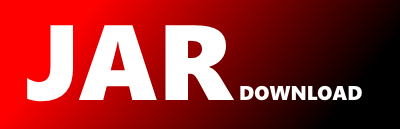
org.glamey.scaffold.json.JsonMapper Maven / Gradle / Ivy
package org.glamey.scaffold.json;
import com.fasterxml.jackson.core.JsonProcessingException;
import com.fasterxml.jackson.core.type.TypeReference;
import com.fasterxml.jackson.databind.DeserializationFeature;
import com.fasterxml.jackson.databind.JavaType;
import com.fasterxml.jackson.databind.ObjectMapper;
import com.fasterxml.jackson.databind.SerializationFeature;
import com.fasterxml.jackson.databind.util.JSONPObject;
import com.google.common.base.Preconditions;
import org.apache.commons.lang3.StringUtils;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import java.io.IOException;
import java.io.Writer;
/**
* 参考文档地址:http://wiki.fasterxml.com/JacksonInFiveMinutes
*
* @author zhouyang.zhou
*/
public class JsonMapper {
private static final Logger LOGGER = LoggerFactory.getLogger(JsonMapper.class);
private static ObjectMapper mapper = null;
private JsonMapper() {
}
/**
* 初始化
*
* @return {@link JsonMapper}
*/
public static JsonMapper create() {
LOGGER.debug("ObjectMapper init...");
mapper = new ObjectMapper();
// mapper.setSerializationInclusion(JsonInclude.Include.NON_NULL); //属性为NULL 不序列化
// mapper.setSerializationInclusion(JsonInclude.Include.NON_DEFAULT); //属性为默认值不序列化
// mapper.setSerializationInclusion(JsonInclude.Include.NON_EMPTY); // 属性为 空(“”) 或者为 NULL 都不序列化
mapper.configure(SerializationFeature.WRITE_DATE_KEYS_AS_TIMESTAMPS, false);
mapper.configure(DeserializationFeature.FAIL_ON_UNKNOWN_PROPERTIES, false);
// mapper.setDateFormat(new java.text.SimpleDateFormat("yyyy-MM-dd HH:mm:ss"));
return new JsonMapper();
}
/**
* 如果对象为Null, 返回"null".
* 如果集合为空集合, 返回"[]".
*
* @param data data
* @param the data type
* @return 返回对象的json字符串
*/
public String toJson(T data) {
try {
return mapper.writeValueAsString(data);
} catch (IOException e) {
throw new RuntimeException("write to json string error:" + data, e);
}
}
/**
* 格式化生成的json
*
* @param data the object to json
* @param the data type
* @return to pretty json result
*/
public String toJsonPretty(T data) {
try {
//代码格式化
return mapper.writerWithDefaultPrettyPrinter().writeValueAsString(data);
} catch (IOException e) {
throw new RuntimeException("write to json string error:" + data, e);
}
}
/**
* @param writer writer
* @param data data
* @param the data type
* @throws IOException 抛出IO异常
*/
public void write(Writer writer, T data) throws IOException {
Preconditions.checkNotNull(writer);
try {
mapper.writeValue(writer, data);
} catch (JsonProcessingException e) {
throw new RuntimeException("jackson format error: " + data.getClass(), e);
}
}
/**
* 反序列化POJO或简单{@code Collection如List}.
*
* 如果JSON字符串为Null或"null"字符串, 返回Null.
* 如果JSON字符串为"[]", 返回空集合.
*
* 如需反序列化复杂Collection如{@code List}, 请使用
*
* @param content the content
* @param clazz the class
* @param the object type will be return
* @return {@code T}
* @see #fromJson(String, JavaType)
*/
public T fromJson(String content, Class clazz) {
if (StringUtils.isEmpty(content)) {
return null;
}
try {
return mapper.readValue(content, clazz);
} catch (IOException e) {
throw new RuntimeException("parse json content error:" + content, e);
}
}
/**
* 反序列化POJO或简单{@code Collection如List}.
*
* 如果JSON字符串为Null或"null"字符串, 返回Null.
* 如果JSON字符串为"[]", 返回空集合.
*
* 如需反序列化复杂Collection如{@code List}, 请使用
*
* @param buffer the bytes content
* @param clazz the class
* @param the object type will be return
* @return {@code T}
* @see #fromJson(String, JavaType)
*/
public T fromJson(byte[] buffer, Class clazz) {
if (buffer == null) {
return null;
}
try {
return mapper.readValue(buffer, clazz);
} catch (IOException e) {
throw new RuntimeException("parse bytes content error:", e);
}
}
/**
* 反序列化复杂Collection如{@code List}, 先使用createCollectionType构造类型,然后调用本函数.
*
* @param content the content
* @param javaType java type
* @param the object type will be return
* @return the object class
* @see #createCollectionType(Class, Class...)
*/
@SuppressWarnings("unchecked")
public T fromJson(String content, JavaType javaType) {
if (StringUtils.isEmpty(content)) {
return null;
}
try {
return mapper.readValue(content, javaType);
} catch (IOException e) {
throw new RuntimeException("parse json content error:" + content, e);
}
}
/**
* 反序列化复杂Collection如{@code List}, 先使用createCollectionType构造类型,然后调用本函数.
*
* @param buffer the json bytes
* @param javaType java type
* @param the object type will be return
* @return the object class
* @see #createCollectionType(Class, Class...)
*/
public T fromJson(byte[] buffer, JavaType javaType) {
if (buffer == null)
return null;
try {
return mapper.readValue(buffer, javaType);
} catch (IOException e) {
throw new RuntimeException("parse byte error:", e);
}
}
/**
* 构造的Collection Type如:
* {@code ArrayList}, 则调用constructCollectionType(ArrayList.class,Bean.class)
* {@code HashMap}, 则调用(HashMap.class,String.class, Bean.class)
*
* @param collectionClass collection class
* @param elementClasses the elements class
* @return java type
*/
public JavaType createCollectionType(Class> collectionClass, Class>... elementClasses) {
return mapper.getTypeFactory().constructParametricType(collectionClass, elementClasses);
}
/**
* 可以通过 {@code new TypeReference