet[jjnewStateCnt++] = 6;
break;
case 7:
if ((0xa400000000L & l) != 0L)
kind = 16;
break;
case 11:
if ((0x100002600L & l) == 0L)
break;
if (kind > 1)
kind = 1;
jjCheckNAddStates(0, 7);
break;
case 12:
if ((0x100002600L & l) == 0L)
break;
if (kind > 1)
kind = 1;
jjCheckNAdd(12);
break;
case 13:
if ((0x100002600L & l) != 0L)
jjCheckNAddTwoStates(13, 17);
break;
case 15:
if ((0x100002600L & l) == 0L)
break;
if (kind > 14)
kind = 14;
jjstateSet[jjnewStateCnt++] = 15;
break;
case 18:
if ((0x100002600L & l) != 0L)
jjCheckNAddTwoStates(18, 5);
break;
case 19:
if ((0x100002600L & l) != 0L)
jjCheckNAddStates(8, 10);
break;
case 21:
if ((0x100002600L & l) == 0L)
break;
if (kind > 15)
kind = 15;
jjstateSet[jjnewStateCnt++] = 21;
break;
case 32:
if (curChar != 58)
break;
if (kind > 19)
kind = 19;
jjCheckNAdd(33);
break;
case 33:
if ((0x100002600L & l) == 0L)
break;
if (kind > 19)
kind = 19;
jjCheckNAdd(33);
break;
case 35:
if (curChar == 40)
jjstateSet[jjnewStateCnt++] = 34;
break;
case 38:
if (curChar != 58)
break;
if (kind > 20)
kind = 20;
jjCheckNAdd(39);
break;
case 39:
if ((0x100002600L & l) == 0L)
break;
if (kind > 20)
kind = 20;
jjCheckNAdd(39);
break;
case 41:
if (curChar == 40)
jjstateSet[jjnewStateCnt++] = 40;
break;
case 44:
if (curChar != 58)
break;
if (kind > 21)
kind = 21;
jjCheckNAdd(45);
break;
case 45:
if ((0x100002600L & l) == 0L)
break;
if (kind > 21)
kind = 21;
jjCheckNAdd(45);
break;
case 47:
if (curChar == 40)
jjstateSet[jjnewStateCnt++] = 46;
break;
default:
break;
}
} while (i != startsAt);
} else if (curChar < 128) {
long l = 1L << (curChar & 077);
do {
switch (jjstateSet[--i]) {
case 1:
if ((0x7fffffe87fffffeL & l) != 0L) {
if (kind > 18)
kind = 18;
jjCheckNAddTwoStates(8, 9);
} else if (curChar == 64) {
if (kind > 15)
kind = 15;
jjstateSet[jjnewStateCnt++] = 6;
}
if ((0x100000001000L & l) != 0L)
jjAddStates(11, 13);
else if ((0x2000000020L & l) != 0L)
jjAddStates(14, 15);
break;
case 49:
case 8:
if ((0x7fffffe87fffffeL & l) == 0L)
break;
if (kind > 18)
kind = 18;
jjCheckNAddTwoStates(8, 9);
break;
case 5:
if (curChar != 64)
break;
kind = 15;
jjstateSet[jjnewStateCnt++] = 6;
break;
case 10:
if ((0x7fffffe87fffffeL & l) == 0L)
break;
if (kind > 18)
kind = 18;
jjstateSet[jjnewStateCnt++] = 10;
break;
case 14:
if ((0x4000000040000L & l) != 0L)
jjstateSet[jjnewStateCnt++] = 15;
break;
case 16:
if ((0x2000000020L & l) != 0L)
jjstateSet[jjnewStateCnt++] = 14;
break;
case 17:
if ((0x1000000010000L & l) != 0L)
jjstateSet[jjnewStateCnt++] = 16;
break;
case 20:
if ((0x2000000020L & l) != 0L)
jjCheckNAdd(21);
break;
case 22:
if ((0x800000008L & l) != 0L)
jjstateSet[jjnewStateCnt++] = 20;
break;
case 23:
if ((0x400000004000L & l) != 0L)
jjstateSet[jjnewStateCnt++] = 22;
break;
case 24:
if ((0x20000000200L & l) != 0L)
jjstateSet[jjnewStateCnt++] = 23;
break;
case 25:
if ((0x8000000080000L & l) != 0L)
jjstateSet[jjnewStateCnt++] = 24;
break;
case 26:
if ((0x200000002000L & l) != 0L)
jjCheckNAdd(21);
break;
case 27:
if ((0x800000008000L & l) != 0L)
jjstateSet[jjnewStateCnt++] = 26;
break;
case 28:
if ((0x4000000040000L & l) != 0L)
jjstateSet[jjnewStateCnt++] = 27;
break;
case 29:
if ((0x4000000040L & l) != 0L)
jjstateSet[jjnewStateCnt++] = 28;
break;
case 30:
if ((0x100000001000L & l) != 0L)
jjAddStates(11, 13);
break;
case 31:
if ((0x2000000020L & l) == 0L)
break;
if (kind > 19)
kind = 19;
jjAddStates(16, 17);
break;
case 34:
if ((0x4000000040000L & l) != 0L)
jjstateSet[jjnewStateCnt++] = 31;
break;
case 36:
if ((0x400000004L & l) != 0L)
jjstateSet[jjnewStateCnt++] = 35;
break;
case 37:
if ((0x2000000020L & l) == 0L)
break;
if (kind > 20)
kind = 20;
jjAddStates(18, 19);
break;
case 40:
if ((0x4000000040000L & l) != 0L)
jjstateSet[jjnewStateCnt++] = 37;
break;
case 42:
if ((0x400000004000L & l) != 0L)
jjstateSet[jjnewStateCnt++] = 41;
break;
case 43:
if ((0x2000000020L & l) == 0L)
break;
if (kind > 21)
kind = 21;
jjAddStates(20, 21);
break;
case 46:
if ((0x4000000040000L & l) != 0L)
jjstateSet[jjnewStateCnt++] = 43;
break;
case 48:
if ((0x8000000080L & l) != 0L)
jjstateSet[jjnewStateCnt++] = 47;
break;
default:
break;
}
} while (i != startsAt);
} else {
int i2 = (curChar & 0xff) >> 6;
long l2 = 1L << (curChar & 077);
do {
switch (jjstateSet[--i]) {
default:
break;
}
} while (i != startsAt);
}
if (kind != 0x7fffffff) {
jjmatchedKind = kind;
jjmatchedPos = curPos;
kind = 0x7fffffff;
}
++curPos;
if ((i = jjnewStateCnt) == (startsAt = 49 - (jjnewStateCnt = startsAt)))
return curPos;
try {
curChar = input_stream.readChar();
} catch (java.io.IOException e) {
return curPos;
}
}
}
static final int[] jjnextStates =
{12, 13, 17, 18, 5, 19, 25, 29, 19, 25, 29, 36, 42, 48, 2, 3, 32, 33, 38, 39, 44, 45,};
/** Token literal values. */
public static final String[] jjstrLiteralImages = {"", null, "\53", "\55", "\72", null, null, null, "\50", "\51",
null, "\136", "\56", "\52", null, null, null, null, null, null, null, null,};
/** Lexer state names. */
public static final String[] lexStateNames = {"DEFAULT",};
protected SimpleCharStream input_stream;
private final int[] jjrounds = new int[49];
private final int[] jjstateSet = new int[98];
protected char curChar;
/** Constructor. */
public StandardUnitFormatTokenManager(SimpleCharStream stream) {
if (SimpleCharStream.staticFlag)
throw new Error("ERROR: Cannot use a static CharStream class with a non-static lexical analyzer.");
input_stream = stream;
}
/** Constructor. */
public StandardUnitFormatTokenManager(SimpleCharStream stream, int lexState) {
this(stream);
SwitchTo(lexState);
}
/** Reinitialise parser. */
public void ReInit(SimpleCharStream stream) {
jjmatchedPos = jjnewStateCnt = 0;
curLexState = defaultLexState;
input_stream = stream;
ReInitRounds();
}
private void ReInitRounds() {
int i;
jjround = 0x80000001;
for (i = 49; i-- > 0;)
jjrounds[i] = 0x80000000;
}
/** Reinitialise parser. */
public void ReInit(SimpleCharStream stream, int lexState) {
ReInit(stream);
SwitchTo(lexState);
}
/** Switch to specified lex state. */
public void SwitchTo(int lexState) {
if (lexState >= 1 || lexState < 0)
throw new TokenMgrError("Error: Ignoring invalid lexical state : " + lexState + ". State unchanged.",
TokenMgrError.INVALID_LEXICAL_STATE);
else
curLexState = lexState;
}
protected Token jjFillToken() {
final Token t;
final String curTokenImage;
final int beginLine;
final int endLine;
final int beginColumn;
final int endColumn;
String im = jjstrLiteralImages[jjmatchedKind];
curTokenImage = (im == null) ? input_stream.GetImage() : im;
beginLine = input_stream.getBeginLine();
beginColumn = input_stream.getBeginColumn();
endLine = input_stream.getEndLine();
endColumn = input_stream.getEndColumn();
t = Token.newToken(jjmatchedKind, curTokenImage);
t.beginLine = beginLine;
t.endLine = endLine;
t.beginColumn = beginColumn;
t.endColumn = endColumn;
return t;
}
int curLexState = 0;
int defaultLexState = 0;
int jjnewStateCnt;
int jjround;
int jjmatchedPos;
int jjmatchedKind;
/** Get the next Token. */
public Token getNextToken() {
Token matchedToken;
int curPos;
EOFLoop: for (;;) {
try {
curChar = input_stream.BeginToken();
} catch (java.io.IOException e) {
jjmatchedKind = 0;
matchedToken = jjFillToken();
return matchedToken;
}
jjmatchedKind = 0x7fffffff;
jjmatchedPos = 0;
curPos = jjMoveStringLiteralDfa0_0();
if (jjmatchedKind != 0x7fffffff) {
if (jjmatchedPos + 1 < curPos)
input_stream.backup(curPos - jjmatchedPos - 1);
matchedToken = jjFillToken();
return matchedToken;
}
int error_line = input_stream.getEndLine();
int error_column = input_stream.getEndColumn();
String error_after = null;
boolean EOFSeen = false;
try {
input_stream.readChar();
input_stream.backup(1);
} catch (java.io.IOException e1) {
EOFSeen = true;
error_after = curPos <= 1 ? "" : input_stream.GetImage();
if (curChar == '\n' || curChar == '\r') {
error_line++;
error_column = 0;
} else
error_column++;
}
if (!EOFSeen) {
input_stream.backup(1);
error_after = curPos <= 1 ? "" : input_stream.GetImage();
}
throw new TokenMgrError(EOFSeen, curLexState, error_line, error_column, error_after, curChar,
TokenMgrError.LEXICAL_ERROR);
}
}
private void jjCheckNAdd(int state) {
if (jjrounds[state] != jjround) {
jjstateSet[jjnewStateCnt++] = state;
jjrounds[state] = jjround;
}
}
private void jjAddStates(int start, int end) {
do {
jjstateSet[jjnewStateCnt++] = jjnextStates[start];
} while (start++ != end);
}
private void jjCheckNAddTwoStates(int state1, int state2) {
jjCheckNAdd(state1);
jjCheckNAdd(state2);
}
private void jjCheckNAddStates(int start, int end) {
do {
jjCheckNAdd(jjnextStates[start]);
} while (start++ != end);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy