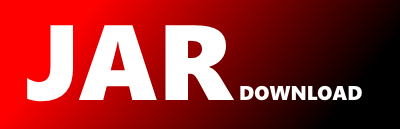
org.geolatte.geom.codec.AbstractWkbDecoder Maven / Gradle / Ivy
Show all versions of geolatte-geom Show documentation
/*
* This file is part of the GeoLatte project.
*
* GeoLatte is free software: you can redistribute it and/or modify
* it under the terms of the GNU Lesser General Public License as published by
* the Free Software Foundation, either version 3 of the License, or
* (at your option) any later version.
*
* GeoLatte is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU Lesser General Public License for more details.
*
* You should have received a copy of the GNU Lesser General Public License
* along with GeoLatte. If not, see .
*
* Copyright (C) 2010 - 2012 and Ownership of code is shared by:
* Qmino bvba - Romeinsestraat 18 - 3001 Heverlee (http://www.qmino.com)
* Geovise bvba - Generaal Eisenhowerlei 9 - 2140 Antwerpen (http://www.geovise.com)
*/
package org.geolatte.geom.codec;
import org.geolatte.geom.*;
import org.geolatte.geom.crs.CoordinateReferenceSystem;
import java.util.ArrayList;
import java.util.List;
import static org.geolatte.geom.Geometries.*;
/**
* Base class for WkbDecoder
s.
*
* @author Karel Maesen, Geovise BVBA
* creation-date: 11/1/12
*/
abstract class AbstractWkbDecoder implements WkbDecoder {
@Override
public Geometry
decode(ByteBuffer byteBuffer, CoordinateReferenceSystem
crs) {
byteBuffer.rewind();
try {
prepare(byteBuffer);
Geometry
geom = decodeGeometry(byteBuffer, crs);
byteBuffer.rewind();
return geom;
} catch (BufferAccessException e) {
throw new WkbDecodeException(e);
}
}
@Override
public Geometry> decode(ByteBuffer byteBuffer) {
return decode(byteBuffer, (CoordinateReferenceSystem>) null);
}
private
Geometry
decodeGeometry(ByteBuffer byteBuffer, CoordinateReferenceSystem
crs) {
alignByteOrder(byteBuffer);
int typeCode = readTypeCode(byteBuffer);
WkbGeometryType wkbType = WkbGeometryType.parse((byte) typeCode);
crs = (CoordinateReferenceSystem
)readCrs(byteBuffer, typeCode, crs);
switch (wkbType) {
case POINT:
return decodePoint(byteBuffer, crs);
case LINE_STRING:
return decodeLineString(byteBuffer, crs);
case POLYGON:
return decodePolygon(byteBuffer, crs);
case GEOMETRY_COLLECTION:
return decodeGeometryCollection(byteBuffer, crs);
case MULTI_POINT:
return decodeMultiPoint(byteBuffer, crs);
case MULTI_POLYGON:
return decodeMultiPolygon(byteBuffer, crs);
case MULTI_LINE_STRING:
return decodeMultiLineString(byteBuffer,crs );
}
throw new WkbDecodeException(String.format("WKBType %s is not supported.", wkbType));
}
private
MultiLineString
decodeMultiLineString(ByteBuffer byteBuffer, CoordinateReferenceSystem
crs) {
int numGeometries = byteBuffer.getInt();
if (numGeometries == 0) {
return new MultiLineString
(crs);
}
List> geometries = new ArrayList>(numGeometries);
for (int i = 0; i < numGeometries; i++) {
geometries.add((LineString)decodeGeometry(byteBuffer, crs));
}
return mkMultiLineString(geometries);
}
private
MultiPoint
decodeMultiPoint(ByteBuffer byteBuffer, CoordinateReferenceSystem
crs) {
int numGeometries = byteBuffer.getInt();
if (numGeometries == 0) {
return new MultiPoint
(crs);
}
List> geometries = new ArrayList>(numGeometries);
for (int i = 0; i < numGeometries; i++) {
geometries.add((Point) decodeGeometry(byteBuffer, crs));
}
return mkMultiPoint(geometries);
}
private
MultiPolygon
decodeMultiPolygon(ByteBuffer byteBuffer, CoordinateReferenceSystem
crs) {
int numGeometries = byteBuffer.getInt();
if (numGeometries == 0) {
return new MultiPolygon
(crs);
}
List> geometries = new ArrayList>(numGeometries);
for (int i = 0; i < numGeometries; i++) {
geometries.add((Polygon) decodeGeometry(byteBuffer, crs));
}
return mkMultiPolygon(geometries);
}
private
AbstractGeometryCollection
>
decodeGeometryCollection(ByteBuffer byteBuffer, CoordinateReferenceSystem
crs) {
int numGeometries = byteBuffer.getInt();
if (numGeometries == 0) {
return new GeometryCollection<>(crs);
}
List> geometries = new ArrayList>(numGeometries);
for (int i = 0; i < numGeometries; i++) {
geometries.add(decodeGeometry(byteBuffer, crs));
}
return mkGeometryCollection(geometries);
}
private Polygon
decodePolygon(ByteBuffer byteBuffer, CoordinateReferenceSystem
crs) {
int numRings = byteBuffer.getInt();
List> rings = readPolygonRings(numRings, byteBuffer, crs);
return mkPolygon(rings);
}
private LineString
decodeLineString(ByteBuffer byteBuffer, CoordinateReferenceSystem
crs) {
int numPoints = byteBuffer.getInt();
PositionSequence
points = readPositions(numPoints, byteBuffer, crs);
return new LineString
(points, crs);
}
private
Point
decodePoint(ByteBuffer byteBuffer, CoordinateReferenceSystem
crs) {
PositionSequence
points = readPositions(1, byteBuffer, crs);
return new Point
(points, crs);
}
private
PositionSequence
readPositions(int numPos, ByteBuffer byteBuffer, CoordinateReferenceSystem
crs) {
PositionSequenceBuilder
psBuilder = PositionSequenceBuilders.fixedSized(numPos, crs.getPositionClass());
double[] coordinates = new double[crs.getCoordinateDimension()];
for (int i = 0; i < numPos; i++) {
readPosition(byteBuffer, coordinates, crs);
psBuilder.add(coordinates);
}
return psBuilder.toPositionSequence();
}
private
void readPosition(ByteBuffer byteBuffer, double[] coordinates, CoordinateReferenceSystem
crs) {
for (int ci = 0; ci < crs.getCoordinateDimension(); ci++) {
coordinates[ci] = byteBuffer.getDouble();
}
}
private
List> readPolygonRings(int numRings, ByteBuffer byteBuffer, CoordinateReferenceSystem crs) {
List> rings = new ArrayList>(numRings);
for (int i = 0; i < numRings; i++) {
rings.add(readRing(byteBuffer, crs));
}
return rings;
}
private LinearRing
readRing(ByteBuffer byteBuffer, CoordinateReferenceSystem
crs) {
int numPoints = byteBuffer.getInt();
PositionSequence
ps = readPositions(numPoints, byteBuffer, crs);
try {
return new LinearRing
(ps, crs);
} catch (IllegalArgumentException e) {
throw new WkbDecodeException(e);
}
}
/**
* Perform any preparatory steps on the bytebuffer before
* starting the decoding.
*
* @param byteBuffer
*/
protected abstract void prepare(ByteBuffer byteBuffer);
/**
* Read and set the SRID (if it is present)
*
* @param byteBuffer
* @param typeCode
* @param crs
*/
protected abstract
CoordinateReferenceSystem
readCrs(ByteBuffer byteBuffer, int typeCode, CoordinateReferenceSystem
crs);
protected abstract boolean hasSrid(int typeCode);
protected int readTypeCode(ByteBuffer byteBuffer) {
return (int) byteBuffer.getUInt();
}
private void alignByteOrder(ByteBuffer byteBuffer) {
byte orderByte = byteBuffer.get();
ByteOrder byteOrder = ByteOrder.valueOf(orderByte);
byteBuffer.setByteOrder(byteOrder);
}
}