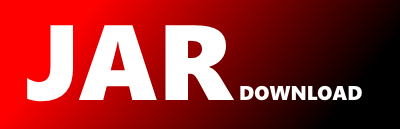
org.gecko.adapter.mqtt.common.GeckoMqttClient Maven / Gradle / Ivy
/*
* Copyright (c) 2012 - 2024 Data In Motion and others.
* All rights reserved.
*
* This program and the accompanying materials are made
* available under the terms of the Eclipse Public License 2.0
* which is available at https://www.eclipse.org/legal/epl-2.0/
* SPDX-License-Identifier: EPL-2.0
*
* Contributors:
* Data In Motion - initial API and implementation
*/
package org.gecko.adapter.mqtt.common;
import java.util.function.Consumer;
import java.util.function.Function;
import org.gecko.adapter.mqtt.MqttConfig;
import org.osgi.util.pushstream.PushEventSource;
/**
* Facade for different implementations or versions of a MQTT client
*/
public interface GeckoMqttClient {
static final String DEFAULT_PASSWORD = "guest"; //NOSONAR
/**
* Connect the client with a given configuration {@link MqttConfig}.
*
* @param config Configuration
* @param onException function executed if an Exception occurs on connection
* @return true
if connected, false
if an error occur
*/
boolean connect(MqttConfig config, Function onException);
/**
* Client is Connected
*
* @return true
if client is connected, else false
*/
boolean isConnected();
/**
* Disconnects the client
*/
void disconnect();
/**
* close the connection
*/
void close();
/**
* Subscribes to a topic with a quality of service. Incoming messages will be
* published to the MqttPushEventSource.
*
* @param topic Topic
* @param qos Quality of Service
* @param src {@link PushEventSource} for incoming messages
*/
void subscribe(String topic, int qos, MqttPushEventSource src);
/**
* Publish content to a broker
*
* @param topic Topic
* @param content Content
* @param qos Quality of service
* @param retained true
to add retrained flag to message.
* @throws Exception
*/
void publish(String topic, byte[] content, int qos, boolean retained) throws Exception;
/**
* Connection lost handling
*
* @param reconnectConsumer consumer executed on connection lost
*/
void connectionLost(Consumer reconnectConsumer);
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy