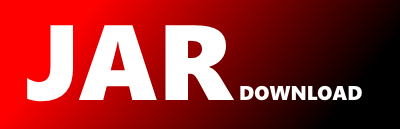
net.opengis.wfsv.util.WfsvAdapterFactory Maven / Gradle / Ivy
/**
*
*
*
* $Id: WfsvAdapterFactory.java 7988 2007-12-12 20:29:15Z aaime $
*/
package net.opengis.wfsv.util;
import net.opengis.wfs.BaseRequestType;
import net.opengis.wfs.DeleteElementType;
import net.opengis.wfs.DescribeFeatureTypeType;
import net.opengis.wfs.FeatureCollectionType;
import net.opengis.wfs.GetFeatureType;
import net.opengis.wfs.NativeType;
import net.opengis.wfs.UpdateElementType;
import net.opengis.wfsv.*;
import org.eclipse.emf.common.notify.Adapter;
import org.eclipse.emf.common.notify.Notifier;
import org.eclipse.emf.common.notify.impl.AdapterFactoryImpl;
import org.eclipse.emf.ecore.EObject;
/**
*
* The Adapter Factory for the model.
* It provides an adapter createXXX
method for each class of the model.
*
* @see net.opengis.wfsv.WfsvPackage
* @generated
*/
public class WfsvAdapterFactory extends AdapterFactoryImpl {
/**
* The cached model package.
*
*
* @generated
*/
protected static WfsvPackage modelPackage;
/**
* Creates an instance of the adapter factory.
*
*
* @generated
*/
public WfsvAdapterFactory() {
if (modelPackage == null) {
modelPackage = WfsvPackage.eINSTANCE;
}
}
/**
* Returns whether this factory is applicable for the type of the object.
*
* This implementation returns true
if the object is either the model's package or is an instance object of the model.
*
* @return whether this factory is applicable for the type of the object.
* @generated
*/
public boolean isFactoryForType(Object object) {
if (object == modelPackage) {
return true;
}
if (object instanceof EObject) {
return ((EObject)object).eClass().getEPackage() == modelPackage;
}
return false;
}
/**
* The switch the delegates to the createXXX
methods.
*
*
* @generated
*/
protected WfsvSwitch modelSwitch =
new WfsvSwitch() {
public Object caseAbstractVersionedFeatureType(AbstractVersionedFeatureType object) {
return createAbstractVersionedFeatureTypeAdapter();
}
public Object caseDescribeVersionedFeatureTypeType(DescribeVersionedFeatureTypeType object) {
return createDescribeVersionedFeatureTypeTypeAdapter();
}
public Object caseDifferenceQueryType(DifferenceQueryType object) {
return createDifferenceQueryTypeAdapter();
}
public Object caseDocumentRoot(DocumentRoot object) {
return createDocumentRootAdapter();
}
public Object caseGetDiffType(GetDiffType object) {
return createGetDiffTypeAdapter();
}
public Object caseGetLogType(GetLogType object) {
return createGetLogTypeAdapter();
}
public Object caseGetVersionedFeatureType(GetVersionedFeatureType object) {
return createGetVersionedFeatureTypeAdapter();
}
public Object caseRollbackType(RollbackType object) {
return createRollbackTypeAdapter();
}
public Object caseVersionedDeleteElementType(VersionedDeleteElementType object) {
return createVersionedDeleteElementTypeAdapter();
}
public Object caseVersionedFeatureCollectionType(VersionedFeatureCollectionType object) {
return createVersionedFeatureCollectionTypeAdapter();
}
public Object caseVersionedUpdateElementType(VersionedUpdateElementType object) {
return createVersionedUpdateElementTypeAdapter();
}
public Object caseBaseRequestType(BaseRequestType object) {
return createBaseRequestTypeAdapter();
}
public Object caseDescribeFeatureTypeType(DescribeFeatureTypeType object) {
return createDescribeFeatureTypeTypeAdapter();
}
public Object caseGetFeatureType(GetFeatureType object) {
return createGetFeatureTypeAdapter();
}
public Object caseNativeType(NativeType object) {
return createNativeTypeAdapter();
}
public Object caseDeleteElementType(DeleteElementType object) {
return createDeleteElementTypeAdapter();
}
public Object caseFeatureCollectionType(FeatureCollectionType object) {
return createFeatureCollectionTypeAdapter();
}
public Object caseUpdateElementType(UpdateElementType object) {
return createUpdateElementTypeAdapter();
}
public Object defaultCase(EObject object) {
return createEObjectAdapter();
}
};
/**
* Creates an adapter for the target
.
*
*
* @param target the object to adapt.
* @return the adapter for the target
.
* @generated
*/
public Adapter createAdapter(Notifier target) {
return (Adapter)modelSwitch.doSwitch((EObject)target);
}
/**
* Creates a new adapter for an object of class '{@link net.opengis.wfsv.AbstractVersionedFeatureType Abstract Versioned Feature Type}'.
*
* This default implementation returns null so that we can easily ignore cases;
* it's useful to ignore a case when inheritance will catch all the cases anyway.
*
* @return the new adapter.
* @see net.opengis.wfsv.AbstractVersionedFeatureType
* @generated
*/
public Adapter createAbstractVersionedFeatureTypeAdapter() {
return null;
}
/**
* Creates a new adapter for an object of class '{@link net.opengis.wfsv.DescribeVersionedFeatureTypeType Describe Versioned Feature Type Type}'.
*
* This default implementation returns null so that we can easily ignore cases;
* it's useful to ignore a case when inheritance will catch all the cases anyway.
*
* @return the new adapter.
* @see net.opengis.wfsv.DescribeVersionedFeatureTypeType
* @generated
*/
public Adapter createDescribeVersionedFeatureTypeTypeAdapter() {
return null;
}
/**
* Creates a new adapter for an object of class '{@link net.opengis.wfsv.DifferenceQueryType Difference Query Type}'.
*
* This default implementation returns null so that we can easily ignore cases;
* it's useful to ignore a case when inheritance will catch all the cases anyway.
*
* @return the new adapter.
* @see net.opengis.wfsv.DifferenceQueryType
* @generated
*/
public Adapter createDifferenceQueryTypeAdapter() {
return null;
}
/**
* Creates a new adapter for an object of class '{@link net.opengis.wfsv.DocumentRoot Document Root}'.
*
* This default implementation returns null so that we can easily ignore cases;
* it's useful to ignore a case when inheritance will catch all the cases anyway.
*
* @return the new adapter.
* @see net.opengis.wfsv.DocumentRoot
* @generated
*/
public Adapter createDocumentRootAdapter() {
return null;
}
/**
* Creates a new adapter for an object of class '{@link net.opengis.wfsv.GetDiffType Get Diff Type}'.
*
* This default implementation returns null so that we can easily ignore cases;
* it's useful to ignore a case when inheritance will catch all the cases anyway.
*
* @return the new adapter.
* @see net.opengis.wfsv.GetDiffType
* @generated
*/
public Adapter createGetDiffTypeAdapter() {
return null;
}
/**
* Creates a new adapter for an object of class '{@link net.opengis.wfsv.GetLogType Get Log Type}'.
*
* This default implementation returns null so that we can easily ignore cases;
* it's useful to ignore a case when inheritance will catch all the cases anyway.
*
* @return the new adapter.
* @see net.opengis.wfsv.GetLogType
* @generated
*/
public Adapter createGetLogTypeAdapter() {
return null;
}
/**
* Creates a new adapter for an object of class '{@link net.opengis.wfsv.GetVersionedFeatureType Get Versioned Feature Type}'.
*
* This default implementation returns null so that we can easily ignore cases;
* it's useful to ignore a case when inheritance will catch all the cases anyway.
*
* @return the new adapter.
* @see net.opengis.wfsv.GetVersionedFeatureType
* @generated
*/
public Adapter createGetVersionedFeatureTypeAdapter() {
return null;
}
/**
* Creates a new adapter for an object of class '{@link net.opengis.wfsv.RollbackType Rollback Type}'.
*
* This default implementation returns null so that we can easily ignore cases;
* it's useful to ignore a case when inheritance will catch all the cases anyway.
*
* @return the new adapter.
* @see net.opengis.wfsv.RollbackType
* @generated
*/
public Adapter createRollbackTypeAdapter() {
return null;
}
/**
* Creates a new adapter for an object of class '{@link net.opengis.wfsv.VersionedDeleteElementType Versioned Delete Element Type}'.
*
* This default implementation returns null so that we can easily ignore cases;
* it's useful to ignore a case when inheritance will catch all the cases anyway.
*
* @return the new adapter.
* @see net.opengis.wfsv.VersionedDeleteElementType
* @generated
*/
public Adapter createVersionedDeleteElementTypeAdapter() {
return null;
}
/**
* Creates a new adapter for an object of class '{@link net.opengis.wfsv.VersionedFeatureCollectionType Versioned Feature Collection Type}'.
*
* This default implementation returns null so that we can easily ignore cases;
* it's useful to ignore a case when inheritance will catch all the cases anyway.
*
* @return the new adapter.
* @see net.opengis.wfsv.VersionedFeatureCollectionType
* @generated
*/
public Adapter createVersionedFeatureCollectionTypeAdapter() {
return null;
}
/**
* Creates a new adapter for an object of class '{@link net.opengis.wfsv.VersionedUpdateElementType Versioned Update Element Type}'.
*
* This default implementation returns null so that we can easily ignore cases;
* it's useful to ignore a case when inheritance will catch all the cases anyway.
*
* @return the new adapter.
* @see net.opengis.wfsv.VersionedUpdateElementType
* @generated
*/
public Adapter createVersionedUpdateElementTypeAdapter() {
return null;
}
/**
* Creates a new adapter for an object of class '{@link net.opengis.wfs.BaseRequestType Base Request Type}'.
*
* This default implementation returns null so that we can easily ignore cases;
* it's useful to ignore a case when inheritance will catch all the cases anyway.
*
* @return the new adapter.
* @see net.opengis.wfs.BaseRequestType
* @generated
*/
public Adapter createBaseRequestTypeAdapter() {
return null;
}
/**
* Creates a new adapter for an object of class '{@link net.opengis.wfs.DescribeFeatureTypeType Describe Feature Type Type}'.
*
* This default implementation returns null so that we can easily ignore cases;
* it's useful to ignore a case when inheritance will catch all the cases anyway.
*
* @return the new adapter.
* @see net.opengis.wfs.DescribeFeatureTypeType
* @generated
*/
public Adapter createDescribeFeatureTypeTypeAdapter() {
return null;
}
/**
* Creates a new adapter for an object of class '{@link net.opengis.wfs.GetFeatureType Get Feature Type}'.
*
* This default implementation returns null so that we can easily ignore cases;
* it's useful to ignore a case when inheritance will catch all the cases anyway.
*
* @return the new adapter.
* @see net.opengis.wfs.GetFeatureType
* @generated
*/
public Adapter createGetFeatureTypeAdapter() {
return null;
}
/**
* Creates a new adapter for an object of class '{@link net.opengis.wfs.NativeType Native Type}'.
*
* This default implementation returns null so that we can easily ignore cases;
* it's useful to ignore a case when inheritance will catch all the cases anyway.
*
* @return the new adapter.
* @see net.opengis.wfs.NativeType
* @generated
*/
public Adapter createNativeTypeAdapter() {
return null;
}
/**
* Creates a new adapter for an object of class '{@link net.opengis.wfs.DeleteElementType Delete Element Type}'.
*
* This default implementation returns null so that we can easily ignore cases;
* it's useful to ignore a case when inheritance will catch all the cases anyway.
*
* @return the new adapter.
* @see net.opengis.wfs.DeleteElementType
* @generated
*/
public Adapter createDeleteElementTypeAdapter() {
return null;
}
/**
* Creates a new adapter for an object of class '{@link net.opengis.wfs.FeatureCollectionType Feature Collection Type}'.
*
* This default implementation returns null so that we can easily ignore cases;
* it's useful to ignore a case when inheritance will catch all the cases anyway.
*
* @return the new adapter.
* @see net.opengis.wfs.FeatureCollectionType
* @generated
*/
public Adapter createFeatureCollectionTypeAdapter() {
return null;
}
/**
* Creates a new adapter for an object of class '{@link net.opengis.wfs.UpdateElementType Update Element Type}'.
*
* This default implementation returns null so that we can easily ignore cases;
* it's useful to ignore a case when inheritance will catch all the cases anyway.
*
* @return the new adapter.
* @see net.opengis.wfs.UpdateElementType
* @generated
*/
public Adapter createUpdateElementTypeAdapter() {
return null;
}
/**
* Creates a new adapter for the default case.
*
* This default implementation returns null.
*
* @return the new adapter.
* @generated
*/
public Adapter createEObjectAdapter() {
return null;
}
} //WfsvAdapterFactory
© 2015 - 2025 Weber Informatics LLC | Privacy Policy