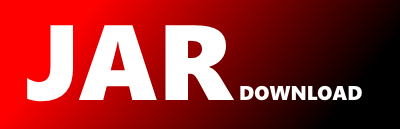
org.genesys2.gringlobal.taxonomy.model.SpeciesRow Maven / Gradle / Ivy
The newest version!
/*
* Copyright 2015 Global Crop Diversity Trust
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.genesys2.gringlobal.taxonomy.model;
import java.io.Serializable;
import java.util.Date;
import com.opencsv.bean.CsvBind;
/**
* "TAXONOMY_SPECIES_ID", "CURRENT_TAXONOMY_SPECIES_ID", "NOMEN_NUMBER", "IS_SPECIFIC_HYBRID", "SPECIES_NAME",
* "SPECIES_AUTHORITY", "IS_SUBSPECIFIC_HYBRID", "SUBSPECIES_NAME", "SUBSPECIES_AUTHORITY", "IS_VARIETAL_HYBRID",
* "VARIETY_NAME", "VARIETY_AUTHORITY", "IS_SUBVARIETAL_HYBRID", "SUBVARIETY_NAME", "SUBVARIETY_AUTHORITY",
* "IS_FORMA_HYBRID", "FORMA_RANK_TYPE", "FORMA_NAME", "FORMA_AUTHORITY", "TAXONOMY_GENUS_ID", "PRIORITY1_SITE_ID",
* "PRIORITY2_SITE_ID", "CURATOR1_COOPERATOR_ID", "CURATOR2_COOPERATOR_ID", "RESTRICTION_CODE", "LIFE_FORM_CODE",
* "COMMON_FERTILIZATION_CODE", "IS_NAME_PENDING", "SYNONYM_CODE", "VERIFIER_COOPERATOR_ID", "NAME_VERIFIED_DATE",
* "NAME", "NAME_AUTHORITY", "PROTOLOGUE", "NOTE", "SITE_NOTE", "ALTERNATE_NAME", "CREATED_DATE", "CREATED_BY",
* "MODIFIED_DATE", "MODIFIED_BY", "OWNED_DATE", "OWNED_BY"
*
* TODO Upgrade to opencsv 3.8 with @CsvDate annotation when available
*/
public class SpeciesRow implements Serializable {
/** The Constant serialVersionUID. */
private static final long serialVersionUID = 5963864285661022518L;
/** The species id. */
@CsvBind
private Long speciesId;
/** The current species id. */
@CsvBind
private Long currentSpeciesId;
/** The nomen number. */
@CsvBind
private Long nomenNumber;
/** The specific hybrid. */
@CsvBind
private Boolean specificHybrid;
/** The species name. */
@CsvBind
private String speciesName;
/** The species authority. */
@CsvBind
private String speciesAuthority;
/** The subspecific hybrid. */
@CsvBind
private Boolean subspecificHybrid;
/** The subspecies name. */
@CsvBind
private String subspeciesName;
/** The subspecies authority. */
@CsvBind
private String subspeciesAuthority;
/** The varietal hybrid. */
@CsvBind
private Boolean varietalHybrid;
/** The variety name. */
@CsvBind
private String varietyName;
/** The variety authority. */
@CsvBind
private String varietyAuthority;
/** The subvarietal hybrid. */
@CsvBind
private Boolean subvarietalHybrid;
/** The subvariety name. */
@CsvBind
private String subvarietyName;
/** The subvariety authority. */
@CsvBind
private String subvarietyAuthority;
/** The forma hybrid. */
@CsvBind
private Boolean formaHybrid;
/** The forma rank type. */
@CsvBind
private String formaRankType;
/** The forma name. */
@CsvBind
private String formaName;
/** The forma authority. */
@CsvBind
private String formaAuthority;
/** The genus id. */
@CsvBind
private Long genusId;
/** The priority site1. */
@CsvBind
private String prioritySite1;
/** The priority site2. */
@CsvBind
private String prioritySite2;
/** The curator1 id. */
@CsvBind
private Long curator1Id;
/** The curator2 id. */
@CsvBind
private Long curator2Id;
/** The restriction code. */
@CsvBind
private String restrictionCode;
/** The life form code. */
@CsvBind
private String lifeFormCode;
/** The common fertilization code. */
@CsvBind
private String commonFertilizationCode;
/** The name pending. */
@CsvBind
private Boolean namePending;
/** The synonym code. */
@CsvBind
private String synonymCode;
/** The verifier id. */
@CsvBind
private Long verifierId;
/** The name verified date. */
@CsvBind
private Date nameVerifiedDate;
/** The name. */
@CsvBind
private String name;
/** The name authority. */
@CsvBind
private String nameAuthority;
/** The protologue. */
@CsvBind
private String protologue;
/** The note. */
@CsvBind
private String note;
/** The site note. */
@CsvBind
private String siteNote;
/** The alternate name. */
@CsvBind
private String alternateName;
/** The created date. */
@CsvBind
private Date createdDate;
/** The created by id. */
@CsvBind
private String createdById;
/** The modified date. */
@CsvBind
private Date modifiedDate;
/** The modified by id. */
@CsvBind
private String modifiedById;
/** The owned date. */
@CsvBind
private Date ownedDate;
/** The owned by id. */
@CsvBind
private String ownedById;
/**
* Gets the species id.
*
* @return the species id
*/
public Long getSpeciesId() {
return speciesId;
}
/**
* Sets the species id.
*
* @param speciesId the new species id
*/
public void setSpeciesId(Long speciesId) {
this.speciesId = speciesId;
}
/**
* Gets the current species id.
*
* @return the current species id
*/
public Long getCurrentSpeciesId() {
return currentSpeciesId;
}
/**
* Sets the current species id.
*
* @param currentSpeciesId the new current species id
*/
public void setCurrentSpeciesId(Long currentSpeciesId) {
this.currentSpeciesId = currentSpeciesId;
}
/**
* Gets the nomen number.
*
* @return the nomen number
*/
public Long getNomenNumber() {
return nomenNumber;
}
/**
* Sets the nomen number.
*
* @param nomenNumber the new nomen number
*/
public void setNomenNumber(Long nomenNumber) {
this.nomenNumber = nomenNumber;
}
/**
* Gets the specific hybrid.
*
* @return the specific hybrid
*/
public Boolean getSpecificHybrid() {
return specificHybrid;
}
/**
* Sets the specific hybrid.
*
* @param specificHybrid the new specific hybrid
*/
public void setSpecificHybrid(Boolean specificHybrid) {
this.specificHybrid = specificHybrid;
}
/**
* Gets the species name.
*
* @return the species name
*/
public String getSpeciesName() {
return speciesName;
}
/**
* Sets the species name.
*
* @param speciesName the new species name
*/
public void setSpeciesName(String speciesName) {
this.speciesName = speciesName;
}
/**
* Gets the species authority.
*
* @return the species authority
*/
public String getSpeciesAuthority() {
return speciesAuthority;
}
/**
* Sets the species authority.
*
* @param speciesAuthority the new species authority
*/
public void setSpeciesAuthority(String speciesAuthority) {
this.speciesAuthority = speciesAuthority;
}
/**
* Gets the subspecific hybrid.
*
* @return the subspecific hybrid
*/
public Boolean getSubspecificHybrid() {
return subspecificHybrid;
}
/**
* Sets the subspecific hybrid.
*
* @param subspecificHybrid the new subspecific hybrid
*/
public void setSubspecificHybrid(Boolean subspecificHybrid) {
this.subspecificHybrid = subspecificHybrid;
}
/**
* Gets the subspecies name.
*
* @return the subspecies name
*/
public String getSubspeciesName() {
return subspeciesName;
}
/**
* Sets the subspecies name.
*
* @param subspeciesName the new subspecies name
*/
public void setSubspeciesName(String subspeciesName) {
this.subspeciesName = subspeciesName;
}
/**
* Gets the subspecies authority.
*
* @return the subspecies authority
*/
public String getSubspeciesAuthority() {
return subspeciesAuthority;
}
/**
* Sets the subspecies authority.
*
* @param subspeciesAuthority the new subspecies authority
*/
public void setSubspeciesAuthority(String subspeciesAuthority) {
this.subspeciesAuthority = subspeciesAuthority;
}
/**
* Gets the varietal hybrid.
*
* @return the varietal hybrid
*/
public Boolean getVarietalHybrid() {
return varietalHybrid;
}
/**
* Sets the varietal hybrid.
*
* @param varietalHybrid the new varietal hybrid
*/
public void setVarietalHybrid(Boolean varietalHybrid) {
this.varietalHybrid = varietalHybrid;
}
/**
* Gets the variety name.
*
* @return the variety name
*/
public String getVarietyName() {
return varietyName;
}
/**
* Sets the variety name.
*
* @param varietyName the new variety name
*/
public void setVarietyName(String varietyName) {
this.varietyName = varietyName;
}
/**
* Gets the variety authority.
*
* @return the variety authority
*/
public String getVarietyAuthority() {
return varietyAuthority;
}
/**
* Sets the variety authority.
*
* @param varietyAuthority the new variety authority
*/
public void setVarietyAuthority(String varietyAuthority) {
this.varietyAuthority = varietyAuthority;
}
/**
* Gets the subvarietal hybrid.
*
* @return the subvarietal hybrid
*/
public Boolean getSubvarietalHybrid() {
return subvarietalHybrid;
}
/**
* Sets the subvarietal hybrid.
*
* @param subvarietalHybrid the new subvarietal hybrid
*/
public void setSubvarietalHybrid(Boolean subvarietalHybrid) {
this.subvarietalHybrid = subvarietalHybrid;
}
/**
* Gets the subvariety name.
*
* @return the subvariety name
*/
public String getSubvarietyName() {
return subvarietyName;
}
/**
* Sets the subvariety name.
*
* @param subvarietyName the new subvariety name
*/
public void setSubvarietyName(String subvarietyName) {
this.subvarietyName = subvarietyName;
}
/**
* Gets the subvariety authority.
*
* @return the subvariety authority
*/
public String getSubvarietyAuthority() {
return subvarietyAuthority;
}
/**
* Sets the subvariety authority.
*
* @param subvarietyAuthority the new subvariety authority
*/
public void setSubvarietyAuthority(String subvarietyAuthority) {
this.subvarietyAuthority = subvarietyAuthority;
}
/**
* Gets the forma hybrid.
*
* @return the forma hybrid
*/
public Boolean getFormaHybrid() {
return formaHybrid;
}
/**
* Sets the forma hybrid.
*
* @param formaHybrid the new forma hybrid
*/
public void setFormaHybrid(Boolean formaHybrid) {
this.formaHybrid = formaHybrid;
}
/**
* Gets the forma rank type.
*
* @return the forma rank type
*/
public String getFormaRankType() {
return formaRankType;
}
/**
* Sets the forma rank type.
*
* @param formaRankType the new forma rank type
*/
public void setFormaRankType(String formaRankType) {
this.formaRankType = formaRankType;
}
/**
* Gets the forma name.
*
* @return the forma name
*/
public String getFormaName() {
return formaName;
}
/**
* Sets the forma name.
*
* @param formaName the new forma name
*/
public void setFormaName(String formaName) {
this.formaName = formaName;
}
/**
* Gets the forma authority.
*
* @return the forma authority
*/
public String getFormaAuthority() {
return formaAuthority;
}
/**
* Sets the forma authority.
*
* @param formaAuthority the new forma authority
*/
public void setFormaAuthority(String formaAuthority) {
this.formaAuthority = formaAuthority;
}
/**
* Gets the genus id.
*
* @return the genus id
*/
public Long getGenusId() {
return genusId;
}
/**
* Sets the genus id.
*
* @param genusId the new genus id
*/
public void setGenusId(Long genusId) {
this.genusId = genusId;
}
/**
* Gets the priority site1.
*
* @return the priority site1
*/
public String getPrioritySite1() {
return prioritySite1;
}
/**
* Sets the priority site1.
*
* @param prioritySite1 the new priority site1
*/
public void setPrioritySite1(String prioritySite1) {
this.prioritySite1 = prioritySite1;
}
/**
* Gets the priority site2.
*
* @return the priority site2
*/
public String getPrioritySite2() {
return prioritySite2;
}
/**
* Sets the priority site2.
*
* @param prioritySite2 the new priority site2
*/
public void setPrioritySite2(String prioritySite2) {
this.prioritySite2 = prioritySite2;
}
/**
* Gets the curator1 id.
*
* @return the curator1 id
*/
public Long getCurator1Id() {
return curator1Id;
}
/**
* Sets the curator1 id.
*
* @param curator1Id the new curator1 id
*/
public void setCurator1Id(Long curator1Id) {
this.curator1Id = curator1Id;
}
/**
* Gets the curator2 id.
*
* @return the curator2 id
*/
public Long getCurator2Id() {
return curator2Id;
}
/**
* Sets the curator2 id.
*
* @param curator2Id the new curator2 id
*/
public void setCurator2Id(Long curator2Id) {
this.curator2Id = curator2Id;
}
/**
* Gets the restriction code.
*
* @return the restriction code
*/
public String getRestrictionCode() {
return restrictionCode;
}
/**
* Sets the restriction code.
*
* @param restrictionCode the new restriction code
*/
public void setRestrictionCode(String restrictionCode) {
this.restrictionCode = restrictionCode;
}
/**
* Gets the life form code.
*
* @return the life form code
*/
public String getLifeFormCode() {
return lifeFormCode;
}
/**
* Sets the life form code.
*
* @param lifeFormCode the new life form code
*/
public void setLifeFormCode(String lifeFormCode) {
this.lifeFormCode = lifeFormCode;
}
/**
* Gets the common fertilization code.
*
* @return the common fertilization code
*/
public String getCommonFertilizationCode() {
return commonFertilizationCode;
}
/**
* Sets the common fertilization code.
*
* @param commonFertilizationCode the new common fertilization code
*/
public void setCommonFertilizationCode(String commonFertilizationCode) {
this.commonFertilizationCode = commonFertilizationCode;
}
/**
* Gets the name pending.
*
* @return the name pending
*/
public Boolean getNamePending() {
return namePending;
}
/**
* Sets the name pending.
*
* @param namePending the new name pending
*/
public void setNamePending(Boolean namePending) {
this.namePending = namePending;
}
/**
* Gets the synonym code.
*
* @return the synonym code
*/
public String getSynonymCode() {
return synonymCode;
}
/**
* Sets the synonym code.
*
* @param synonymCode the new synonym code
*/
public void setSynonymCode(String synonymCode) {
this.synonymCode = synonymCode;
}
/**
* Gets the verifier id.
*
* @return the verifier id
*/
public Long getVerifierId() {
return verifierId;
}
/**
* Sets the verifier id.
*
* @param verifierId the new verifier id
*/
public void setVerifierId(Long verifierId) {
this.verifierId = verifierId;
}
/**
* Gets the name verified date.
*
* @return the name verified date
*/
public Date getNameVerifiedDate() {
return nameVerifiedDate;
}
/**
* Sets the name verified date.
*
* @param nameVerifiedDate the new name verified date
*/
public void setNameVerifiedDate(Date nameVerifiedDate) {
this.nameVerifiedDate = nameVerifiedDate;
}
/**
* Gets the full scientific name.
*
* @return the name
*/
public String getName() {
return name;
}
/**
* Sets the name.
*
* @param name the new name
*/
public void setName(String name) {
this.name = name;
}
/**
* Gets the name authority.
*
* @return the name authority
*/
public String getNameAuthority() {
return nameAuthority;
}
/**
* Sets the name authority.
*
* @param nameAuthority the new name authority
*/
public void setNameAuthority(String nameAuthority) {
this.nameAuthority = nameAuthority;
}
/**
* Gets the protologue.
*
* @return the protologue
*/
public String getProtologue() {
return protologue;
}
/**
* Sets the protologue.
*
* @param protologue the new protologue
*/
public void setProtologue(String protologue) {
this.protologue = protologue;
}
/**
* Gets the note.
*
* @return the note
*/
public String getNote() {
return note;
}
/**
* Sets the note.
*
* @param note the new note
*/
public void setNote(String note) {
this.note = note;
}
/**
* Gets the site note.
*
* @return the site note
*/
public String getSiteNote() {
return siteNote;
}
/**
* Sets the site note.
*
* @param siteNote the new site note
*/
public void setSiteNote(String siteNote) {
this.siteNote = siteNote;
}
/**
* Gets the alternate name.
*
* @return the alternate name
*/
public String getAlternateName() {
return alternateName;
}
/**
* Sets the alternate name.
*
* @param alternateName the new alternate name
*/
public void setAlternateName(String alternateName) {
this.alternateName = alternateName;
}
/**
* Gets the created date.
*
* @return the created date
*/
public Date getCreatedDate() {
return createdDate;
}
/**
* Sets the created date.
*
* @param createdDate the new created date
*/
public void setCreatedDate(Date createdDate) {
this.createdDate = createdDate;
}
/**
* Gets the created by id.
*
* @return the created by id
*/
public String getCreatedById() {
return createdById;
}
/**
* Sets the created by id.
*
* @param createdById the new created by id
*/
public void setCreatedById(String createdById) {
this.createdById = createdById;
}
/**
* Gets the modified date.
*
* @return the modified date
*/
public Date getModifiedDate() {
return modifiedDate;
}
/**
* Sets the modified date.
*
* @param modifiedDate the new modified date
*/
public void setModifiedDate(Date modifiedDate) {
this.modifiedDate = modifiedDate;
}
/**
* Gets the modified by id.
*
* @return the modified by id
*/
public String getModifiedById() {
return modifiedById;
}
/**
* Sets the modified by id.
*
* @param modifiedById the new modified by id
*/
public void setModifiedById(String modifiedById) {
this.modifiedById = modifiedById;
}
/**
* Gets the owned date.
*
* @return the owned date
*/
public Date getOwnedDate() {
return ownedDate;
}
/**
* Sets the owned date.
*
* @param ownedDate the new owned date
*/
public void setOwnedDate(Date ownedDate) {
this.ownedDate = ownedDate;
}
/**
* Gets the owned by id.
*
* @return the owned by id
*/
public String getOwnedById() {
return ownedById;
}
/**
* Sets the owned by id.
*
* @param ownedById the new owned by id
*/
public void setOwnedById(String ownedById) {
this.ownedById = ownedById;
}
/**
* Is this record still current?.
*
* @return true
when {@link #currentSpeciesId} equals {@link #speciesId}
*/
public boolean isCurrent() {
return speciesId.equals(currentSpeciesId);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy