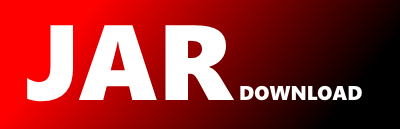
org.glassfish.grizzly.servlet.CookieWrapper Maven / Gradle / Ivy
/*
* Copyright (c) 2008, 2020 Oracle and/or its affiliates. All rights reserved.
*
* This program and the accompanying materials are made available under the
* terms of the Eclipse Public License v. 2.0, which is available at
* http://www.eclipse.org/legal/epl-2.0.
*
* This Source Code may also be made available under the following Secondary
* Licenses when the conditions for such availability set forth in the
* Eclipse Public License v. 2.0 are satisfied: GNU General Public License,
* version 2 with the GNU Classpath Exception, which is available at
* https://www.gnu.org/software/classpath/license.html.
*
* SPDX-License-Identifier: EPL-2.0 OR GPL-2.0 WITH Classpath-exception-2.0
*/
package org.glassfish.grizzly.servlet;
import org.glassfish.grizzly.http.Cookie;
/**
* Simple Wrapper around {@link Cookie}.
*
* @author jfarcand
*/
public class CookieWrapper extends Cookie {
/**
* Constructs a cookie with a specified name and value.
*
*
* The name must conform to RFC 2109. That means it can contain only ASCII alphanumeric characters and cannot contain
* commas, semicolons, or white space or begin with a $ character. The cookie's name cannot be changed after creation.
*
*
* The value can be anything the server chooses to send. Its value is probably of interest only to the server. The
* cookie's value can be changed after creation with the setValue
method.
*
*
* By default, cookies are created according to the Netscape cookie specification. The version can be changed with the
* setVersion
method.
*
*
* @param name a String
specifying the name of the cookie
*
* @param value a String
specifying the value of the cookie
*
* @throws IllegalArgumentException if the cookie name contains illegal characters (for example, a comma, space, or
* semicolon) or it is one of the tokens reserved for use by the cookie protocol
* @see #setValue
* @see #setVersion
*
*/
public CookieWrapper(String name, String value) {
super(name, value);
}
private jakarta.servlet.http.Cookie wrappedCookie = null;
/**
*
* Specifies a comment that describes a cookie's purpose. The comment is useful if the browser presents the cookie to
* the user. Comments are not supported by Netscape Version 0 cookies.
*
* @param purpose a String
specifying the comment to display to the user
*
* @see #getComment
*
*/
@Override
public void setComment(String purpose) {
wrappedCookie.setComment(purpose);
}
/**
* Returns the comment describing the purpose of this cookie, or null
if the cookie has no comment.
*
* @return a String
containing the comment, or null
if none
*
* @see #setComment
*
*/
@Override
public String getComment() {
return wrappedCookie.getComment();
}
/**
*
* Specifies the domain within which this cookie should be presented.
*
*
* The form of the domain name is specified by RFC 2109. A domain name begins with a dot (.foo.com
) and
* means that the cookie is visible to servers in a specified Domain Name System (DNS) zone (for example,
* www.foo.com
, but not a.b.foo.com
). By default, cookies are only returned to the server that
* sent them.
*
*
* @param pattern a String
containing the domain name within which this cookie is visible; form is
* according to RFC 2109
*
* @see #getDomain
*
*/
@Override
public void setDomain(String pattern) {
wrappedCookie.setDomain(pattern);
}
/**
* Returns the domain name set for this cookie. The form of the domain name is set by RFC 2109.
*
* @return a String
containing the domain name
*
* @see #setDomain
*
*/
@Override
public String getDomain() {
return wrappedCookie.getDomain();
}
/**
* Sets the maximum age of the cookie in seconds.
*
*
* A positive value indicates that the cookie will expire after that many seconds have passed. Note that the value is
* the maximum age when the cookie will expire, not the cookie's current age.
*
*
* A negative value means that the cookie is not stored persistently and will be deleted when the Web browser exits. A
* zero value causes the cookie to be deleted.
*
* @param expiry an integer specifying the maximum age of the cookie in seconds; if negative, means the cookie is not
* stored; if zero, deletes the cookie
*
*
* @see #getMaxAge
*
*/
@Override
public void setMaxAge(int expiry) {
wrappedCookie.setMaxAge(expiry);
}
/**
* Returns the maximum age of the cookie, specified in seconds, By default, -1
indicating the cookie will
* persist until browser shutdown.
*
*
* @return an integer specifying the maximum age of the cookie in seconds; if negative, means the cookie persists until
* browser shutdown
*
*
* @see #setMaxAge
*
*/
@Override
public int getMaxAge() {
return wrappedCookie.getMaxAge();
}
/**
* Specifies a path for the cookie to which the client should return the cookie.
*
*
* The cookie is visible to all the pages in the directory you specify, and all the pages in that directory's
* subdirectories. A cookie's path must include the servlet that set the cookie, for example, /catalog, which
* makes the cookie visible to all directories on the server under /catalog.
*
*
* Consult RFC 2109 (available on the Internet) for more information on setting path names for cookies.
*
*
* @param uri a String
specifying a path
*
*
* @see #getPath
*
*/
@Override
public void setPath(String uri) {
wrappedCookie.setPath(uri);
}
/**
* Returns the path on the server to which the browser returns this cookie. The cookie is visible to all subpaths on the
* server.
*
*
* @return a String
specifying a path that contains a servlet name, for example, /catalog
*
* @see #setPath
*
*/
@Override
public String getPath() {
return wrappedCookie.getPath();
}
/**
* Indicates to the browser whether the cookie should only be sent using a secure protocol, such as HTTPS or SSL.
*
*
* The default value is false.
*
* @param flag if true, sends the cookie from the browser to the server only when using a secure protocol; if
* false, sent on any protocol
*
* @see #isSecure()
*
*/
@Override
public void setSecure(boolean flag) {
wrappedCookie.setSecure(flag);
}
/**
* Returns true if the browser is sending cookies only over a secure protocol, or false if the browser
* can send cookies using any protocol.
*
* @return true if the browser uses a secure protocol; otherwise, true
*
* @see #setSecure
*
*/
@Override
public boolean isSecure() {
return wrappedCookie.getSecure();
}
/**
* Returns the name of the cookie. The name cannot be changed after creation.
*
* @return a String
specifying the cookie's name
*
*/
@Override
public String getName() {
return wrappedCookie.getName();
}
/**
*
* Assigns a new value to a cookie after the cookie is created. If you use a binary value, you may want to use BASE64
* encoding.
*
*
* With Version 0 cookies, values should not contain white space, brackets, parentheses, equals signs, commas, double
* quotes, slashes, question marks, at signs, colons, and semicolons. Empty values may not behave the same way on all
* browsers.
*
* @param newValue a String
specifying the new value
*
*
* @see #getValue
* @see Cookie
*
*/
@Override
public void setValue(String newValue) {
wrappedCookie.setValue(newValue);
}
/**
* Returns the value of the cookie.
*
* @return a String
containing the cookie's present value
*
* @see #setValue
* @see Cookie
*
*/
@Override
public String getValue() {
return wrappedCookie.getValue();
}
/**
* Returns the version of the protocol this cookie complies with. Version 1 complies with RFC 2109, and version 0
* complies with the original cookie specification drafted by Netscape. Cookies provided by a browser use and identify
* the browser's cookie version.
*
*
* @return 0 if the cookie complies with the original Netscape specification; 1 if the cookie complies with RFC 2109
*
* @see #setVersion
*
*/
@Override
public int getVersion() {
return wrappedCookie.getVersion();
}
/**
* Sets the version of the cookie protocol this cookie complies with. Version 0 complies with the original Netscape
* cookie specification. Version 1 complies with RFC 2109.
*
*
* Since RFC 2109 is still somewhat new, consider version 1 as experimental; do not use it yet on production sites.
*
*
* @param v 0 if the cookie should comply with the original Netscape specification; 1 if the cookie should comply with
* RFC 2109
*
* @see #getVersion
*
*/
@Override
public void setVersion(int v) {
wrappedCookie.setVersion(v);
}
/**
* {@inheritDoc}
*/
@Override
public boolean isHttpOnly() {
return wrappedCookie.isHttpOnly();
}
/**
* {@inheritDoc}
*/
@Override
public void setHttpOnly(boolean isHttpOnly) {
wrappedCookie.setHttpOnly(isHttpOnly);
}
@SuppressWarnings("UnusedDeclaration")
public Object cloneCookie() {
return wrappedCookie.clone();
}
public jakarta.servlet.http.Cookie getWrappedCookie() {
return wrappedCookie;
}
public void setWrappedCookie(jakarta.servlet.http.Cookie wrappedCookie) {
this.wrappedCookie = wrappedCookie;
}
}