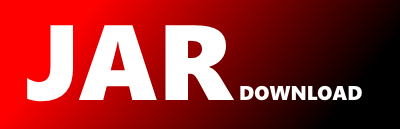
net.opengis.wfsv.WfsvPackage Maven / Gradle / Ivy
/**
*
*
*
* $Id: WfsvPackage.java 7988 2007-12-12 20:29:15Z aaime $
*/
package net.opengis.wfsv;
import net.opengis.wfs.WfsPackage;
import org.eclipse.emf.ecore.EAttribute;
import org.eclipse.emf.ecore.EClass;
import org.eclipse.emf.ecore.EPackage;
import org.eclipse.emf.ecore.EReference;
/**
*
* The Package for the model.
* It contains accessors for the meta objects to represent
*
* - each class,
* - each feature of each class,
* - each enum,
* - and each data type
*
*
* @see net.opengis.wfsv.WfsvFactory
* @model kind="package"
* @generated
*/
public interface WfsvPackage extends EPackage {
/**
* The package name.
*
*
* @generated
*/
String eNAME = "wfsv";
/**
* The package namespace URI.
*
*
* @generated
*/
String eNS_URI = "http://www.opengis.net/wfsv";
/**
* The package namespace name.
*
*
* @generated
*/
String eNS_PREFIX = "wfsv";
/**
* The singleton instance of the package.
*
*
* @generated
*/
WfsvPackage eINSTANCE = net.opengis.wfsv.impl.WfsvPackageImpl.init();
/**
* The meta object id for the '{@link net.opengis.wfsv.impl.AbstractVersionedFeatureTypeImpl Abstract Versioned Feature Type}' class.
*
*
* @see net.opengis.wfsv.impl.AbstractVersionedFeatureTypeImpl
* @see net.opengis.wfsv.impl.WfsvPackageImpl#getAbstractVersionedFeatureType()
* @generated
*/
int ABSTRACT_VERSIONED_FEATURE_TYPE = 0;
/**
* The feature id for the 'Version' attribute.
*
*
* @generated
* @ordered
*/
int ABSTRACT_VERSIONED_FEATURE_TYPE__VERSION = 0;
/**
* The feature id for the 'Author' attribute.
*
*
* @generated
* @ordered
*/
int ABSTRACT_VERSIONED_FEATURE_TYPE__AUTHOR = 1;
/**
* The feature id for the 'Date' attribute.
*
*
* @generated
* @ordered
*/
int ABSTRACT_VERSIONED_FEATURE_TYPE__DATE = 2;
/**
* The feature id for the 'Message' attribute.
*
*
* @generated
* @ordered
*/
int ABSTRACT_VERSIONED_FEATURE_TYPE__MESSAGE = 3;
/**
* The number of structural features of the 'Abstract Versioned Feature Type' class.
*
*
* @generated
* @ordered
*/
int ABSTRACT_VERSIONED_FEATURE_TYPE_FEATURE_COUNT = 4;
/**
* The meta object id for the '{@link net.opengis.wfsv.impl.DescribeVersionedFeatureTypeTypeImpl Describe Versioned Feature Type Type}' class.
*
*
* @see net.opengis.wfsv.impl.DescribeVersionedFeatureTypeTypeImpl
* @see net.opengis.wfsv.impl.WfsvPackageImpl#getDescribeVersionedFeatureTypeType()
* @generated
*/
int DESCRIBE_VERSIONED_FEATURE_TYPE_TYPE = 1;
/**
* The feature id for the 'Handle' attribute.
*
*
* @generated
* @ordered
*/
int DESCRIBE_VERSIONED_FEATURE_TYPE_TYPE__HANDLE = WfsPackage.DESCRIBE_FEATURE_TYPE_TYPE__HANDLE;
/**
* The feature id for the 'Service' attribute.
*
*
* @generated
* @ordered
*/
int DESCRIBE_VERSIONED_FEATURE_TYPE_TYPE__SERVICE = WfsPackage.DESCRIBE_FEATURE_TYPE_TYPE__SERVICE;
/**
* The feature id for the 'Version' attribute.
*
*
* @generated
* @ordered
*/
int DESCRIBE_VERSIONED_FEATURE_TYPE_TYPE__VERSION = WfsPackage.DESCRIBE_FEATURE_TYPE_TYPE__VERSION;
/**
* The feature id for the 'Type Name' attribute list.
*
*
* @generated
* @ordered
*/
int DESCRIBE_VERSIONED_FEATURE_TYPE_TYPE__TYPE_NAME = WfsPackage.DESCRIBE_FEATURE_TYPE_TYPE__TYPE_NAME;
/**
* The feature id for the 'Output Format' attribute.
*
*
* @generated
* @ordered
*/
int DESCRIBE_VERSIONED_FEATURE_TYPE_TYPE__OUTPUT_FORMAT = WfsPackage.DESCRIBE_FEATURE_TYPE_TYPE__OUTPUT_FORMAT;
/**
* The feature id for the 'Versioned' attribute.
*
*
* @generated
* @ordered
*/
int DESCRIBE_VERSIONED_FEATURE_TYPE_TYPE__VERSIONED = WfsPackage.DESCRIBE_FEATURE_TYPE_TYPE_FEATURE_COUNT + 0;
/**
* The number of structural features of the 'Describe Versioned Feature Type Type' class.
*
*
* @generated
* @ordered
*/
int DESCRIBE_VERSIONED_FEATURE_TYPE_TYPE_FEATURE_COUNT = WfsPackage.DESCRIBE_FEATURE_TYPE_TYPE_FEATURE_COUNT + 1;
/**
* The meta object id for the '{@link net.opengis.wfsv.impl.DifferenceQueryTypeImpl Difference Query Type}' class.
*
*
* @see net.opengis.wfsv.impl.DifferenceQueryTypeImpl
* @see net.opengis.wfsv.impl.WfsvPackageImpl#getDifferenceQueryType()
* @generated
*/
int DIFFERENCE_QUERY_TYPE = 2;
/**
* The feature id for the 'Filter' attribute.
*
*
* @generated
* @ordered
*/
int DIFFERENCE_QUERY_TYPE__FILTER = 0;
/**
* The feature id for the 'From Feature Version' attribute.
*
*
* @generated
* @ordered
*/
int DIFFERENCE_QUERY_TYPE__FROM_FEATURE_VERSION = 1;
/**
* The feature id for the 'Srs Name' attribute.
*
*
* @generated
* @ordered
*/
int DIFFERENCE_QUERY_TYPE__SRS_NAME = 2;
/**
* The feature id for the 'To Feature Version' attribute.
*
*
* @generated
* @ordered
*/
int DIFFERENCE_QUERY_TYPE__TO_FEATURE_VERSION = 3;
/**
* The feature id for the 'Type Name' attribute.
*
*
* @generated
* @ordered
*/
int DIFFERENCE_QUERY_TYPE__TYPE_NAME = 4;
/**
* The number of structural features of the 'Difference Query Type' class.
*
*
* @generated
* @ordered
*/
int DIFFERENCE_QUERY_TYPE_FEATURE_COUNT = 5;
/**
* The meta object id for the '{@link net.opengis.wfsv.impl.DocumentRootImpl Document Root}' class.
*
*
* @see net.opengis.wfsv.impl.DocumentRootImpl
* @see net.opengis.wfsv.impl.WfsvPackageImpl#getDocumentRoot()
* @generated
*/
int DOCUMENT_ROOT = 3;
/**
* The feature id for the 'Mixed' attribute list.
*
*
* @generated
* @ordered
*/
int DOCUMENT_ROOT__MIXED = 0;
/**
* The feature id for the 'XMLNS Prefix Map' map.
*
*
* @generated
* @ordered
*/
int DOCUMENT_ROOT__XMLNS_PREFIX_MAP = 1;
/**
* The feature id for the 'XSI Schema Location' map.
*
*
* @generated
* @ordered
*/
int DOCUMENT_ROOT__XSI_SCHEMA_LOCATION = 2;
/**
* The feature id for the 'Describe Versioned Feature Type' attribute.
*
*
* @generated
* @ordered
*/
int DOCUMENT_ROOT__DESCRIBE_VERSIONED_FEATURE_TYPE = 3;
/**
* The feature id for the 'Difference Query' containment reference.
*
*
* @generated
* @ordered
*/
int DOCUMENT_ROOT__DIFFERENCE_QUERY = 4;
/**
* The feature id for the 'Get Diff' containment reference.
*
*
* @generated
* @ordered
*/
int DOCUMENT_ROOT__GET_DIFF = 5;
/**
* The feature id for the 'Get Log' containment reference.
*
*
* @generated
* @ordered
*/
int DOCUMENT_ROOT__GET_LOG = 6;
/**
* The feature id for the 'Get Versioned Feature' containment reference.
*
*
* @generated
* @ordered
*/
int DOCUMENT_ROOT__GET_VERSIONED_FEATURE = 7;
/**
* The feature id for the 'Rollback' containment reference.
*
*
* @generated
* @ordered
*/
int DOCUMENT_ROOT__ROLLBACK = 8;
/**
* The feature id for the 'Versioned Delete' containment reference.
*
*
* @generated
* @ordered
*/
int DOCUMENT_ROOT__VERSIONED_DELETE = 9;
/**
* The feature id for the 'Versioned Feature Collection' containment reference.
*
*
* @generated
* @ordered
*/
int DOCUMENT_ROOT__VERSIONED_FEATURE_COLLECTION = 10;
/**
* The feature id for the 'Versioned Update' containment reference.
*
*
* @generated
* @ordered
*/
int DOCUMENT_ROOT__VERSIONED_UPDATE = 11;
/**
* The number of structural features of the 'Document Root' class.
*
*
* @generated
* @ordered
*/
int DOCUMENT_ROOT_FEATURE_COUNT = 12;
/**
* The meta object id for the '{@link net.opengis.wfsv.impl.GetDiffTypeImpl Get Diff Type}' class.
*
*
* @see net.opengis.wfsv.impl.GetDiffTypeImpl
* @see net.opengis.wfsv.impl.WfsvPackageImpl#getGetDiffType()
* @generated
*/
int GET_DIFF_TYPE = 4;
/**
* The feature id for the 'Handle' attribute.
*
*
* @generated
* @ordered
*/
int GET_DIFF_TYPE__HANDLE = WfsPackage.BASE_REQUEST_TYPE__HANDLE;
/**
* The feature id for the 'Service' attribute.
*
*
* @generated
* @ordered
*/
int GET_DIFF_TYPE__SERVICE = WfsPackage.BASE_REQUEST_TYPE__SERVICE;
/**
* The feature id for the 'Version' attribute.
*
*
* @generated
* @ordered
*/
int GET_DIFF_TYPE__VERSION = WfsPackage.BASE_REQUEST_TYPE__VERSION;
/**
* The feature id for the 'Difference Query' containment reference list.
*
*
* @generated
* @ordered
*/
int GET_DIFF_TYPE__DIFFERENCE_QUERY = WfsPackage.BASE_REQUEST_TYPE_FEATURE_COUNT + 0;
/**
* The feature id for the 'Output Format' attribute.
*
*
* @generated
* @ordered
*/
int GET_DIFF_TYPE__OUTPUT_FORMAT = WfsPackage.BASE_REQUEST_TYPE_FEATURE_COUNT + 1;
/**
* The number of structural features of the 'Get Diff Type' class.
*
*
* @generated
* @ordered
*/
int GET_DIFF_TYPE_FEATURE_COUNT = WfsPackage.BASE_REQUEST_TYPE_FEATURE_COUNT + 2;
/**
* The meta object id for the '{@link net.opengis.wfsv.impl.GetLogTypeImpl Get Log Type}' class.
*
*
* @see net.opengis.wfsv.impl.GetLogTypeImpl
* @see net.opengis.wfsv.impl.WfsvPackageImpl#getGetLogType()
* @generated
*/
int GET_LOG_TYPE = 5;
/**
* The feature id for the 'Handle' attribute.
*
*
* @generated
* @ordered
*/
int GET_LOG_TYPE__HANDLE = WfsPackage.BASE_REQUEST_TYPE__HANDLE;
/**
* The feature id for the 'Service' attribute.
*
*
* @generated
* @ordered
*/
int GET_LOG_TYPE__SERVICE = WfsPackage.BASE_REQUEST_TYPE__SERVICE;
/**
* The feature id for the 'Version' attribute.
*
*
* @generated
* @ordered
*/
int GET_LOG_TYPE__VERSION = WfsPackage.BASE_REQUEST_TYPE__VERSION;
/**
* The feature id for the 'Difference Query' containment reference list.
*
*
* @generated
* @ordered
*/
int GET_LOG_TYPE__DIFFERENCE_QUERY = WfsPackage.BASE_REQUEST_TYPE_FEATURE_COUNT + 0;
/**
* The feature id for the 'Max Features' attribute.
*
*
* @generated
* @ordered
*/
int GET_LOG_TYPE__MAX_FEATURES = WfsPackage.BASE_REQUEST_TYPE_FEATURE_COUNT + 1;
/**
* The feature id for the 'Output Format' attribute.
*
*
* @generated
* @ordered
*/
int GET_LOG_TYPE__OUTPUT_FORMAT = WfsPackage.BASE_REQUEST_TYPE_FEATURE_COUNT + 2;
/**
* The feature id for the 'Result Type' attribute.
*
*
* @generated
* @ordered
*/
int GET_LOG_TYPE__RESULT_TYPE = WfsPackage.BASE_REQUEST_TYPE_FEATURE_COUNT + 3;
/**
* The number of structural features of the 'Get Log Type' class.
*
*
* @generated
* @ordered
*/
int GET_LOG_TYPE_FEATURE_COUNT = WfsPackage.BASE_REQUEST_TYPE_FEATURE_COUNT + 4;
/**
* The meta object id for the '{@link net.opengis.wfsv.impl.GetVersionedFeatureTypeImpl Get Versioned Feature Type}' class.
*
*
* @see net.opengis.wfsv.impl.GetVersionedFeatureTypeImpl
* @see net.opengis.wfsv.impl.WfsvPackageImpl#getGetVersionedFeatureType()
* @generated
*/
int GET_VERSIONED_FEATURE_TYPE = 6;
/**
* The feature id for the 'Handle' attribute.
*
*
* @generated
* @ordered
*/
int GET_VERSIONED_FEATURE_TYPE__HANDLE = WfsPackage.GET_FEATURE_TYPE__HANDLE;
/**
* The feature id for the 'Service' attribute.
*
*
* @generated
* @ordered
*/
int GET_VERSIONED_FEATURE_TYPE__SERVICE = WfsPackage.GET_FEATURE_TYPE__SERVICE;
/**
* The feature id for the 'Version' attribute.
*
*
* @generated
* @ordered
*/
int GET_VERSIONED_FEATURE_TYPE__VERSION = WfsPackage.GET_FEATURE_TYPE__VERSION;
/**
* The feature id for the 'Query' containment reference list.
*
*
* @generated
* @ordered
*/
int GET_VERSIONED_FEATURE_TYPE__QUERY = WfsPackage.GET_FEATURE_TYPE__QUERY;
/**
* The feature id for the 'Max Features' attribute.
*
*
* @generated
* @ordered
*/
int GET_VERSIONED_FEATURE_TYPE__MAX_FEATURES = WfsPackage.GET_FEATURE_TYPE__MAX_FEATURES;
/**
* The feature id for the 'Output Format' attribute.
*
*
* @generated
* @ordered
*/
int GET_VERSIONED_FEATURE_TYPE__OUTPUT_FORMAT = WfsPackage.GET_FEATURE_TYPE__OUTPUT_FORMAT;
/**
* The feature id for the 'Result Type' attribute.
*
*
* @generated
* @ordered
*/
int GET_VERSIONED_FEATURE_TYPE__RESULT_TYPE = WfsPackage.GET_FEATURE_TYPE__RESULT_TYPE;
/**
* The feature id for the 'Traverse Xlink Depth' attribute.
*
*
* @generated
* @ordered
*/
int GET_VERSIONED_FEATURE_TYPE__TRAVERSE_XLINK_DEPTH = WfsPackage.GET_FEATURE_TYPE__TRAVERSE_XLINK_DEPTH;
/**
* The feature id for the 'Traverse Xlink Expiry' attribute.
*
*
* @generated
* @ordered
*/
int GET_VERSIONED_FEATURE_TYPE__TRAVERSE_XLINK_EXPIRY = WfsPackage.GET_FEATURE_TYPE__TRAVERSE_XLINK_EXPIRY;
/**
* The number of structural features of the 'Get Versioned Feature Type' class.
*
*
* @generated
* @ordered
*/
int GET_VERSIONED_FEATURE_TYPE_FEATURE_COUNT = WfsPackage.GET_FEATURE_TYPE_FEATURE_COUNT + 0;
/**
* The meta object id for the '{@link net.opengis.wfsv.impl.RollbackTypeImpl Rollback Type}' class.
*
*
* @see net.opengis.wfsv.impl.RollbackTypeImpl
* @see net.opengis.wfsv.impl.WfsvPackageImpl#getRollbackType()
* @generated
*/
int ROLLBACK_TYPE = 7;
/**
* The feature id for the 'Safe To Ignore' attribute.
*
*
* @generated
* @ordered
*/
int ROLLBACK_TYPE__SAFE_TO_IGNORE = WfsPackage.NATIVE_TYPE__SAFE_TO_IGNORE;
/**
* The feature id for the 'Vendor Id' attribute.
*
*
* @generated
* @ordered
*/
int ROLLBACK_TYPE__VENDOR_ID = WfsPackage.NATIVE_TYPE__VENDOR_ID;
/**
* The feature id for the 'Filter' attribute.
*
*
* @generated
* @ordered
*/
int ROLLBACK_TYPE__FILTER = WfsPackage.NATIVE_TYPE_FEATURE_COUNT + 0;
/**
* The feature id for the 'Handle' attribute.
*
*
* @generated
* @ordered
*/
int ROLLBACK_TYPE__HANDLE = WfsPackage.NATIVE_TYPE_FEATURE_COUNT + 1;
/**
* The feature id for the 'To Feature Version' attribute.
*
*
* @generated
* @ordered
*/
int ROLLBACK_TYPE__TO_FEATURE_VERSION = WfsPackage.NATIVE_TYPE_FEATURE_COUNT + 2;
/**
* The feature id for the 'Type Name' attribute.
*
*
* @generated
* @ordered
*/
int ROLLBACK_TYPE__TYPE_NAME = WfsPackage.NATIVE_TYPE_FEATURE_COUNT + 3;
/**
* The feature id for the 'User' attribute.
*
*
* @generated
* @ordered
*/
int ROLLBACK_TYPE__USER = WfsPackage.NATIVE_TYPE_FEATURE_COUNT + 4;
/**
* The number of structural features of the 'Rollback Type' class.
*
*
* @generated
* @ordered
*/
int ROLLBACK_TYPE_FEATURE_COUNT = WfsPackage.NATIVE_TYPE_FEATURE_COUNT + 5;
/**
* The meta object id for the '{@link net.opengis.wfsv.impl.VersionedDeleteElementTypeImpl Versioned Delete Element Type}' class.
*
*
* @see net.opengis.wfsv.impl.VersionedDeleteElementTypeImpl
* @see net.opengis.wfsv.impl.WfsvPackageImpl#getVersionedDeleteElementType()
* @generated
*/
int VERSIONED_DELETE_ELEMENT_TYPE = 8;
/**
* The feature id for the 'Filter' attribute.
*
*
* @generated
* @ordered
*/
int VERSIONED_DELETE_ELEMENT_TYPE__FILTER = WfsPackage.DELETE_ELEMENT_TYPE__FILTER;
/**
* The feature id for the 'Handle' attribute.
*
*
* @generated
* @ordered
*/
int VERSIONED_DELETE_ELEMENT_TYPE__HANDLE = WfsPackage.DELETE_ELEMENT_TYPE__HANDLE;
/**
* The feature id for the 'Type Name' attribute.
*
*
* @generated
* @ordered
*/
int VERSIONED_DELETE_ELEMENT_TYPE__TYPE_NAME = WfsPackage.DELETE_ELEMENT_TYPE__TYPE_NAME;
/**
* The feature id for the 'Feature Version' attribute.
*
*
* @generated
* @ordered
*/
int VERSIONED_DELETE_ELEMENT_TYPE__FEATURE_VERSION = WfsPackage.DELETE_ELEMENT_TYPE_FEATURE_COUNT + 0;
/**
* The number of structural features of the 'Versioned Delete Element Type' class.
*
*
* @generated
* @ordered
*/
int VERSIONED_DELETE_ELEMENT_TYPE_FEATURE_COUNT = WfsPackage.DELETE_ELEMENT_TYPE_FEATURE_COUNT + 1;
/**
* The meta object id for the '{@link net.opengis.wfsv.impl.VersionedFeatureCollectionTypeImpl Versioned Feature Collection Type}' class.
*
*
* @see net.opengis.wfsv.impl.VersionedFeatureCollectionTypeImpl
* @see net.opengis.wfsv.impl.WfsvPackageImpl#getVersionedFeatureCollectionType()
* @generated
*/
int VERSIONED_FEATURE_COLLECTION_TYPE = 9;
/**
* The feature id for the 'Version' attribute.
*
*
* @generated
* @ordered
*/
int VERSIONED_FEATURE_COLLECTION_TYPE__VERSION = WfsPackage.FEATURE_COLLECTION_TYPE_FEATURE_COUNT + 0;
/**
* The number of structural features of the 'Versioned Feature Collection Type' class.
*
*
* @generated
* @ordered
*/
int VERSIONED_FEATURE_COLLECTION_TYPE_FEATURE_COUNT = WfsPackage.FEATURE_COLLECTION_TYPE_FEATURE_COUNT + 1;
/**
* The meta object id for the '{@link net.opengis.wfsv.impl.VersionedUpdateElementTypeImpl Versioned Update Element Type}' class.
*
*
* @see net.opengis.wfsv.impl.VersionedUpdateElementTypeImpl
* @see net.opengis.wfsv.impl.WfsvPackageImpl#getVersionedUpdateElementType()
* @generated
*/
int VERSIONED_UPDATE_ELEMENT_TYPE = 10;
/**
* The feature id for the 'Property' containment reference list.
*
*
* @generated
* @ordered
*/
int VERSIONED_UPDATE_ELEMENT_TYPE__PROPERTY = WfsPackage.UPDATE_ELEMENT_TYPE__PROPERTY;
/**
* The feature id for the 'Filter' attribute.
*
*
* @generated
* @ordered
*/
int VERSIONED_UPDATE_ELEMENT_TYPE__FILTER = WfsPackage.UPDATE_ELEMENT_TYPE__FILTER;
/**
* The feature id for the 'Handle' attribute.
*
*
* @generated
* @ordered
*/
int VERSIONED_UPDATE_ELEMENT_TYPE__HANDLE = WfsPackage.UPDATE_ELEMENT_TYPE__HANDLE;
/**
* The feature id for the 'Input Format' attribute.
*
*
* @generated
* @ordered
*/
int VERSIONED_UPDATE_ELEMENT_TYPE__INPUT_FORMAT = WfsPackage.UPDATE_ELEMENT_TYPE__INPUT_FORMAT;
/**
* The feature id for the 'Srs Name' attribute.
*
*
* @generated
* @ordered
*/
int VERSIONED_UPDATE_ELEMENT_TYPE__SRS_NAME = WfsPackage.UPDATE_ELEMENT_TYPE__SRS_NAME;
/**
* The feature id for the 'Type Name' attribute.
*
*
* @generated
* @ordered
*/
int VERSIONED_UPDATE_ELEMENT_TYPE__TYPE_NAME = WfsPackage.UPDATE_ELEMENT_TYPE__TYPE_NAME;
/**
* The feature id for the 'Feature Version' attribute.
*
*
* @generated
* @ordered
*/
int VERSIONED_UPDATE_ELEMENT_TYPE__FEATURE_VERSION = WfsPackage.UPDATE_ELEMENT_TYPE_FEATURE_COUNT + 0;
/**
* The number of structural features of the 'Versioned Update Element Type' class.
*
*
* @generated
* @ordered
*/
int VERSIONED_UPDATE_ELEMENT_TYPE_FEATURE_COUNT = WfsPackage.UPDATE_ELEMENT_TYPE_FEATURE_COUNT + 1;
/**
* Returns the meta object for class '{@link net.opengis.wfsv.AbstractVersionedFeatureType Abstract Versioned Feature Type}'.
*
*
* @return the meta object for class 'Abstract Versioned Feature Type'.
* @see net.opengis.wfsv.AbstractVersionedFeatureType
* @generated
*/
EClass getAbstractVersionedFeatureType();
/**
* Returns the meta object for the attribute '{@link net.opengis.wfsv.AbstractVersionedFeatureType#getVersion Version}'.
*
*
* @return the meta object for the attribute 'Version'.
* @see net.opengis.wfsv.AbstractVersionedFeatureType#getVersion()
* @see #getAbstractVersionedFeatureType()
* @generated
*/
EAttribute getAbstractVersionedFeatureType_Version();
/**
* Returns the meta object for the attribute '{@link net.opengis.wfsv.AbstractVersionedFeatureType#getAuthor Author}'.
*
*
* @return the meta object for the attribute 'Author'.
* @see net.opengis.wfsv.AbstractVersionedFeatureType#getAuthor()
* @see #getAbstractVersionedFeatureType()
* @generated
*/
EAttribute getAbstractVersionedFeatureType_Author();
/**
* Returns the meta object for the attribute '{@link net.opengis.wfsv.AbstractVersionedFeatureType#getDate Date}'.
*
*
* @return the meta object for the attribute 'Date'.
* @see net.opengis.wfsv.AbstractVersionedFeatureType#getDate()
* @see #getAbstractVersionedFeatureType()
* @generated
*/
EAttribute getAbstractVersionedFeatureType_Date();
/**
* Returns the meta object for the attribute '{@link net.opengis.wfsv.AbstractVersionedFeatureType#getMessage Message}'.
*
*
* @return the meta object for the attribute 'Message'.
* @see net.opengis.wfsv.AbstractVersionedFeatureType#getMessage()
* @see #getAbstractVersionedFeatureType()
* @generated
*/
EAttribute getAbstractVersionedFeatureType_Message();
/**
* Returns the meta object for class '{@link net.opengis.wfsv.DescribeVersionedFeatureTypeType Describe Versioned Feature Type Type}'.
*
*
* @return the meta object for class 'Describe Versioned Feature Type Type'.
* @see net.opengis.wfsv.DescribeVersionedFeatureTypeType
* @generated
*/
EClass getDescribeVersionedFeatureTypeType();
/**
* Returns the meta object for the attribute '{@link net.opengis.wfsv.DescribeVersionedFeatureTypeType#isVersioned Versioned}'.
*
*
* @return the meta object for the attribute 'Versioned'.
* @see net.opengis.wfsv.DescribeVersionedFeatureTypeType#isVersioned()
* @see #getDescribeVersionedFeatureTypeType()
* @generated
*/
EAttribute getDescribeVersionedFeatureTypeType_Versioned();
/**
* Returns the meta object for class '{@link net.opengis.wfsv.DifferenceQueryType Difference Query Type}'.
*
*
* @return the meta object for class 'Difference Query Type'.
* @see net.opengis.wfsv.DifferenceQueryType
* @generated
*/
EClass getDifferenceQueryType();
/**
* Returns the meta object for the attribute '{@link net.opengis.wfsv.DifferenceQueryType#getFilter Filter}'.
*
*
* @return the meta object for the attribute 'Filter'.
* @see net.opengis.wfsv.DifferenceQueryType#getFilter()
* @see #getDifferenceQueryType()
* @generated
*/
EAttribute getDifferenceQueryType_Filter();
/**
* Returns the meta object for the attribute '{@link net.opengis.wfsv.DifferenceQueryType#getFromFeatureVersion From Feature Version}'.
*
*
* @return the meta object for the attribute 'From Feature Version'.
* @see net.opengis.wfsv.DifferenceQueryType#getFromFeatureVersion()
* @see #getDifferenceQueryType()
* @generated
*/
EAttribute getDifferenceQueryType_FromFeatureVersion();
/**
* Returns the meta object for the attribute '{@link net.opengis.wfsv.DifferenceQueryType#getSrsName Srs Name}'.
*
*
* @return the meta object for the attribute 'Srs Name'.
* @see net.opengis.wfsv.DifferenceQueryType#getSrsName()
* @see #getDifferenceQueryType()
* @generated
*/
EAttribute getDifferenceQueryType_SrsName();
/**
* Returns the meta object for the attribute '{@link net.opengis.wfsv.DifferenceQueryType#getToFeatureVersion To Feature Version}'.
*
*
* @return the meta object for the attribute 'To Feature Version'.
* @see net.opengis.wfsv.DifferenceQueryType#getToFeatureVersion()
* @see #getDifferenceQueryType()
* @generated
*/
EAttribute getDifferenceQueryType_ToFeatureVersion();
/**
* Returns the meta object for the attribute '{@link net.opengis.wfsv.DifferenceQueryType#getTypeName Type Name}'.
*
*
* @return the meta object for the attribute 'Type Name'.
* @see net.opengis.wfsv.DifferenceQueryType#getTypeName()
* @see #getDifferenceQueryType()
* @generated
*/
EAttribute getDifferenceQueryType_TypeName();
/**
* Returns the meta object for class '{@link net.opengis.wfsv.DocumentRoot Document Root}'.
*
*
* @return the meta object for class 'Document Root'.
* @see net.opengis.wfsv.DocumentRoot
* @generated
*/
EClass getDocumentRoot();
/**
* Returns the meta object for the attribute list '{@link net.opengis.wfsv.DocumentRoot#getMixed Mixed}'.
*
*
* @return the meta object for the attribute list 'Mixed'.
* @see net.opengis.wfsv.DocumentRoot#getMixed()
* @see #getDocumentRoot()
* @generated
*/
EAttribute getDocumentRoot_Mixed();
/**
* Returns the meta object for the map '{@link net.opengis.wfsv.DocumentRoot#getXMLNSPrefixMap XMLNS Prefix Map}'.
*
*
* @return the meta object for the map 'XMLNS Prefix Map'.
* @see net.opengis.wfsv.DocumentRoot#getXMLNSPrefixMap()
* @see #getDocumentRoot()
* @generated
*/
EReference getDocumentRoot_XMLNSPrefixMap();
/**
* Returns the meta object for the map '{@link net.opengis.wfsv.DocumentRoot#getXSISchemaLocation XSI Schema Location}'.
*
*
* @return the meta object for the map 'XSI Schema Location'.
* @see net.opengis.wfsv.DocumentRoot#getXSISchemaLocation()
* @see #getDocumentRoot()
* @generated
*/
EReference getDocumentRoot_XSISchemaLocation();
/**
* Returns the meta object for the attribute '{@link net.opengis.wfsv.DocumentRoot#getDescribeVersionedFeatureType Describe Versioned Feature Type}'.
*
*
* @return the meta object for the attribute 'Describe Versioned Feature Type'.
* @see net.opengis.wfsv.DocumentRoot#getDescribeVersionedFeatureType()
* @see #getDocumentRoot()
* @generated
*/
EAttribute getDocumentRoot_DescribeVersionedFeatureType();
/**
* Returns the meta object for the containment reference '{@link net.opengis.wfsv.DocumentRoot#getDifferenceQuery Difference Query}'.
*
*
* @return the meta object for the containment reference 'Difference Query'.
* @see net.opengis.wfsv.DocumentRoot#getDifferenceQuery()
* @see #getDocumentRoot()
* @generated
*/
EReference getDocumentRoot_DifferenceQuery();
/**
* Returns the meta object for the containment reference '{@link net.opengis.wfsv.DocumentRoot#getGetDiff Get Diff}'.
*
*
* @return the meta object for the containment reference 'Get Diff'.
* @see net.opengis.wfsv.DocumentRoot#getGetDiff()
* @see #getDocumentRoot()
* @generated
*/
EReference getDocumentRoot_GetDiff();
/**
* Returns the meta object for the containment reference '{@link net.opengis.wfsv.DocumentRoot#getGetLog Get Log}'.
*
*
* @return the meta object for the containment reference 'Get Log'.
* @see net.opengis.wfsv.DocumentRoot#getGetLog()
* @see #getDocumentRoot()
* @generated
*/
EReference getDocumentRoot_GetLog();
/**
* Returns the meta object for the containment reference '{@link net.opengis.wfsv.DocumentRoot#getGetVersionedFeature Get Versioned Feature}'.
*
*
* @return the meta object for the containment reference 'Get Versioned Feature'.
* @see net.opengis.wfsv.DocumentRoot#getGetVersionedFeature()
* @see #getDocumentRoot()
* @generated
*/
EReference getDocumentRoot_GetVersionedFeature();
/**
* Returns the meta object for the containment reference '{@link net.opengis.wfsv.DocumentRoot#getRollback Rollback}'.
*
*
* @return the meta object for the containment reference 'Rollback'.
* @see net.opengis.wfsv.DocumentRoot#getRollback()
* @see #getDocumentRoot()
* @generated
*/
EReference getDocumentRoot_Rollback();
/**
* Returns the meta object for the containment reference '{@link net.opengis.wfsv.DocumentRoot#getVersionedDelete Versioned Delete}'.
*
*
* @return the meta object for the containment reference 'Versioned Delete'.
* @see net.opengis.wfsv.DocumentRoot#getVersionedDelete()
* @see #getDocumentRoot()
* @generated
*/
EReference getDocumentRoot_VersionedDelete();
/**
* Returns the meta object for the containment reference '{@link net.opengis.wfsv.DocumentRoot#getVersionedFeatureCollection Versioned Feature Collection}'.
*
*
* @return the meta object for the containment reference 'Versioned Feature Collection'.
* @see net.opengis.wfsv.DocumentRoot#getVersionedFeatureCollection()
* @see #getDocumentRoot()
* @generated
*/
EReference getDocumentRoot_VersionedFeatureCollection();
/**
* Returns the meta object for the containment reference '{@link net.opengis.wfsv.DocumentRoot#getVersionedUpdate Versioned Update}'.
*
*
* @return the meta object for the containment reference 'Versioned Update'.
* @see net.opengis.wfsv.DocumentRoot#getVersionedUpdate()
* @see #getDocumentRoot()
* @generated
*/
EReference getDocumentRoot_VersionedUpdate();
/**
* Returns the meta object for class '{@link net.opengis.wfsv.GetDiffType Get Diff Type}'.
*
*
* @return the meta object for class 'Get Diff Type'.
* @see net.opengis.wfsv.GetDiffType
* @generated
*/
EClass getGetDiffType();
/**
* Returns the meta object for the containment reference list '{@link net.opengis.wfsv.GetDiffType#getDifferenceQuery Difference Query}'.
*
*
* @return the meta object for the containment reference list 'Difference Query'.
* @see net.opengis.wfsv.GetDiffType#getDifferenceQuery()
* @see #getGetDiffType()
* @generated
*/
EReference getGetDiffType_DifferenceQuery();
/**
* Returns the meta object for the attribute '{@link net.opengis.wfsv.GetDiffType#getOutputFormat Output Format}'.
*
*
* @return the meta object for the attribute 'Output Format'.
* @see net.opengis.wfsv.GetDiffType#getOutputFormat()
* @see #getGetDiffType()
* @generated
*/
EAttribute getGetDiffType_OutputFormat();
/**
* Returns the meta object for class '{@link net.opengis.wfsv.GetLogType Get Log Type}'.
*
*
* @return the meta object for class 'Get Log Type'.
* @see net.opengis.wfsv.GetLogType
* @generated
*/
EClass getGetLogType();
/**
* Returns the meta object for the containment reference list '{@link net.opengis.wfsv.GetLogType#getDifferenceQuery Difference Query}'.
*
*
* @return the meta object for the containment reference list 'Difference Query'.
* @see net.opengis.wfsv.GetLogType#getDifferenceQuery()
* @see #getGetLogType()
* @generated
*/
EReference getGetLogType_DifferenceQuery();
/**
* Returns the meta object for the attribute '{@link net.opengis.wfsv.GetLogType#getMaxFeatures Max Features}'.
*
*
* @return the meta object for the attribute 'Max Features'.
* @see net.opengis.wfsv.GetLogType#getMaxFeatures()
* @see #getGetLogType()
* @generated
*/
EAttribute getGetLogType_MaxFeatures();
/**
* Returns the meta object for the attribute '{@link net.opengis.wfsv.GetLogType#getOutputFormat Output Format}'.
*
*
* @return the meta object for the attribute 'Output Format'.
* @see net.opengis.wfsv.GetLogType#getOutputFormat()
* @see #getGetLogType()
* @generated
*/
EAttribute getGetLogType_OutputFormat();
/**
* Returns the meta object for the attribute '{@link net.opengis.wfsv.GetLogType#getResultType Result Type}'.
*
*
* @return the meta object for the attribute 'Result Type'.
* @see net.opengis.wfsv.GetLogType#getResultType()
* @see #getGetLogType()
* @generated
*/
EAttribute getGetLogType_ResultType();
/**
* Returns the meta object for class '{@link net.opengis.wfsv.GetVersionedFeatureType Get Versioned Feature Type}'.
*
*
* @return the meta object for class 'Get Versioned Feature Type'.
* @see net.opengis.wfsv.GetVersionedFeatureType
* @generated
*/
EClass getGetVersionedFeatureType();
/**
* Returns the meta object for class '{@link net.opengis.wfsv.RollbackType Rollback Type}'.
*
*
* @return the meta object for class 'Rollback Type'.
* @see net.opengis.wfsv.RollbackType
* @generated
*/
EClass getRollbackType();
/**
* Returns the meta object for the attribute '{@link net.opengis.wfsv.RollbackType#getFilter Filter}'.
*
*
* @return the meta object for the attribute 'Filter'.
* @see net.opengis.wfsv.RollbackType#getFilter()
* @see #getRollbackType()
* @generated
*/
EAttribute getRollbackType_Filter();
/**
* Returns the meta object for the attribute '{@link net.opengis.wfsv.RollbackType#getHandle Handle}'.
*
*
* @return the meta object for the attribute 'Handle'.
* @see net.opengis.wfsv.RollbackType#getHandle()
* @see #getRollbackType()
* @generated
*/
EAttribute getRollbackType_Handle();
/**
* Returns the meta object for the attribute '{@link net.opengis.wfsv.RollbackType#getToFeatureVersion To Feature Version}'.
*
*
* @return the meta object for the attribute 'To Feature Version'.
* @see net.opengis.wfsv.RollbackType#getToFeatureVersion()
* @see #getRollbackType()
* @generated
*/
EAttribute getRollbackType_ToFeatureVersion();
/**
* Returns the meta object for the attribute '{@link net.opengis.wfsv.RollbackType#getTypeName Type Name}'.
*
*
* @return the meta object for the attribute 'Type Name'.
* @see net.opengis.wfsv.RollbackType#getTypeName()
* @see #getRollbackType()
* @generated
*/
EAttribute getRollbackType_TypeName();
/**
* Returns the meta object for the attribute '{@link net.opengis.wfsv.RollbackType#getUser User}'.
*
*
* @return the meta object for the attribute 'User'.
* @see net.opengis.wfsv.RollbackType#getUser()
* @see #getRollbackType()
* @generated
*/
EAttribute getRollbackType_User();
/**
* Returns the meta object for class '{@link net.opengis.wfsv.VersionedDeleteElementType Versioned Delete Element Type}'.
*
*
* @return the meta object for class 'Versioned Delete Element Type'.
* @see net.opengis.wfsv.VersionedDeleteElementType
* @generated
*/
EClass getVersionedDeleteElementType();
/**
* Returns the meta object for the attribute '{@link net.opengis.wfsv.VersionedDeleteElementType#getFeatureVersion Feature Version}'.
*
*
* @return the meta object for the attribute 'Feature Version'.
* @see net.opengis.wfsv.VersionedDeleteElementType#getFeatureVersion()
* @see #getVersionedDeleteElementType()
* @generated
*/
EAttribute getVersionedDeleteElementType_FeatureVersion();
/**
* Returns the meta object for class '{@link net.opengis.wfsv.VersionedFeatureCollectionType Versioned Feature Collection Type}'.
*
*
* @return the meta object for class 'Versioned Feature Collection Type'.
* @see net.opengis.wfsv.VersionedFeatureCollectionType
* @generated
*/
EClass getVersionedFeatureCollectionType();
/**
* Returns the meta object for the attribute '{@link net.opengis.wfsv.VersionedFeatureCollectionType#getVersion Version}'.
*
*
* @return the meta object for the attribute 'Version'.
* @see net.opengis.wfsv.VersionedFeatureCollectionType#getVersion()
* @see #getVersionedFeatureCollectionType()
* @generated
*/
EAttribute getVersionedFeatureCollectionType_Version();
/**
* Returns the meta object for class '{@link net.opengis.wfsv.VersionedUpdateElementType Versioned Update Element Type}'.
*
*
* @return the meta object for class 'Versioned Update Element Type'.
* @see net.opengis.wfsv.VersionedUpdateElementType
* @generated
*/
EClass getVersionedUpdateElementType();
/**
* Returns the meta object for the attribute '{@link net.opengis.wfsv.VersionedUpdateElementType#getFeatureVersion Feature Version}'.
*
*
* @return the meta object for the attribute 'Feature Version'.
* @see net.opengis.wfsv.VersionedUpdateElementType#getFeatureVersion()
* @see #getVersionedUpdateElementType()
* @generated
*/
EAttribute getVersionedUpdateElementType_FeatureVersion();
/**
* Returns the factory that creates the instances of the model.
*
*
* @return the factory that creates the instances of the model.
* @generated
*/
WfsvFactory getWfsvFactory();
/**
*
* Defines literals for the meta objects that represent
*
* - each class,
* - each feature of each class,
* - each enum,
* - and each data type
*
*
* @generated
*/
interface Literals {
/**
* The meta object literal for the '{@link net.opengis.wfsv.impl.AbstractVersionedFeatureTypeImpl Abstract Versioned Feature Type}' class.
*
*
* @see net.opengis.wfsv.impl.AbstractVersionedFeatureTypeImpl
* @see net.opengis.wfsv.impl.WfsvPackageImpl#getAbstractVersionedFeatureType()
* @generated
*/
EClass ABSTRACT_VERSIONED_FEATURE_TYPE = eINSTANCE.getAbstractVersionedFeatureType();
/**
* The meta object literal for the 'Version' attribute feature.
*
*
* @generated
*/
EAttribute ABSTRACT_VERSIONED_FEATURE_TYPE__VERSION = eINSTANCE.getAbstractVersionedFeatureType_Version();
/**
* The meta object literal for the 'Author' attribute feature.
*
*
* @generated
*/
EAttribute ABSTRACT_VERSIONED_FEATURE_TYPE__AUTHOR = eINSTANCE.getAbstractVersionedFeatureType_Author();
/**
* The meta object literal for the 'Date' attribute feature.
*
*
* @generated
*/
EAttribute ABSTRACT_VERSIONED_FEATURE_TYPE__DATE = eINSTANCE.getAbstractVersionedFeatureType_Date();
/**
* The meta object literal for the 'Message' attribute feature.
*
*
* @generated
*/
EAttribute ABSTRACT_VERSIONED_FEATURE_TYPE__MESSAGE = eINSTANCE.getAbstractVersionedFeatureType_Message();
/**
* The meta object literal for the '{@link net.opengis.wfsv.impl.DescribeVersionedFeatureTypeTypeImpl Describe Versioned Feature Type Type}' class.
*
*
* @see net.opengis.wfsv.impl.DescribeVersionedFeatureTypeTypeImpl
* @see net.opengis.wfsv.impl.WfsvPackageImpl#getDescribeVersionedFeatureTypeType()
* @generated
*/
EClass DESCRIBE_VERSIONED_FEATURE_TYPE_TYPE = eINSTANCE.getDescribeVersionedFeatureTypeType();
/**
* The meta object literal for the 'Versioned' attribute feature.
*
*
* @generated
*/
EAttribute DESCRIBE_VERSIONED_FEATURE_TYPE_TYPE__VERSIONED = eINSTANCE.getDescribeVersionedFeatureTypeType_Versioned();
/**
* The meta object literal for the '{@link net.opengis.wfsv.impl.DifferenceQueryTypeImpl Difference Query Type}' class.
*
*
* @see net.opengis.wfsv.impl.DifferenceQueryTypeImpl
* @see net.opengis.wfsv.impl.WfsvPackageImpl#getDifferenceQueryType()
* @generated
*/
EClass DIFFERENCE_QUERY_TYPE = eINSTANCE.getDifferenceQueryType();
/**
* The meta object literal for the 'Filter' attribute feature.
*
*
* @generated
*/
EAttribute DIFFERENCE_QUERY_TYPE__FILTER = eINSTANCE.getDifferenceQueryType_Filter();
/**
* The meta object literal for the 'From Feature Version' attribute feature.
*
*
* @generated
*/
EAttribute DIFFERENCE_QUERY_TYPE__FROM_FEATURE_VERSION = eINSTANCE.getDifferenceQueryType_FromFeatureVersion();
/**
* The meta object literal for the 'Srs Name' attribute feature.
*
*
* @generated
*/
EAttribute DIFFERENCE_QUERY_TYPE__SRS_NAME = eINSTANCE.getDifferenceQueryType_SrsName();
/**
* The meta object literal for the 'To Feature Version' attribute feature.
*
*
* @generated
*/
EAttribute DIFFERENCE_QUERY_TYPE__TO_FEATURE_VERSION = eINSTANCE.getDifferenceQueryType_ToFeatureVersion();
/**
* The meta object literal for the 'Type Name' attribute feature.
*
*
* @generated
*/
EAttribute DIFFERENCE_QUERY_TYPE__TYPE_NAME = eINSTANCE.getDifferenceQueryType_TypeName();
/**
* The meta object literal for the '{@link net.opengis.wfsv.impl.DocumentRootImpl Document Root}' class.
*
*
* @see net.opengis.wfsv.impl.DocumentRootImpl
* @see net.opengis.wfsv.impl.WfsvPackageImpl#getDocumentRoot()
* @generated
*/
EClass DOCUMENT_ROOT = eINSTANCE.getDocumentRoot();
/**
* The meta object literal for the 'Mixed' attribute list feature.
*
*
* @generated
*/
EAttribute DOCUMENT_ROOT__MIXED = eINSTANCE.getDocumentRoot_Mixed();
/**
* The meta object literal for the 'XMLNS Prefix Map' map feature.
*
*
* @generated
*/
EReference DOCUMENT_ROOT__XMLNS_PREFIX_MAP = eINSTANCE.getDocumentRoot_XMLNSPrefixMap();
/**
* The meta object literal for the 'XSI Schema Location' map feature.
*
*
* @generated
*/
EReference DOCUMENT_ROOT__XSI_SCHEMA_LOCATION = eINSTANCE.getDocumentRoot_XSISchemaLocation();
/**
* The meta object literal for the 'Describe Versioned Feature Type' attribute feature.
*
*
* @generated
*/
EAttribute DOCUMENT_ROOT__DESCRIBE_VERSIONED_FEATURE_TYPE = eINSTANCE.getDocumentRoot_DescribeVersionedFeatureType();
/**
* The meta object literal for the 'Difference Query' containment reference feature.
*
*
* @generated
*/
EReference DOCUMENT_ROOT__DIFFERENCE_QUERY = eINSTANCE.getDocumentRoot_DifferenceQuery();
/**
* The meta object literal for the 'Get Diff' containment reference feature.
*
*
* @generated
*/
EReference DOCUMENT_ROOT__GET_DIFF = eINSTANCE.getDocumentRoot_GetDiff();
/**
* The meta object literal for the 'Get Log' containment reference feature.
*
*
* @generated
*/
EReference DOCUMENT_ROOT__GET_LOG = eINSTANCE.getDocumentRoot_GetLog();
/**
* The meta object literal for the 'Get Versioned Feature' containment reference feature.
*
*
* @generated
*/
EReference DOCUMENT_ROOT__GET_VERSIONED_FEATURE = eINSTANCE.getDocumentRoot_GetVersionedFeature();
/**
* The meta object literal for the 'Rollback' containment reference feature.
*
*
* @generated
*/
EReference DOCUMENT_ROOT__ROLLBACK = eINSTANCE.getDocumentRoot_Rollback();
/**
* The meta object literal for the 'Versioned Delete' containment reference feature.
*
*
* @generated
*/
EReference DOCUMENT_ROOT__VERSIONED_DELETE = eINSTANCE.getDocumentRoot_VersionedDelete();
/**
* The meta object literal for the 'Versioned Feature Collection' containment reference feature.
*
*
* @generated
*/
EReference DOCUMENT_ROOT__VERSIONED_FEATURE_COLLECTION = eINSTANCE.getDocumentRoot_VersionedFeatureCollection();
/**
* The meta object literal for the 'Versioned Update' containment reference feature.
*
*
* @generated
*/
EReference DOCUMENT_ROOT__VERSIONED_UPDATE = eINSTANCE.getDocumentRoot_VersionedUpdate();
/**
* The meta object literal for the '{@link net.opengis.wfsv.impl.GetDiffTypeImpl Get Diff Type}' class.
*
*
* @see net.opengis.wfsv.impl.GetDiffTypeImpl
* @see net.opengis.wfsv.impl.WfsvPackageImpl#getGetDiffType()
* @generated
*/
EClass GET_DIFF_TYPE = eINSTANCE.getGetDiffType();
/**
* The meta object literal for the 'Difference Query' containment reference list feature.
*
*
* @generated
*/
EReference GET_DIFF_TYPE__DIFFERENCE_QUERY = eINSTANCE.getGetDiffType_DifferenceQuery();
/**
* The meta object literal for the 'Output Format' attribute feature.
*
*
* @generated
*/
EAttribute GET_DIFF_TYPE__OUTPUT_FORMAT = eINSTANCE.getGetDiffType_OutputFormat();
/**
* The meta object literal for the '{@link net.opengis.wfsv.impl.GetLogTypeImpl Get Log Type}' class.
*
*
* @see net.opengis.wfsv.impl.GetLogTypeImpl
* @see net.opengis.wfsv.impl.WfsvPackageImpl#getGetLogType()
* @generated
*/
EClass GET_LOG_TYPE = eINSTANCE.getGetLogType();
/**
* The meta object literal for the 'Difference Query' containment reference list feature.
*
*
* @generated
*/
EReference GET_LOG_TYPE__DIFFERENCE_QUERY = eINSTANCE.getGetLogType_DifferenceQuery();
/**
* The meta object literal for the 'Max Features' attribute feature.
*
*
* @generated
*/
EAttribute GET_LOG_TYPE__MAX_FEATURES = eINSTANCE.getGetLogType_MaxFeatures();
/**
* The meta object literal for the 'Output Format' attribute feature.
*
*
* @generated
*/
EAttribute GET_LOG_TYPE__OUTPUT_FORMAT = eINSTANCE.getGetLogType_OutputFormat();
/**
* The meta object literal for the 'Result Type' attribute feature.
*
*
* @generated
*/
EAttribute GET_LOG_TYPE__RESULT_TYPE = eINSTANCE.getGetLogType_ResultType();
/**
* The meta object literal for the '{@link net.opengis.wfsv.impl.GetVersionedFeatureTypeImpl Get Versioned Feature Type}' class.
*
*
* @see net.opengis.wfsv.impl.GetVersionedFeatureTypeImpl
* @see net.opengis.wfsv.impl.WfsvPackageImpl#getGetVersionedFeatureType()
* @generated
*/
EClass GET_VERSIONED_FEATURE_TYPE = eINSTANCE.getGetVersionedFeatureType();
/**
* The meta object literal for the '{@link net.opengis.wfsv.impl.RollbackTypeImpl Rollback Type}' class.
*
*
* @see net.opengis.wfsv.impl.RollbackTypeImpl
* @see net.opengis.wfsv.impl.WfsvPackageImpl#getRollbackType()
* @generated
*/
EClass ROLLBACK_TYPE = eINSTANCE.getRollbackType();
/**
* The meta object literal for the 'Filter' attribute feature.
*
*
* @generated
*/
EAttribute ROLLBACK_TYPE__FILTER = eINSTANCE.getRollbackType_Filter();
/**
* The meta object literal for the 'Handle' attribute feature.
*
*
* @generated
*/
EAttribute ROLLBACK_TYPE__HANDLE = eINSTANCE.getRollbackType_Handle();
/**
* The meta object literal for the 'To Feature Version' attribute feature.
*
*
* @generated
*/
EAttribute ROLLBACK_TYPE__TO_FEATURE_VERSION = eINSTANCE.getRollbackType_ToFeatureVersion();
/**
* The meta object literal for the 'Type Name' attribute feature.
*
*
* @generated
*/
EAttribute ROLLBACK_TYPE__TYPE_NAME = eINSTANCE.getRollbackType_TypeName();
/**
* The meta object literal for the 'User' attribute feature.
*
*
* @generated
*/
EAttribute ROLLBACK_TYPE__USER = eINSTANCE.getRollbackType_User();
/**
* The meta object literal for the '{@link net.opengis.wfsv.impl.VersionedDeleteElementTypeImpl Versioned Delete Element Type}' class.
*
*
* @see net.opengis.wfsv.impl.VersionedDeleteElementTypeImpl
* @see net.opengis.wfsv.impl.WfsvPackageImpl#getVersionedDeleteElementType()
* @generated
*/
EClass VERSIONED_DELETE_ELEMENT_TYPE = eINSTANCE.getVersionedDeleteElementType();
/**
* The meta object literal for the 'Feature Version' attribute feature.
*
*
* @generated
*/
EAttribute VERSIONED_DELETE_ELEMENT_TYPE__FEATURE_VERSION = eINSTANCE.getVersionedDeleteElementType_FeatureVersion();
/**
* The meta object literal for the '{@link net.opengis.wfsv.impl.VersionedFeatureCollectionTypeImpl Versioned Feature Collection Type}' class.
*
*
* @see net.opengis.wfsv.impl.VersionedFeatureCollectionTypeImpl
* @see net.opengis.wfsv.impl.WfsvPackageImpl#getVersionedFeatureCollectionType()
* @generated
*/
EClass VERSIONED_FEATURE_COLLECTION_TYPE = eINSTANCE.getVersionedFeatureCollectionType();
/**
* The meta object literal for the 'Version' attribute feature.
*
*
* @generated
*/
EAttribute VERSIONED_FEATURE_COLLECTION_TYPE__VERSION = eINSTANCE.getVersionedFeatureCollectionType_Version();
/**
* The meta object literal for the '{@link net.opengis.wfsv.impl.VersionedUpdateElementTypeImpl Versioned Update Element Type}' class.
*
*
* @see net.opengis.wfsv.impl.VersionedUpdateElementTypeImpl
* @see net.opengis.wfsv.impl.WfsvPackageImpl#getVersionedUpdateElementType()
* @generated
*/
EClass VERSIONED_UPDATE_ELEMENT_TYPE = eINSTANCE.getVersionedUpdateElementType();
/**
* The meta object literal for the 'Feature Version' attribute feature.
*
*
* @generated
*/
EAttribute VERSIONED_UPDATE_ELEMENT_TYPE__FEATURE_VERSION = eINSTANCE.getVersionedUpdateElementType_FeatureVersion();
}
} //WfsvPackage
© 2015 - 2025 Weber Informatics LLC | Privacy Policy