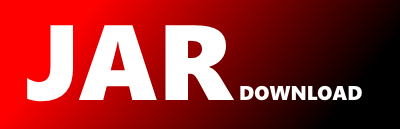
org.geojsf.mbean.admin.AbstractSldDynamicBean Maven / Gradle / Ivy
package org.geojsf.mbean.admin;
import java.io.Serializable;
import java.util.List;
import org.geojsf.factory.ejb.sld.EjbGeoSldFactory;
import org.geojsf.interfaces.facade.GeoJsfFacade;
import org.geojsf.interfaces.model.core.GeoJsfCategory;
import org.geojsf.interfaces.model.core.GeoJsfLayer;
import org.geojsf.interfaces.model.core.GeoJsfMap;
import org.geojsf.interfaces.model.core.GeoJsfService;
import org.geojsf.interfaces.model.core.GeoJsfView;
import org.geojsf.interfaces.model.meta.GeoJsfDataSource;
import org.geojsf.interfaces.model.meta.GeoJsfViewPort;
import org.geojsf.interfaces.model.sld.GeoJsfSld;
import org.geojsf.interfaces.model.sld.GeoJsfSldRule;
import org.geojsf.interfaces.model.sld.GeoJsfSldTemplate;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import net.sf.ahtutils.exception.ejb.UtilsConstraintViolationException;
import net.sf.ahtutils.exception.ejb.UtilsLockingException;
import net.sf.ahtutils.exception.ejb.UtilsNotFoundException;
import net.sf.ahtutils.factory.ejb.status.EjbDescriptionFactory;
import net.sf.ahtutils.factory.ejb.status.EjbLangFactory;
import net.sf.ahtutils.interfaces.model.graphic.UtilsGraphic;
import net.sf.ahtutils.interfaces.model.status.UtilsDescription;
import net.sf.ahtutils.interfaces.model.status.UtilsLang;
import net.sf.ahtutils.interfaces.model.status.UtilsStatus;
import net.sf.ahtutils.web.mbean.util.AbstractLogMessage;
public class AbstractSldDynamicBean ,
GT extends UtilsStatus,
GS extends UtilsStatus,
CATEGORY extends GeoJsfCategory,
SERVICE extends GeoJsfService,
LAYER extends GeoJsfLayer,
MAP extends GeoJsfMap,
VIEW extends GeoJsfView,
VP extends GeoJsfViewPort,
DS extends GeoJsfDataSource,
SLDTEMPLATE extends GeoJsfSldTemplate,
SLDTYPE extends UtilsStatus,
SLD extends GeoJsfSld,
RULE extends GeoJsfSldRule
>
implements Serializable
{
private static final long serialVersionUID = 1L;
final static Logger logger = LoggerFactory.getLogger(AbstractSldDynamicBean.class);
protected EjbLangFactory efLang;
protected EjbDescriptionFactory efDescription;
protected EjbGeoSldFactory efSld;
private GeoJsfFacade fGeo;
private String[] langKeys;
private Class cSld;
private Class cTemplate;
protected List templates; public List getTemplates(){return templates;}
protected List slds; public List getSlds() {return slds;} public void setSlds(List slds) {this.slds = slds;}
public void initSuper(String[] langKeys, GeoJsfFacade fGeo, final Class cLang, final Class clDescription,final Class cSld, final Class cType,final Class cTemplate)
{
this.langKeys=langKeys;
this.fGeo=fGeo;
this.cSld=cSld;
this.cTemplate=cTemplate;
efLang = EjbLangFactory.createFactory(cLang);
efDescription = EjbDescriptionFactory.createFactory(clDescription);
efSld = EjbGeoSldFactory.factory(cSld);
templates = fGeo.all(cTemplate);
}
protected void reloadSlds()
{
slds = fGeo.fGlobalSlds(cSld);
}
//TEMPLATE
protected SLD sld; public SLD getSld() {return sld;} public void setSld(SLD sld) {this.sld = sld;}
public void selectSld() throws UtilsNotFoundException, UtilsConstraintViolationException, UtilsLockingException
{
logger.info(AbstractLogMessage.selectEntity(sld));
}
public void addSld()
{
logger.info(AbstractLogMessage.addEntity(cSld));
sld = efSld.build(null,true);
sld.setName(efLang.createEmpty(langKeys));
sld.setDescription(efDescription.createEmpty(langKeys));
}
public void cancelSld()
{
sld=null;
}
public void saveSld() throws UtilsConstraintViolationException, UtilsLockingException
{
logger.info(AbstractLogMessage.saveEntity(sld));
sld.setTemplate(fGeo.find(cTemplate, sld.getTemplate()));
sld = fGeo.save(sld);
reloadSlds();
}
public void rmSld() throws UtilsConstraintViolationException, UtilsLockingException
{
logger.info(AbstractLogMessage.rmEntity(sld));
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy