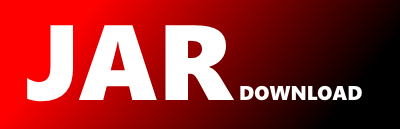
org.github.evenjn.align.alphabet.TupleAlignmentAlphabetDeserializer Maven / Gradle / Ivy
/**
*
* Copyright 2016 Marco Trevisan
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*
*/
package org.github.evenjn.align.alphabet;
import java.util.Vector;
import java.util.function.Function;
import java.util.regex.Pattern;
import org.github.evenjn.knit.KnittingTuple;
import org.github.evenjn.yarn.SkipException;
import org.github.evenjn.yarn.SkipFold;
public class TupleAlignmentAlphabetDeserializer
implements
SkipFold> {
private final Function a_deserializer;
private final Function b_deserializer;
private final TupleAlignmentAlphabet result =
new TupleAlignmentAlphabet<>( );
private final static Pattern splitter = Pattern.compile( "," );
private boolean closed = false;
@Override
public TupleAlignmentAlphabet end( ) {
if ( closed ) {
throw new IllegalStateException( );
}
closed = true;
return result;
}
public TupleAlignmentAlphabetDeserializer(
Function a_deserializer,
Function b_deserializer) {
this.a_deserializer = a_deserializer;
this.b_deserializer = b_deserializer;
}
@Override
public TupleAlignmentAlphabet next( String object )
throws SkipException {
if ( closed ) {
throw new IllegalStateException( );
}
String[] split = splitter.split( object );
int id = Integer.parseInt( split[0] );
if ( result.size( ) != id ) {
throw new IllegalStateException( );
}
SymbolAbove sa = a_deserializer.apply( split[1] );
Vector below = new Vector<>( );
for ( int i = 2; i < split.length; i++ ) {
below.add( b_deserializer.apply( split[i] ) );
}
TupleAlignmentAlphabetPair cp = new TupleAlignmentAlphabetPair<>( );
cp.above = sa;
cp.below = KnittingTuple.wrap( below );
result.add( cp );
throw SkipException.neo;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy