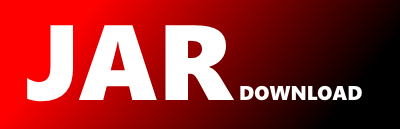
org.geolatte.maprenderer.java2D.ImageComparator Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of geolatte-maprenderer Show documentation
Show all versions of geolatte-maprenderer Show documentation
A renderer with a geo-centered API.
The newest version!
/*
* This file is part of the GeoLatte project.
*
* GeoLatte is free software: you can redistribute it and/or modify
* it under the terms of the GNU Lesser General Public License as published by
* the Free Software Foundation, either version 3 of the License, or
* (at your option) any later version.
*
* GeoLatte is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU Lesser General Public License for more details.
*
* You should have received a copy of the GNU Lesser General Public License
* along with GeoLatte. If not, see .
*
* Copyright (C) 2010 - 2011 and Ownership of code is shared by:
* Qmino bvba - Esperantolaan 4 - 3001 Heverlee (http://www.qmino.com)
* Geovise bvba - Generaal Eisenhowerlei 9 - 2140 Antwerpen (http://www.geovise.com)
*/
package org.geolatte.maprenderer.java2D;
import java.awt.*;
import java.awt.image.Raster;
import java.awt.image.RenderedImage;
/**
* @author Karel Maesen, Geovise BVBA
* creation-date: 9/10/11
*/
public class ImageComparator {
public boolean equals(RenderedImage img1, RenderedImage img2) {
if (img1 == img2) return true;
if (img1 == null || img2 == null) return false;
Raster r1 = img1.getData();
Raster r2 = img2.getData();
if (!sameBounds(r1, r2)) return false;
if (!sameBands(r1, r2)) return false;
if (!sameRasterData(r1, r2)) return false;
return true;
}
//Turns out testing on equality of samplemodel and colormodel is too strict.
//This is often modified by ImageIO.
// private boolean sameSampleModel(Raster r1, Raster r2) {
// SampleModel model1 = r1.getSampleModel();
// SampleModel model2 = r2.getSampleModel();
// if (model1.getDataType() != model2.getDataType()) return false;
// return true;
// }
//
// private boolean sameColorModel(RenderedImage img1, RenderedImage img2) {
// return img1.getColorModel().equals(img2.getColorModel());
// }
private boolean sameBands(Raster r1, Raster r2) {
return r1.getNumBands() == r2.getNumBands();
}
private boolean sameBounds(Raster r1, Raster r2) {
Rectangle r1Bounds = r1.getBounds();
Rectangle r2Bounds = r2.getBounds();
return (r1Bounds.equals(r1Bounds));
}
private boolean sameRasterData(Raster r1, Raster r2) {
for (int i = 0; i < r1.getNumBands(); i++) {
if (!sameRasterBand(r1, r2, i)) return false;
}
return true;
}
private boolean sameRasterBand(Raster r1, Raster r2, int band) {
for (int xIdx = 0; xIdx < r1.getWidth(); xIdx++) {
for (int yIdx = 0; yIdx < r1.getHeight(); yIdx++) {
if (r1.getSampleDouble(xIdx + r1.getMinX(), yIdx + r1.getMinY(), band) !=
r2.getSampleDouble(xIdx + r2.getMinX(), yIdx + r2.getMinY(), band)) {
return false;
}
}
}
return true;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy