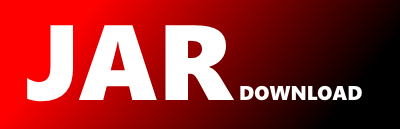
org.glassfish.jersey.examples.xmlmoxy.beans.Customer Maven / Gradle / Ivy
The newest version!
/*
* Copyright (c) 2010, 2020 Oracle and/or its affiliates. All rights reserved.
*
* This program and the accompanying materials are made available under the
* terms of the Eclipse Distribution License v. 1.0, which is available at
* http://www.eclipse.org/org/documents/edl-v10.php.
*
* SPDX-License-Identifier: BSD-3-Clause
*/
package org.glassfish.jersey.examples.xmlmoxy.beans;
import java.util.ArrayList;
import java.util.List;
import jakarta.xml.bind.annotation.XmlRootElement;
import jakarta.xml.bind.annotation.XmlType;
import org.eclipse.persistence.oxm.annotations.XmlPath;
/**
*
* @author Jakub Podlesak
*/
@XmlRootElement
@XmlType(propOrder = {"name", "address", "phoneNumbers"})
public class Customer {
private String name;
private Address address;
private List phoneNumbers;
public Customer() {
phoneNumbers = new ArrayList();
}
@XmlPath("personal-info/name/text()")
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
@XmlPath("contact-info/address")
public Address getAddress() {
return address;
}
public void setAddress(Address address) {
this.address = address;
}
@XmlPath("contact-info/phone-number")
public List getPhoneNumbers() {
return phoneNumbers;
}
public void setPhoneNumbers(List phoneNumbers) {
this.phoneNumbers = phoneNumbers;
}
@Override
public boolean equals(Object obj) {
if (obj == null) {
return false;
}
if (getClass() != obj.getClass()) {
return false;
}
final Customer other = (Customer) obj;
if ((this.name == null) ? (other.name != null) : !this.name.equals(other.name)) {
return false;
}
if (this.address != other.address && (this.address == null || !this.address.equals(other.address))) {
return false;
}
if (this.phoneNumbers != other.phoneNumbers && (this.phoneNumbers == null || !this.phoneNumbers
.equals(other.phoneNumbers))) {
return false;
}
return true;
}
@Override
public int hashCode() {
int hash = 7;
hash = 97 * hash + (this.name != null ? this.name.hashCode() : 0);
hash = 97 * hash + (this.address != null ? this.address.hashCode() : 0);
hash = 97 * hash + (this.phoneNumbers != null ? this.phoneNumbers.hashCode() : 0);
return hash;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy